How Can You Exit a Program in Python Effectively?
When programming in Python, knowing how to effectively manage the flow of your application is crucial. One common scenario developers encounter is the need to exit a program gracefully. Whether you’re building a simple script or a complex application, understanding the various methods to terminate your program can enhance both functionality and user experience. In this article, we will explore the different ways to exit a Python program, providing you with the tools you need to control your program’s lifecycle.
Exiting a Python program can be achieved through several built-in functions and methods, each serving specific use cases. For instance, using the `exit()` function is a straightforward way to terminate your script, but it’s essential to understand when and why to use it. Additionally, Python offers other mechanisms, such as raising exceptions or using the `sys.exit()` function, which can provide more control over how and when your program ends.
As we delve deeper into this topic, we will discuss the implications of exiting a program, including how to handle cleanup tasks and manage resources effectively. By understanding these concepts, you can ensure that your Python applications not only terminate correctly but also maintain a robust and user-friendly experience. Get ready to enhance your programming skills with insights into exiting Python programs!
Using the `exit()` Function
The simplest way to exit a Python program is by using the `exit()` function. This function is available in the built-in `sys` module, which means you’ll need to import it before use. The `exit()` function raises a `SystemExit` exception, which effectively terminates the program.
Here’s how you can use it:
“`python
import sys
Your code here
sys.exit()
“`
You can also pass an optional integer argument to `exit()`, where `0` indicates a successful termination, and any non-zero value typically indicates an error.
Using the `quit()` Function
Similar to `exit()`, the `quit()` function can also be used to terminate a program. It is essentially an alias for `sys.exit()`. This function is particularly useful when working in an interactive Python shell but can also be used in scripts.
Example usage:
“`python
Your code here
quit()
“`
Using the `raise SystemExit` Statement
Another way to exit a program is by raising the `SystemExit` exception directly. This method provides more control, as you can specify an exit status.
“`python
Your code here
raise SystemExit
“`
You can also pass a status code as follows:
“`python
raise SystemExit(1) Indicates an error
“`
Using `os._exit()`
For situations requiring an immediate exit without cleanup, `os._exit()` can be utilized. This method does not call cleanup handlers or flush standard I/O buffers, making it suitable for child processes.
“`python
import os
Your code here
os._exit(0)
“`
This function takes an integer argument indicating the exit status.
Exit Codes Overview
When exiting a program, it is important to understand the meaning behind exit codes. Below is a table summarizing common exit codes:
Exit Code | Description |
---|---|
0 | Success |
1 | General error |
2 | Misuse of shell builtins |
126 | Command invoked cannot execute |
127 | Command not found |
130 | Script terminated by Control-C |
Understanding these exit codes can help in debugging and managing the flow of your Python applications, especially when they are part of larger systems or scripts.
Methods to Exit a Program in Python
In Python, there are several methods to terminate a running program. The choice of method often depends on the context in which the program is running and whether it needs to exit gracefully or forcefully.
Using the `exit()` Function
The `exit()` function is part of the `sys` module and is commonly used to terminate a program. It can be used in scripts or interactive sessions. When called, it raises the `SystemExit` exception, which can be caught if needed.
- Syntax:
“`python
import sys
sys.exit([status])
“`
- Parameters:
- `status`: An optional numeric exit status (default is zero, indicating success). Non-zero values typically indicate an error.
Using the `quit()` Function
Similar to `exit()`, the `quit()` function is also used to exit Python programs. It is typically used in interactive sessions.
- Usage:
“`python
quit()
“`
Using the `os._exit()` Function
For situations requiring an immediate exit without cleanup, `os._exit()` can be employed. This function terminates the process without calling cleanup handlers, flushing standard I/O buffers, etc.
- Syntax:
“`python
import os
os._exit(status)
“`
Using the `raise SystemExit` Statement
You can also exit a program by raising the `SystemExit` exception directly. This method allows you to control the exit status more explicitly.
- Example:
“`python
raise SystemExit(1) Exits with status 1
“`
Using a Keyboard Interrupt
In a running script, pressing `Ctrl+C` will raise a `KeyboardInterrupt` exception, which can be caught to perform cleanup actions before exiting.
