How Can You Create Text Box Graphics in Python?
In the realm of Python programming, the ability to create visually appealing interfaces is essential for enhancing user experience and engagement. One of the key elements in building interactive applications is the use of graphics, particularly text boxes. Whether you’re developing a simple desktop application or a complex game, incorporating text box graphics can significantly elevate your project. This article will guide you through the process of integrating text box graphics into your Python applications, unlocking new possibilities for creativity and functionality.
Creating text box graphics in Python opens up a world of opportunities for developers looking to make their applications more user-friendly and visually appealing. Text boxes serve as fundamental components for user input, allowing users to interact seamlessly with your application. By leveraging various libraries and frameworks available in Python, you can easily implement these graphics, customizing them to fit your design needs and enhance the overall user experience.
As we delve deeper into this topic, we’ll explore the different libraries that can be utilized for creating text box graphics, along with practical examples and tips to streamline your development process. Whether you’re a beginner seeking to understand the basics or an experienced developer looking to refine your skills, this guide will provide valuable insights into making your Python applications stand out with dynamic text box graphics.
Creating Text Box Graphics in Python
To create text box graphics in Python, several libraries can be utilized, with `Tkinter`, `Pygame`, and `Matplotlib` being among the most popular. Each library has its own strengths and is suited for different types of applications. Below, we will delve into how to implement text box graphics using these libraries.
Using Tkinter for Text Box Graphics
`Tkinter` is the standard GUI toolkit for Python and provides a straightforward way to create text boxes. To create a simple text box, you can use the `Entry` or `Text` widget, depending on whether you need a single-line or multi-line text input.
Here’s how to create a basic text box:
“`python
import tkinter as tk
def submit_action():
print(entry.get())
root = tk.Tk()
entry = tk.Entry(root)
entry.pack()
submit_button = tk.Button(root, text=”Submit”, command=submit_action)
submit_button.pack()
root.mainloop()
“`
This code snippet creates a window with a text box and a submit button. When the button is pressed, the text entered in the box is printed to the console.
Utilizing Pygame for Text Box Graphics
For game development or graphical applications, `Pygame` is a powerful library that allows for more control over graphics. To create a text box in Pygame, you would typically render text to the screen in a defined area.
Here is an example:
“`python
import pygame
import sys
pygame.init()
screen = pygame.display.set_mode((400, 300))
font = pygame.font.Font(None, 36)
input_box = pygame.Rect(100, 100, 200, 50)
color_inactive = pygame.Color(‘lightskyblue3’)
color_active = pygame.Color(‘dodgerblue2’)
color = color_inactive
text = ”
active =
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
if event.type == pygame.MOUSEBUTTONDOWN:
if input_box.collidepoint(event.pos):
active = not active
else:
active =
color = color_active if active else color_inactive
if event.type == pygame.KEYDOWN:
if active:
if event.key == pygame.K_RETURN:
print(text)
text = ”
elif event.key == pygame.K_BACKSPACE:
text = text[:-1]
else:
text += event.unicode
screen.fill((30, 30, 30))
txt_surface = font.render(text, True, color)
width = max(200, txt_surface.get_width()+10)
input_box.w = width
screen.blit(txt_surface, (input_box.x+5, input_box.y+5))
pygame.draw.rect(screen, color, input_box, 2)
pygame.display.flip()
“`
In this example, a text input box is created that allows users to enter text. The text is displayed in real-time and can be submitted by pressing the Enter key.
Employing Matplotlib for Text Box Annotations
`Matplotlib` can also be used to create text boxes, particularly for annotations in plots. The `text` function allows for placing text at a specific location within a figure.
Here’s a simple example:
“`python
import matplotlib.pyplot as plt
plt.figure()
plt.plot([0, 1], [0, 1])
plt.text(0.5, 0.5, ‘Sample Text Box’, bbox=dict(facecolor=’white’, alpha=0.5))
plt.show()
“`
This code generates a plot with a semi-transparent text box at the center, demonstrating how to annotate graphics with text.
Comparison of Libraries for Text Box Graphics
Library | Best For | Complexity | Features |
---|---|---|---|
Tkinter | GUI Applications | Low | Simple text boxes and buttons |
Pygame | Game Development | Medium | Dynamic graphics and real-time input |
Matplotlib | Data Visualization | Medium | Plot annotations |
By evaluating the needs of your project and the capabilities of each library, you can choose the most suitable method for implementing text box graphics in Python.
Creating Text Box Graphics in Python
To create text box graphics in Python, several libraries can be utilized depending on the requirements of your project. The most commonly used libraries for graphical user interfaces (GUIs) and visualizations include Tkinter, Pygame, and Matplotlib. Below are methodologies for implementing text box graphics using these libraries.
Using Tkinter for Text Boxes
Tkinter is the standard GUI toolkit for Python and is suitable for creating simple applications with text boxes.
- Installation: Tkinter is included with Python installations. Ensure you have Python installed on your system.
- Example Code:
“`python
import tkinter as tk
def submit_action():
print(“Text Box Input:”, entry.get())
root = tk.Tk()
root.title(“Text Box Example”)
label = tk.Label(root, text=”Enter text:”)
label.pack()
entry = tk.Entry(root, width=30)
entry.pack()
submit_button = tk.Button(root, text=”Submit”, command=submit_action)
submit_button.pack()
root.mainloop()
“`
- Features:
- Text entry using the `Entry` widget.
- Button to trigger actions based on input.
Creating Text Boxes with Pygame
Pygame is a library designed for writing video games, but it can also be used for creating dynamic graphics, including text boxes.
- Installation: Use pip to install Pygame.
