How Can You Find the Smallest Number in a List Using Python?
When working with data in Python, one of the most fundamental tasks you might encounter is identifying the smallest number in a list. Whether you’re analyzing statistical data, processing user inputs, or simply organizing information, knowing how to efficiently find the minimum value can be a game-changer. This seemingly simple operation is a building block for more complex algorithms and data manipulations, making it essential for both novice and experienced programmers alike.
In Python, there are several methods to find the smallest number in a list, each with its own advantages. You can leverage built-in functions, utilize loops, or even apply more advanced techniques depending on your specific needs and the nature of your data. Understanding these approaches not only enhances your coding skills but also deepens your comprehension of Python’s capabilities in handling lists and data structures.
As we delve deeper into the various methods for finding the smallest number in a list, we’ll explore both the straightforward and the more nuanced techniques. Whether you’re looking for a quick solution or aiming to optimize performance for larger datasets, this guide will equip you with the knowledge and tools to tackle this common programming challenge with confidence.
Finding the Smallest Number Using Built-in Functions
One of the most straightforward ways to find the smallest number in a list in Python is by using the built-in `min()` function. This function takes an iterable as an argument and returns the smallest item. Here’s how to use it:
“`python
numbers = [4, 2, 9, 1, 5]
smallest_number = min(numbers)
print(smallest_number) Output: 1
“`
The `min()` function is efficient and concise, making it an excellent choice for quick operations. It can also handle lists containing various data types if they are comparable.
Finding the Smallest Number Using a Loop
If you prefer a more manual approach or need to implement custom logic, you can find the smallest number by iterating through the list. This method provides greater control over the process and allows for additional conditions or operations.
Here is a sample code snippet demonstrating this approach:
“`python
numbers = [4, 2, 9, 1, 5]
smallest_number = numbers[0] Assume the first number is the smallest
for number in numbers:
if number < smallest_number:
smallest_number = number
print(smallest_number) Output: 1
```
This method initializes the smallest number with the first element and then compares each subsequent number to update the smallest number accordingly.
Finding the Smallest Number with List Comprehension
List comprehension can also be utilized to filter and find the smallest number by creating a new list of elements that are less than a certain value. However, for finding the smallest element directly, it is less common but can be adapted creatively.
For instance, you can create a new list by filtering out elements and then apply `min()`:
“`python
numbers = [4, 2, 9, 1, 5]
smallest_number = min([num for num in numbers]) Output: 1
“`
This method retains the clarity of intent while leveraging the advantages of list comprehensions.
Comparison of Methods
The following table summarizes the methods for finding the smallest number in a list:
Method | Description | Pros | Cons |
---|---|---|---|
min() | Built-in function to get the smallest item | Simple and efficient | Less control over the process |
Loop | Iterating through the list manually | More control, can implement custom logic | More verbose |
List Comprehension | Creating a new list and applying min() | Concise and readable | Can be less efficient for large lists |
Each method has its unique advantages and is suitable for different scenarios depending on the specific needs of the program or algorithm being developed.
Methods to Find the Smallest Number in a List
In Python, there are several methods to identify the smallest number in a list. Each method has its own advantages, depending on the context of use. Below are some of the most common techniques.
Using the `min()` Function
The simplest and most efficient way to find the smallest number in a list is by using the built-in `min()` function. This function takes an iterable as an argument and returns the smallest item.
“`python
numbers = [5, 2, 9, 1, 5, 6]
smallest_number = min(numbers)
print(smallest_number) Output: 1
“`
Using a Loop
If you prefer or need to implement the logic manually, you can use a loop to iterate through the list and determine the smallest number. This approach is particularly useful for educational purposes or when you need to customize the logic.
“`python
numbers = [5, 2, 9, 1, 5, 6]
smallest_number = numbers[0]
for number in numbers:
if number < smallest_number:
smallest_number = number
print(smallest_number) Output: 1
```
Using the `sorted()` Function
Another method is to sort the list and then access the first element. This method is less efficient due to the overhead of sorting, but it can be useful when you need the numbers in order.
