How Can You Generate a Source Map for Your TypeScript Project?
In the world of web development, TypeScript has emerged as a powerful ally for developers seeking to write robust, maintainable code. However, with the added complexity of this statically typed superset of JavaScript comes the need for effective debugging tools. Enter source maps—an essential feature that bridges the gap between your TypeScript code and the JavaScript that browsers understand. If you’ve ever found yourself lost in a sea of minified code during a debugging session, you know just how invaluable source maps can be. In this article, we will explore how to generate source maps for your TypeScript projects, enhancing your debugging experience and making your development process smoother.
Source maps serve as a vital tool in the development toolkit, allowing developers to map their compiled JavaScript back to the original TypeScript source files. This means that when an error occurs in the browser, you can trace it back to the exact line in your TypeScript code, rather than sifting through the generated JavaScript. Understanding how to generate these source maps is crucial for any TypeScript developer looking to streamline their debugging process and improve code quality.
Generating source maps for a TypeScript project is a straightforward process that integrates seamlessly into your build workflow. Whether you’re using the TypeScript compiler directly or leveraging build tools
Configuring TypeScript for Source Maps
To generate source maps in a TypeScript project, you’ll need to adjust your `tsconfig.json` file. Source maps are crucial for debugging as they map your compiled JavaScript code back to the original TypeScript source, allowing you to track down errors more easily.
In your `tsconfig.json`, you can enable source map generation by setting the `sourceMap` option to `true`. Below is an example configuration:
“`json
{
“compilerOptions”: {
“target”: “es5”,
“module”: “commonjs”,
“sourceMap”: true,
“outDir”: “./dist”,
“strict”: true
},
“include”: [“src/**/*”],
“exclude”: [“node_modules”]
}
“`
Key options to note include:
- target: Specifies the ECMAScript target version.
- module: Defines the module system to use.
- sourceMap: When set to `true`, TypeScript will generate `.map` files.
- outDir: Indicates where to output the compiled JavaScript files.
Building the Project
After configuring your `tsconfig.json`, you can build your TypeScript project. Use the TypeScript compiler (tsc) to compile your code, which will generate both the JavaScript files and the corresponding source maps.
To build the project, run the following command in your terminal:
“`bash
tsc
“`
Once the compilation completes, you will find `.js` and `.js.map` files in the specified `outDir`.
Verifying Source Map Generation
After building your project, it’s essential to verify that source maps have been generated correctly. Check the output directory for `.map` files corresponding to each `.js` file. You can also manually inspect a generated source map file to ensure it contains the expected mappings.
Here’s a simple table displaying the relationship between TypeScript files and their generated JavaScript and source map files:
TypeScript File | Generated JavaScript File | Generated Source Map File |
---|---|---|
app.ts | app.js | app.js.map |
utils.ts | utils.js | utils.js.map |
Using Source Maps in Development
When you run your application in a browser or a debugging tool, it should automatically recognize the source maps if they are correctly generated. This allows you to set breakpoints directly in your TypeScript files rather than the transpiled JavaScript.
To ensure your development environment supports source maps:
- Use modern browsers that support source maps.
- Check your build tool (e.g., Webpack, Rollup) for additional source map settings if you’re integrating TypeScript with these tools.
By following these steps, you can effectively generate and utilize source maps in your TypeScript projects, enhancing your debugging capabilities.
Configuring TypeScript for Source Maps
To generate source maps in a TypeScript project, you need to adjust your TypeScript configuration file, typically named `tsconfig.json`. The source maps enable developers to trace errors in the compiled JavaScript back to the original TypeScript source code.
Steps to Enable Source Maps
- Open your `tsconfig.json` file.
- Locate the `compilerOptions` section.
- Add or modify the `sourceMap` option to `true`.
Here is an example configuration:
“`json
{
“compilerOptions”: {
“target”: “es5”,
“module”: “commonjs”,
“sourceMap”: true,
// other options…
}
}
“`
Additional Compiler Options
In addition to `sourceMap`, you may consider setting the following options for improved debugging and development:
- `inlineSourceMap`: Generates source maps as a base64 encoded string in the JavaScript file.
- `outDir`: Specifies the output folder for the compiled JavaScript files.
- `rootDir`: Sets the root directory of input files, which helps in structuring output paths.
Example with additional options:
“`json
{
“compilerOptions”: {
“target”: “es5”,
“module”: “commonjs”,
“sourceMap”: true,
“inlineSourceMap”: ,
“outDir”: “./dist”,
“rootDir”: “./src”
}
}
“`
Compiling TypeScript
After configuring the `tsconfig.json`, compile your TypeScript files using the TypeScript compiler (`tsc`). You can run the following command in your terminal:
“`bash
tsc
“`
This command will read the `tsconfig.json` file and generate the corresponding JavaScript files along with the source maps in the specified output directory.
