How Can You Exit Python in Terminal: A Step-by-Step Guide?
Navigating the world of programming can sometimes feel like a labyrinth, especially when you’re immersed in a coding environment like Python. Whether you’re a seasoned developer or just dipping your toes into the waters of coding, there are moments when you find yourself in a terminal session, fingers poised over the keyboard, and suddenly, you need to exit. The simplicity of leaving a Python shell may seem trivial, but understanding how to do it efficiently can save you time and frustration. In this article, we will explore the various methods to gracefully exit Python in the terminal, ensuring that you can transition smoothly between your coding tasks.
When you enter the Python interactive shell, you’re greeted with a powerful tool that allows you to test snippets of code and experiment with various functions. However, there may come a time when you need to step away from this environment, whether to run a different script, troubleshoot an issue, or simply take a break. Knowing how to exit Python properly is essential for maintaining an organized workflow and preventing any potential data loss or confusion.
In the following sections, we will delve into the different techniques for exiting the Python terminal, including keyboard shortcuts and command-line instructions. Each method has its unique advantages, and understanding these will empower you to navigate your coding sessions with confidence. So
Exiting Python Interactive Shell
When you find yourself in the Python interactive shell (REPL), there are several straightforward ways to exit. The method you choose may depend on your specific environment or personal preference. Here are the most common approaches:
- Using exit() or quit():
- You can simply type `exit()` or `quit()` and then press Enter. Both commands will terminate the Python interpreter.
- Keyboard shortcuts:
- On most systems, you can exit by pressing `Ctrl + Z` followed by `Enter` on Windows, or `Ctrl + D` on Unix/Linux/Mac systems.
- Using the `sys` module:
- If you have imported the `sys` module, you can use `sys.exit()` to leave the interactive shell.
Exiting Python Scripts in Terminal
If you are running a Python script in the terminal and wish to exit it prematurely, there are specific methods you can employ:
- Keyboard Interrupt:
- You can typically stop a running script by pressing `Ctrl + C`. This sends a KeyboardInterrupt signal to the Python process, terminating the execution.
- Exit through code:
- You can use `sys.exit()` within your script to programmatically terminate it at any point. This is particularly useful for handling errors or conditions that require an early exit.
- Close the terminal:
- As a last resort, you can always close the terminal window. However, this may not be the cleanest method as it does not allow for graceful shutdowns.
Common Exit Commands
Here’s a summary table for quick reference regarding various exit methods in Python:
Method | Command | Description |
---|---|---|
Exit Python Interactive Shell | exit() | Type `exit()` and press Enter. |
Exit Python Interactive Shell | quit() | Type `quit()` and press Enter. |
Keyboard Interrupt | Ctrl + C | Interrupt and terminate running script in terminal. |
Use sys module | sys.exit() | Programmatically exit the script. |
Close Terminal | N/A | Close the terminal window to exit. |
By utilizing these methods, you can effectively manage your session in Python, whether in an interactive shell or running scripts in a terminal.
Exiting the Python Interpreter
When working in the Python interpreter via the terminal, there are several methods to exit. Depending on your environment and preferences, you can choose any of the following methods:
- Using the `exit()` Function: Simply type `exit()` and press Enter. This is a straightforward way to leave the Python shell.
- Using the `quit()` Function: Similar to `exit()`, typing `quit()` followed by Enter will also terminate the session.
- Keyboard Shortcut: You can use the keyboard shortcut `Ctrl + D` (on Unix/Linux and Mac) or `Ctrl + Z` followed by Enter (on Windows) to exit the interpreter quickly.
- Using `sys.exit()`: If you have imported the `sys` module, you can exit by calling `sys.exit()`. This method can be useful in scripts where you want to exit based on certain conditions.
Examples of Exiting Python
Here are examples demonstrating how to exit the Python interpreter using the methods mentioned:
“`python
Using exit()
>>> exit()
Using quit()
>>> quit()
Using sys.exit()
>>> import sys
>>> sys.exit()
“`
Common Pitfalls
While exiting the Python interpreter is generally straightforward, users may encounter certain pitfalls:
- Forgetting to Import `sys`: If you choose to use `sys.exit()` without importing the `sys` module, you will receive a `NameError`. Always ensure the module is imported before usage.
