How Can You Easily Find Your Node.js Version?
Are you diving into the world of Node.js and eager to harness its power for your next project? Understanding the version of Node.js you’re working with is crucial, as it can significantly impact your development environment, compatibility with packages, and access to the latest features. Whether you’re a seasoned developer or just starting out, knowing how to find your Node.js version is a fundamental skill that ensures your applications run smoothly and efficiently.
In the ever-evolving landscape of web development, Node.js has emerged as a powerhouse, enabling developers to build scalable network applications with ease. However, with frequent updates and new releases, it’s essential to stay informed about the version you’re using. Different versions may introduce new functionalities, deprecate older features, or fix critical bugs, making it vital to keep track of your Node.js environment.
Finding your Node.js version is a straightforward process, yet it can be overlooked by many. Whether you’re troubleshooting an issue, ensuring compatibility with libraries, or simply curious about the capabilities at your disposal, this knowledge will empower you to make informed decisions in your development journey. In the following sections, we’ll explore the various methods to check your Node.js version, ensuring you’re well-equipped to maximize your coding experience.
Using the Command Line to Check Node.js Version
To find out which version of Node.js is currently installed on your system, the command line is the most straightforward method. By executing a simple command, you can quickly retrieve the version information. Here’s how to do it:
- Open your command prompt (Windows) or terminal (macOS/Linux).
- Type the following command and press Enter:
“`bash
node -v
“`
- The output will display the version number, for example, `v16.13.0`.
This command works effectively across various operating systems, ensuring you can check the Node.js version regardless of your development environment.
Using Node.js REPL to Check Version
Another method to check the Node.js version is by utilizing the Read-Eval-Print Loop (REPL). This interactive environment allows you to execute JavaScript code in real-time. Here’s how you can access it and find the version:
- Open your command prompt or terminal.
- Type `node` and press Enter to launch the REPL.
- Once in the REPL, type the following command:
“`javascript
process.version
“`
- Press Enter, and you will see the version number displayed, such as `v16.13.0`.
This method can be particularly useful if you are already working within a Node.js script or application.
Checking Node.js Version in Package.json
If you’re working within a Node.js project, you might also find the version information documented in the `package.json` file. This file contains metadata about the project, including the version of Node.js that the project is compatible with. Here’s how to check it:
- Open the `package.json` file located in the root directory of your Node.js project.
- Look for the `engines` field, which specifies the version of Node.js required. It may look like this:
“`json
“engines”: {
“node”: “>=14.0.0”
}
“`
This entry indicates that the project is intended to run on Node.js version 14.0.0 or higher.
Understanding Node.js Versioning
Node.js uses Semantic Versioning, which consists of three numbers separated by dots: `MAJOR.MINOR.PATCH`. Understanding what each of these components signifies can help you determine compatibility and stability:
- MAJOR: Incremented for incompatible API changes.
- MINOR: Incremented when functionality is added in a backward-compatible manner.
- PATCH: Incremented for backward-compatible bug fixes.
Here’s a brief overview of versioning:
Version Number | Description |
---|---|
16.0.0 | Major release with breaking changes. |
16.1.0 | Minor release with new features. |
16.1.1 | Patch release fixing bugs. |
Understanding these components can assist developers in managing their Node.js applications effectively and ensuring compatibility with libraries and frameworks.
Checking Node.js Version via Command Line
To determine the installed version of Node.js on your system, the most straightforward method is to use the command line interface. This can be done on various operating systems, including Windows, macOS, and Linux.
- Open Command Line Interface:
- Windows: Press `Win + R`, type `cmd`, and hit Enter.
- macOS: Open `Terminal` from Applications or search for it using Spotlight.
- Linux: Open a terminal from your applications menu or use the keyboard shortcut `Ctrl + Alt + T`.
- Run the Version Command:
Execute the following command:
“`bash
node -v
“`
or
“`bash
node –version
“`
Upon running this command, the terminal will display the installed version of Node.js, such as `v14.17.0`.
Finding Node.js Version in Package.json
In a Node.js project, the version of Node.js required for the application may be specified within the `package.json` file. This is particularly useful when collaborating with others to ensure compatibility.
