How Can You Import a Python File into Another Python File?
In the world of Python programming, the ability to import files and modules is a cornerstone of efficient and organized coding. Whether you’re building a small script or a complex application, understanding how to import a Python file into another can significantly enhance your workflow and modularize your code. This practice not only promotes reusability but also helps in maintaining clean and manageable codebases. If you’ve ever found yourself tangled in a web of functions and classes, fear not—this guide will illuminate the path to seamless file integration.
When you import a Python file into another, you’re essentially telling the interpreter to include the functionality defined in that file, allowing you to leverage pre-written code without having to rewrite it. This process can streamline your development, enabling you to focus on building new features rather than reinventing the wheel. Additionally, it fosters collaboration and code sharing, making it easier to work on projects with multiple contributors.
Throughout this article, we will explore the various methods of importing Python files, the nuances of module organization, and best practices to ensure your code remains clean and efficient. Whether you’re a beginner looking to grasp the basics or an experienced developer seeking to refine your skills, understanding how to import Python files is an essential step in your programming journey. Get ready to unlock the full potential
Using the import Statement
To import a Python file into another, you can use the `import` statement. This allows you to access functions, classes, and variables defined in the imported file. The basic syntax is as follows:
“`python
import module_name
“`
This statement imports the entire module, making its contents accessible with the `module_name.` prefix. For example, if you have a file named `my_module.py` with a function called `my_function`, you would use:
“`python
import my_module
my_module.my_function()
“`
Importing Specific Functions or Classes
If you need only specific functions or classes from a module, you can use the `from` keyword. This method allows for a cleaner namespace and avoids the need to prefix the module name. The syntax is:
“`python
from module_name import function_name
“`
For instance:
“`python
from my_module import my_function
my_function()
“`
Using Aliases
You can also assign an alias to a module or a function using the `as` keyword. This is particularly useful for modules with long names or when you want to avoid name conflicts. The syntax is:
“`python
import module_name as alias_name
“`
Example:
“`python
import my_module as mm
mm.my_function()
“`
Directory Structure and Module Discovery
When importing a module, Python searches for it in the directories listed in `sys.path`. This list includes the directory of the script being run, the PYTHONPATH environment variable, and the installation-dependent default paths.
To ensure successful imports, consider the following directory structure:
“`
project/
│
├── main.py
└── my_module.py
“`
In `main.py`, you can import `my_module` directly as shown earlier.
Using __init__.py for Packages
If you wish to create a package, ensure that the directory contains an `__init__.py` file. This file can be empty or can execute the package initialization code. The presence of `__init__.py` allows Python to recognize the directory as a package, enabling you to structure imports more hierarchically.
Example structure:
“`
project/
│
├── main.py
└── my_package/
├── __init__.py
└── my_module.py
“`
To import `my_module` from `my_package`, you would use:
“`python
from my_package import my_module
“`
Table of Import Methods
Method | Description |
---|---|
import module_name | Imports the entire module. |
from module_name import function_name | Imports a specific function or class. |
import module_name as alias_name | Imports the module with an alias. |
from module_name import function_name as alias_name | Imports a specific function with an alias. |
Common Errors and Troubleshooting
While importing files, you might encounter several common errors:
- ModuleNotFoundError: This indicates that the specified module cannot be found. Ensure that the module is in the correct directory or that `PYTHONPATH` is set appropriately.
- ImportError: This occurs when the module is found, but the specified function or class does not exist. Check for typos in the names or verify the contents of the module.
Utilizing the import functionality effectively allows for modular programming in Python, enhancing code organization and reusability.
Importing a Python File
To import a Python file into another, you primarily utilize the `import` statement. This allows you to access functions, classes, and variables defined in one file from another. The process is straightforward but requires attention to detail regarding file organization and naming conventions.
Basic Import Syntax
The simplest way to import a file is by using the following syntax:
“`python
import module_name
“`
Here, `module_name` refers to the name of the Python file you wish to import, excluding the `.py` extension. For instance, if your file is named `example.py`, you would use:
“`python
import example
“`
Importing Specific Items
If you want to import specific functions or classes from a module, you can use the `from` keyword:
“`python
from module_name import specific_function
“`
For example:
“`python
from example import my_function
“`
This method allows you to use `my_function` directly without needing to prefix it with the module name.
