How Can You Determine the Length of an Array in Python?
When diving into the world of Python programming, one of the fundamental tasks you’ll encounter is managing collections of data. Arrays, or more commonly in Python, lists, are essential structures that allow you to store and manipulate multiple items efficiently. Understanding how to interact with these data structures is crucial for any aspiring programmer. Among the many operations you can perform on arrays, determining their length is a foundational skill that lays the groundwork for more complex data manipulation and analysis.
In Python, measuring the length of an array is straightforward, yet it serves as a gateway to understanding how data is organized and accessed. The length of an array not only tells you how many elements it contains but also helps you make informed decisions about iterations, indexing, and data processing. Whether you are working on simple scripts or developing sophisticated applications, knowing how to retrieve the length of an array will enhance your coding efficiency and effectiveness.
As you explore the various methods to obtain the length of an array in Python, you’ll discover that the language offers intuitive and user-friendly approaches. From built-in functions to leveraging libraries, each method provides unique advantages depending on the context of your project. By mastering this essential skill, you’ll be better equipped to handle data structures in Python and elevate your programming prowess to new heights.
Using the len() Function
The most straightforward method to determine the length of an array in Python is by using the built-in `len()` function. This function returns the number of elements in a given object, including lists, tuples, and strings. The syntax is simple:
“`python
length = len(array)
“`
For example:
“`python
my_array = [1, 2, 3, 4, 5]
length = len(my_array)
print(length) Output: 5
“`
This method is efficient and preferred for its simplicity.
Using the numpy Library
When working with numerical data, especially large datasets, the `numpy` library is often employed. It provides a method called `.size` to get the length of an array. The advantage of using `numpy` is its performance and functionality for multi-dimensional arrays.
Here’s how to use it:
“`python
import numpy as np
my_array = np.array([1, 2, 3, 4, 5])
length = my_array.size
print(length) Output: 5
“`
Comparison of Methods
Method | Syntax | Description | Use Case |
---|---|---|---|
len() | `len(array)` | Returns the number of elements | General-purpose arrays |
numpy.size | `array.size` | Returns the total number of elements | Numerical computations |
Handling Multi-Dimensional Arrays
For multi-dimensional arrays, the `len()` function can provide the length of the first dimension (number of rows), while `numpy` allows you to get the size of each dimension.
Consider the following examples:
“`python
Using len() on a 2D list
matrix = [[1, 2, 3], [4, 5, 6]]
rows = len(matrix) Output: 2 (number of rows)
Using numpy on a 2D array
import numpy as np
matrix_np = np.array([[1, 2, 3], [4, 5, 6]])
shape = matrix_np.shape Output: (2, 3) indicating 2 rows and 3 columns
“`
In this case, `len(matrix)` gives the number of rows, while `matrix_np.shape` provides a tuple representing the dimensions of the array.
Conclusion on Array Length Retrieval
Understanding how to retrieve the length of an array is fundamental in Python programming. Whether using the built-in `len()` function or leveraging the `numpy` library for advanced numerical operations, Python provides efficient and clear methods to achieve this task. Each approach has its context and use cases, allowing developers to choose based on their specific needs.
Methods to Determine the Length of an Array in Python
In Python, arrays can be represented using lists or the `array` module. Each method for determining the length may vary slightly based on the data structure used. Below are the primary ways to retrieve the length of an array in Python.
Using Built-in Functions
The most straightforward way to get the length of an array (or a list) in Python is by using the built-in `len()` function. This function returns the number of items in a list or array.
- Syntax:
“`python
length = len(array)
“`
- Example:
“`python
my_list = [1, 2, 3, 4, 5]
length = len(my_list)
print(length) Output: 5
“`
Using the Array Module
For users who opt for the `array` module, which provides a more efficient array structure, the `len()` function works similarly.
- Importing the Module:
“`python
import array
“`
- Creating an Array:
“`python
my_array = array.array(‘i’, [1, 2, 3, 4, 5])
“`
- Getting Length:
“`python
length = len(my_array)
print(length) Output: 5
“`
Using NumPy Arrays
When working with numerical data, the NumPy library offers powerful array capabilities. To obtain the length of a NumPy array, you can use the `shape` attribute or the `len()` function.
