How Can You Easily Find the Sum of a List in Python?
When working with data in Python, one of the most fundamental tasks you’ll encounter is calculating the sum of a list. Whether you’re analyzing numerical datasets, aggregating scores, or simply performing basic arithmetic, knowing how to efficiently compute the sum of a list is an essential skill for any programmer. Python, with its intuitive syntax and powerful built-in functions, makes this operation not only straightforward but also a great opportunity to explore the language’s capabilities.
In Python, lists are versatile data structures that can hold a collection of items, and summing these items can be accomplished in various ways. From using built-in functions to employing loops and list comprehensions, there are multiple approaches to achieve this goal. Each method has its own advantages, depending on the context and specific requirements of your project. Understanding these different techniques will not only enhance your coding efficiency but also deepen your grasp of Python’s functionality.
As you delve into the methods for summing a list, you’ll discover how Python’s simplicity allows you to focus on solving problems rather than getting bogged down in complex syntax. Whether you’re a beginner looking to grasp the basics or an experienced developer seeking to refine your skills, mastering the art of summing lists will empower you to tackle a wide range of programming challenges with confidence.
Using Built-in Functions
Python provides several built-in functions that make it straightforward to find the sum of a list. The most commonly used function for this purpose is `sum()`, which takes an iterable as its argument and returns the total sum of its elements. The basic syntax is as follows:
“`python
total = sum(list_name)
“`
For example, if you have a list of integers:
“`python
numbers = [1, 2, 3, 4, 5]
total = sum(numbers)
print(total) Output: 15
“`
This method is efficient and concise, making it the preferred choice for summing elements in a list.
Using a Loop
Another method to calculate the sum of a list is by using a loop. This approach provides more control over the summation process, allowing for additional logic to be implemented if necessary. Here’s how you can achieve this:
“`python
total = 0
for number in numbers:
total += number
print(total) Output: 15
“`
This method explicitly iterates through each element of the list, adding each value to a running total. While this is more verbose than using the `sum()` function, it can be useful when you need to apply conditions during the summation.
Using List Comprehensions
List comprehensions provide a compact way to process lists and can be combined with the `sum()` function for more complex scenarios. For instance, if you only want to sum even numbers from a list, you can do so as follows:
“`python
even_sum = sum(number for number in numbers if number % 2 == 0)
print(even_sum) Output: 6
“`
This approach is particularly powerful for filtering and summing in a single line of code.
Using NumPy for Large Lists
When dealing with large datasets, the NumPy library can be advantageous. It is optimized for numerical operations and can handle large arrays more efficiently than standard Python lists. To sum elements using NumPy, follow these steps:
- Install NumPy if you haven’t already:
“`bash
pip install numpy
“`
- Use NumPy to sum the array:
“`python
import numpy as np
numbers_array = np.array(numbers)
total = np.sum(numbers_array)
print(total) Output: 15
“`
This method is particularly efficient for large-scale data operations.
Comparison of Methods
The following table summarizes the various methods for calculating the sum of a list in Python:
Method | Advantages | Disadvantages |
---|---|---|
Built-in sum() | Simplicity and readability | Limited flexibility for complex operations |
Loop | Flexibility for custom logic | More verbose code |
List Comprehensions | Conciseness and filtering capability | Can be less readable for complex conditions |
NumPy | Efficiency with large datasets | Requires additional library |
Choosing the right method depends on your specific use case, data size, and the complexity of the operations required during summation. Each approach offers unique benefits that can be leveraged based on the context.
Using the Built-in sum() Function
The most straightforward method to calculate the sum of a list in Python is by utilizing the built-in `sum()` function. This function takes an iterable, such as a list, and returns the total of its elements.
“`python
numbers = [1, 2, 3, 4, 5]
total = sum(numbers)
print(total) Output: 15
“`
Key features of the `sum()` function include:
- Simplicity: It requires minimal code.
- Flexibility: You can provide an optional second argument to specify a starting value.
Example of using a starting value:
“`python
total_with_start = sum(numbers, 10)
print(total_with_start) Output: 25
“`
Using a For Loop
A manual approach to summing a list is through a for loop. This method allows for more control and is beneficial for adding conditional logic.