Verifying Source Map Generation
Once the compilation is complete, you should check the output directory to ensure that `.js.map` files are created alongside the compiled `.js` files. The presence of these files indicates that source maps are successfully generated.
Using Source Maps in Development
To utilize source maps effectively, ensure that your development environment (such as a browser or IDE) is configured to recognize them. Most modern browsers automatically use source maps for JavaScript debugging. You can inspect the original TypeScript files directly in the browser’s developer tools, facilitating easier debugging.
Troubleshooting Common Issues
If source maps are not generated as expected, consider the following:
- Ensure that `sourceMap` is set to `true` in your `tsconfig.json`.
- Verify that there are no conflicting TypeScript compiler options.
- Check that you are compiling the TypeScript files from the correct directory.
- Look for any errors in the terminal output during compilation that might indicate issues with your TypeScript files.
By following these guidelines, you can effectively generate and utilize source maps in your TypeScript projects, making debugging a more straightforward and efficient process.
Expert Insights on Generating Source Maps for TypeScript Projects
Jessica Lin (Senior Software Engineer, CodeCraft Solutions). “Generating source maps in a TypeScript project is crucial for debugging. It allows developers to trace errors back to the original TypeScript code rather than the compiled JavaScript. To enable source maps, ensure that your `tsconfig.json` file includes the property `sourceMap: true`. This simple configuration can significantly enhance your debugging experience.”
Mark Thompson (Frontend Development Lead, Tech Innovations Inc.). “For TypeScript projects, utilizing source maps is essential for effective error tracking in production environments. In addition to setting `sourceMap: true` in your `tsconfig.json`, consider using build tools like Webpack or Rollup, which can further optimize the source map generation process and provide additional features like minification and tree-shaking.”
Elena Garcia (DevOps Engineer, Cloud Solutions Group). “When working with TypeScript, generating source maps is not just about enabling debugging; it is also about maintaining a smooth development workflow. I recommend automating the source map generation as part of your CI/CD pipeline. This ensures that every build includes the necessary source maps, making it easier for developers to diagnose issues quickly and efficiently.”
Frequently Asked Questions (FAQs)
What is a source map in a TypeScript project?
A source map is a file that maps the transformed, minified code back to the original source code. It enables developers to debug their TypeScript code in the browser, providing a clearer view of the original code instead of the compiled JavaScript.
How do I enable source map generation in TypeScript?
To enable source map generation in a TypeScript project, set the `sourceMap` option to `true` in your `tsconfig.json` file. This will instruct the TypeScript compiler to generate `.map` files alongside the compiled JavaScript files.
Can I generate source maps using the command line?
Yes, you can generate source maps using the TypeScript compiler from the command line. Use the command `tsc –sourceMap` along with your TypeScript files to compile them while generating the corresponding source maps.
What are the benefits of using source maps in TypeScript development?
Source maps facilitate easier debugging by allowing developers to see the original TypeScript code in browser developer tools. They help trace errors and understand the flow of the application without having to sift through the compiled JavaScript.
Are there any performance considerations when using source maps?
While source maps improve debugging, they can increase the size of the output files and may expose your source code if not handled properly. It is advisable to disable source maps in production environments to enhance performance and protect your source code.
How can I verify that source maps are working correctly in my project?
To verify that source maps are functioning, open your browser’s developer tools, navigate to the Sources tab, and check if your original TypeScript files appear. Set breakpoints and ensure you can debug the TypeScript code directly, confirming proper mapping.
Generating source maps for a TypeScript project is a crucial step in the development process, as it enhances the debugging experience by mapping the compiled JavaScript code back to the original TypeScript source. This allows developers to trace errors and understand the flow of their code more intuitively. To enable source map generation, developers need to adjust the TypeScript configuration file, typically `tsconfig.json`, by setting the `sourceMap` option to `true`. This simple adjustment ensures that the TypeScript compiler produces corresponding `.map` files alongside the compiled JavaScript files.
In addition to the configuration changes, it is important to consider the environment in which the TypeScript code will be executed. For instance, when using build tools or bundlers like Webpack or Rollup, developers may need to ensure that these tools are also configured to handle source maps properly. This may involve setting specific options in their configuration files to ensure that source maps are correctly generated and served in development environments.
Another key takeaway is the importance of testing the generated source maps to ensure they function as intended. Developers should verify that the source maps accurately reflect the original TypeScript files and that they can effectively navigate to the source code during debugging sessions. This verification process can save time
Author Profile
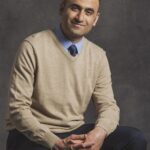
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?