How Can You Easily Determine the Length of an Array in Python?
When working with data in Python, arrays are a fundamental structure that allows for efficient storage and manipulation of collections of items. Whether you’re dealing with a simple list of numbers, a complex dataset, or even strings, understanding how to work with arrays is crucial for any programmer. One of the most common tasks you’ll encounter is determining the length of an array. This seemingly straightforward operation can unlock a wealth of possibilities in your coding endeavors, from controlling loops to validating data integrity. In this article, we will explore the various methods available in Python to get the length of an array, empowering you to handle your data with confidence and precision.
In Python, arrays can be represented using lists or the specialized array module, each with its own unique characteristics and functionalities. Knowing how to retrieve the length of these structures is essential for effective data manipulation. The length of an array not only informs you about the number of elements it contains but also plays a critical role in functions that require iteration or conditional logic.
As we delve deeper, we will examine the built-in functions and methods that Python offers for this purpose, highlighting their simplicity and efficiency. By mastering these techniques, you will enhance your programming toolkit and streamline your data handling processes. Whether you are a novice or an experienced coder, understanding how to
Using the Built-in `len()` Function
In Python, the most straightforward way to obtain the length of an array (or list) is by using the built-in `len()` function. This function takes a single argument, which is the array or list in question, and returns the number of elements it contains.
For example:
“`python
my_array = [1, 2, 3, 4, 5]
length = len(my_array)
print(length) Output: 5
“`
The `len()` function is efficient and works seamlessly with other data structures, including tuples, dictionaries, and strings.
Using NumPy for Arrays
When dealing with numerical data, it is common to use the NumPy library, which provides support for arrays. To find the length of a NumPy array, you can use the `shape` attribute or the `len()` function. However, for multidimensional arrays, `shape` provides more detailed information.
Here’s how you can do it:
“`python
import numpy as np
my_array = np.array([[1, 2, 3], [4, 5, 6]])
length = my_array.shape[0] Number of rows
print(length) Output: 2
“`
To get the total number of elements in the array, you can use:
“`python
total_elements = my_array.size
print(total_elements) Output: 6
“`
Comparing Different Methods of Getting Length
Here is a comparison of methods to retrieve the length of arrays and lists in Python:
Method | Type | Usage | Returns |
---|---|---|---|
len() | Built-in Function | len(array) | Number of elements |
array.shape | NumPy Attribute | array.shape | Tuple of dimensions |
array.size | NumPy Attribute | array.size | Total number of elements |
Considerations for Performance
When working with large datasets, choosing the right method to get the length of an array can impact performance. The `len()` function is optimized for speed and should be preferred for standard Python lists. For NumPy arrays, using the `shape` attribute is usually more efficient than using `len()` because it directly accesses the array’s metadata.
In summary, while retrieving the length of an array in Python can be accomplished through various means, understanding the context and type of data structure you are working with will help you select the most efficient approach.
Using the `len()` Function
In Python, the most straightforward method to determine the length of an array (or more accurately, a list) is by using the built-in `len()` function. This function returns the number of items in an object, including lists, strings, and tuples.
“`python
my_list = [1, 2, 3, 4, 5]
length = len(my_list)
print(length) Output: 5
“`
The `len()` function provides an efficient way to retrieve the size of the list in constant time, O(1).
Using NumPy for Arrays
When working with arrays in scientific computing or data analysis, the NumPy library offers enhanced capabilities. NumPy arrays can also utilize the `len()` function, but it is important to highlight the difference between the length of a one-dimensional array and the shape of multi-dimensional arrays.
“`python
import numpy as np
One-dimensional array
array_1d = np.array([1, 2, 3, 4, 5])
length_1d = len(array_1d)
print(length_1d) Output: 5
Two-dimensional array
array_2d = np.array([[1, 2], [3, 4], [5, 6]])
length_2d = array_2d.shape[0] Number of rows
print(length_2d) Output: 3
“`
- For one-dimensional arrays, `len(array)` works as expected.
- For two-dimensional arrays, use `array.shape` to access dimensions:
- `array.shape[0]` for the number of rows.
- `array.shape[1]` for the number of columns.
