How Can You Easily Retrieve Input Values in JavaScript?
In the ever-evolving landscape of web development, JavaScript stands as a cornerstone language that empowers developers to create dynamic and interactive user experiences. One of the fundamental aspects of building responsive web applications is the ability to capture user input effectively. Whether you’re designing a simple form for user feedback or a complex interface for data entry, understanding how to get the input value in JavaScript is crucial. This skill not only enhances user engagement but also ensures that your applications function smoothly and intuitively.
At its core, retrieving input values in JavaScript involves interacting with HTML elements through the Document Object Model (DOM). By leveraging various methods and properties, developers can access and manipulate the data entered by users, enabling real-time updates and validations. This process is essential for tasks such as form submissions, data processing, and user interactions, all of which contribute to a seamless user experience.
As we delve deeper into this topic, we will explore the different techniques and best practices for capturing input values in JavaScript. From understanding event listeners to utilizing modern frameworks, you’ll gain insights that will empower you to harness user input effectively, paving the way for more interactive and responsive web applications. Whether you’re a novice just starting out or an experienced developer looking to refine your skills, mastering this aspect of JavaScript will undoubtedly enhance
Accessing Input Values Using JavaScript
To retrieve the value from an input field in JavaScript, you can use the Document Object Model (DOM) methods. The most common approach is to select the input element and then access its `value` property. Here are the steps to achieve this:
- Select the Input Element: You can use methods like `getElementById`, `getElementsByClassName`, or `querySelector` to select the input element.
- Access the Value: Once you have a reference to the input element, simply read its `value` property.
Here’s an example of how to do this:
In this example, when the button is clicked, the current value of the input field is retrieved and displayed in a paragraph.
Different Input Types
JavaScript can handle various input types, and the method to access their values remains consistent. Here are some common input types and their usage:
- Text Input: Standard text field for user input.
- Checkbox: Used for boolean values (true/).
- Radio Buttons: Allows selection of one option among multiple choices.
- Select Dropdown: Used for selecting an option from a list.
The following table summarizes how to retrieve values from different input types:
Input Type | JavaScript Code | Description |
---|---|---|
Text | document.getElementById(“inputId”).value; | Gets the text from a text input. |
Checkbox | document.getElementById(“checkboxId”).checked; | Returns true if checked, otherwise. |
Radio | document.querySelector(“input[name=’groupName’]:checked”).value; | Gets the value of the selected radio button. |
Select | document.getElementById(“selectId”).value; | Gets the selected option value from a dropdown. |
Handling Input Events
In addition to directly accessing input values, you can also capture user input dynamically by listening to events. Common events include `input`, `change`, and `focus`. Here’s how to use these events:
- Input Event: Triggered whenever the value of an input changes.
- Change Event: Triggered when an input loses focus after its value has changed.
- Focus Event: Triggered when the input gains focus.
Here’s an example of using the `input` event:
This code updates the displayed text in real-time as the user types in the input field.
By understanding these methods and events, you can effectively handle and manipulate input values in your JavaScript applications.
Accessing Input Values
To retrieve the value from an input element in JavaScript, you typically interact with the Document Object Model (DOM). Here are the primary methods to access input values:
- Using `getElementById`:
This method is one of the most common ways to access an input element directly by its unique ID.
javascript
var inputValue = document.getElementById(‘inputId’).value;
- Using `querySelector`:
This method allows for more flexibility with CSS selectors, making it easier to select elements that may not have an ID.
javascript
var inputValue = document.querySelector(‘.inputClass’).value;
- Using `getElementsByClassName`:
This method retrieves elements by their class name. Note that it returns a collection, so you may need to specify the index.
javascript
var inputValue = document.getElementsByClassName(‘inputClass’)[0].value;
- Using `getElementsByName`:
This method is useful when your input elements share a name attribute. It also returns a collection.
javascript
var inputValue = document.getElementsByName(‘inputName’)[0].value;
Event Handling for Input Values
To get the value of an input field dynamically as the user types, you can utilize event listeners. The most common events are `input` and `change`.
