How Can You Retrieve a Specific Key Value from a Dictionary in Python?
In the world of Python programming, dictionaries stand out as one of the most versatile and powerful data structures available. With their ability to store key-value pairs, they provide a seamless way to organize and access data efficiently. But as you delve deeper into the intricacies of dictionaries, you might find yourself asking a fundamental question: how do you retrieve a particular key’s value from a dictionary? Whether you’re a beginner looking to grasp the basics or an experienced programmer seeking to refine your skills, understanding this concept is essential for effective data manipulation in Python.
At its core, accessing values in a dictionary is straightforward, yet it opens the door to a myriad of possibilities in data handling. Python’s syntax allows for intuitive retrieval methods that can be applied in various scenarios, from simple lookups to more complex operations involving nested dictionaries. As you explore this topic, you’ll discover the nuances of different techniques, including the use of built-in functions and error handling, which can enhance your programming toolkit.
Moreover, the ability to extract specific values from dictionaries is not just a technical skill; it’s a gateway to unlocking the full potential of your data. By mastering this skill, you can streamline your code, optimize performance, and ultimately create more robust applications. So, let’s embark on this journey to demyst
Accessing Values by Keys
To retrieve a value associated with a specific key in a Python dictionary, you can utilize several methods. The most straightforward approach is using square brackets. If the key exists, this method returns the value; if not, it raises a `KeyError`.
Example:
“`python
my_dict = {‘name’: ‘Alice’, ‘age’: 30}
value = my_dict[‘name’] Returns ‘Alice’
“`
However, if you are uncertain whether the key exists, using the `get()` method is advisable. This method allows you to specify a default value that will be returned if the key is not found, preventing potential errors.
Example:
“`python
value = my_dict.get(‘gender’, ‘Not specified’) Returns ‘Not specified’
“`
Handling Missing Keys
When accessing dictionary values, handling missing keys gracefully is crucial. The approaches mentioned earlier serve this purpose effectively, but there are additional strategies worth considering:
- Using `in` Keyword: This checks for the existence of a key before attempting to access its value.
- Default Values: By providing a default value in the `get()` method, you can ensure that your program does not throw exceptions.
Example of using `in`:
“`python
if ‘age’ in my_dict:
value = my_dict[‘age’]
else:
value = ‘Key not found’
“`
Iterating Over Keys and Values
Sometimes, you may want to access multiple values from a dictionary. You can iterate over the keys and values using a loop. This can be particularly useful for processing data or generating reports.
Example:
“`python
for key, value in my_dict.items():
print(f”{key}: {value}”)
“`
Nested Dictionaries
In cases where dictionaries are nested, accessing a particular key value requires chaining the keys. This structure can be useful for organizing complex data.
Example of a nested dictionary:
“`python
person = {
‘name’: ‘Bob’,
‘details’: {
‘age’: 25,
‘address’: {
‘city’: ‘New York’,
‘zip’: ‘10001’
}
}
}
city = person[‘details’][‘address’][‘city’] Returns ‘New York’
“`
Table: Dictionary Access Methods
Method | Description | Example |
---|---|---|
Square Brackets | Direct access to a value using the key. | my_dict[‘key’] |
get() | Access with a default value if the key is absent. | my_dict.get(‘key’, ‘default’) |
in Keyword | Check if a key exists before accessing it. | if ‘key’ in my_dict: |
Iteration | Loop through keys and values. | for key, value in my_dict.items(): |
These methods provide a robust framework for accessing dictionary values in Python, accommodating various scenarios and data structures.
Accessing Values in a Dictionary
To retrieve a particular key’s value from a dictionary in Python, you can use the key directly in square brackets or the `get()` method. Each approach has its specific advantages.
Using Square Brackets
The most straightforward way to access a value is by using the key inside square brackets. This method will raise a `KeyError` if the key does not exist.
“`python
my_dict = {‘name’: ‘Alice’, ‘age’: 30, ‘city’: ‘New York’}
value = my_dict[‘name’] Returns ‘Alice’
“`
Considerations:
- Ensure the key exists to avoid exceptions.
- This method is concise and preferred when you are confident the key is present.
Using the get() Method
The `get()` method provides a safe way to retrieve values. It allows you to specify a default value if the key is not found, thus avoiding exceptions.
“`python
value = my_dict.get(‘age’, ‘Not Found’) Returns 30
value_not_found = my_dict.get(‘country’, ‘Not Found’) Returns ‘Not Found’
“`
Advantages:
- Prevents `KeyError` if the key is absent.
- Allows for custom default values.
