How Can You Import a Class from Another Python File?
When diving into the world of Python programming, one of the essential skills you’ll encounter is the ability to organize your code effectively. As your projects grow in complexity, managing multiple files becomes crucial to maintaining clarity and efficiency. One common task you’ll face is importing classes from one Python file to another, a practice that not only enhances code reusability but also promotes a modular approach to development. Whether you’re building a simple script or a large application, understanding how to seamlessly integrate different components will elevate your coding prowess and streamline your workflow.
Importing classes in Python is a fundamental concept that allows you to harness the power of object-oriented programming. By structuring your code across multiple files, you can create a more manageable and organized codebase. This practice not only helps in avoiding redundancy but also makes it easier to collaborate with others, as different functionalities can be developed in isolation and then brought together. As you explore this topic, you’ll discover various techniques and best practices that will empower you to effectively share and reuse your code.
In this article, we will guide you through the nuances of importing classes from other Python files. You’ll learn about the different methods available, how to handle potential pitfalls, and the importance of maintaining a clear project structure. Whether you’re a beginner eager to learn or an experienced developer
Importing Classes from Another Python File
To import a class from another Python file, you typically use the `import` statement. This allows you to access the class and utilize its methods and properties within your current script. Here’s a step-by-step guide on how to achieve this effectively.
Basic Import Syntax
Assuming you have two files: `file_one.py` containing the class definition, and `file_two.py` where you want to use that class, you can import the class as follows:
- Define the class in `file_one.py`:
“`python
file_one.py
class MyClass:
def greet(self):
return “Hello from MyClass!”
“`
- Import the class in `file_two.py`:
“`python
file_two.py
from file_one import MyClass
my_instance = MyClass()
print(my_instance.greet())
“`
Relative vs Absolute Imports
Python supports both relative and absolute imports. Understanding the difference is crucial, especially when working on larger projects.
- Absolute Import: This method specifies the full path to the module, starting from the project’s root directory. For instance:
“`python
from package.module import MyClass
“`
- Relative Import: This approach uses the dot (`.`) notation to refer to the current and parent directories. For example:
“`python
from .module import MyClass Current directory
from ..module import MyClass Parent directory
“`
Organizing Your Project Structure
A well-organized project structure can help manage imports more effectively. Here is a sample directory structure:
“`
my_project/
│
├── main.py
├── module_a/
│ └── __init__.py
│ └── class_a.py
└── module_b/
└── __init__.py
└── class_b.py
“`
In this structure, if you want to import `class_a` into `class_b`, you would do the following:
“`python
class_b.py
from module_a.class_a import MyClass
“`
Importing Multiple Classes
You can also import multiple classes from a single file. This can be done by separating the class names with commas:
“`python
from file_one import MyClass, AnotherClass
“`
Alternatively, if you need to import all classes from a module, you can use the wildcard `*`. However, this is generally not recommended due to the potential for name conflicts.
“`python
from file_one import *
“`
Common Pitfalls
When working with imports, you may encounter several common issues:
- Module Not Found Error: This usually occurs when Python cannot locate the file you are trying to import. Ensure the file is in the same directory or correctly specify the path.
- Circular Imports: This happens when two modules try to import each other. To avoid this, restructure your code to minimize interdependencies.
- File Naming Conflicts: Ensure that your file names do not conflict with standard library module names.
Issue | Solution |
---|---|
Module Not Found Error | Check file path and naming. |
Circular Imports | Refactor code to avoid mutual dependencies. |
File Naming Conflicts | Rename your files to avoid clashes with built-in modules. |
By following these guidelines, you can effectively manage class imports across different Python files, leading to more organized and maintainable code.
Importing Classes from Another Python File
To import a class from another Python file, you need to ensure that both files are in the same directory or that the directory containing the file you want to import is included in your Python path. Here are the steps to follow:
File Structure
Consider a simple file structure as follows:
“`
/project
├── main.py
└── my_class.py
“`
In this example, `my_class.py` contains the class you want to import into `main.py`.
