How Can You Retrieve an Element from a Set in Python?
In the ever-evolving landscape of Python programming, sets stand out as a powerful and versatile data structure. Unlike lists or tuples, sets are unordered collections that automatically handle duplicates, making them ideal for scenarios where uniqueness is paramount. Whether you’re managing a collection of unique items, performing mathematical set operations, or simply looking to optimize your data handling, understanding how to effectively retrieve elements from a set is essential for any Python developer. In this article, we will delve into the intricacies of accessing elements within sets, uncovering the best practices and techniques that will enhance your coding prowess.
When working with sets in Python, it’s important to grasp the fundamental characteristics that distinguish them from other data types. Sets are mutable and dynamic, allowing for the addition and removal of elements, yet they do not maintain any specific order. This unique property can pose challenges when it comes to retrieving elements, especially if you are accustomed to the indexed nature of lists. However, with a little knowledge about the methods and functions available in Python, you can easily navigate these challenges and access the elements you need.
In this exploration, we will cover various approaches to extracting elements from sets, including the use of built-in functions and techniques for iterating through set contents. Whether you are a beginner looking to
Accessing Elements in a Python Set
To retrieve an element from a set in Python, it’s essential to understand that sets are unordered collections of unique elements. This characteristic means that you cannot access elements by index, as you would with a list or a tuple. Instead, you can use several methods to access or check for the presence of elements within a set.
Using Membership Operators
The most common way to check for an element in a set is by using the membership operator `in`. This operator returns a boolean value indicating whether the specified element exists in the set.
Example:
“`python
my_set = {1, 2, 3, 4, 5}
element = 3
if element in my_set:
print(f”{element} is in the set.”)
else:
print(f”{element} is not in the set.”)
“`
This method is efficient and straightforward, allowing for quick checks without the need for additional methods or functions.
Iterating Through a Set
If you need to access elements for processing, you can iterate over the set using a loop. This method allows you to work with each element individually.
Example:
“`python
for item in my_set:
print(item)
“`
This approach is useful when you want to perform operations on each element or when you need to extract values based on certain conditions.
Using Set Methods
Python sets provide various built-in methods that can help in accessing and manipulating elements. Here are a few relevant methods:
- `pop()`: Removes and returns an arbitrary element from the set. If the set is empty, it raises a `KeyError`.
- `discard(element)`: Removes an element from the set if it is present. Does not raise an error if the element is not found.
- `remove(element)`: Similar to `discard()`, but raises a `KeyError` if the element is not found.
Example:
“`python
element = my_set.pop() Removes and returns an arbitrary element
print(f”Removed element: {element}”)
“`
Performance Considerations
When accessing elements in a set, it is important to consider the performance implications. Sets in Python are implemented using hash tables, providing average time complexity for lookups, additions, and deletions of O(1).
Here is a comparison of common data structures in Python regarding access time:
Data Structure | Average Access Time |
---|---|
Set | O(1) |
List | O(n) |
Dictionary | O(1) |
Tuple | O(n) |
This table illustrates the efficiency of using sets for membership tests compared to other data structures in Python.
Accessing elements from a set in Python can be efficiently accomplished through membership testing, iteration, and specific set methods. Understanding these approaches allows for effective manipulation and checking of elements within a set.
Accessing Elements in a Set
In Python, sets are unordered collections of unique elements. This characteristic means that accessing elements by index, as one would do with lists or tuples, is not possible. However, there are several methods to retrieve elements from a set.
Using Iteration
The most straightforward way to access elements in a set is through iteration. This allows you to traverse each element in the set. Here’s how you can do this:
“`python
my_set = {1, 2, 3, 4, 5}
for element in my_set:
print(element)
“`
This code will print each element in the set, but the order may vary since sets are unordered.
Using the `next()` Function
If you need to retrieve a single element from a set, you can use the `next()` function along with an iterator. This method is efficient and straightforward. Here’s an example:
“`python
my_set = {10, 20, 30}
element = next(iter(my_set))
print(element)
“`
This code snippet retrieves and prints one element from `my_set`. The specific element returned can vary across different executions.
