How Can You Retrieve the Current Month by Name in Python?
In the world of programming, working with dates and times is a fundamental skill that can significantly enhance the functionality of your applications. Whether you’re developing a calendar feature, generating reports, or simply logging events, knowing how to manipulate and display dates is crucial. One common requirement is to retrieve the current month by name, which can add a layer of readability and context to your data. In this article, we’ll explore how to easily obtain the current month in Python using its built-in libraries, enabling you to present date-related information in a user-friendly manner.
Python offers a variety of libraries that simplify date and time manipulation, making it accessible for developers of all levels. By leveraging these libraries, you can seamlessly extract the current month in a format that is both intuitive and informative. Understanding how to convert numerical representations of months into their corresponding names not only enhances your code’s clarity but also improves user experience.
As we delve deeper into the topic, we will cover the methods available for achieving this task, including the use of the `datetime` module and other relevant functions. Whether you are a seasoned programmer or just starting your coding journey, mastering this technique will empower you to create more dynamic and engaging applications. Join us as we unlock the simple yet powerful ways to get the current month by
Getting the Current Month by Name in Python
To retrieve the current month by name in Python, you can utilize the `datetime` module, which provides classes for manipulating dates and times. The `datetime` class has a method that allows you to access the current date, and from there, you can extract the month and convert it into its corresponding name.
Here’s a straightforward approach to accomplish this:
- Import the `datetime` module.
- Get the current date using `datetime.now()`.
- Use the `strftime` method to format the date and retrieve the month’s name.
Here’s a sample code snippet demonstrating this:
python
from datetime import datetime
# Get the current date
current_date = datetime.now()
# Format the month as a full name
current_month_name = current_date.strftime(“%B”)
print(“Current Month:”, current_month_name)
In this snippet, the `strftime` method is used with the format specifier `%B`, which returns the full month name.
Alternative Methods to Retrieve the Month Name
While the above method is effective, there are alternative approaches to get the current month name, such as using the `calendar` module.
### Using the Calendar Module
The `calendar` module can also provide the names of the months. You can access the month name by its index:
python
import calendar
from datetime import datetime
# Get the current month number
current_month_index = datetime.now().month
# Retrieve the month name
current_month_name = calendar.month_name[current_month_index]
print(“Current Month:”, current_month_name)
This method retrieves the month number from the `datetime` object and uses it to fetch the corresponding name from the `calendar.month_name` list.
Comparison of Methods
Method | Description | Complexity |
---|---|---|
`strftime` | Directly formats the date to get the month name. | Low |
`calendar.month_name` | Uses index to fetch month name from a list. | Medium |
Both methods are efficient; however, using `strftime` is often more straightforward for simply obtaining the month name.
In summary, retrieving the current month by name in Python can be easily achieved using either the `datetime` or `calendar` modules. Each approach has its advantages depending on the context of the application.
Using the datetime Module
To obtain the current month in Python by name, the `datetime` module provides a straightforward approach. This module includes various classes for manipulating dates and times, and it allows you to easily extract the month.
python
from datetime import datetime
# Get the current date
current_date = datetime.now()
# Extract the month name
current_month_name = current_date.strftime(“%B”)
print(current_month_name)
In this example, `strftime` is used with the format code `%B`, which returns the full month name.
Alternative Method with Calendar Module
Another method to obtain the current month name is through the `calendar` module. This module provides useful functions related to the calendar.
python
import calendar
from datetime import datetime
# Get the current month number
current_month_number = datetime.now().month
# Get the month name using the calendar module
current_month_name = calendar.month_name[current_month_number]
print(current_month_name)
This code first retrieves the current month number and then accesses the `month_name` list, which contains names of the months indexed from 1 to 12.
Using a List of Month Names
You can also create a custom list of month names if you prefer to avoid built-in modules. This method is simple and allows for customization.
python
# List of month names
month_names = [
“January”, “February”, “March”, “April”, “May”, “June”,
“July”, “August”, “September”, “October”, “November”, “December”
]
# Get the current month number
current_month_number = datetime.now().month
# Get the month name from the list
current_month_name = month_names[current_month_number – 1]
print(current_month_name)
By using a predefined list, you can easily manage the month names and retrieve them based on the current month number.
Summary of Methods
Method | Code Example | Description |
---|---|---|
datetime module | `current_date.strftime(“%B”)` | Directly retrieves the full month name. |
calendar module | `calendar.month_name[current_month_number]` | Uses the month number to access month names. |
Custom list of month names | `month_names[current_month_number – 1]` | Customizable list for month names. |
Each method offers a reliable way to get the current month by name in Python, allowing you to choose based on your specific needs or preferences.
Expert Insights on Retrieving the Current Month by Name in Python
Dr. Emily Carter (Senior Software Engineer, Python Development Institute). “To retrieve the current month by name in Python, utilizing the `datetime` module is essential. By using `datetime.now()` combined with `strftime(‘%B’)`, developers can effectively obtain the full month name, which is both intuitive and efficient.”
Michael Thompson (Lead Data Scientist, Tech Innovations Lab). “When working with date and time in Python, leveraging the `calendar` module can also be beneficial. The `calendar.month_name` list allows for a straightforward approach to access month names, making it a great option for those who prefer a list-based method.”
Jessica Lin (Python Instructor, Code Academy). “For beginners, I recommend using the `strftime` method as it not only retrieves the month name but also enhances understanding of date formatting in Python. This method is widely applicable in various programming scenarios, making it a fundamental skill for new programmers.”
Frequently Asked Questions (FAQs)
How can I get the current month in Python by name?
You can use the `datetime` module in Python. Import the module and use `datetime.now().strftime(‘%B’)` to retrieve the full name of the current month.
What does the `strftime` function do in Python?
The `strftime` function formats date and time objects as strings. It allows you to specify the format in which you want to display the date and time.
Can I get the abbreviated name of the current month in Python?
Yes, you can obtain the abbreviated month name by using `datetime.now().strftime(‘%b’)`, which will return a three-letter abbreviation of the current month.
Is it possible to get the month number along with its name in Python?
Yes, you can concatenate the month number with its name by using `datetime.now().strftime(‘%m %B’)`, which will return the month number followed by the full month name.
What if I want the month name in a different language?
To get the month name in a different language, you can use the `locale` module to set the desired locale before calling `strftime`. For example, `locale.setlocale(locale.LC_TIME, ‘fr_FR’)` for French.
Are there any libraries that simplify date and time handling in Python?
Yes, libraries such as `pandas` and `arrow` provide more advanced functionalities for date and time manipulation, making it easier to work with dates in various formats.
In Python, obtaining the current month by name can be accomplished using the built-in `datetime` module. This module provides a straightforward way to access the current date and time, from which the month can be extracted. By utilizing the `strftime` method, one can format the date to display the month as a full name, such as “January” or “February.” This method is both efficient and easy to implement, making it a popular choice among developers.
To achieve this, you can first import the `datetime` module and then call `datetime.datetime.now()` to get the current date and time. Following this, the `strftime(‘%B’)` function can be applied to retrieve the full month name. This approach is not only simple but also versatile, allowing for various formatting options to suit different requirements.
In summary, Python offers a convenient way to get the current month by name through the `datetime` module. This functionality is essential for applications that require date manipulation or display. By understanding and utilizing these built-in features, developers can enhance their code’s readability and functionality while adhering to best practices in date handling.
Author Profile
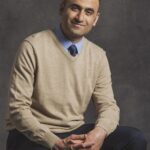
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?