How Can You Import a Function from Another File in Python?
### Introduction
In the world of Python programming, the ability to organize and modularize your code is essential for creating efficient and maintainable applications. As projects grow in complexity, the need to share and reuse code across different files becomes increasingly important. This is where the concept of importing functions from one file to another comes into play. Whether you’re building a small script or a large-scale application, mastering the art of importing functions can significantly enhance your workflow and streamline your development process.
When you import a function from another file in Python, you unlock a powerful way to structure your code. This practice not only promotes code reusability but also helps in keeping your codebase clean and organized. By dividing your code into separate modules, you can focus on specific functionalities without cluttering your main program. This modular approach not only makes your code easier to read but also simplifies debugging and testing.
Understanding the mechanics of importing functions is crucial for any aspiring Python developer. It involves knowing how to properly structure your files, utilize the import statement, and manage namespaces effectively. As you delve deeper into this topic, you’ll discover the various methods of importing, including absolute and relative imports, and how each can be applied in different scenarios. Get ready to elevate your coding skills and embrace the power of modular programming
Importing Functions Using the `import` Statement
In Python, functions can be imported from other files (modules) using the `import` statement. This allows for better organization of code and promotes reusability. The basic syntax for importing a function is as follows:
python
from module_name import function_name
This syntax imports the specified function from the given module. Alternatively, you can import all functions from a module using the wildcard `*`:
python
from module_name import *
However, this practice is generally discouraged as it may lead to confusion regarding the origin of functions.
Using the `import` Statement
To import functions from another file, follow these steps:
- Ensure Both Files Are in the Same Directory: For the import to work seamlessly, the Python files should reside in the same directory, or the directory containing the files should be added to the Python path.
- Create the Module File: Suppose you have a file named `math_functions.py` with a function defined as follows:
python
def add(a, b):
return a + b
- Importing the Function in Another File: In another file, say `main.py`, you can import the `add` function using:
python
from math_functions import add
result = add(5, 3)
print(result) # Output will be 8
Importing Multiple Functions
When you need to import multiple functions from the same module, you can do so by separating the function names with commas:
python
from math_functions import add, subtract
Alternatively, you can import the entire module and use the dot notation to access its functions:
python
import math_functions
result = math_functions.add(5, 3)
This method can enhance readability, especially when dealing with multiple functions.
Table of Import Styles
Import Style | Description | Example |
---|---|---|
Specific Import | Imports a specific function from a module. | from module_name import function_name |
Wildcard Import | Imports all functions from a module (not recommended). | from module_name import * |
Module Import | Imports the entire module, allowing access to all functions with dot notation. | import module_name |
Best Practices for Importing Functions
When importing functions, consider the following best practices:
- Use Specific Imports: Always prefer importing specific functions to avoid namespace pollution and improve code clarity.
- Organize Modules Logically: Group related functions together in modules to make them easier to find and use.
- Maintain Consistent Naming Conventions: This helps in understanding what each function does and where it is defined.
By adhering to these practices, developers can maintain clean, efficient, and manageable codebases.
Importing Functions in Python
In Python, importing functions from another file (or module) allows for better organization and reuse of code. The process involves using the `import` statement, which makes the functions defined in one file accessible in another.
Basic Import Syntax
To import a function from another file, follow these steps:
- Ensure that both files are in the same directory or that the directory containing the file to be imported is in your Python path.
- Use the following syntax to import a specific function:
python
from filename import function_name
For example, if you have a file named `utilities.py` that contains a function called `calculate_area`, you would import it as follows:
python
from utilities import calculate_area
Importing All Functions
If you want to import all functions from a module, you can use the asterisk `*` operator:
python
from filename import *
This method is generally discouraged as it can lead to namespace pollution, making it unclear which functions are available.