Accessing Length in Different Data Structures
Python offers a variety of data structures beyond lists and arrays. Each type has its method for obtaining length:
Data Structure | Method to Get Length | Example Code |
---|---|---|
List | `len()` | `len(my_list)` |
Tuple | `len()` | `len(my_tuple)` |
Dictionary | `len()` | `len(my_dict)` |
Set | `len()` | `len(my_set)` |
String | `len()` | `len(my_string)` |
Each of these structures provides an efficient way to determine the number of elements they contain.
Custom Classes
For custom classes, you can define the `__len__()` method to allow the use of `len()` on instances of your class. This allows for flexibility in how you define the length of your objects.
“`python
class MyClass:
def __init__(self, items):
self.items = items
def __len__(self):
return len(self.items)
my_object = MyClass([1, 2, 3, 4, 5])
print(len(my_object)) Output: 5
“`
Defining `__len__()` allows instances of your custom classes to integrate seamlessly with Python’s built-in functions, promoting code reusability and clarity.
Performance Considerations
While using the `len()` function is efficient, understanding its performance context is essential:
- Lists and Tuples: Accessing length is O(1) due to the way Python stores these objects.
- Dictionaries and Sets: These also return lengths in O(1) time, making them efficient for size checks.
- Custom Classes: Performance depends on the implementation of the `__len__()` method. Aim for O(1) complexity when possible.
Using these methods will ensure that you can effectively manage and analyze the lengths of various data structures in Python.
Understanding Array Length in Python: Expert Insights
Dr. Emily Carter (Senior Data Scientist, Tech Innovations Inc.). “In Python, the most efficient way to determine the length of an array is by using the built-in `len()` function. This function is not only straightforward but also optimized for performance, making it a preferred choice among developers.”
James Liu (Software Engineer, Python Development Group). “When working with lists or arrays in Python, the `len()` function provides a clear and concise way to access the number of elements. It’s essential for controlling loops and managing data structures effectively.”
Maria Gonzalez (Python Instructor, Code Academy). “Understanding how to get the length of an array is fundamental for any Python programmer. The `len()` function is universally applicable, whether you’re dealing with lists, tuples, or other iterable objects, ensuring consistency across your code.”
Frequently Asked Questions (FAQs)
How do I get the length of an array in Python?
You can use the built-in `len()` function to obtain the length of an array. For example, `len(my_array)` will return the number of elements in `my_array`.
Can I use `len()` on a list in Python?
Yes, the `len()` function works on lists as well as arrays. It returns the total number of items in the list.
What will `len()` return for an empty array?
The `len()` function will return `0` for an empty array, indicating that there are no elements present.
Is there a difference between arrays and lists in Python regarding length?
No, both arrays (from the `array` module) and lists can use the `len()` function to determine their length. The method of obtaining length is the same.
Can I use `len()` on multidimensional arrays?
Yes, you can use `len()` on multidimensional arrays. It will return the length of the first dimension. To get the length of other dimensions, you can access the specific sub-array and apply `len()` again.
Are there any performance considerations when using `len()` on large arrays?
Using `len()` is efficient and has a time complexity of O(1), meaning it retrieves the length in constant time, regardless of the array size.
In Python, determining the length of an array or any iterable is a straightforward process, primarily accomplished using the built-in `len()` function. This function returns the number of elements present in the array, making it an essential tool for developers working with data structures. It is important to note that the `len()` function is not limited to arrays alone; it can also be applied to lists, tuples, strings, and other collections in Python.
When working with arrays specifically, it is common to use the `array` module or libraries like NumPy, which provide additional functionalities. For instance, if using NumPy, the length of a NumPy array can also be obtained using the `len()` function, or alternatively, the `shape` attribute can be used to determine the dimensions of the array. This flexibility allows developers to choose the most appropriate method based on their specific needs and the data structures they are utilizing.
In summary, the `len()` function is a versatile and efficient way to retrieve the length of arrays and other iterable objects in Python. Understanding how to use this function effectively is crucial for managing data structures and performing operations that depend on the size of the collection. By leveraging this knowledge, developers can enhance their programming skills
Author Profile
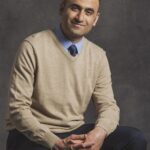
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?