- Using `len()`:
“`python
import numpy as np
my_numpy_array = np.array([1, 2, 3, 4, 5])
length = len(my_numpy_array)
print(length) Output: 5
“`
- Using `shape`:
“`python
length = my_numpy_array.shape[0]
print(length) Output: 5
“`
Comparison of Methods
Method | Syntax | Example Output |
---|---|---|
Built-in `len()` | `len(my_list)` | 5 |
`array` module | `len(my_array)` | 5 |
NumPy `len()` | `len(my_numpy_array)` | 5 |
NumPy `shape` | `my_numpy_array.shape[0]` | 5 |
Considerations
- The `len()` function is versatile and works across different data structures, making it the preferred choice for simplicity.
- For NumPy arrays, understanding the shape is critical, especially for multi-dimensional arrays where `shape` provides a tuple of dimensions.
- When using custom classes or data structures, ensure that they implement the `__len__` method to be compatible with the `len()` function.
Understanding Array Length in Python: Expert Insights
Dr. Emily Carter (Senior Data Scientist, Tech Innovations Inc.). “In Python, the simplest and most efficient way to determine the length of an array is by using the built-in `len()` function. This function returns the number of elements in the array, making it a fundamental tool for data manipulation and analysis.”
Michael Thompson (Python Software Engineer, CodeCraft Solutions). “When working with arrays in Python, especially when using libraries like NumPy, it is crucial to understand that the `len()` function will return the length of the outermost dimension. For multi-dimensional arrays, you may need to use `array.shape` to get a comprehensive view of all dimensions.”
Lisa Chen (Computer Science Educator, Future Coders Academy). “Teaching students how to get the length of an array in Python often highlights the importance of understanding data structures. The `len()` function is not only intuitive but also serves as a gateway to more complex operations, reinforcing the concept of data size in programming.”
Frequently Asked Questions (FAQs)
How do I get the length of an array in Python?
You can obtain the length of an array in Python by using the built-in `len()` function. For example, `len(my_array)` will return the number of elements in `my_array`.
Can I use the `len()` function on lists as well?
Yes, the `len()` function works on lists in Python, as lists are a type of array. It will return the total number of items in the list.
Is there a difference between the length of a list and an array in Python?
In Python, lists and arrays can both be measured using `len()`, but lists are more flexible and can hold different data types, while arrays (from the `array` module) are more efficient for numerical data.
What will `len()` return for an empty array?
The `len()` function will return `0` for an empty array, indicating that there are no elements present.
Can I use `len()` on multi-dimensional arrays?
Yes, `len()` can be used on multi-dimensional arrays. It will return the length of the first dimension. To get the lengths of other dimensions, you can index into the array, e.g., `len(my_array[0])` for the second dimension.
Are there any performance considerations when using `len()` on large arrays?
The `len()` function operates in constant time, O(1), regardless of the size of the array. Therefore, it is efficient and does not have significant performance implications, even for large arrays.
In Python, obtaining the length of an array is a straightforward process that can be accomplished using the built-in `len()` function. This function returns the number of elements present in the array, allowing developers to easily assess the size of their data structures. It is important to note that this method is applicable not only to arrays but also to other iterable objects such as lists, tuples, and strings, making it a versatile tool in Python programming.
One of the key takeaways is the simplicity and efficiency of the `len()` function. It provides a direct and intuitive way to retrieve the length of an array without the need for additional libraries or complex code. This feature is particularly beneficial for developers who prioritize clean and readable code, as it minimizes the overhead associated with length calculations.
Furthermore, understanding how to determine the length of an array is essential for effective data manipulation and algorithm implementation. Knowing the size of an array can influence decision-making processes in loops, conditionals, and other control structures. Thus, mastering the use of the `len()` function is a fundamental skill for any Python programmer, enhancing their ability to work with data efficiently.
Author Profile
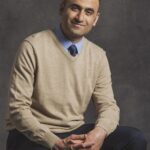
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?