How Can You Calculate the Mean in Python?
When it comes to data analysis and statistical calculations, finding the mean is one of the most fundamental tasks. Whether you’re a seasoned data scientist or a beginner exploring the world of Python programming, understanding how to calculate the mean is essential for interpreting data effectively. The mean, often referred to as the average, provides a quick snapshot of a dataset, allowing you to gauge central tendencies and make informed decisions based on numerical data. In this article, we will delve into the various methods available in Python to calculate the mean, empowering you to harness the power of this programming language in your analytical endeavors.
Python, with its rich ecosystem of libraries and tools, offers multiple approaches for calculating the mean of a dataset. From utilizing built-in functions to leveraging powerful libraries like NumPy and Pandas, the flexibility of Python makes it easy to perform this crucial operation. Each method has its own advantages, whether you are dealing with simple lists or complex data structures, and understanding these options will enhance your coding efficiency and accuracy.
As we explore the different techniques for finding the mean in Python, you’ll discover how to apply these methods in practical scenarios. We will also touch on the importance of data types and structures, ensuring that you have a comprehensive understanding of how to manipulate and analyze your data effectively. So, let
Calculating the Mean Using Python’s Built-in Functions
In Python, calculating the mean of a dataset can be efficiently performed using built-in functions. The simplest way to find the mean is by using the built-in `sum()` function in conjunction with the `len()` function. Here’s how it can be done:
“`python
data = [10, 20, 30, 40, 50]
mean = sum(data) / len(data)
print(“Mean:”, mean)
“`
This method is straightforward and works for small datasets. However, for larger datasets or when performance is a concern, using libraries such as NumPy is recommended.
Using NumPy to Calculate the Mean
NumPy is a powerful library for numerical computing in Python. It provides a dedicated function to calculate the mean, which is optimized for performance. To utilize NumPy, first, you need to install it if you haven’t already:
“`bash
pip install numpy
“`
Once NumPy is installed, you can calculate the mean as follows:
“`python
import numpy as np
data = np.array([10, 20, 30, 40, 50])
mean = np.mean(data)
print(“Mean:”, mean)
“`
This approach is not only more concise but also faster, especially for large datasets.
Calculating the Mean with Pandas
Pandas, another powerful library in Python, is specifically designed for data manipulation and analysis. It makes calculating the mean from a DataFrame or Series particularly easy. First, ensure you have Pandas installed:
“`bash
pip install pandas
“`
Here’s how to calculate the mean using Pandas:
“`python
import pandas as pd
data = pd.Series([10, 20, 30, 40, 50])
mean = data.mean()
print(“Mean:”, mean)
“`
Pandas also offers additional functionalities that can be useful when dealing with larger datasets, such as handling missing values seamlessly.
Comparison of Methods
Below is a comparison table of the methods discussed for calculating the mean in Python:
Method | Library | Code Example | Performance |
---|---|---|---|
Built-in Functions | None | sum(data) / len(data) | Good for small datasets |
NumPy | NumPy | np.mean(data) | Fast for large datasets |
Pandas | Pandas | data.mean() | Excellent for data analysis |
This table highlights the strengths and appropriate contexts for each method, allowing for informed choices depending on the specific needs of your data analysis tasks.
Calculating Mean Using Built-in Functions
Python provides built-in functions that make it easy to compute the mean of a dataset. The most common method is using the `sum()` and `len()` functions, which can be applied to lists or other iterable objects.
To calculate the mean:
- Sum the values in the dataset.
- Divide the total by the number of values.
Example code:
“`python
data = [10, 20, 30, 40, 50]
mean = sum(data) / len(data)
print(“Mean:”, mean)
“`
Using NumPy for Mean Calculation
NumPy is a powerful library for numerical computing in Python. It provides a convenient method, `numpy.mean()`, which simplifies the process of calculating the mean.
To use NumPy:
- Install NumPy if it’s not already installed:
“`bash
pip install numpy
“`
- Import NumPy in your script.
- Use the `mean` function to calculate the mean.
Example code:
“`python
import numpy as np
data = [10, 20, 30, 40, 50]
mean = np.mean(data)
print(“Mean using NumPy:”, mean)
“`
Pandas for Mean Calculation
Pandas is another library that offers a robust way to handle data in Python, particularly with DataFrames. The `mean()` method can be applied to Series or DataFrames.
To compute the mean using Pandas:
- Install Pandas if necessary:
“`bash
pip install pandas
“`
- Import Pandas into your script.
- Create a Series or DataFrame and call the `mean()` method.
Example code:
“`python
import pandas as pd
data = pd.Series([10, 20, 30, 40, 50])
mean = data.mean()
print(“Mean using Pandas:”, mean)
“`
Handling Missing Values
When calculating the mean, it is essential to consider how to handle missing values. Both NumPy and Pandas provide options to exclude NaN values.
- NumPy: Use `np.nanmean()` to ignore NaNs.
