How Can You Import a File in Python? A Step-by-Step Guide
Introduction
In the world of programming, the ability to import files is a fundamental skill that can significantly enhance your workflow and efficiency. Whether you’re working with data files, modules, or libraries, understanding how to import a file in Python is essential for any aspiring developer or data scientist. This process not only allows you to leverage existing code and resources but also enables you to manage your projects more effectively. As you dive into the world of Python, mastering file imports will open the door to a myriad of possibilities, from data analysis to web development.
When you import a file in Python, you’re essentially bringing in external code or data that can be utilized within your program. This can include anything from standard libraries provided by Python itself to custom modules you’ve created or third-party packages that enhance your project’s functionality. The process is straightforward, yet it holds the key to unlocking the full potential of your coding endeavors. By understanding the various methods and best practices for importing files, you’ll be well-equipped to streamline your projects and collaborate with others seamlessly.
As you explore the intricacies of file imports, you’ll discover the differences between importing entire modules versus specific functions, as well as the nuances of relative and absolute imports. These concepts form the backbone of effective code organization and modular programming in Python. So,
Using the Import Statement
To import a file in Python, the most common method is to use the `import` statement. This allows you to include modules and access functions, classes, and variables defined in those modules. A module can be a Python file with a `.py` extension. Here’s how to do it:
- Basic import: To import an entire module, use the following syntax:
python
import module_name
- Importing specific functions or classes: If you only need certain parts of a module, you can import specific items:
python
from module_name import function_name
- Importing with an alias: You can also assign an alias to a module for easier access:
python
import module_name as alias_name
Importing Custom Files
When working with custom files, ensure that the file you want to import is in the same directory as your script or in your Python path. Here’s how to import a custom Python file:
- Place your custom file (e.g., `my_module.py`) in the same directory as your main script.
- Use the import statement to access it:
python
import my_module
To access functions or variables within `my_module.py`, refer to them as follows:
python
my_module.function_name()
Using the `importlib` Module
For more advanced import scenarios, the `importlib` module provides a programmatic way to import modules. This can be particularly useful when the module name is generated at runtime. An example of using `importlib` is shown below:
python
import importlib
module_name = ‘my_module’
my_module = importlib.import_module(module_name)
This approach is beneficial for dynamic imports and can enhance the flexibility of your code.
Handling Import Errors
When importing files, it’s essential to handle potential errors gracefully. Common issues include missing modules or syntax errors in the imported file. Use try-except blocks to manage these exceptions:
python
try:
import my_module
except ImportError as e:
print(f”Error importing module: {e}”)
Table of Import Variations
Import Type | Syntax | Description |
---|---|---|
Full Module | import module_name |
Imports the entire module |
Specific Function | from module_name import function_name |
Imports a specific function from the module |
Alias Import | import module_name as alias_name |
Imports the module with an alias |
Dynamic Import | importlib.import_module(module_name) |
Imports a module dynamically at runtime |
By understanding these various methods of importing files in Python, you can effectively manage your code organization and reuse functionality across different scripts.
Importing Modules in Python
To import a file in Python, primarily a module or package, you use the `import` statement. This allows you to access functions, classes, and variables defined in other files.
- Basic Import: To import an entire module, use the following syntax:
python
import module_name
- Importing Specific Attributes: If you only need certain functions or classes from a module, you can import them directly:
python
from module_name import function_name
- Importing with an Alias: To avoid naming conflicts or for convenience, you can assign an alias to the module:
python
import module_name as alias_name
Importing Local Files
When importing a local file, ensure that it is in the same directory as your script, or adjust your Python path accordingly.
- Example of Importing a Local Module: Assuming you have a file named `my_module.py`, you can import it as follows:
python
import my_module
- Using Functions from the Local Module: After importing, you can utilize the functions defined in `my_module.py`:
python
my_module.my_function()
Using the `importlib` Module
For dynamic imports, Python provides the `importlib` module, which allows you to import modules during runtime.