“`python
numbers = [5, 2, 9, 1, 5, 6]
sorted_numbers = sorted(numbers)
smallest_number = sorted_numbers[0]
print(smallest_number) Output: 1
“`
Using List Comprehensions
For those who prefer a more Pythonic approach, a list comprehension can be leveraged to filter out numbers and find the smallest one. While this is not the most efficient, it demonstrates Python’s expressive capabilities.
“`python
numbers = [5, 2, 9, 1, 5, 6]
smallest_number = [num for num in numbers if num == min(numbers)][0]
print(smallest_number) Output: 1
“`
Comparative Performance
The following table summarizes the performance characteristics of each method:
Method | Time Complexity | Space Complexity |
---|---|---|
`min()` | O(n) | O(1) |
Loop | O(n) | O(1) |
`sorted()` | O(n log n) | O(n) |
List Comprehension | O(n) | O(n) |
Choosing the appropriate method to find the smallest number in a list depends on the specific requirements of your program, such as performance needs and code readability. The `min()` function is generally the most efficient and straightforward approach, while loops and comprehensions can be beneficial for educational purposes or specific custom logic implementations.
Expert Insights on Finding the Smallest Number in a List in Python
Dr. Emily Carter (Data Scientist, Tech Innovations Inc.). “To efficiently find the smallest number in a list in Python, utilizing the built-in `min()` function is the most straightforward approach. This function is optimized for performance and readability, making it a preferred choice for both beginners and experienced programmers.”
James Liu (Software Engineer, CodeCraft Solutions). “While the `min()` function is excellent for simplicity, implementing a custom algorithm, such as a linear search, can provide deeper insights into algorithmic thinking. This method involves iterating through each element and comparing values, which is a fundamental skill in programming.”
Sarah Thompson (Python Developer, Open Source Community). “For those interested in performance optimization, especially with large datasets, consider using libraries like NumPy. The `numpy.min()` function is highly efficient for numerical operations and can significantly reduce execution time compared to native Python lists.”
Frequently Asked Questions (FAQs)
How can I find the smallest number in a list using Python?
You can use the built-in `min()` function to find the smallest number in a list. For example, `smallest = min(my_list)` will return the smallest element in `my_list`.
Is it possible to find the smallest number without using the min() function?
Yes, you can iterate through the list using a loop and compare each element to find the smallest one. Initialize a variable with the first element and update it whenever a smaller element is found.
What happens if the list is empty when using min()?
If the list is empty, calling `min()` will raise a `ValueError`. It is advisable to check if the list is empty before using the function.
Can I find the smallest number in a list of strings representing numbers?
Yes, you can convert the strings to integers or floats using a list comprehension before applying the `min()` function. For example, `smallest = min(int(x) for x in my_list)`.
How do I handle lists with negative numbers when finding the smallest number?
The `min()` function will correctly identify the smallest number, regardless of whether the numbers are positive or negative. It compares all numbers based on their value.
What if I want to find the smallest number based on a specific condition?
You can use a list comprehension to filter the list based on your condition and then apply the `min()` function. For example, `smallest = min(x for x in my_list if x > 0)` will find the smallest positive number.
Finding the smallest number in a list in Python can be accomplished using several methods, each with its own advantages. The most straightforward approach is to utilize the built-in `min()` function, which efficiently returns the smallest element from an iterable. This method is not only concise but also optimized for performance, making it a preferred choice for many developers.
Alternatively, one can implement a custom solution by iterating through the list and comparing each element to determine the smallest value. This method provides a deeper understanding of how algorithms work and can be useful in scenarios where one might need to apply additional logic during the comparison process. However, it is generally less efficient than using the built-in function.
In summary, while the `min()` function is the most efficient and simplest way to find the smallest number in a list, understanding how to manually iterate through the list can enhance one’s programming skills. Each method has its place depending on the context and requirements of the task at hand. Developers should choose the approach that best fits their needs while considering factors such as readability, performance, and maintainability.
Author Profile
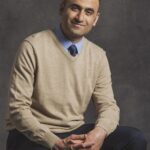
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?