Example of Dictionary Value Retrieval
The following table illustrates how both methods can be used in practice:
Method | Key | Output | Behavior on Missing Key |
---|---|---|---|
Square Brackets | ‘city’ | ‘New York’ | Raises `KeyError` |
`get()` | ‘country’ | ‘Not Found’ | Returns default value |
Nested Dictionaries
Accessing values in nested dictionaries requires chaining keys. For example, consider the following dictionary:
“`python
nested_dict = {
‘person’: {
‘name’: ‘Bob’,
‘age’: 25,
‘address’: {
‘city’: ‘Los Angeles’,
‘state’: ‘CA’
}
}
}
“`
To access the city value, you would use:
“`python
city = nested_dict[‘person’][‘address’][‘city’] Returns ‘Los Angeles’
“`
Alternatively, using the `get()` method can provide a safer approach:
“`python
city = nested_dict.get(‘person’, {}).get(‘address’, {}).get(‘city’, ‘Not Found’)
“`
Iterating Over Keys and Values
In scenarios where you need to find a key dynamically, iteration can be useful:
“`python
for key, value in my_dict.items():
if key == ‘name’:
print(value) Outputs: Alice
“`
This method allows for more complex logic and conditions when accessing dictionary values.
Expert Insights on Extracting Key Values from Python Dictionaries
Dr. Emily Carter (Senior Python Developer, Tech Innovations Inc.). “To retrieve a specific key value from a dictionary in Python, one can simply use the syntax `dictionary_name[key]`. This method is efficient and straightforward, provided that the key exists in the dictionary. If there is a possibility that the key may not be present, utilizing the `get()` method is advisable, as it allows for a default value to be returned instead of raising an error.”
James Liu (Data Scientist, AI Research Lab). “In Python, dictionaries are inherently designed for quick lookups, making them ideal for accessing key values. When you’re unsure if a key exists, using `if key in dictionary_name:` can prevent potential KeyErrors. This practice enhances code robustness, especially in data-driven applications where dictionary keys may vary.”
Sarah Thompson (Software Engineer, CodeCraft Solutions). “For more complex scenarios, such as nested dictionaries, one can access values by chaining keys, like `dictionary_name[key1][key2]`. This method is powerful but requires careful handling to avoid errors. Always ensure that each key in the chain exists to maintain the integrity of your code.”
Frequently Asked Questions (FAQs)
How can I access a specific key’s value in a Python dictionary?
You can access a specific key’s value by using square brackets or the `get()` method. For example, `value = my_dict[‘key’]` or `value = my_dict.get(‘key’)`.
What happens if I try to access a key that does not exist in the dictionary?
If you use square brackets and the key does not exist, a `KeyError` will be raised. Using the `get()` method will return `None` or a specified default value instead.
Can I retrieve multiple values from a dictionary at once?
Yes, you can retrieve multiple values by using a list of keys in a list comprehension. For example, `values = [my_dict[key] for key in keys if key in my_dict]`.
Is there a way to check if a key exists in a dictionary before accessing its value?
Yes, you can use the `in` keyword to check if a key exists. For example, `if ‘key’ in my_dict:` allows you to safely access the value without raising an error.
What is the difference between using `get()` and direct access with brackets?
The `get()` method provides a safer way to access values, allowing you to specify a default value if the key is not found, whereas direct access with brackets will raise an error if the key does not exist.
Can I use nested dictionaries to access values in Python?
Yes, you can use nested dictionaries. Access values by chaining keys, such as `value = my_dict[‘outer_key’][‘inner_key’]`, ensuring that each key exists to avoid errors.
In Python, retrieving a specific key’s value from a dictionary is a straightforward process that can be accomplished using several methods. The most common approach is to use the bracket notation, where you specify the key within square brackets following the dictionary’s name. Alternatively, the `get()` method can be employed, which allows for a more graceful handling of cases where the key might not exist, as it can return a default value instead of raising a KeyError.
Understanding the nuances between these methods is crucial for effective dictionary manipulation. While bracket notation is concise and direct, it is essential to ensure that the key exists to avoid exceptions. On the other hand, the `get()` method provides a safer option, especially in scenarios where the presence of the key is uncertain. This flexibility can enhance the robustness of your code and prevent runtime errors.
In summary, mastering the retrieval of key values from dictionaries in Python is fundamental for efficient data handling. By leveraging either the bracket notation or the `get()` method, developers can access values seamlessly while maintaining code integrity. Ultimately, choosing the appropriate method depends on the specific requirements of the task at hand and the need for error handling.
Author Profile
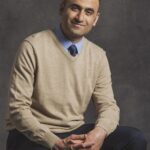
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?