How Can You Import an Excel File into Python Effortlessly?
In today’s data-driven world, the ability to manipulate and analyze data is more critical than ever. Excel files, with their widespread use in business, finance, and research, often serve as the backbone for data storage and reporting. However, as we delve deeper into data analysis using Python, the question arises: how do we seamlessly import these Excel files into our Python environment? Whether you’re a seasoned data scientist or a budding programmer, understanding the process of importing Excel files can unlock a treasure trove of insights waiting to be uncovered.
Importing Excel files into Python is not just a technical task; it’s a gateway to transforming raw data into actionable intelligence. With a variety of libraries available, such as Pandas and OpenPyXL, Python provides robust tools to handle Excel files efficiently. These libraries offer a range of functionalities, from reading and writing data to performing complex data manipulations, making it easier for users to integrate Excel data into their workflows.
As we explore the methods and best practices for importing Excel files, you’ll discover how to streamline your data analysis processes and enhance your productivity. Whether you’re looking to analyze sales data, manage inventory, or conduct research, mastering the art of importing Excel files in Python will equip you with the skills necessary to tackle any data challenge
Using Pandas to Import Excel Files
Pandas is a powerful data manipulation library in Python that simplifies the process of reading and writing data in various formats, including Excel files. To utilize Pandas for importing Excel files, you first need to ensure that the library is installed. If it is not installed, you can add it using pip:
“`bash
pip install pandas openpyxl
“`
The `openpyxl` library is required for reading `.xlsx` files. Once you have Pandas installed, you can easily read Excel files with the following command:
“`python
import pandas as pd
Load an Excel file
df = pd.read_excel(‘path_to_your_file.xlsx’, sheet_name=’Sheet1′)
“`
In this example, `df` becomes a DataFrame containing the data from the specified sheet in the Excel file. You can specify the sheet name or the sheet index (starting from 0).
Parameters for Importing Excel Files
When using `pd.read_excel()`, several parameters can be customized to cater to specific needs. Here are some commonly used parameters:
- sheet_name: Specifies the name of the sheet or the index of the sheet you want to read.
- header: Indicates which row to use as the column names. Default is 0 (first row).
- index_col: Specifies which column to use as the row labels of the DataFrame.
- usecols: Allows you to specify which columns to read from the Excel file.
- dtype: Allows you to specify the data type for data or columns.
Parameter | Description |
---|---|
sheet_name | Name or index of the sheet to read. |
header | Row to use for the column labels. |
index_col | Column to use as the index. |
usecols | Columns to read from the file. |
dtype | Data type for the columns. |
Handling Multiple Sheets
If you need to import multiple sheets from an Excel file, you can pass a list of sheet names or indices to the `sheet_name` parameter. For example:
“`python
Load multiple sheets into a dictionary of DataFrames
dfs = pd.read_excel(‘path_to_your_file.xlsx’, sheet_name=[‘Sheet1’, ‘Sheet2’])
“`
In this case, `dfs` will be a dictionary where keys are sheet names and values are the corresponding DataFrames. This allows for easy access and manipulation of data across different sheets.
Reading Specific Columns
Sometimes, you may only want to read specific columns from an Excel file. You can achieve this using the `usecols` parameter. For instance:
“`python
Read only specific columns
df = pd.read_excel(‘path_to_your_file.xlsx’, usecols=[‘A’, ‘C’, ‘D’])
“`
This command will import only the columns A, C, and D from the specified Excel file, which can help reduce memory usage and improve performance when working with large datasets.
By utilizing Pandas, importing data from Excel files becomes a straightforward task, allowing for efficient data analysis and manipulation.
Using Pandas to Import Excel Files
Pandas is a powerful data manipulation library in Python that simplifies the process of importing Excel files. To utilize Pandas for importing an Excel file, you first need to install the library if it’s not already available in your Python environment.
- Install Pandas using pip:
“`bash
pip install pandas
“`
- Additionally, for Excel file support, install `openpyxl` or `xlrd` for `.xlsx` and `.xls` files respectively:
“`bash
pip install openpyxl
pip install xlrd
“`
Once Pandas is installed, you can import an Excel file with the following code snippet:
“`python
import pandas as pd
Load an Excel file
data = pd.read_excel(‘path_to_file.xlsx’, sheet_name=’Sheet1′)
“`
Parameters for `pd.read_excel()`
- `io`: The path to the Excel file (string).
- `sheet_name`: The name or index of the sheet to read (optional, default is 0).
- `header`: Row(s) to use as the column headers (default is 0).
- `index_col`: Column(s) to set as the index (optional).
- `usecols`: Specify which columns to read (optional).
- `dtype`: Data type for data or columns (optional).