“`python
numbers = [1, 2, 3, 4, 5]
total = 0
for number in numbers:
total += number
print(total) Output: 15
“`
This approach can be modified to include conditions, such as summing only even numbers:
“`python
total_even = 0
for number in numbers:
if number % 2 == 0:
total_even += number
print(total_even) Output: 6
“`
Using List Comprehensions
List comprehensions can be a concise alternative for summing elements that meet specific criteria. This method combines filtering and summing in one line.
“`python
total_even = sum(number for number in numbers if number % 2 == 0)
print(total_even) Output: 6
“`
This approach is useful when you want to apply conditions directly within the summing process.
Using the reduce() Function
The `reduce()` function from the `functools` module provides another way to calculate the sum. It applies a binary function cumulatively to the items of an iterable.
“`python
from functools import reduce
numbers = [1, 2, 3, 4, 5]
total = reduce(lambda x, y: x + y, numbers)
print(total) Output: 15
“`
This method is more suited for complex operations, where a custom function might be necessary.
Performance Considerations
When choosing a method to sum a list, consider the following performance aspects:
Method | Time Complexity | Readability |
---|---|---|
`sum()` | O(n) | High |
For Loop | O(n) | Moderate |
List Comprehensions | O(n) | High |
`reduce()` | O(n) | Moderate |
The `sum()` function is generally the most efficient and readable for simple summations, while for loops and list comprehensions may offer flexibility for more complex scenarios.
The choice of method to sum a list in Python depends on the specific requirements of the task, including readability, performance, and the need for conditional logic. By understanding these various approaches, one can efficiently compute sums in diverse programming situations.
Expert Insights on Summing Lists in Python
Dr. Emily Carter (Senior Data Scientist, Tech Innovations Inc.). “Utilizing Python’s built-in `sum()` function is the most efficient way to find the sum of a list. It is not only concise but also optimized for performance, making it ideal for handling large datasets.”
Michael Chen (Software Engineer, CodeCraft Solutions). “When summing a list in Python, one should also consider the use of list comprehensions for more complex summations. This allows for conditional summing and enhances code readability.”
Sarah Patel (Python Programming Instructor, LearnCode Academy). “For beginners, it is crucial to understand the difference between using `sum()` and manually iterating through the list. While both methods achieve the same result, the former is preferable for its simplicity and clarity.”
Frequently Asked Questions (FAQs)
How can I calculate the sum of a list in Python?
You can calculate the sum of a list in Python using the built-in `sum()` function. For example, `sum(my_list)` will return the total of all elements in `my_list`.
What types of elements can I sum in a Python list?
You can sum numeric types such as integers and floats. If the list contains non-numeric types, a `TypeError` will occur.
Can I sum a list of lists in Python?
Yes, you can sum a list of lists by using a combination of list comprehensions and the `sum()` function. For example, `sum(my_list_of_lists, [])` flattens the list before summing.
Is it possible to sum only specific elements in a list?
Yes, you can sum specific elements by using list comprehensions or the `filter()` function. For example, `sum(x for x in my_list if x > 10)` sums only the elements greater than 10.
What happens if I try to sum an empty list in Python?
If you sum an empty list using `sum()`, the result will be `0`, as the sum of no elements is defined to be zero.
Are there any performance considerations when summing large lists?
Yes, for very large lists, using `numpy` can be more efficient due to its optimized performance for numerical operations. You can use `numpy.sum(my_array)` for better performance with large datasets.
Finding the sum of a list in Python is a straightforward task that can be accomplished using various methods. The most common and efficient way to achieve this is by utilizing the built-in `sum()` function, which takes an iterable as an argument and returns the total of its elements. This method is not only concise but also optimized for performance, making it suitable for lists of varying sizes.
In addition to the `sum()` function, Python also offers alternative approaches, such as using a for loop or list comprehensions. While these methods provide flexibility and can be useful in specific scenarios, they tend to be less efficient than the built-in function. It is essential to consider the context in which you are summing the list, as performance can vary significantly based on the chosen method.
Overall, leveraging Python’s built-in capabilities, such as the `sum()` function, is the best practice for summing the elements of a list. This approach not only simplifies the code but also enhances readability and maintainability. Understanding these options allows developers to make informed decisions based on their specific needs and the characteristics of the data they are working with.
Author Profile
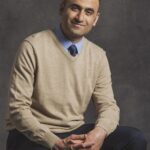
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?