How Can You Effectively Exit a Function in Python?
When coding in Python, functions serve as the building blocks of reusable code, allowing developers to encapsulate logic and streamline their programs. However, there are moments when you may need to exit a function prematurely, whether due to a specific condition being met or an error occurring. Understanding how to effectively exit a function is crucial for maintaining control flow and ensuring that your program behaves as expected. In this article, we will explore the various methods for exiting a function in Python, providing you with the tools to manage your code’s execution seamlessly.
Exiting a function can be achieved through several mechanisms, each suited for different scenarios. The most common method is using the `return` statement, which not only terminates the function but can also pass back a value to the caller. However, there are times when you might want to exit a function without returning a value, such as when handling exceptions or breaking out of nested loops. In these cases, understanding the nuances of control flow in Python becomes essential.
Additionally, the context in which you need to exit a function can influence your approach. Whether you’re dealing with simple functions, complex algorithms, or error handling, knowing the right techniques will empower you to write cleaner, more efficient code. As we delve deeper into this topic, you’ll discover practical examples
Using the return Statement
The primary method for exiting a function in Python is by using the `return` statement. When the `return` statement is executed, the function stops executing and returns a value to the caller. If no value is specified, `None` is returned by default.
“`python
def calculate_sum(a, b):
return a + b
result = calculate_sum(3, 5) result will be 8
“`
In the example above, the function `calculate_sum` exits when the `return` statement is executed, returning the sum of `a` and `b`.
Exiting Early with Conditional Returns
You can also use conditional statements to exit a function early based on certain conditions. This practice can enhance code readability and prevent unnecessary computations.
“`python
def check_positive(number):
if number < 0:
return "Negative number"
return "Positive number"
print(check_positive(-5)) Output: Negative number
print(check_positive(10)) Output: Positive number
```
In this example, if the input number is negative, the function exits early with a corresponding message.
Using Exceptions for Exiting Functions
In some situations, you may want to exit a function due to an error or exceptional condition. Python allows you to raise exceptions, which will immediately terminate the function execution.
“`python
def divide(a, b):
if b == 0:
raise ValueError(“Cannot divide by zero”)
return a / b
try:
result = divide(10, 0)
except ValueError as e:
print(e) Output: Cannot divide by zero
“`
In this case, if the second argument is zero, a `ValueError` is raised, and the function exits without returning a value.
Using the return Statement with Multiple Exit Points
It’s common to have multiple `return` statements in a single function. This approach provides flexibility in exiting the function based on different conditions.
“`python
def evaluate_score(score):
if score < 0:
return "Invalid score"
if score < 50:
return "Fail"
return "Pass"
print(evaluate_score(75)) Output: Pass
```
Here, the function can exit at various points based on the input score.
Comparison of Function Exit Methods
The following table summarizes different methods for exiting a function in Python:
Method | Description |
---|---|
return | Exits the function and optionally returns a value. |
Conditional return | Exits early based on specific conditions. |
Raise Exception | Exits the function due to an error or exceptional condition. |
Understanding these methods allows for effective function management in Python, enhancing both functionality and maintainability of the code.
Using the `return` Statement
In Python, the most common way to exit a function is by using the `return` statement. This not only exits the function but can also return a value to the caller.
- Basic Syntax:
“`python
def my_function():
return
“`
- Returning a Value:
“`python
def add(a, b):
return a + b
result = add(3, 5) result will be 8
“`
When `return` is executed, the function terminates immediately, and control is passed back to the calling code.
Using `break` and `continue` Statements
The `break` and `continue` statements are primarily used in loops. However, they can be used in conjunction with functions to alter the flow of execution.
- Using `break`:
If a function contains a loop, `break` can be used to exit that loop, effectively terminating the function if it’s the only loop present.
“`python
def find_value(values, target):
for value in values:
if value == target:
print(“Value found!”)
break Exits the loop
“`
- Using `continue`:
While `continue` does not exit a function, it can skip the current iteration of a loop within a function.