- Using the `input` event: This event triggers whenever the value of an input changes.
javascript
var inputField = document.getElementById(‘inputId’);
inputField.addEventListener(‘input’, function() {
console.log(inputField.value);
});
- Using the `change` event: This event triggers when the input loses focus and its value has changed.
javascript
var inputField = document.getElementById(‘inputId’);
inputField.addEventListener(‘change’, function() {
console.log(inputField.value);
});
Working with Different Input Types
Different types of input elements require similar but slightly varied approaches. Below is a table summarizing how to access values from various input types:
Input Type | Example Code | Notes |
---|---|---|
Text | `document.getElementById(‘textInput’).value` | Standard text input |
Checkbox | `document.getElementById(‘checkboxInput’).checked` | Use `checked` for boolean value |
Radio | `document.querySelector(‘input[name=”radioGroup”]:checked’).value` | Gets selected radio button value |
Select (dropdown) | `document.getElementById(‘selectInput’).value` | Retrieves selected option value |
Textarea | `document.getElementById(‘textareaInput’).value` | Retrieves text from a textarea |
Best Practices for Input Handling
When working with input elements, consider these best practices:
- Validation: Always validate input values to ensure they conform to expected formats (e.g., email, numbers).
- Accessibility: Use labels associated with input fields for better accessibility. Use the `for` attribute in `
- Debouncing Input Events: For performance, especially in forms with multiple inputs, debounce input events to limit the number of times functions are called.
- Use Modern Features: Consider using `async` functions or Promises when dealing with inputs that require server validation.
By adhering to these methods and practices, you can effectively manage user input in your JavaScript applications.
Expert Insights on Retrieving Input Values in JavaScript
Dr. Emily Carter (Senior Web Developer, Tech Innovations Inc.). “To effectively retrieve input values in JavaScript, one must utilize the DOM API. The most common method involves selecting the input element using document.getElementById or document.querySelector, followed by accessing the value property of the selected element.”
Michael Chen (JavaScript Instructor, Code Academy). “Understanding event handling is crucial for obtaining input values. By adding event listeners to your input elements, you can capture user input dynamically and process it in real-time, enhancing user experience significantly.”
Sarah Patel (Frontend Engineer, Web Solutions Group). “For modern web applications, it is advisable to use frameworks like React or Vue.js, which provide built-in mechanisms for managing state and input values. This approach simplifies the process and ensures that your application remains responsive and maintainable.”
Frequently Asked Questions (FAQs)
How can I retrieve the value of an input field in JavaScript?
You can retrieve the value of an input field by using the `value` property of the input element. For example, if you have an input with an ID of “myInput”, you can get its value using `document.getElementById(‘myInput’).value`.
What is the difference between `getElementById` and `querySelector`?
`getElementById` selects an element by its unique ID, while `querySelector` can select elements using CSS selectors, allowing for more complex queries. For example, `document.querySelector(‘#myInput’)` achieves the same result as `document.getElementById(‘myInput’)`.
How do I get the value of an input field on a button click?
You can add an event listener to the button that retrieves the input value when clicked. For example:
javascript
document.getElementById(‘myButton’).addEventListener(‘click’, function() {
var inputValue = document.getElementById(‘myInput’).value;
console.log(inputValue);
});
Can I get the value of multiple input fields at once?
Yes, you can loop through a collection of input elements using `document.querySelectorAll` and retrieve their values. For example:
javascript
var inputs = document.querySelectorAll(‘input[type=”text”]’);
inputs.forEach(function(input) {
console.log(input.value);
});
How can I handle input values in a form submission?
You can use the `onsubmit` event of the form to handle input values. By preventing the default submission behavior, you can access the input values before they are sent. For example:
javascript
document.getElementById(‘myForm’).onsubmit = function(event) {
event.preventDefault();
var inputValue = document.getElementById(‘myInput’).value;
console.log(inputValue);
};
What should I do if my input field is dynamically created?
For dynamically created input fields, ensure that you attach event listeners after the elements are added to the DOM. You can use event delegation or reattach listeners after the input is created to access their values effectively.
In JavaScript, obtaining the input value from various form elements is a fundamental task that can be accomplished through several methods. The most common approach involves using the Document Object Model (DOM) to access the input element and retrieve its value. This can be done by utilizing methods such as `getElementById`, `querySelector`, or by iterating through form elements. Each method provides a straightforward way to interact with user input, which is essential for dynamic web applications.
Moreover, it is crucial to understand the context in which the input value is being retrieved. For instance, whether the input is part of a form submission or being accessed through an event listener can influence the method used. Additionally, handling input values effectively often involves validating the data to ensure it meets the required criteria before further processing. This step is vital for maintaining data integrity and enhancing user experience.
In summary, mastering how to get input values in JavaScript is a key skill for developers. It allows for the creation of interactive and responsive web applications. By utilizing the appropriate DOM methods and considering the context of data retrieval, developers can efficiently manage user input, leading to more robust and user-friendly applications.
Author Profile
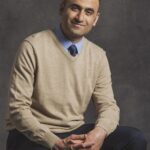
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?