How Can You Import Files in Python Effectively?
In the world of programming, the ability to seamlessly integrate and manipulate data is paramount. Python, with its rich ecosystem of libraries and modules, offers a powerful yet straightforward approach to importing files. Whether you’re working with CSVs, JSON, or even plain text files, knowing how to effectively import these resources can significantly enhance your coding efficiency and data handling capabilities. This article will guide you through the essential techniques and best practices for importing files in Python, empowering you to unlock the full potential of your projects.
When working with Python, importing files is a fundamental skill that every programmer should master. This process allows you to access and utilize external data, enabling you to perform analyses, generate reports, or feed information into your applications. With a variety of file formats available, Python provides built-in functions and libraries that simplify the import process, making it accessible even for beginners. Understanding these methods not only streamlines your workflow but also enhances your ability to collaborate with others who may be sharing data in different formats.
As you delve deeper into the world of file imports, you’ll discover the nuances of handling various types of data, including structured and unstructured formats. From reading data into pandas DataFrames for complex data analysis to parsing JSON for web applications, the techniques you learn will be
Using the import Statement
The primary way to import files in Python is through the `import` statement. This allows you to access the functions, classes, and variables defined in another Python module. The basic syntax is as follows:
“`python
import module_name
“`
Once imported, you can utilize the module’s content by prefixing it with the module name. For instance:
“`python
import math
result = math.sqrt(16)
“`
To import specific items from a module, you can use the `from` keyword, which allows you to access the items directly without the module prefix. The syntax is:
“`python
from module_name import item_name
“`
Example:
“`python
from math import pi
print(pi)
“`
Importing Multiple Items
To import multiple items from a module, you can list them within parentheses, separated by commas. The structure is as follows:
“`python
from module_name import item1, item2, item3
“`
For example:
“`python
from math import sin, cos, tan
“`
Alternatively, you can use an asterisk `*` to import all items from a module, though this is generally discouraged due to potential namespace conflicts:
“`python
from module_name import *
“`
Creating and Importing Custom Modules
To create a custom module, simply write your functions and classes in a `.py` file. For example, if you create a file named `mymodule.py` with the following content:
“`python
def greet(name):
return f”Hello, {name}!”
“`
You can import and use it in another file as follows:
“`python
import mymodule
print(mymodule.greet(“Alice”))
“`
Using Packages
A package in Python is a directory that contains multiple modules. To create a package, follow these steps:
- Create a directory with an `__init__.py` file.
- Add your module files within this directory.
Example directory structure:
“`
mypackage/
__init__.py
module1.py
module2.py
“`
You can import modules from the package like this:
“`python
from mypackage import module1
“`
Importing from Subdirectories
To import modules from subdirectories, you need to ensure that the subdirectory is treated as a package. This requires an `__init__.py` file in the subdirectory. You can then import as follows:
“`python
from mypackage.subdirectory import module
“`
Common Import Errors
When importing modules or packages, you may encounter various errors. Here are some common issues and their solutions:
Error Type | Description | Solution |
---|---|---|
ModuleNotFoundError | The specified module cannot be found. | Check the module name and path. |
ImportError | The module was found, but there was an issue loading it. | Verify that the module is valid. |
Circular Import Error | Two modules are importing each other. | Refactor the code to avoid circular dependencies. |
Understanding these concepts and applying the correct import methods will enhance your efficiency in managing and utilizing Python files and modules effectively.
Importing Modules in Python
In Python, importing modules allows you to access functions, classes, and variables defined in other files. The most common way to import a module is by using the `import` statement.
- Basic Import: To import an entire module, use:
“`python
import module_name
“`
- Importing Specific Functions or Classes: To import specific items from a module, use:
“`python
from module_name import function_name
“`
- Renaming Imports: You can rename an imported module or function using the `as` keyword:
“`python
import module_name as alias_name
“`
- Importing Multiple Items: You can import multiple functions or classes using commas:
“`python
from module_name import function_one, function_two
“`
Importing Files from the Same Directory
When you have a Python file that you wish to import, it is essential to ensure that both files are in the same directory or package. For example, if you have a file named `my_module.py`, you can import it as follows:
“`python
import my_module
“`
For importing specific functions or variables:
“`python
from my_module import my_function
“`
Importing Files from Different Directories
To import a module from a different directory, you can use the `sys` module to add the directory to the Python path:
“`python
import sys
sys.path.append(‘/path/to/your/directory’)
import my_module
“`
Alternatively, if the directory structure is set up as a package, you can use relative imports. For example, if you have the following structure:
“`
/project
/package
__init__.py
my_module.py
main.py
“`
You can use:
“`python
from package import my_module
“`
Importing JSON and CSV Files
Python provides built-in libraries for handling different file formats, such as JSON and CSV.