“`python
def process_items(items):
for item in items:
if item < 0:
continue Skips negative items
print(item)
```
Raising Exceptions
Another method to exit a function is by raising an exception. This is useful when an error condition arises and you want to terminate the function immediately.
- Basic Usage:
“`python
def check_value(value):
if value < 0:
raise ValueError("Value must be non-negative")
```
When an exception is raised, the function exits, and control is transferred to the nearest exception handler.
Using `sys.exit()` for Immediate Termination
For scenarios where you want to exit not just the function but the entire script, `sys.exit()` can be employed. This is typically reserved for situations requiring immediate termination of the program.
- Example:
“`python
import sys
def terminate_script():
print(“Exiting the script.”)
sys.exit()
“`
This function will halt the execution of the script entirely when called.
Returning `None` Implicitly
If a function does not contain a `return` statement, it will implicitly return `None` when it reaches the end. This behavior can be leveraged to exit a function.
- Example:
“`python
def no_return():
print(“This function will return None.”)
result = no_return() result will be None
“`
This method is often used in functions where the primary purpose is to perform an action rather than to compute a value.
Exiting a function in Python can be accomplished through various means, including using the `return` statement, breaking from loops, raising exceptions, or invoking `sys.exit()`. Understanding these methods allows for greater control over function execution and flow.
Expert Insights on Exiting Functions in Python
Dr. Emily Carter (Senior Python Developer, Tech Innovations Inc.). “Exiting a function in Python can be accomplished using the `return` statement, which not only stops the execution of the function but also allows you to send a value back to the caller. This is essential for maintaining control flow and ensuring that your code remains efficient and readable.”
Michael Chen (Lead Software Engineer, CodeCraft Solutions). “In Python, if you need to exit a function prematurely based on a condition, the `return` statement can be used without any value. This effectively terminates the function’s execution and returns `None` by default, which is a useful technique for handling error states or exceptional conditions.”
Sarah Thompson (Python Programming Instructor, Code Academy). “Understanding how to exit a function properly is crucial for writing clean and maintainable code. Using `return` not only exits the function but also clarifies the intent of your code, making it easier for others to understand the flow of your program.”
Frequently Asked Questions (FAQs)
How do you exit a function in Python?
You can exit a function in Python using the `return` statement. This immediately terminates the function and optionally returns a value to the caller.
What happens if you use return without a value?
Using `return` without a value exits the function and returns `None` by default. This indicates that the function has completed without producing a specific output.
Can you exit a function early based on a condition?
Yes, you can use an `if` statement to check a condition and call `return` to exit the function early if the condition is met.
Is it possible to exit a function using an exception?
Yes, you can raise an exception to exit a function. This will terminate the function and propagate the exception up the call stack unless it is caught by a surrounding `try` block.
What is the difference between return and break in a function?
The `return` statement exits the function and optionally returns a value, while `break` is used to exit loops. `break` cannot be used to exit a function directly.
Can a function return multiple values in Python?
Yes, a function can return multiple values by separating them with commas. Python will return these values as a tuple, which can be unpacked by the caller.
Exiting a function in Python can be accomplished using several methods, each serving different purposes depending on the context of the function’s execution. The most common way to exit a function is by using the `return` statement. This statement not only terminates the function but also allows you to send a value back to the caller. If no value is specified, the function will return `None` by default.
In addition to the `return` statement, one can utilize exceptions to exit a function abruptly. If an error condition arises, raising an exception will immediately halt the function’s execution and propagate the error up the call stack. This is particularly useful for handling unexpected situations without returning a value.
Another method to exit a function is through the use of the `break` or `continue` statements within loops. While these statements are primarily used to control loop execution, they can also influence the flow of a function if the function contains loops. However, it is essential to understand that these statements do not exit the function itself but rather affect loop behavior within the function.
In summary, understanding how to exit a function in Python is crucial for effective programming. The `return` statement is the standard approach for normal function termination
Author Profile
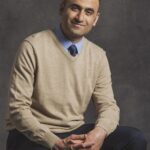
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?