How Can You Effectively Exit Python: Tips and Tricks?
How to Exit Python: A Comprehensive Guide
As you dive into the world of Python programming, you may find yourself immersed in the intricacies of code, algorithms, and data manipulation. Whether you’re a seasoned developer or a curious beginner, there comes a time when you need to gracefully exit your Python environment. Understanding how to exit Python effectively is essential for maintaining a smooth workflow and ensuring that your programs run as intended. In this article, we will explore the various methods to exit Python, whether you are working in an interactive shell, a script, or an integrated development environment (IDE).
Exiting Python might seem like a straightforward task, but it can vary depending on your working environment and specific needs. For those using the Python interactive shell, there are simple commands that can be executed to terminate the session. Meanwhile, when running scripts, you may want to ensure that your program ends cleanly after completing its tasks. Additionally, integrated development environments often provide their own shortcuts and methods for exiting, which can enhance your coding efficiency.
In the following sections, we will delve into the different ways to exit Python, covering everything from basic commands to more advanced techniques. Whether you are looking to close a terminal session, stop a running script, or navigate the exit options in your favorite
Exiting Python from the Command Line
To exit Python when running in the command line interface, you can use several methods depending on your needs and the environment you are in. Here are the most common ways:
- Using the exit() function: This is a built-in function that you can call to exit the Python interpreter.
- Using the quit() function: Similar to exit(), this function serves the same purpose and can be used interchangeably.
- Keyboard shortcut: If you are in an interactive session, you can simply press `Ctrl + Z` on Windows or `Ctrl + D` on Unix/Linux-based systems to exit.
Exiting Python from Scripts
When running Python scripts, you may want to programmatically exit the script under specific conditions. This can be achieved using the `sys.exit()` function from the `sys` module. Here’s how to implement it:
“`python
import sys
Some code logic here
if some_condition:
sys.exit(“Exiting due to some condition”)
“`
- You can specify an exit status code with `sys.exit()`, where `0` generally indicates a successful exit, and any non-zero value indicates an error.
Exiting Python in Integrated Development Environments (IDEs)
When working within an IDE such as PyCharm or Visual Studio Code, the methods to exit the Python interpreter may vary slightly:
- Stop Button: Most IDEs have a stop button in the console or run window that allows you to terminate the running script.
- Keyboard Shortcuts: Commonly, `Ctrl + C` can be used to interrupt a running script in the terminal or console.
Exit Codes in Python
Understanding exit codes is crucial for debugging and ensuring your scripts behave as expected. Here’s a simple table summarizing common exit codes:
Exit Code | Description |
---|---|
0 | Successful termination |
1 | General error |
2 | Misuse of shell builtins (according to Bash documentation) |
127 | Command not found |
130 | Script terminated by Control-C |
By using these methods and understanding exit codes, you can effectively manage the termination of Python programs in various environments.
Exiting Python in Different Environments
Exiting Python can vary based on the environment in which you are working, such as the command line, an integrated development environment (IDE), or a Jupyter notebook. Below are the methods applicable to each environment.
Using the Command Line
When working in the Python interactive shell or a script run from the command line, you can exit Python using the following methods:
- Keyboard Shortcut:
- Press `Ctrl + Z` (Windows) or `Ctrl + D` (Linux/Mac) and then hit `Enter`.
- Exit Command:
- Type `exit()` and press `Enter`.
- Alternatively, you can use `quit()` to achieve the same result.
- System Exit:
- You can also use the `sys` module to exit Python:
“`python
import sys
sys.exit()
“`
Exiting Python in Integrated Development Environments (IDEs)
Different IDEs may have specific commands or buttons for exiting Python. Here are some common ones:
IDE | Exit Method |
---|---|
PyCharm | Click on the “Stop” button in the run panel or use `Ctrl + F2`. |
Visual Studio Code | Click on the “Stop” button in the terminal or use `Ctrl + C`. |
Anaconda Spyder | Use the “Stop” button in the console or choose “Restart Kernel” from the menu. |
Exiting Jupyter Notebooks
In Jupyter notebooks, exiting can be done by:
- Stopping the Kernel:
- Click on “Kernel” in the menu and select “Shutdown” to stop the execution of the notebook.