- Uncaught Exceptions: If you are in the middle of handling exceptions, exiting without resolving them may lead to confusion in your code’s flow.
- Interactive Mode: Ensure you are indeed in the Python interactive mode; otherwise, the commands above will not apply.
Exiting from a Running Script
When you are running a Python script and wish to exit before it has completed, you can use the following methods:
- Keyboard Interrupt: Press `Ctrl + C` in the terminal while the script is running. This raises a `KeyboardInterrupt` exception, stopping the execution immediately.
- Returning from a Function: If you have control over the code, you can use a return statement in a function to exit early from that function, eventually leading to the script’s end.
- Using Exit Codes: Implementing `sys.exit(
)` allows you to exit the script and optionally return a status code, which can be useful in batch processing or when called from other scripts.
Conclusion on Python Exit Techniques
Exiting the Python interpreter or a running script can be done efficiently using various methods. Understanding these techniques and their appropriate contexts enhances your ability to manage Python sessions effectively.
By being aware of common pitfalls and exit strategies, you can navigate your coding environment with greater ease and confidence.
Expert Insights on Exiting Python in Terminal
Dr. Emily Carter (Senior Software Engineer, CodeCraft Solutions). "To exit the Python interactive shell in the terminal, you can simply type 'exit()' or 'quit()' and press Enter. Alternatively, you can use the keyboard shortcut Ctrl + D, which is a quick way to terminate the session."
Michael Chen (Lead Developer, Tech Innovations Inc.). "When working in the Python terminal, it's important to remember that you can also exit by pressing Ctrl + Z on Windows or Ctrl + D on Unix-based systems. This effectively sends an EOF (End Of File) signal, which closes the interpreter."
Sarah Thompson (Python Instructor, LearnPythonNow Academy). "If you're running a script in Python and wish to exit the interpreter, using 'exit()' or 'quit()' is the most straightforward method. However, if you are in a loop or a blocking call, you may need to use Ctrl + C to interrupt the process before exiting."
Frequently Asked Questions (FAQs)
How do I exit the Python interpreter in the terminal?
To exit the Python interpreter, you can type `exit()` or `quit()` and press Enter. Alternatively, you can use the keyboard shortcut Ctrl + Z on Windows or Ctrl + D on Unix-based systems.
What happens if I press Ctrl + C in the Python terminal?
Pressing Ctrl + C sends a KeyboardInterrupt signal to the Python interpreter, which stops the current execution and returns you to the prompt. This does not exit the interpreter.
Can I close the terminal window to exit Python?
Yes, closing the terminal window will terminate the Python interpreter along with all running processes. However, this method does not allow for a graceful exit.
Is there a command to save my work before exiting Python?
Python does not have a built-in command to save your work directly. You should manually save your scripts in a text editor or use a version control system before exiting.
What if I want to exit Python but keep the terminal open?
You can exit Python while keeping the terminal open by using the `exit()` or `quit()` commands, which will return you to the shell prompt without closing the terminal.
Are there any differences in exiting Python between different operating systems?
The commands `exit()` and `quit()` work across all operating systems. However, the keyboard shortcuts differ: Ctrl + Z is used on Windows, while Ctrl + D is used on Unix-based systems.
In summary, exiting the Python interpreter in a terminal can be accomplished through several straightforward methods. The most common approach is to use the command `exit()` or `quit()`, both of which are built-in functions designed specifically for this purpose. Alternatively, users can also exit by pressing `Ctrl + D` on Unix-like systems or `Ctrl + Z` followed by `Enter` on Windows systems. Each of these methods effectively terminates the Python session and returns the user to the command prompt.
It is essential to be aware of these exit commands, especially for those who frequently engage with Python in a terminal environment. Understanding how to exit the interpreter not only enhances workflow efficiency but also prevents potential confusion for users who may be new to the Python programming language. Familiarity with these commands can significantly improve the overall user experience when working within the terminal.
Ultimately, knowing how to exit Python in the terminal is a fundamental skill for any programmer or developer. Mastering these commands ensures a smoother transition between coding sessions and the command line, thereby facilitating a more productive programming environment. As users grow more comfortable with Python, these simple yet crucial commands will become second nature, contributing to their overall proficiency in the language.
Author Profile
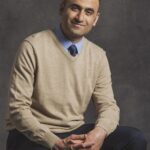
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?