How Can You Import Functions from Another File in Python?
In the world of Python programming, the ability to organize and reuse code is essential for creating efficient and maintainable applications. As your projects grow in complexity, you may find yourself needing to split your code into multiple files or modules. This not only helps in keeping your codebase clean but also allows for better collaboration among developers. One of the fundamental skills every Python programmer should master is how to import functions from another file. This seemingly simple task opens the door to a more modular approach to coding, enabling you to leverage existing functions and enhance your workflow.
Importing functions from another file in Python is a straightforward process, yet it is a powerful feature that can significantly streamline your development process. By understanding how to structure your code across different files, you can avoid redundancy and promote code reuse. Whether you’re working on a small script or a large application, knowing how to effectively manage imports will save you time and effort, allowing you to focus on building features rather than rewriting code.
As we delve deeper into this topic, we will explore the various methods of importing functions, the nuances of Python’s import system, and best practices that can help you maintain a clean and efficient codebase. Whether you’re a beginner looking to grasp the basics or an experienced developer seeking to refine your skills, this guide
Importing Functions Using the Import Statement
To import functions from another file in Python, you typically use the `import` statement. This allows you to access functions defined in one module from another. The syntax for importing is straightforward:
“`python
import module_name
“`
After importing, you can call the functions using the dot notation:
“`python
module_name.function_name()
“`
For example, if you have a file named `math_operations.py` with a function `add`, you can import and use it as follows:
“`python
import math_operations
result = math_operations.add(5, 3)
print(result) Output: 8
“`
Importing Specific Functions
In cases where you only need specific functions from a module, you can import them directly. This is done using the following syntax:
“`python
from module_name import function_name
“`
For example:
“`python
from math_operations import add
result = add(5, 3)
print(result) Output: 8
“`
This method makes the function available without the need to prefix it with the module name.
Importing Multiple Functions
You can also import multiple functions from a module in a single statement, enhancing code readability. The syntax is as follows:
“`python
from module_name import function1, function2
“`
Example:
“`python
from math_operations import add, subtract
result_add = add(5, 3)
result_subtract = subtract(5, 3)
print(result_add) Output: 8
print(result_subtract) Output: 2
“`
Using Aliases for Imported Functions
In scenarios where function names might conflict or if you want to simplify access, you can use aliases. This is accomplished with the `as` keyword:
“`python
from module_name import function_name as alias_name
“`
Example:
“`python
from math_operations import add as addition
result = addition(5, 3)
print(result) Output: 8
“`
Table of Import Methods
Import Method | Syntax | Description |
---|---|---|
Import Entire Module | import module_name |
Imports the entire module, requiring dot notation to access functions. |
Import Specific Function | from module_name import function_name |
Imports a specific function directly for use without dot notation. |
Import Multiple Functions | from module_name import function1, function2 |
Imports multiple functions in a single statement. |
Using Aliases | from module_name import function_name as alias_name |
Imports a function under a different name to avoid conflicts. |
Relative Imports
Relative imports allow you to import functions from modules within the same package. Use a dot notation to specify the current or parent package:
“`python
from .module_name import function_name Current package
from ..module_name import function_name Parent package
“`
This is particularly useful in larger projects with a well-defined package structure. However, relative imports can only be used in modules that are part of a package, and the script should be executed as part of the package.
Importing Functions from Another File in Python
In Python, importing functions from another file allows for better organization and modularity in code. This is typically done using the `import` statement.
Creating a Python Module
To import functions, first, you need a Python module, which is simply a file with a `.py` extension containing your functions. For instance, create a file named `mymodule.py`:
“`python
mymodule.py
def greet(name):
return f”Hello, {name}!”
def add(a, b):
return a + b
“`
Importing the Entire Module
You can import the entire module using the following syntax:
“`python
import mymodule
“`
To use the functions defined in the module, prefix them with the module name:
“`python
print(mymodule.greet(“Alice”)) Output: Hello, Alice!
print(mymodule.add(5, 3)) Output: 8
“`
Importing Specific Functions
If you only need specific functions, you can import them directly:
“`python
from mymodule import greet, add
“`
This allows you to call the functions without the module prefix:
“`python
print(greet(“Bob”)) Output: Hello, Bob!
print(add(10, 5)) Output: 15
“`
Using Aliases
You can also use aliases for easier access:
“`python
from mymodule import greet as hello
“`
Now you can call the function with the alias:
“`python
print(hello(“Charlie”)) Output: Hello, Charlie!