Reading Multiple Sheets
To read multiple sheets from an Excel file, you can provide a list of sheet names or use `None` to read all sheets:
“`python
Load all sheets into a dictionary of DataFrames
all_sheets = pd.read_excel(‘path_to_file.xlsx’, sheet_name=None)
“`
The resulting `all_sheets` dictionary will have sheet names as keys and DataFrames as values. You can access a specific sheet like this:
“`python
sheet1_data = all_sheets[‘Sheet1’]
“`
Handling Excel File Types
Pandas supports multiple Excel file formats, primarily `.xlsx` and `.xls`. The choice of engine may vary based on the file type:
File Format | Engine Used |
---|---|
`.xlsx` | `openpyxl` |
`.xls` | `xlrd` |
For files that use different encoding or formats, ensure the appropriate engine is specified if necessary:
“`python
data = pd.read_excel(‘path_to_file.xls’, engine=’xlrd’)
“`
Exporting Data to Excel
In addition to importing, Pandas allows you to export DataFrames back to Excel files. Use the `to_excel()` method:
“`python
data.to_excel(‘output_file.xlsx’, sheet_name=’Sheet1′, index=)
“`
Key Parameters for `to_excel()`
- `excel_writer`: The file path or ExcelWriter object (string).
- `sheet_name`: Name of the sheet to write to (default is ‘Sheet1’).
- `index`: Whether to write row names (default is True).
This functionality provides a seamless way to manipulate and analyze data before saving it back into an Excel format.
Common Issues and Troubleshooting
When importing Excel files, you might encounter several common issues:
- File Not Found Error: Ensure that the file path is correct.
- Unsupported File Format Error: Verify that the file is indeed an Excel format and not corrupted.
- Data Type Inference Issues: Specify the `dtype` parameter to avoid unexpected data types.
Utilizing these methods and parameters will enhance your ability to work with Excel files efficiently in Python, leveraging the capabilities of the Pandas library effectively.
Expert Insights on Importing Excel Files in Python
Dr. Emily Carter (Data Scientist, Analytics Innovations). “When importing Excel files in Python, utilizing libraries such as `pandas` is essential. The `read_excel` function allows for seamless data manipulation and analysis, making it a powerful tool for data scientists.”
Michael Thompson (Software Engineer, Tech Solutions Inc.). “For developers working with Excel files, I recommend using `openpyxl` for reading and writing Excel 2010 xlsx/xlsm/xltx/xltm files. It provides a more granular control over the Excel file structure compared to other libraries.”
Linda Zhang (Python Educator, Code Academy). “Understanding the different libraries available for importing Excel files in Python is crucial. While `pandas` is great for data analysis, `xlrd` is useful for reading older Excel files. Choosing the right library based on your specific needs can significantly enhance your workflow.”
Frequently Asked Questions (FAQs)
How can I import an Excel file in Python?
You can import an Excel file in Python using the `pandas` library. First, install the library using `pip install pandas openpyxl`. Then, use the `pd.read_excel(‘filename.xlsx’)` function to read the Excel file into a DataFrame.
What libraries are commonly used to import Excel files in Python?
The most commonly used libraries for importing Excel files in Python are `pandas` and `openpyxl`. `pandas` provides a high-level interface for data manipulation, while `openpyxl` is used for reading and writing Excel files directly.
Can I read multiple sheets from an Excel file using Python?
Yes, you can read multiple sheets from an Excel file using the `pandas` library. Use the `pd.read_excel(‘filename.xlsx’, sheet_name=None)` function to read all sheets into a dictionary of DataFrames, where keys are sheet names.
What formats of Excel files can be imported in Python?
Python can import both `.xls` and `.xlsx` formats of Excel files. The `pandas` library supports these formats natively, with `openpyxl` handling `.xlsx` files and `xlrd` handling `.xls` files.
Is it possible to import Excel files without using pandas?
Yes, it is possible to import Excel files without using `pandas`. Libraries such as `openpyxl` and `xlrd` can be used to read data directly from Excel files, but they may require more manual handling of data compared to `pandas`.
What are some common errors when importing Excel files in Python?
Common errors include `FileNotFoundError`, which occurs if the file path is incorrect, and `ValueError`, which may arise if the specified sheet name does not exist. Additionally, issues with file formats can lead to import errors.
Importing Excel files in Python is a fundamental task that can be accomplished using various libraries, with the most popular being Pandas and OpenPyXL. Pandas provides a powerful and flexible way to read and manipulate Excel files, allowing users to easily convert Excel data into DataFrames, which are essential for data analysis. The `read_excel()` function in Pandas simplifies the process, enabling users to specify parameters such as the sheet name and data types, thus facilitating a tailored data import experience.
In addition to Pandas, OpenPyXL is another robust library that allows for reading and writing Excel files in the .xlsx format. This library is particularly useful for scenarios that require more control over the Excel file structure, such as modifying cell styles or managing formulas. Users can choose between these libraries based on their specific needs, whether they prioritize ease of use or require advanced features.
Furthermore, it is important to consider the installation of these libraries, as they may not be included in the standard Python distribution. Users can easily install them using package managers like pip. Additionally, handling potential issues such as missing data or incorrect formats during the import process is crucial for ensuring data integrity and accuracy in analysis.
In summary, importing Excel
Author Profile
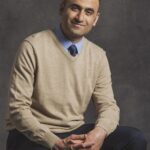
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?