Example:
“`python
data = [10, 20, np.nan, 40, 50]
mean = np.nanmean(data)
print(“Mean ignoring NaN:”, mean)
“`
- Pandas: The `mean()` method automatically skips NaNs by default.
Example:
“`python
data = pd.Series([10, 20, None, 40, 50])
mean = data.mean()
print(“Mean ignoring None:”, mean)
“`
Mean of Multi-dimensional Arrays
For multi-dimensional arrays, both NumPy and Pandas allow you to specify the axis along which to calculate the mean.
- In NumPy, set the `axis` parameter in the `mean()` function.
Example:
“`python
data = np.array([[1, 2, 3], [4, 5, 6]])
mean_rows = np.mean(data, axis=0) Mean of each column
mean_cols = np.mean(data, axis=1) Mean of each row
print(“Mean of columns:”, mean_rows)
print(“Mean of rows:”, mean_cols)
“`
- In Pandas, use the `mean()` method on DataFrames and specify the `axis`.
Example:
“`python
data = pd.DataFrame([[1, 2, 3], [4, 5, 6]])
mean_columns = data.mean(axis=0)
mean_rows = data.mean(axis=1)
print(“Mean of columns in DataFrame:”, mean_columns)
print(“Mean of rows in DataFrame:”, mean_rows)
“`
Calculating the mean in Python can be accomplished using various methods, including built-in functions, NumPy, and Pandas. Each approach has its advantages depending on the complexity and structure of the data being analyzed.
Expert Insights on Calculating the Mean in Python
Dr. Emily Carter (Data Scientist, Tech Innovations Inc.). “When calculating the mean in Python, utilizing libraries such as NumPy can significantly simplify the process. The function `numpy.mean()` provides an efficient and straightforward method to compute the mean of an array or list, making it an essential tool for data analysis.”
Michael Chen (Software Engineer, Data Insights LLC). “For those new to Python, it’s important to understand that the mean can also be calculated using pure Python functions. By summing the elements of a list and dividing by the length of the list, you can achieve the same result without relying on external libraries.”
Laura Simmons (Educator and Python Programming Specialist). “Teaching students how to find the mean in Python involves demonstrating both the built-in functions and the use of libraries. This dual approach not only enhances understanding but also prepares them for real-world applications where efficiency is key.”
Frequently Asked Questions (FAQs)
How do I calculate the mean of a list of numbers in Python?
You can calculate the mean by using the `mean()` function from the `statistics` module. For example:
“`python
import statistics
data = [1, 2, 3, 4, 5]
mean_value = statistics.mean(data)
“`
Can I find the mean using NumPy in Python?
Yes, you can use the `mean()` function from the NumPy library. First, import NumPy and then apply the function as follows:
“`python
import numpy as np
data = [1, 2, 3, 4, 5]
mean_value = np.mean(data)
“`
What is the difference between using the statistics module and NumPy for calculating the mean?
The `statistics` module is part of the standard library and is suitable for basic statistical calculations. NumPy, on the other hand, is optimized for numerical operations on large datasets and provides additional functionality for array manipulations.
Can I calculate the mean of a DataFrame column in pandas?
Yes, you can calculate the mean of a specific column in a pandas DataFrame using the `mean()` method. For example:
“`python
import pandas as pd
df = pd.DataFrame({‘A’: [1, 2, 3, 4, 5]})
mean_value = df[‘A’].mean()
“`
What happens if I try to calculate the mean of an empty list in Python?
Calculating the mean of an empty list will raise a `StatisticsError` when using the `statistics` module, and it will return `nan` when using NumPy. Always ensure that the list is not empty before performing the calculation.
Is it possible to find the mean of non-numeric data in Python?
No, the mean can only be calculated for numeric data types. Attempting to calculate the mean of non-numeric data will result in a `TypeError`. Ensure that all elements in the dataset are numeric before performing the calculation.
Finding the mean in Python can be accomplished through various methods, each suited for different use cases. The most common approaches include using built-in functions, leveraging libraries such as NumPy and Pandas, and implementing custom functions. Python’s built-in functions like `sum()` and `len()` can be combined to calculate the mean for simple lists or arrays. However, for more complex data structures or larger datasets, utilizing libraries like NumPy or Pandas is often more efficient and provides additional functionality.
Using the NumPy library, the mean can be calculated easily with the `numpy.mean()` function, which is optimized for performance and can handle multi-dimensional arrays. Similarly, Pandas offers the `DataFrame.mean()` method, which is particularly useful for statistical analysis on tabular data. These libraries not only simplify the calculation of the mean but also provide a wealth of additional statistical functions that can enhance data analysis capabilities.
In summary, Python provides multiple ways to find the mean, catering to both simple and complex data scenarios. Understanding the context in which you are working will help determine the best method to use. By leveraging the power of libraries like NumPy and Pandas, users can perform more sophisticated data analysis while ensuring accuracy and efficiency in their
Author Profile
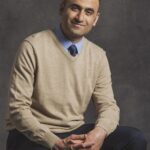
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?