- Importing JSON: To read a JSON file, use the `json` module:
“`python
import json
with open(‘data.json’) as json_file:
data = json.load(json_file)
“`
- Importing CSV: The `csv` module is used for reading and writing CSV files:
“`python
import csv
with open(‘data.csv’, newline=”) as csvfile:
reader = csv.reader(csvfile)
for row in reader:
print(row)
“`
Using Packages
Packages are directories containing an `__init__.py` file and can be imported in a similar manner. If you have a package structure like this:
“`
/project
/mypackage
__init__.py
module_a.py
module_b.py
“`
You can import from these modules as follows:
“`python
from mypackage import module_a
from mypackage.module_b import my_function
“`
Handling Import Errors
When importing modules, you may encounter several types of errors. Common ones include:
- ModuleNotFoundError: Raised if the module cannot be found. Ensure the module is correctly named and located in the appropriate directory.
- ImportError: Raised if you attempt to import a specific attribute that does not exist in the module.
To handle these errors gracefully, use:
“`python
try:
import my_module
except ModuleNotFoundError:
print(“Module not found. Please check the module name and path.”)
“`
Expert Insights on Importing Files in Python
Dr. Emily Carter (Senior Data Scientist, Tech Innovations Inc.). “Importing files in Python is a fundamental skill for any data professional. Utilizing libraries such as Pandas and NumPy not only simplifies data manipulation but also enhances efficiency when handling large datasets.”
Michael Chen (Software Engineer, CodeCraft Solutions). “Understanding the various methods of importing files, including CSV, JSON, and Excel formats, is crucial. Each method has its own advantages, and selecting the right one can significantly impact your project’s performance.”
Sarah Thompson (Python Developer, Open Source Community). “When importing files in Python, it is essential to handle exceptions properly. This ensures that your program can gracefully manage errors related to file paths or formats, which is a common issue in file handling.”
Frequently Asked Questions (FAQs)
How do I import a standard library module in Python?
To import a standard library module in Python, use the `import` statement followed by the module name. For example, `import math` allows you to access mathematical functions and constants defined in the `math` module.
What is the difference between `import` and `from … import`?
The `import` statement imports the entire module, requiring you to prefix functions or classes with the module name (e.g., `math.sqrt()`). The `from … import` statement imports specific functions or classes directly, allowing you to use them without the module prefix (e.g., `sqrt()`).
Can I import a module from a different directory?
Yes, you can import a module from a different directory by modifying the `sys.path` list to include the directory containing the module or by using relative imports if the module is part of a package.
How do I import a file that is not a Python module?
To import a file that is not a Python module, such as a CSV or JSON file, you can use libraries like `pandas` or `json`. For example, use `import pandas as pd` and then `df = pd.read_csv(‘file.csv’)` to read a CSV file.
What should I do if I get an ImportError?
If you encounter an ImportError, verify that the module name is spelled correctly, ensure the module is installed in your environment, and check if the module is located in the Python path. You can also use `pip install module_name` to install missing packages.
How can I import multiple modules in one line?
You can import multiple modules in one line by separating them with commas. For example, `import os, sys` imports both the `os` and `sys` modules simultaneously.
Importing files in Python is a fundamental skill that enables developers to utilize external data and modules effectively. The process typically involves using the built-in `open()` function for reading or writing files, along with context managers to ensure proper resource management. Additionally, the `import` statement is crucial for bringing in modules and libraries, which can significantly enhance the functionality of Python programs.
Several methods exist for importing files, depending on the file type. For text files, using `open()` with modes such as ‘r’ for reading or ‘w’ for writing is common. For structured data formats like CSV or JSON, Python provides specialized libraries such as `csv` and `json`, which simplify the process of data manipulation. Furthermore, the ability to import custom modules enhances code organization and reusability, allowing developers to maintain cleaner and more efficient codebases.
Key takeaways from this discussion include the importance of understanding file modes and the significance of using context managers for file operations. Additionally, leveraging Python’s extensive standard library can streamline the process of working with various file formats. By mastering these techniques, developers can enhance their productivity and ensure their applications are robust and maintainable.
Author Profile
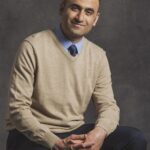
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?