How Can You Check the Status of a Kubernetes Node Using Golang?
In the ever-evolving landscape of cloud-native technologies, Kubernetes has emerged as a cornerstone for deploying, managing, and scaling applications. As organizations increasingly rely on this powerful orchestration tool, understanding the status of Kubernetes nodes becomes crucial for maintaining optimal performance and reliability. Whether you’re a seasoned developer or a newcomer to the Kubernetes ecosystem, leveraging Go (Golang) to interact with the Kubernetes API can unlock a wealth of insights that enhance your operational capabilities.
In this article, we will delve into the process of retrieving the status of Kubernetes nodes using Go. By tapping into the Kubernetes client-go library, you can seamlessly communicate with your cluster and extract vital information about node health, resource utilization, and overall cluster performance. This knowledge not only aids in troubleshooting but also empowers you to make informed decisions about scaling and resource allocation.
As we explore this topic, we will cover the essential steps to set up your Go environment for Kubernetes interactions, the key API calls required to fetch node status, and best practices for handling the data you retrieve. Whether you’re looking to automate monitoring tasks or simply gain a deeper understanding of your Kubernetes infrastructure, this guide will equip you with the tools and techniques necessary to harness the full potential of Go in your Kubernetes journey.
Using Go Client for Kubernetes
To get the status of a Kubernetes node using Go, you will need to utilize the official Kubernetes Go client. This client provides a comprehensive API for interacting with a Kubernetes cluster. Below are the steps involved in setting up the client and retrieving the status of a node.
Setting Up the Go Client
- Install the Kubernetes Client-Go Library: First, you need to install the Kubernetes client-go library. You can do this by running the following command:
“`bash
go get k8s.io/client-go@latest
“`
- Configure Your Kubernetes Client: You will need to create a Kubernetes client configuration. This can typically be done by loading the kubeconfig file.
“`go
import (
“context”
“fmt”
“k8s.io/client-go/kubernetes”
“k8s.io/client-go/tools/clientcmd”
)
func getKubeClient() (*kubernetes.Clientset, error) {
kubeconfig := “/path/to/your/kubeconfig”
config, err := clientcmd.BuildConfigFromFlags(“”, kubeconfig)
if err != nil {
return nil, err
}
return kubernetes.NewForConfig(config)
}
“`
Retrieving Node Status
Once you have the client set up, you can retrieve the status of a node by accessing the nodes API. Below is a code snippet demonstrating how to list nodes and obtain their statuses.
“`go
import (
“context”
“fmt”
“k8s.io/api/core/v1”
metav1 “k8s.io/apimachinery/pkg/apis/meta/v1”
)
func getNodeStatus(clientset *kubernetes.Clientset) {
nodes, err := clientset.CoreV1().Nodes().List(context.TODO(), metav1.ListOptions{})
if err != nil {
fmt.Printf(“Error fetching nodes: %v\n”, err)
return
}
for _, node := range nodes.Items {
fmt.Printf(“Name: %s\n”, node.Name)
fmt.Printf(“Status: %s\n”, node.Status.Conditions)
}
}
“`
Understanding Node Conditions
The `Conditions` field of the node status provides vital information about the health and availability of the node. Each condition contains a type, status, and reason. Below is a table summarizing key condition types:
Condition Type | Description |
---|---|
Ready | Indicates whether the node is ready to accept pods. |
DiskPressure | Indicates whether the node is under disk pressure. |
MemoryPressure | Indicates whether the node is under memory pressure. |
PIDPressure | Indicates whether the node is under PID pressure. |
NetworkUnavailable | Indicates whether the network is available on the node. |
By checking these conditions, you can ascertain the health of your Kubernetes nodes and take necessary action if any issues are detected.
Accessing Kubernetes Node Status with Go
To retrieve the status of Kubernetes nodes using Go, you can utilize the official Kubernetes client-go library. This library provides an interface to communicate with the Kubernetes API server and access various resources, including nodes.
Setting Up Your Go Environment
- Install Go: Ensure you have Go installed on your machine. You can download it from the official Go website.
- Create a Go Module: Initialize your Go module by running the following commands:
“`bash
mkdir k8s-node-status
cd k8s-node-status
go mod init k8s-node-status
“`
- Install client-go: Add the Kubernetes client-go library to your module:
“`bash
go get k8s.io/client-go@latest
go get k8s.io/apimachinery@latest
“`
Code to Get Node Status
The following code snippet demonstrates how to connect to the Kubernetes cluster and retrieve the status of nodes:
“`go
package main
import (
“context”
“fmt”
“log”
“os”
metav1 “k8s.io/apimachinery/pkg/apis/meta/v1”
“k8s.io/client-go/kubernetes”
“k8s.io/client-go/tools/clientcmd”
)
func main() {
// Load the kubeconfig file
kubeconfig := os.Getenv(“KUBECONFIG”)
if kubeconfig == “” {
kubeconfig = “/path/to/your/kubeconfig.yaml” // Update with your kubeconfig path
}
config, err := clientcmd.BuildConfigFromFlags(“”, kubeconfig)
if err != nil {
log.Fatalf(“Error building kubeconfig: %s”, err.Error())
}
// Create a clientset
clientset, err := kubernetes.NewForConfig(config)
if err != nil {
log.Fatalf(“Error creating Kubernetes client: %s”, err.Error())
}
// Get the nodes
nodes, err := clientset.CoreV1().Nodes().List(context.TODO(), metav1.ListOptions{})
if err != nil {
log.Fatalf(“Error fetching nodes: %s”, err.Error())
}
// Print node statuses
for _, node := range nodes.Items {
fmt.Printf(“Name: %s, Status: %s\n”, node.Name, node.Status.Conditions[0].Status)
}
}
“`
Understanding the Code
- Kubeconfig: The path to your Kubernetes configuration file, typically located at `~/.kube/config`.
