How Can You Easily Retrieve the Last Element in a Python List?
When working with lists in Python, you often find yourself needing to access specific elements quickly and efficiently. Among these tasks, retrieving the last element of a list can be particularly useful, whether you’re processing data, managing collections, or simply iterating through items. Understanding how to do this not only enhances your coding skills but also empowers you to handle lists more adeptly in your projects. In this article, we will explore various methods to access the last element of a list in Python, equipping you with the knowledge to streamline your coding practices.
Python’s list data structure is versatile and widely used, making it essential for developers to master its functionalities. Accessing elements by their index is a fundamental operation, and while most of us are familiar with retrieving the first few items, the last element often requires a different approach. This is particularly true in scenarios where the list’s length may vary, and hardcoding an index could lead to errors. By understanding how Python handles negative indexing and other techniques, you can easily retrieve the last element, regardless of the list’s size.
In this article, we will delve into the various methods available for accessing the last element of a list in Python. From simple indexing to leveraging built-in functions, we will cover the most efficient approaches, ensuring you have
Accessing the Last Element of a List
To retrieve the last element of a list in Python, several methods can be utilized, each with its own advantages depending on the context of use. Below are the most common approaches:
- Using Negative Indexing: Python supports negative indexing, allowing you to directly access elements from the end of a list. The last element can be accessed using the index `-1`.
“`python
my_list = [10, 20, 30, 40, 50]
last_element = my_list[-1]
“`
- Using the `len()` Function: Another method is to calculate the length of the list and subtract one to get the index of the last element.
“`python
last_element = my_list[len(my_list) – 1]
“`
- Using the `pop()` Method: If you want to retrieve and remove the last element from the list, the `pop()` method can be used. This modifies the original list by removing the last item.
“`python
last_element = my_list.pop()
“`
Each of these methods can be useful in different scenarios:
Method | Modification of List | Syntax Example |
---|---|---|
Negative Indexing | No | my_list[-1] |
Using len() | No | my_list[len(my_list) – 1] |
Using pop() | Yes | my_list.pop() |
Considerations When Accessing the Last Element
While accessing the last element is straightforward, there are important considerations to keep in mind:
- Empty Lists: Attempting to access the last element of an empty list will raise an `IndexError`. It is prudent to check whether the list is empty before trying to access its elements.
“`python
if my_list:
last_element = my_list[-1]
else:
last_element = None or handle as appropriate
“`
- Performance: For large lists, using negative indexing or `len()` is generally more efficient than using `pop()` since the latter involves modifying the list.
- Readability: Code clarity is essential. While all methods are valid, choosing the one that best conveys your intent to other developers is crucial. Negative indexing is often favored for its simplicity and conciseness.
Using these techniques will enable you to effectively access the last element in a list in Python, enhancing both functionality and code clarity in your projects.
Accessing the Last Element in a List
To retrieve the last element from a list in Python, several methods can be utilized. The most common approaches include using negative indexing, the `pop()` method, and the `slice` technique. Each method has its own use case and advantages.
Using Negative Indexing
Negative indexing is a straightforward way to access elements from the end of a list. In Python, the last element can be accessed using the index `-1`. This method is efficient and highly readable.
“`python
my_list = [10, 20, 30, 40, 50]
last_element = my_list[-1]
print(last_element) Output: 50
“`
Using the `pop()` Method
The `pop()` method not only retrieves the last element but also removes it from the list. This is useful when you need to both access and modify the list simultaneously.
“`python
my_list = [10, 20, 30, 40, 50]
last_element = my_list.pop()
print(last_element) Output: 50
print(my_list) Output: [10, 20, 30, 40]
“`
Keep in mind that using `pop()` changes the original list by removing the last element.
Using Slicing Technique
Slicing can also be employed to obtain the last element. This method is particularly helpful when you are working with a subset of the list or if you prefer a slice format.
“`python
my_list = [10, 20, 30, 40, 50]
last_element = my_list[-1:] Returns a list containing the last element
print(last_element) Output: [50]
“`
While this returns a list, you can access the first element of the slice to get just the last element:
“`python
last_element = my_list[-1:][0]
print(last_element) Output: 50
“`
Comparative Overview of Methods
The following table summarizes the characteristics of each method for accessing the last element:
Method | Returns | Modifies List | Use Case |
---|---|---|---|
Negative Indexing | Single element | No | Fast and efficient access |
`pop()` | Single element | Yes | Access and remove from list |
Slicing | List (single item) | No | When working with subsets or slices |
Each method has its context of use, and the choice largely depends on whether you need to modify the original list or just read its last element.
Expert Insights on Accessing the Last Element in a Python List
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “To retrieve the last element in a list in Python, one can simply use the index -1. This approach is not only concise but also highly efficient, as it directly accesses the last item without the need for additional computations.”
Michael Chen (Python Developer, CodeMaster Solutions). “Utilizing negative indexing is a powerful feature in Python. By using list_name[-1], developers can easily access the last element of a list, which is particularly useful in scenarios involving dynamic data structures.”
Sarah Johnson (Data Scientist, Analytics Hub). “In data manipulation tasks, being able to access the last element of a list efficiently can streamline processes. The method of using the index -1 is not only Pythonic but also enhances code readability, making it easier for teams to collaborate.”
Frequently Asked Questions (FAQs)
How can I access the last element of a list in Python?
You can access the last element of a list in Python using the index `-1`. For example, `my_list[-1]` will return the last item in `my_list`.
Is there a built-in function to get the last element of a list in Python?
Python does not have a specific built-in function for this purpose, but you can achieve it easily with indexing as mentioned. Alternatively, you can use the `pop()` method to retrieve and remove the last element.
What happens if I try to access the last element of an empty list?
Attempting to access the last element of an empty list will raise an `IndexError`. It is advisable to check if the list is not empty before accessing its elements.
Can I use slicing to get the last element of a list?
Yes, you can use slicing to get the last element by using `my_list[-1:]`, which returns a new list containing the last element. However, this is less efficient than direct indexing.
Is there a difference between using `list[-1]` and `list.pop()` to get the last element?
Yes, `list[-1]` retrieves the last element without modifying the list, while `list.pop()` retrieves and removes the last element from the list.
What if I want to get the last n elements of a list?
To get the last n elements, use slicing with a negative index, such as `my_list[-n:]`, which returns a new list containing the last n elements of `my_list`.
In Python, retrieving the last element of a list can be accomplished using several straightforward methods. The most common approach is to utilize negative indexing, specifically by accessing the list with an index of -1. This method is efficient and concise, allowing for quick access to the last item without needing to calculate the length of the list. Additionally, using the `pop()` method can also be employed, which not only retrieves the last element but also removes it from the list, thereby modifying the original list structure.
Another method to access the last element involves using the `len()` function to determine the total number of elements in the list and then subtracting one to obtain the index of the last item. While this approach is effective, it is generally less preferred due to its additional computational overhead compared to negative indexing. Regardless of the method chosen, Python provides flexibility and ease of use when working with list data structures.
In summary, understanding how to efficiently access the last element of a list is fundamental for effective programming in Python. Utilizing negative indexing is the most recommended approach due to its simplicity and directness. However, developers should also be aware of other methods, such as using `pop()` or `len()`, to choose the best option based
Author Profile
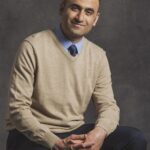
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?