Defining a Class
In `my_class.py`, define your class as follows:
“`python
my_class.py
class MyClass:
def __init__(self):
self.message = “Hello from MyClass!”
def greet(self):
return self.message
“`
Importing the Class
In `main.py`, you can import `MyClass` using the following syntax:
“`python
main.py
from my_class import MyClass
Create an instance of MyClass
my_instance = MyClass()
print(my_instance.greet())
“`
Import Syntax Options
You can use various import statements depending on your needs:
- Direct Import:
“`python
from my_class import MyClass
“`
- Importing Multiple Classes:
“`python
from my_class import MyClass, AnotherClass
“`
- Importing All Classes:
“`python
from my_class import *
“`
- Import with Alias:
“`python
import my_class as mc
my_instance = mc.MyClass()
“`
Handling Different Directories
If your files are structured in different directories, you can adjust the import statement accordingly. For example, with the following structure:
“`
/project
├── main.py
└── classes/
└── my_class.py
“`
You would modify your import statement in `main.py`:
“`python
main.py
from classes.my_class import MyClass
“`
Common Errors
When importing classes, you may encounter errors. Here are some common issues:
Error Type | Description |
---|---|
ModuleNotFoundError | The file name does not match the import statement. |
ImportError | The class name is misspelled or not defined in the file. |
Circular Import | Two files importing each other can lead to this error. |
To resolve these, ensure that file names and class names are spelled correctly, avoid circular dependencies, and check that your file structure is correctly set up.
Best Practices
- Use descriptive class names to improve code readability.
- Organize related classes into modules or packages.
- Avoid using wildcard imports (`from module import *`) as it can lead to confusion and namespace pollution.
By following these guidelines and examples, you can effectively manage class imports across your Python files.
Expert Insights on Importing Classes in Python
Dr. Emily Carter (Senior Python Developer, Tech Innovations Inc.). “When importing a class from another Python file, ensure that both files are in the same directory or that the directory containing the file is included in your Python path. Use the syntax ‘from filename import ClassName’ to directly import the specific class you need.”
James Liu (Software Engineer, CodeCraft Solutions). “It is essential to understand the importance of the __init__.py file in package directories. This file allows Python to recognize the directory as a package, enabling seamless imports of classes from different modules within that package.”
Maria Gonzalez (Python Educator, LearnPythonToday). “Utilizing relative imports can simplify your code structure, especially in larger projects. By using the syntax ‘from .filename import ClassName’, you can maintain clarity and organization within your package hierarchy.”
Frequently Asked Questions (FAQs)
How do I import a class from another Python file?
To import a class from another Python file, use the syntax `from filename import ClassName`. Ensure the file is in the same directory or adjust the Python path accordingly.
What should I do if the Python files are in different directories?
If the files are in different directories, you can modify the `PYTHONPATH` or use relative imports. For example, use `from ..directory.filename import ClassName` in a package structure.
Can I import multiple classes from the same file?
Yes, you can import multiple classes by separating them with commas, like this: `from filename import Class1, Class2`.
What happens if I import a class that doesn’t exist?
If you attempt to import a class that doesn’t exist, Python will raise an `ImportError`, indicating that the specified class cannot be found.
Is it possible to import a class with an alias?
Yes, you can import a class with an alias using the `as` keyword. For example, `from filename import ClassName as AliasName` allows you to reference the class as `AliasName`.
How can I check if the import was successful?
To check if the import was successful, you can use the `try` and `except` blocks. If the import fails, it will raise an `ImportError`, which you can catch and handle accordingly.
In Python, importing a class from another file is a fundamental skill that enhances code organization and modularity. To achieve this, developers typically use the `import` statement, specifying the module name from which the class is to be imported. The syntax can vary slightly depending on whether the entire module is imported or just specific classes or functions. For example, using `from module_name import ClassName` allows for direct access to the class without needing to prefix it with the module name.
Additionally, understanding the structure of Python packages is crucial when dealing with multiple files. When working within a package, it is important to ensure that the `__init__.py` file is present, as it signifies to Python that the directory should be treated as a package. This organization not only aids in importing classes but also improves code readability and maintainability. Developers should also be aware of relative imports, which can be useful when importing classes from sibling modules within the same package.
In summary, mastering the importation of classes from other Python files is essential for effective programming. It promotes code reuse and helps maintain a clean codebase. By utilizing the appropriate import statements and understanding package structures, developers can streamline their coding practices and enhance collaboration in larger projects.
Author Profile
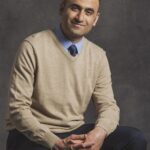
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?