- **Locate the `package.json` File**:
Open the root directory of your Node.js application where the `package.json` file is located.
- **Look for the `engines` Field**:
Within the `package.json`, check for an `engines` section that specifies the Node.js version. It will look something like this:
“`json
“engines”: {
“node”: “>=14.0.0”
}
“`
This indicates that the project is compatible with Node.js versions 14.0.0 and above.
Using Node Version Manager (NVM)
If you are using Node Version Manager (NVM) to manage multiple Node.js versions, you can easily check which version is currently active.
- Check Current Version:
Open your terminal and run:
“`bash
nvm current
“`
- List Installed Versions:
To see all Node.js versions installed via NVM, use:
“`bash
nvm ls
“`
This will display a list, highlighting the currently active version.
Viewing Node.js Version in Application Code
In some cases, you might want to check the Node.js version programmatically within your application. You can access the version using the `process` global object.
- Example Code Snippet:
Here’s a simple example:
“`javascript
console.log(`Node.js version: ${process.version}`);
“`
Executing this code will output the Node.js version to the console when the application runs.
Checking Node.js Version in Docker Containers
If you are using Docker containers, you can also check the Node.js version from within a running container.
- Access the Container:
Use the following command to access the container’s shell:
“`bash
docker exec -it
“`
- Run Version Command:
Once inside the container, run:
“`bash
node -v
“`
This will display the Node.js version installed in the container environment.
Expert Insights on Determining Your Node.js Version
Dr. Emily Chen (Senior Software Engineer, Tech Innovations Corp). “To find the Node.js version installed on your system, simply open your terminal or command prompt and type ‘node -v’. This command will return the current version number, allowing you to ensure compatibility with your applications.”
Michael Thompson (Lead Developer, OpenSource Solutions). “Using ‘node –version’ in your command line is another effective way to check your Node.js version. This command is particularly useful for scripting and automation, as it can be easily integrated into build processes.”
Sarah Patel (Technical Writer, DevOps Digest). “For those using Node Version Manager (nvm), you can check the version by executing ‘nvm current’. This is especially beneficial for developers who manage multiple Node.js versions on their machines.”
Frequently Asked Questions (FAQs)
How can I check the Node.js version installed on my system?
You can check the installed Node.js version by running the command `node -v` or `node –version` in your terminal or command prompt.
What does the Node.js version number signify?
The Node.js version number follows the semantic versioning format, which consists of three parts: major, minor, and patch (e.g., 14.17.0). The major version indicates breaking changes, the minor version adds functionality, and the patch version includes bug fixes.
Can I find the Node.js version programmatically within my application?
Yes, you can retrieve the Node.js version programmatically by accessing `process.version` in your JavaScript code, which returns the version as a string.
Is there a way to check the Node.js version using npm?
Yes, you can check the Node.js version using npm by running the command `npm version node` in your terminal.
What should I do if I have multiple versions of Node.js installed?
If you have multiple versions of Node.js installed, consider using a version manager like `nvm` (Node Version Manager) to manage and switch between different versions easily.
How can I update my Node.js version?
You can update Node.js by downloading the latest version from the official Node.js website or using a version manager like `nvm` to install the desired version.
In summary, determining the version of Node.js installed on a system is a straightforward process that can be accomplished using the command line interface. The primary command utilized for this purpose is `node -v`, which outputs the current version number of Node.js. This command is a quick and effective way to verify the installation and ensure that the correct version is being used for development or deployment purposes.
Additionally, users can also employ the command `node –version` to achieve the same result. Both commands serve the same function and can be used interchangeably. It is essential for developers to be aware of their Node.js version, as different versions may have varying features, performance enhancements, and security updates that can impact their applications.
Furthermore, keeping Node.js up to date is crucial for maintaining application security and performance. Regularly checking the version and updating when necessary can help developers leverage the latest improvements and avoid potential vulnerabilities. Utilizing version management tools, such as nvm (Node Version Manager), can also facilitate the process of switching between different Node.js versions as needed for various projects.
Author Profile
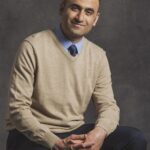
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?