“`bash
pip install pygame
“`
- Example Code:
“`python
import pygame
import sys
pygame.init()
screen = pygame.display.set_mode((400, 300))
font = pygame.font.Font(None, 36)
input_box = pygame.Rect(100, 100, 200, 50)
color_inactive = pygame.Color(‘lightskyblue3’)
color_active = pygame.Color(‘dodgerblue2’)
color = color_inactive
text = ”
active =
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
if event.type == pygame.MOUSEBUTTONDOWN:
if input_box.collidepoint(event.pos):
active = not active
else:
active =
color = color_active if active else color_inactive
if event.type == pygame.KEYDOWN:
if active:
if event.key == pygame.K_RETURN:
print(“Input:”, text)
text = ”
elif event.key == pygame.K_BACKSPACE:
text = text[:-1]
else:
text += event.unicode
screen.fill((30, 30, 30))
txt_surface = font.render(text, True, color)
width = max(200, txt_surface.get_width()+10)
input_box.w = width
screen.blit(txt_surface, (input_box.x+5, input_box.y+5))
pygame.draw.rect(screen, color, input_box, 2)
pygame.display.flip()
“`
- Features:
- Interactive text box to capture user input.
- Real-time input handling with keyboard events.
Implementing Text Boxes in Matplotlib
Matplotlib is primarily a plotting library, but it offers functionalities to create text boxes within plots.
- Installation: Install Matplotlib via pip if not already installed.
“`bash
pip install matplotlib
“`
- Example Code:
“`python
import matplotlib.pyplot as plt
fig, ax = plt.subplots()
ax.set_title(‘Text Box in Matplotlib’)
Create a text box
text_box = plt.text(0.5, 0.5, ‘This is a text box’, fontsize=12, ha=’center’, va=’center’,
bbox=dict(boxstyle=’round’, facecolor=’lightblue’, edgecolor=’blue’, alpha=0.5))
plt.show()
“`
- Features:
- Customizable text box with background color and border.
- Integration within plots for annotations and descriptions.
Comparison of Libraries
Library | Use Case | Ease of Use | Customization Level | Ideal For |
---|---|---|---|---|
Tkinter | Simple GUI applications | High | Medium | Basic applications |
Pygame | Game development and graphics | Medium | High | Interactive graphics |
Matplotlib | Data visualization and plotting | High | Medium | Scientific plots |
By selecting the appropriate library based on your project needs, you can efficiently implement text box graphics in Python.
Expert Insights on Creating Text Box Graphics in Python
Dr. Emily Carter (Senior Data Visualization Specialist, TechViz Solutions). “To create effective text box graphics in Python, utilizing libraries such as Matplotlib and Seaborn is essential. These libraries provide robust tools for customizing text boxes, including positioning, styling, and integrating them seamlessly into your visualizations.”
Mark Thompson (Lead Software Engineer, CodeCraft Innovations). “Incorporating text boxes in your Python graphics can enhance user understanding. I recommend exploring the Tkinter library for GUI applications, as it allows for the easy addition of text boxes with interactive features, making your application more user-friendly.”
Laura Kim (Data Science Consultant, Insightful Analytics). “For those looking to add text boxes to plots, I suggest using Plotly. This library not only supports dynamic text boxes but also offers interactivity, which can significantly improve the user experience when presenting data.”
Frequently Asked Questions (FAQs)
How can I create a text box in Python using Tkinter?
You can create a text box in Python using the Tkinter library by utilizing the `Text` widget. Here’s a simple example:
“`python
import tkinter as tk
root = tk.Tk()
text_box = tk.Text(root, height=10, width=40)
text_box.pack()
root.mainloop()
“`
What libraries can I use to create graphics with text boxes in Python?
You can use several libraries to create graphics with text boxes in Python, including Tkinter, Pygame, and Matplotlib. Each library has its own methods for rendering text and graphics.
How do I customize the appearance of a text box in Tkinter?
You can customize the appearance of a text box in Tkinter by using options such as `bg` for background color, `fg` for text color, and `font` for font style. For example:
“`python
text_box = tk.Text(root, bg=’lightblue’, fg=’black’, font=(‘Arial’, 12))
“`
Can I add borders to a text box in Tkinter?
Yes, you can add borders to a text box in Tkinter by using the `bd` (border width) option. For instance:
“`python
text_box = tk.Text(root, bd=2)
“`
How can I retrieve text from a text box in Tkinter?
You can retrieve text from a text box in Tkinter using the `get` method. Specify the range of characters you want to retrieve, for example:
“`python
text_content = text_box.get(“1.0”, “end-1c”) Gets all text from the box
“`
Is it possible to create a text box with multiple lines in Pygame?
Yes, you can create a multi-line text box in Pygame by managing the text rendering manually. You can use the `pygame.font.Font` class to render each line of text separately and display them in a designated area.
In summary, creating text box graphics in Python can be achieved through various libraries that cater to different needs and preferences. Libraries such as Tkinter, Pygame, and Matplotlib provide robust options for designing and rendering text boxes with customizable features. Each library has its unique strengths, making it essential to choose one that aligns with the specific requirements of your project.
For instance, Tkinter is an excellent choice for building GUI applications, offering straightforward methods to create text boxes with user interaction capabilities. On the other hand, Pygame is more suited for game development, allowing for dynamic text rendering within graphical environments. Meanwhile, Matplotlib excels in creating visualizations, including annotated text boxes, which can enhance the clarity of data presentations.
Additionally, understanding the properties and methods associated with each library can significantly improve the quality and functionality of your text box graphics. It is advisable to explore the documentation and community resources available for each library to maximize their potential. By leveraging the right tools and techniques, developers can create visually appealing and functional text box graphics in Python.
Author Profile
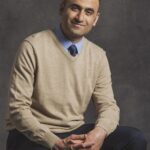
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?