- Dynamic Import Example:
python
import importlib
my_module = importlib.import_module(‘my_module’)
my_module.my_function()
Handling Import Errors
When importing files, you may encounter errors. Here are common issues and how to handle them:
Error Type | Description | Solution |
---|---|---|
ModuleNotFoundError | The specified module cannot be found. | Ensure the module name is correct and in the path. |
ImportError | The module exists but cannot be imported. | Check for syntax errors or circular imports. |
Importing Packages
Packages in Python are collections of modules organized in directories. To import a package, the structure should include an `__init__.py` file.
- Importing a Package: Assuming a package named `my_package`:
python
import my_package
- Importing a Submodule from a Package: If you need a submodule:
python
from my_package import submodule
Best Practices for Imports
- Always place import statements at the top of your Python files.
- Use absolute imports over relative imports for clarity.
- Import only what you need to maintain readability and efficiency.
By following these guidelines, you can effectively manage file imports in your Python projects, enhancing modularity and code organization.
Expert Insights on Importing Files in Python
Dr. Emily Carter (Senior Python Developer, Tech Innovations Inc.). “Importing files in Python is a fundamental skill that every programmer must master. Utilizing the built-in `import` statement allows you to access modules and libraries efficiently, which enhances code reusability and organization.”
Michael Chen (Data Scientist, Analytics Solutions Group). “When importing files, especially data files like CSVs, it is crucial to use libraries such as `pandas` for seamless data manipulation. This not only simplifies the import process but also provides robust tools for data analysis.”
Sarah Thompson (Software Engineer, CodeCraft Labs). “Understanding the difference between relative and absolute imports in Python is essential. It allows developers to structure their projects effectively, ensuring that modules are correctly located and imported without conflicts.”
Frequently Asked Questions (FAQs)
How do I import a CSV file in Python?
To import a CSV file in Python, use the `pandas` library. First, install it using `pip install pandas`. Then, use the following code:
python
import pandas as pd
data = pd.read_csv(‘filename.csv’)
What is the difference between import and from…import?
The `import` statement imports the entire module, while `from…import` allows you to import specific attributes or functions from a module. For example:
python
import math # imports the entire math module
from math import sqrt # imports only the sqrt function
Can I import a Python file from a different directory?
Yes, you can import a Python file from a different directory by modifying the `sys.path` list or by using relative imports. For example:
python
import sys
sys.path.append(‘/path/to/directory’)
import my_module
How do I handle import errors in Python?
To handle import errors, use a try-except block. This allows you to catch `ImportError` and handle it gracefully. For example:
python
try:
import my_module
except ImportError:
print(“Module not found.”)
Is it possible to import multiple modules in one line?
Yes, you can import multiple modules in one line by separating them with commas. For example:
python
import os, sys, math
How can I import a module conditionally?
You can import a module conditionally by using an if statement. For example:
python
if condition:
import my_module
Importing a file in Python is a fundamental skill that enables users to work with external data efficiently. Python offers various methods to import files, depending on the file type and the specific requirements of the task. The most common approaches include using built-in functions such as `open()` for text files, and libraries like `pandas` for structured data formats such as CSV or Excel. Understanding the appropriate method for file importation is crucial for effective data manipulation and analysis.
Additionally, error handling is an essential aspect of file importation in Python. Implementing try-except blocks can help manage exceptions that may arise during the file opening process, such as file not found errors or permission issues. This practice not only enhances the robustness of the code but also improves the user experience by providing informative feedback when issues occur.
Moreover, it is important to consider the file encoding when importing text files. Specifying the correct encoding, such as UTF-8, ensures that the data is read accurately, particularly when dealing with special characters. Awareness of these nuances can significantly affect the outcome of data processing tasks.
In summary, mastering the techniques for importing files in Python is vital for anyone working with data. By leveraging the appropriate methods,
Author Profile
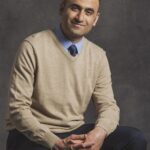
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?