Module Structure
The structure of a module can impact the import process. Here’s a simple example of how to structure your files:
- project_directory/
- main.py
- utilities.py
In `utilities.py`:
python
def calculate_area(radius):
return 3.14 * radius * radius
In `main.py`, you would import the function as follows:
python
from utilities import calculate_area
print(calculate_area(5))
Using Aliases
You can also use aliases to avoid name conflicts or to simplify long names. This is done using the `as` keyword:
python
from filename import function_name as alias_name
For instance:
python
from utilities import calculate_area as area
Now you can use `area()` to call the function.
Relative Imports
When working with larger projects, you may want to use relative imports. This is particularly useful in packages. The syntax is as follows:
python
from .module import function_name
For example, if you have a package structure like this:
- my_package/
- __init__.py
- module_a.py
- module_b.py
In `module_b.py`, you can import from `module_a.py` using:
python
from .module_a import some_function
Common Import Errors
While importing functions, you may encounter several common errors:
Error Type | Description |
---|---|
ImportError | Indicates that the module or function cannot be found. |
ModuleNotFoundError | The specified module does not exist in the path. |
NameError | The function being imported does not exist in the module. |
To resolve these issues, ensure that the module names are spelled correctly, and verify that the files are located in the correct directories.
Expert Insights on Importing Functions in Python
Dr. Emily Carter (Senior Python Developer, Tech Innovations Inc.). “To import a function from another file in Python, one must ensure that both files are in the same directory or that the directory containing the file is included in the Python path. The syntax ‘from filename import function_name’ is straightforward and allows for seamless integration of code across files.”
Michael Zhang (Lead Software Engineer, CodeCraft Solutions). “Utilizing the ‘import’ statement effectively is crucial for maintaining clean and modular code. It is important to remember that when importing, the filename should not include the .py extension. This practice not only enhances readability but also promotes reusability of functions across different projects.”
Sarah Thompson (Python Educator, LearnPython Academy). “When teaching how to import functions, I emphasize the importance of understanding the scope and namespace. Using ‘import filename’ allows access to all functions within that file, but using ‘from filename import function_name’ provides a more concise way to access specific functions, which can lead to cleaner and more efficient code.”
Frequently Asked Questions (FAQs)
How do I import a specific function from another file in Python?
To import a specific function, use the syntax `from filename import function_name`. Ensure that the file is in the same directory or in your Python path.
Can I import multiple functions from the same file?
Yes, you can import multiple functions by separating them with commas, like this: `from filename import function1, function2`.
What if the function I want to import is in a subdirectory?
You can import functions from a subdirectory by using the dot notation. For example, `from subdirectory.filename import function_name`.
How do I import all functions from a file?
To import all functions, use the asterisk syntax: `from filename import *`. However, this practice is generally discouraged as it can lead to namespace conflicts.
What is the difference between import and from import in Python?
Using `import filename` imports the entire module, requiring you to prefix the function with the module name (e.g., `filename.function_name`). In contrast, `from filename import function_name` allows direct access to the function without the module prefix.
Can I rename a function when importing it?
Yes, you can rename a function during import by using the `as` keyword, like this: `from filename import function_name as new_name`. This allows you to use `new_name` in your code.
Importing a function from another file in Python is a fundamental skill that enhances code organization and reusability. This process typically involves using the `import` statement, which allows you to access functions, classes, and variables defined in one module from another. By structuring your code into separate files, you can maintain clarity and manage complexity, especially in larger projects.
To import a function, you can use the syntax `from module_name import function_name`, where `module_name` is the name of the file (without the .py extension) containing the function. Alternatively, you can import the entire module using `import module_name` and then call the function with the syntax `module_name.function_name()`. This flexibility allows developers to choose the most appropriate method based on their specific needs and coding style.
Key takeaways include the importance of naming conventions and the organization of your Python files. It is crucial to ensure that the module is in the same directory or in the Python path to avoid import errors. Additionally, understanding the difference between absolute and relative imports can further enhance your ability to manage dependencies within your projects effectively.
Author Profile
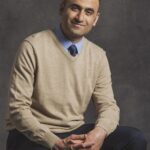
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?