- Example:
“`python
try:
while True:
pass Simulate long-running process
except KeyboardInterrupt:
print(“Exiting program.”)
sys.exit(0)
“`
Graceful Exits in Functions
When you need to exit from a function but want to ensure that the program continues running, you can use `return` statements.
- Usage Example:
“`python
def my_function():
if condition_met():
return Exits the function, but not the program
“`
Exit Codes
It is common practice to return specific exit codes to indicate the reason for termination. Below is a table summarizing standard exit codes:
Exit Code | Meaning |
---|---|
0 | Successful termination |
1 | General error |
2 | Misuse of shell builtins |
126 | Command invoked cannot run |
127 | Command not found |
130 | Script terminated by Control-C |
Each method has its appropriate use case, and understanding these can help you manage program termination effectively.
Expert Insights on Exiting Programs in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Exiting a program in Python can be efficiently handled using the built-in `sys.exit()` function, which allows for a graceful termination of the script. This method also enables the passing of an exit status, which can be crucial for debugging and signaling success or failure to the operating system.”
Michael Chen (Python Developer Advocate, CodeCraft). “While `sys.exit()` is the most common approach, it is important to understand that raising a `SystemExit` exception can also serve the purpose of terminating a program. This method provides more flexibility, especially when you want to handle cleanup operations before exiting.”
Linda Foster (Lead Instructor, Python Programming Bootcamp). “For beginners, it is essential to remember that using `exit()` or `quit()` in interactive environments like IDLE or Jupyter Notebooks can lead to unexpected behavior. It is advisable to stick with `sys.exit()` for consistency and reliability across different execution contexts.”
Frequently Asked Questions (FAQs)
How can I exit a Python program using the exit() function?
You can exit a Python program by using the `exit()` function from the `sys` module. First, import the module with `import sys`, then call `sys.exit()`. This function can also take an optional exit status code.
What is the purpose of the quit() function in Python?
The `quit()` function serves a similar purpose as `exit()`. It is a built-in function that raises the `SystemExit` exception, terminating the program. It is primarily used in interactive sessions.
How do I exit a Python program using a keyboard interrupt?
You can exit a running Python program by pressing `Ctrl + C` in the terminal or command prompt. This sends a keyboard interrupt signal, which raises a `KeyboardInterrupt` exception, terminating the program.
Can I exit a Python program with a specific exit code?
Yes, you can exit a Python program with a specific exit code by passing an integer argument to `sys.exit()`. A code of `0` typically indicates success, while any non-zero code indicates an error or abnormal termination.
Is there a way to exit a Python script from within a function?
Yes, you can exit a Python script from within a function by calling `sys.exit()` or `raise SystemExit`. This will terminate the program regardless of where the call is made.
What happens if I do not handle exceptions before exiting a Python program?
If exceptions are not handled before exiting, Python will print a traceback of the error to the console. This can provide useful debugging information but may not be desirable in production environments.
Exiting a program in Python can be accomplished through various methods, each suited for different scenarios. The most common way to terminate a Python program is by using the built-in function `exit()`, which is part of the `sys` module. This method is straightforward and effectively halts the execution of the program. Another option is to use `sys.exit()`, which provides additional flexibility by allowing you to specify an exit status code, indicating whether the program completed successfully or encountered an error.
Additionally, the `quit()` function serves a similar purpose to `exit()` and is often used in interactive sessions. It is important to note that both `exit()` and `quit()` are primarily intended for use in the interpreter and are not recommended for use in production code. In a more structured application, raising an exception, such as `SystemExit`, can also be an effective way to exit the program while providing the opportunity to handle cleanup operations or resource deallocation.
Understanding these methods for exiting a Python program is crucial for effective program control and error handling. Each method has its own use case, and selecting the appropriate one can enhance the robustness of your code. Furthermore, being aware of the implications of each approach ensures that the program exits
Author Profile
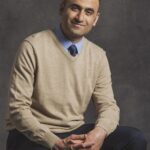
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?