How Can You Properly Exit a Python Program?
When diving into the world of Python programming, one of the fundamental skills every developer must master is how to effectively manage the lifecycle of their applications. Whether you’re running a simple script or developing a complex software solution, knowing how to exit a Python program gracefully is crucial. This seemingly straightforward task can have significant implications for resource management, data integrity, and user experience. In this article, we will explore various methods to exit a Python program, ensuring that you have the tools and knowledge to handle this essential aspect of programming with confidence.
Exiting a Python program can be as simple as hitting the stop button in your IDE or terminal, but there are more nuanced approaches that can be employed depending on your specific needs. From using built-in functions to handling exceptions, Python offers a variety of ways to terminate a program safely and effectively. Understanding these methods not only enhances your coding skills but also prepares you for scenarios where you might need to exit a program under specific conditions or in response to user input.
As we delve deeper into this topic, we will examine the different techniques available for exiting a Python program, including the use of the `exit()` function, the `sys.exit()` method, and the implications of using exceptions. By the end of this article, you’ll be equipped with a comprehensive understanding
Using the exit() Function
The simplest way to exit a Python program is by using the built-in `exit()` function. This function is part of the `sys` module, which provides access to some variables used or maintained by the interpreter. To use it, you must first import the module. Here’s an example of how to do this:
python
import sys
sys.exit()
The `exit()` function can also take an optional argument that can specify an exit status. By convention, a status of `0` indicates a successful termination, while any non-zero value indicates an error.
Using the quit() Function
Similar to `exit()`, the `quit()` function can also be used to terminate a Python program. It behaves in the same way and is intended for use in interactive sessions. Here’s how you can use it:
python
quit()
Just like `exit()`, it can take an optional exit status argument. While `quit()` is more user-friendly for beginners, it is not recommended for use in scripts, as it is primarily designed for interactive use.
Raising SystemExit Exception
Another method to exit a Python program is by raising the `SystemExit` exception. This approach allows you to exit the program with a specific exit code. Here’s how you can implement it:
python
raise SystemExit(0)
This method provides more control over the exit process and can be useful in situations where you need to perform some cleanup or logging before exiting.
Using os._exit() for Immediate Termination
The `os._exit()` function can be used for immediate termination of the program without calling cleanup handlers, flushing stdio buffers, etc. This function is typically used in child processes after a fork. Here’s an example:
python
import os
os._exit(0)
This function is less common in regular scripts but is crucial in specific scenarios where immediate termination is necessary.
Comparison of Exit Methods
To summarize the various methods for exiting a Python program, the following table outlines key differences:
Method | Module | Purpose | Exit Status |
---|---|---|---|
exit() | sys | General exit | Can specify |
quit() | sys | Interactive sessions | Can specify |
raise SystemExit | – | Programmatically raise exit | Can specify |
os._exit() | os | Immediate termination | Can specify |
Each method has its own use case and should be chosen based on the specific requirements of the program being developed.
Using the Exit Function
In Python, the simplest way to exit a program is by using the built-in `exit()` function. This function is part of the `sys` module, which means you need to import it before use. The `exit()` function can take an optional argument, which is an integer that represents the exit status.
python
import sys
# Exiting with a status code of 0 (success)
sys.exit(0)
# Exiting with a status code of 1 (error)
sys.exit(1)
Using a status code is particularly useful in scripts, as it allows other programs or scripts calling your Python code to determine whether it executed successfully or encountered an error.
Using the Quit Function
Another straightforward method to terminate a Python program is by using the `quit()` function. This function behaves similarly to `exit()`, with the same ability to accept an optional exit status.
python
quit()
`quit()` is often used in interactive sessions and behaves the same way as `exit()`. However, it is advisable to use `sys.exit()` in scripts for consistency and clarity.
Using the Raise SystemExit Exception
For more advanced users, you can also terminate a Python program by raising the `SystemExit` exception. This approach offers greater control, as you can raise this exception in your code wherever necessary.
python
raise SystemExit(“Exiting the program with a message.”)
This method is particularly useful when you want to provide a custom exit message or to terminate the program conditionally based on certain criteria.