Using Set Comprehensions
Set comprehensions can also be used to create a new set based on conditions applied to existing elements. While this does not directly retrieve a single element, it allows for filtering and manipulation. Here’s an example:
“`python
my_set = {1, 2, 3, 4, 5}
filtered_set = {x for x in my_set if x > 3}
print(filtered_set) Output: {4, 5}
“`
In this example, `filtered_set` contains only elements greater than 3 from the original set.
Checking Membership
Before attempting to access an element, it’s often useful to check if an element exists in the set. This can be done using the `in` keyword:
“`python
my_set = {1, 2, 3}
if 2 in my_set:
print(“2 is present in the set.”)
“`
This approach enhances your code’s efficiency by preventing unnecessary operations on elements that may not exist.
Summary of Methods
Method | Description |
---|---|
Iteration | Traverse each element using a for loop. |
`next()` with `iter()` | Retrieve a single element from the set. |
Set comprehensions | Create a new set based on conditions applied. |
Membership check (`in`) | Check if an element exists before accessing it. |
By using these techniques, you can effectively manage and access elements within a set in Python, despite the limitations imposed by its unordered nature.
Expert Insights on Accessing Elements from Sets in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “To access an element from a set in Python, one must remember that sets are unordered collections. Therefore, while you cannot access elements by index like in lists, you can use membership tests or convert the set to a list if the order is necessary for your application.”
Mark Thompson (Data Scientist, Analytics Hub). “Utilizing the `in` keyword is the most efficient way to check for the presence of an element in a set. This operation is average O(1) in time complexity, making sets a powerful choice for membership testing in Python.”
Lisa Chen (Python Developer, CodeCraft Solutions). “If you need to retrieve an element from a set, consider using a loop or a comprehension to filter the set based on specific criteria. This approach allows for more complex queries while still leveraging the efficiency of sets.”
Frequently Asked Questions (FAQs)
How can I retrieve an element from a set in Python?
To retrieve an element from a set in Python, you can use the `in` keyword to check for membership. However, sets do not support indexing, so you cannot access elements by position. Instead, you can convert the set to a list or iterate through the set.
Can I access elements of a set using an index?
No, sets in Python are unordered collections, meaning they do not maintain a specific order and do not support indexing. You must use iteration or convert the set to a list to access elements by index.
What is the best way to check if an element exists in a set?
The best way to check for the existence of an element in a set is to use the `in` keyword. For example, `if element in my_set:` will return `True` if the element exists in the set.
How do I iterate through all elements in a set?
You can iterate through all elements in a set using a `for` loop. For example:
“`python
for element in my_set:
print(element)
“`
This will print each element in the set.
Can I retrieve multiple elements from a set at once?
Sets do not support direct retrieval of multiple elements by index. You can create a new set containing the desired elements or use list comprehension to filter elements based on specific criteria.
What happens if I try to access an element that is not in the set?
If you try to check for an element that is not in the set using the `in` keyword, it will simply return “. No error will be raised, as membership testing in sets is designed to handle such cases gracefully.
In Python, a set is an unordered collection of unique elements. To retrieve an element from a set, one must recognize that sets do not support indexing like lists or tuples. Instead, elements can be accessed through iteration or by using methods such as `pop()` or `discard()`. The `pop()` method removes and returns an arbitrary element from the set, while `discard()` can be used to remove a specific element without raising an error if the element is not found.
It is important to understand that since sets are unordered, the order of elements is not guaranteed. Therefore, if the specific element’s position is critical, alternative data structures such as lists or dictionaries may be more appropriate. Additionally, using a loop to iterate through the set allows for accessing all elements, which can be useful when performing operations that require examining each item.
To summarize, while retrieving elements from a set in Python is straightforward, it requires an understanding of the nature of sets as unordered collections. Utilizing methods like `pop()` or `discard()` provides effective means to access or remove elements, but for ordered access, other data structures should be considered. This knowledge is essential for writing efficient and effective Python code when working with sets.
Author Profile
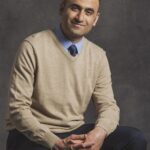
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?