- Clientset: An instance of Kubernetes client that provides access to the Kubernetes API.
- Node List: The code fetches a list of nodes using `clientset.CoreV1().Nodes().List`.
- Output: Each node’s name and its status are printed, specifically focusing on the first condition in the status.
Running the Program
- Ensure your Go environment is correctly set up with access to your Kubernetes cluster.
- Execute the program:
“`bash
go run main.go
“`
This command will compile and run your Go application, printing the status of each node in your Kubernetes cluster.
Additional Considerations
- Error Handling: Ensure proper error handling is implemented for production use, especially in network calls.
- Node Conditions: You may want to loop through all conditions of the node to fetch more detailed status information.
- Permissions: Ensure the service account you are using has the necessary permissions to access node resources in your Kubernetes cluster.
This approach provides a straightforward method for accessing and displaying the status of Kubernetes nodes programmatically using Go.
Expert Insights on Retrieving Kubernetes Node Status with Go
Dr. Emily Chen (Kubernetes Specialist, CloudTech Innovations). “To effectively retrieve the status of a Kubernetes node using Go, one must leverage the client-go library, which provides a robust interface for interacting with the Kubernetes API. By creating a clientset and using the corev1.Nodes().Get method, developers can easily access node information, including its health and conditions.”
Michael Thompson (Senior Software Engineer, DevOps Solutions). “When implementing a solution in Go to check the status of Kubernetes nodes, it’s crucial to handle errors gracefully. Network issues or API changes can lead to unexpected results. Therefore, implementing retries and logging will enhance the reliability of your application while interacting with the Kubernetes API.”
Jessica Patel (Cloud Infrastructure Architect, TechWave). “In addition to using client-go, consider utilizing the Kubernetes Informers to monitor node status changes in real-time. This approach allows for efficient resource utilization and ensures your application can respond promptly to changes in the cluster’s state.”
Frequently Asked Questions (FAQs)
How can I check the status of a Kubernetes node using Go?
You can check the status of a Kubernetes node in Go by using the client-go library. First, create a Kubernetes client, then use the `CoreV1().Nodes()` method to retrieve the node objects and their statuses.
What libraries do I need to use to interact with Kubernetes in Go?
To interact with Kubernetes in Go, you primarily need the client-go library, which provides the necessary APIs to communicate with the Kubernetes cluster. Additionally, you may need other libraries for specific functionalities, such as controller-runtime for building controllers.
How do I authenticate with a Kubernetes cluster in Go?
Authentication with a Kubernetes cluster in Go can be achieved by using a kubeconfig file, which contains the necessary credentials. You can load the configuration using `clientcmd.BuildConfigFromFlags()` to create a client configuration.
What information can I retrieve about a Kubernetes node?
You can retrieve various pieces of information about a Kubernetes node, including its name, status, conditions, capacity, allocatable resources, and labels. This data is crucial for monitoring and managing cluster resources.
How do I handle errors when fetching node status in Go?
When fetching node status in Go, you should implement error handling using Go’s error checking conventions. Check for errors after each API call and log or handle them appropriately to ensure robust application behavior.
Can I use the Kubernetes API directly instead of client-go?
Yes, you can use the Kubernetes API directly by making HTTP requests to the API server. However, using client-go is recommended as it simplifies the process of interacting with the API and handles authentication, retries, and other complexities.
To retrieve the status of a Kubernetes node using Go, developers can leverage the official Kubernetes client-go library. This library provides a robust set of tools and APIs that facilitate interaction with the Kubernetes API server, allowing for efficient querying of node status and other resources. By utilizing the client-go library, developers can easily authenticate with the Kubernetes cluster, construct the necessary API requests, and handle the responses to extract relevant node information.
Key steps in this process include setting up the client configuration, creating a clientset, and using the appropriate methods to access node resources. Specifically, developers can utilize the `CoreV1().Nodes()` interface to list or get specific node details. Additionally, it is important to handle errors and ensure that the context is properly managed during API calls to maintain application stability and performance.
In summary, using Go to get the status of Kubernetes nodes is a straightforward task when employing the client-go library. This approach not only simplifies the interaction with the Kubernetes API but also promotes best practices in error handling and resource management. With the growing importance of Kubernetes in cloud-native applications, mastering these techniques is essential for developers aiming to build efficient and scalable solutions.
Author Profile
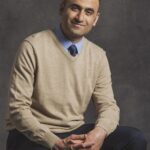
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?