Using Keyboard Interrupts
When running a Python program in a console or terminal, you can also stop execution manually by sending a keyboard interrupt. This is typically done by pressing `Ctrl + C`. This action raises a `KeyboardInterrupt` exception, which can be handled in the code if desired.
python
try:
while True:
pass # Simulating a long-running process
except KeyboardInterrupt:
print(“Program terminated by user.”)
This is a common practice for terminating long-running scripts safely.
Using os._exit() for Immediate Termination
In some scenarios, you may need to terminate a program immediately without cleaning up resources. The `os._exit()` function allows you to do this. This function takes an exit status as an argument and terminates the process immediately.
python
import os
os._exit(0)
This method should be used cautiously, as it does not call cleanup handlers, flush standard I/O buffers, or close files gracefully.
Summary of Exit Methods
The following table summarizes the various methods to exit a Python program:
Method | Description | Status Code |
---|---|---|
`sys.exit()` | Graceful exit with optional status code | Yes |
`quit()` | Similar to `exit()`, mainly for interactive sessions | Yes |
`raise SystemExit` | Raise an exception to exit, allows custom messages | Yes |
`KeyboardInterrupt` | Manual termination via `Ctrl + C` | No |
`os._exit()` | Immediate termination without cleanup | Yes |
Each method serves different scenarios, and the choice of which to use depends on the specific requirements of the program being developed.
Expert Insights on Exiting Python Programs Effectively
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Exiting a Python program can be accomplished using the built-in function `sys.exit()`. This method allows developers to specify an exit status, which can be particularly useful for signaling success or failure to the operating system.”
Michael Chen (Lead Python Developer, CodeCraft Solutions). “For simpler scripts, using the `exit()` function is often sufficient. However, it is important to ensure that all resources are properly released before calling this function to avoid potential memory leaks.”
Sarah Thompson (Python Programming Instructor, LearnPython Academy). “In interactive environments, such as Jupyter notebooks, one might prefer using `quit()` or `exit()`. These functions provide a user-friendly way to terminate the session without needing to import the `sys` module.”
Frequently Asked Questions (FAQs)
How can I exit a Python program using the exit() function?
You can exit a Python program by calling the `exit()` function from the `sys` module. Use `import sys` followed by `sys.exit()` to terminate the program gracefully.
What is the purpose of the quit() function in Python?
The `quit()` function serves a similar purpose as `exit()`, allowing users to terminate the program. It is primarily intended for use in interactive sessions.
Can I use the Ctrl+C keyboard shortcut to exit a Python program?
Yes, pressing Ctrl+C in the terminal will raise a KeyboardInterrupt exception, effectively terminating the running Python program.
Is there a way to exit a Python program with a specific exit code?
Yes, you can exit a Python program with a specific exit code by using `sys.exit(code)`, where `code` is an integer representing the exit status.
What happens if I do not handle exceptions when exiting a Python program?
If exceptions are not handled, the program may terminate unexpectedly, potentially leaving resources unfreed or data unsaved. It is advisable to use try-except blocks for graceful exits.
Can I use the os._exit() function to exit a Python program?
Yes, `os._exit()` can be used to exit a Python program immediately without calling cleanup handlers, which is useful in certain scenarios like child processes.
Exiting a Python program can be accomplished through various methods, each suited for different scenarios. The most common approach is to use the built-in function `exit()` or `sys.exit()`, which allows for a graceful termination of the program. This is particularly useful when you want to stop execution based on a certain condition or after completing a task. Additionally, using `raise SystemExit` can also serve as an effective way to exit a program, providing a way to specify an exit status.
In interactive environments, such as Python shells or Jupyter notebooks, the `quit()` function can be utilized to exit the session. However, it is important to note that using these functions in scripts or larger applications should be approached with caution, as they can lead to abrupt terminations that might not allow for proper cleanup of resources or saving of data.
Key takeaways include the importance of choosing the appropriate exit method based on the context of the program. For instance, in production code, it is advisable to handle exits more gracefully, ensuring that all necessary cleanup operations are performed before termination. Understanding these various methods and their implications can lead to more robust and maintainable Python applications.
Author Profile
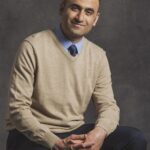
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?