“`
Handling Import Errors
When importing, it is essential to handle potential errors gracefully. Use a `try` and `except` block to catch import errors:
“`python
try:
from mymodule import greet
except ImportError:
print(“Module not found.”)
“`
Organizing Modules in Packages
For larger projects, you can organize modules into packages. Create a directory with an `__init__.py` file:
“`
my_package/
__init__.py
mymodule.py
“`
You can then import functions like this:
“`python
from my_package.mymodule import greet
“`
Best Practices for Importing
- Use absolute imports for clarity.
- Avoid wildcard imports (`from module import *`) as it can lead to confusion.
- Keep imports organized: standard libraries first, third-party libraries second, and local modules last.
- Group imports: separate them by type and provide a blank line between groups.
Import Type | Example |
---|---|
Standard Libraries | `import os` |
Third-Party Libraries | `import numpy as np` |
Local Modules | `from mymodule import greet` |
By adhering to these practices, you can maintain clean and readable code throughout your projects.
Expert Insights on Importing Functions in Python
Dr. Emily Carter (Senior Software Engineer, Python Development Group). “To effectively import functions from another file in Python, one must ensure that the file containing the functions is in the same directory or in the Python path. Utilizing the ‘import’ statement followed by the filename (without the .py extension) allows seamless access to the functions defined within.”
Michael Chen (Lead Python Instructor, Code Academy). “When importing functions, it is crucial to understand the difference between ‘import module’ and ‘from module import function’. The former imports the entire module, while the latter allows direct access to specific functions, promoting cleaner and more efficient code.”
Sarah Thompson (Software Architect, Tech Innovations Inc.). “For larger projects, organizing functions into separate files and using relative imports can enhance code maintainability. This practice not only improves readability but also facilitates easier debugging and testing of individual components.”
Frequently Asked Questions (FAQs)
How do I import a function from another Python file?
To import a function from another Python file, use the `import` statement followed by the filename (without the `.py` extension) and the function name. For example, if you have a file named `my_functions.py` with a function `my_function`, you can import it using `from my_functions import my_function`.
Can I import multiple functions from the same file?
Yes, you can import multiple functions from the same file by separating the function names with commas. For example: `from my_functions import function1, function2`.
What if the function I want to import is in a subdirectory?
If the function is in a subdirectory, ensure that the subdirectory contains an `__init__.py` file, then use the dot notation to import the function. For example: `from subdirectory.my_functions import my_function`.
How can I import all functions from a file?
To import all functions from a file, use the asterisk (*) wildcard. For example: `from my_functions import *`. However, this practice is generally discouraged as it may lead to namespace conflicts.
What should I do if I encounter an ImportError?
An ImportError can occur for several reasons, such as the file not being in the same directory or not being in the Python path. Ensure the file exists, check the spelling, and verify the directory structure.
Can I rename a function when importing it?
Yes, you can rename a function during import using the `as` keyword. For example: `from my_functions import my_function as new_function_name`. This allows you to use `new_function_name` in your code instead of `my_function`.
Importing functions from another file in Python is a fundamental practice that enhances code organization and reusability. By utilizing the import statement, developers can access functions defined in separate modules, which allows for cleaner code and better project structure. This practice not only helps in managing larger codebases but also encourages the separation of concerns, making it easier to maintain and debug code.
There are several methods to import functions, including using the `import` statement for the entire module or the `from … import …` syntax for specific functions. Each method has its advantages; for instance, importing the entire module keeps the namespace clean, while importing specific functions can reduce typing and improve readability. Understanding the nuances of these import methods is essential for effective Python programming.
Additionally, it is important to be aware of the Python module search path, which determines where Python looks for modules to import. Developers can customize this path if necessary, but it is generally best to keep modules organized within the same project directory or in installed packages. Properly structuring your code and understanding the import mechanism can significantly enhance collaboration and scalability in software development.
Author Profile
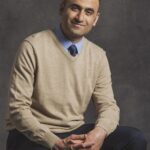
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?