- Closing the Notebook:
- Simply close the browser tab or window. This does not terminate the kernel but disconnects the interface.
- Using Command Mode:
- Press `Esc` to enter command mode, then hit `0` twice to restart or stop the kernel.
Best Practices for Exiting Python
When exiting Python, consider the following best practices to ensure that your work is saved and that you exit cleanly:
- Save your Work:
- Always save your files before exiting to avoid losing any unsaved changes.
- Close Open Connections:
- If you have any open database connections or file handlers, ensure they are properly closed before exiting.
- Terminate Long-Running Processes:
- If your script is running a long process, consider implementing a way to gracefully terminate it, such as using signal handling or checking for user input.
By following these methods and best practices, you can effectively exit Python in a variety of environments while ensuring that your work is preserved.
Expert Insights on Exiting Python Properly
Dr. Emily Carter (Senior Software Engineer, CodeMasters Inc.). “Exiting Python can be accomplished through several methods, including using the exit() function or the sys.exit() command. It is essential to choose the appropriate method depending on the context of your application, especially in larger projects where graceful shutdowns are necessary.”
James Lin (Python Developer Advocate, Tech Innovations). “For beginners, the simplest way to exit a Python script is by using the keyboard shortcut Ctrl + C in the terminal. However, for more controlled exits, utilizing the sys.exit() function allows for the passing of an exit status, which can be crucial for debugging and process management.”
Sarah Thompson (Lead Python Instructor, Code Academy). “When teaching Python, I emphasize the importance of understanding how to exit scripts properly. Using the exit() function can help ensure that all resources are released and that the program terminates cleanly, which is particularly important in applications that handle files or network connections.”
Frequently Asked Questions (FAQs)
How do I exit a Python script from the command line?
To exit a Python script running in the command line, you can simply type `exit()` or `quit()` and press Enter. Alternatively, you can use `Ctrl + Z` on Windows or `Ctrl + D` on Unix/Linux systems to terminate the session.
What is the keyboard shortcut to exit the Python interactive shell?
In the Python interactive shell, you can exit by pressing `Ctrl + Z` followed by Enter on Windows, or `Ctrl + D` on Unix/Linux systems.
Can I exit a Python program using a specific exit code?
Yes, you can exit a Python program with a specific exit code by using `sys.exit(code)`, where `code` is an integer. A code of `0` typically indicates successful termination, while any non-zero code indicates an error.
How can I programmatically exit a Python script?
You can programmatically exit a Python script by importing the `sys` module and calling `sys.exit()`. You can optionally pass an exit code or message as an argument.
Is there a way to exit Python without using the exit commands?
Yes, you can exit Python by terminating the process through your operating system’s task manager or by closing the terminal or command prompt window in which the Python script is running.
What happens if I don’t exit a Python script properly?
If you do not exit a Python script properly, resources such as file handles and network connections may not be released, potentially leading to memory leaks or other unintended behavior in your application.
Exiting Python can be accomplished through several methods, each suitable for different scenarios. The most common way to exit an interactive Python session is by using the `exit()` or `quit()` functions. These functions are user-friendly and designed specifically for terminating the Python interpreter gracefully. Additionally, the keyboard shortcut Ctrl + Z (on Windows) or Ctrl + D (on Unix/Linux) can also be employed to exit the session quickly.
For script execution, the `sys.exit()` function from the `sys` module is often utilized. This method allows for more control, as it can accept an exit status code, which can be useful for signaling success or failure of the script execution. It is important to note that using `sys.exit()` will raise a `SystemExit` exception, which can be caught if necessary, allowing for cleanup or logging before exiting.
In summary, understanding the various methods to exit Python is essential for effective programming and debugging. Whether working in an interactive shell or running scripts, knowing how to exit properly ensures that resources are released and any necessary finalization tasks are completed. By leveraging these techniques, users can maintain better control over their Python environments and enhance their overall coding experience.
Author Profile
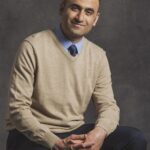
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?