Using Aliases
You can also use an alias for a module or specific imports to simplify usage or avoid naming conflicts. This can be done using the `as` keyword:
“`python
import module_name as alias_name
“`
Example:
“`python
import example as ex
“`
You can now call functions from `example` using the alias:
“`python
ex.my_function()
“`
Organizing Your Python Files
When importing Python files, it is important to keep your files organized in a way that Python can easily locate them. Consider the following structure:
“`
project/
├── main.py
├── utils.py
└── submodule/
├── __init__.py
└── helper.py
“`
In this structure:
- `main.py` can import `utils.py` using `import utils`.
- To import `helper.py`, you would need to use `from submodule import helper`.
Handling Import Errors
When dealing with imports, you might encounter errors. Common issues include:
- ModuleNotFoundError: This occurs when Python cannot find the specified module. Ensure the module name is correct and that the file is in the correct directory.
- ImportError: This happens when an import statement fails. Check if the function or class you’re trying to import exists in the specified module.
Using the `__init__.py` File
If you are importing from a directory, ensure that it contains an `__init__.py` file. This file can be empty but signifies to Python that the directory should be treated as a package. The presence of this file allows for more organized imports.
File Structure | Purpose |
---|---|
`__init__.py` | Marks a directory as a package |
`module.py` | Contains reusable code (functions/classes) |
Relative Imports
In a package, you can also use relative imports. This is especially useful for larger projects. You can import modules relative to the current module’s location using:
“`python
from . import sibling_module
from .. import parent_module
“`
These statements help maintain a clear hierarchy and avoid hardcoding paths.
Best Practices for Importing
- Always use absolute imports when possible for clarity.
- Group imports at the top of your file, organized into standard libraries, third-party libraries, and local application imports.
- Avoid wildcard imports (e.g., `from module import *`) as they can lead to unclear code and potential name conflicts.
By following these guidelines and structures, you can effectively manage and import Python files within your projects, enhancing modularity and code reuse.
Expert Insights on Importing Python Files
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “To import a Python file into another, you simply use the import statement followed by the filename without the ‘.py’ extension. This allows you to access functions, classes, and variables defined in that file, promoting modular programming and code reusability.”
Michael Chen (Lead Python Developer, CodeCraft Solutions). “When importing a Python file, ensure that the file is in the same directory or in the Python path. Using relative imports can also help when dealing with packages, but it is essential to understand the structure of your project to avoid import errors.”
Sarah Thompson (Python Programming Instructor, Code Academy). “It’s crucial to be mindful of naming conflicts when importing files. If two files have the same name, Python will import the first one it finds in the directory. Utilizing unique module names and organizing your files into packages can significantly mitigate this issue.”
Frequently Asked Questions (FAQs)
How do I import a Python file into another Python file?
You can import a Python file by using the `import` statement followed by the name of the file without the `.py` extension. For example, if you have a file named `module.py`, you can import it using `import module`.
What is the difference between `import` and `from … import`?
The `import` statement imports the entire module, while `from … import` allows you to import specific functions or classes from a module. For instance, `from module import function_name` imports only `function_name` from `module.py`.
Can I import a Python file from a different directory?
Yes, you can import a Python file from a different directory by modifying the `sys.path` list to include the directory path or by using relative imports if the files are part of a package.
What should I do if I encounter an ImportError?
An ImportError typically occurs when Python cannot find the module you are trying to import. Ensure that the module name is correct, the file is in the correct directory, and the directory is included in your Python path.
Is it possible to import a Python file conditionally?
Yes, you can conditionally import a Python file using an `if` statement. This allows you to control the import based on certain conditions in your code.
How can I import a module and give it an alias?
You can import a module and assign it an alias using the `as` keyword. For example, `import module as mod` allows you to refer to `module` as `mod` throughout your code.
In summary, importing a Python file into another is a fundamental aspect of Python programming that enhances modularity and code reuse. The process typically involves using the `import` statement, which allows developers to access functions, classes, and variables defined in one file from another. This can be achieved through various methods, including importing entire modules, specific functions or classes, or using aliases for convenience. Understanding the structure of Python packages and modules is essential for effective imports.
Additionally, Python’s import system supports relative and absolute imports, which provide flexibility in organizing code within packages. Absolute imports specify the full path to the module, while relative imports allow for referencing modules based on their location within the package hierarchy. Properly managing imports can help prevent naming conflicts and improve code clarity.
Key takeaways include the importance of maintaining a clear directory structure for your Python files, as this directly impacts the import process. Developers should also be aware of the potential for circular imports, which can lead to complications in code execution. By following best practices for imports, such as keeping modules focused and minimizing dependencies, programmers can create more maintainable and efficient codebases.
Author Profile
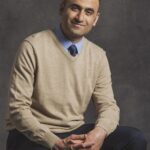
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?