How Can I Import Functions from Another Python File?
### Introduction
In the world of programming, efficiency and organization are paramount. As your Python projects grow in complexity, the need to structure your code becomes increasingly important. One of the most effective ways to achieve this is by modularizing your code into separate files, allowing you to maintain clarity and reusability. If you’ve ever wondered how to import functions from another Python file, you’re not alone. This fundamental skill not only enhances your coding practice but also empowers you to build more scalable and manageable applications.
When working with multiple Python files, understanding how to import functions seamlessly can significantly streamline your development process. By leveraging modules, you can encapsulate related functionalities into distinct files, making it easier to navigate and modify your codebase. This approach not only promotes better organization but also encourages collaboration, as different team members can work on separate components without stepping on each other’s toes.
Moreover, mastering the art of importing functions opens up a world of possibilities for code reuse. Instead of rewriting common functionalities, you can simply import them from a library or another file, saving time and reducing the risk of errors. As we delve deeper into the mechanics of importing functions in Python, you’ll discover various techniques and best practices that will elevate your coding skills and enhance your overall programming experience.
Importing Functions Using the Import Statement
To import functions from another Python file, you can utilize the `import` statement. This allows you to access functions defined in a separate module, facilitating code organization and reusability. The basic syntax for importing a module is as follows:
python
import module_name
Once the module is imported, you can access its functions using the dot notation:
python
module_name.function_name()
Importing Specific Functions
If you only need specific functions from a module, you can import them directly. This is done using the `from` keyword:
python
from module_name import function_name
This approach allows you to call the function directly without needing to prefix it with the module name:
python
function_name()
Using Aliases for Modules and Functions
To simplify your code or avoid naming conflicts, you can use aliases while importing. This can be achieved with the `as` keyword. For example:
python
import module_name as alias_name
Or, for specific functions:
python
from module_name import function_name as alias_function
This way, you can call the functions using the aliases you’ve defined:
python
alias_name.function_name()
alias_function()
Importing All Functions from a Module
If you want to import all functions from a module, you can use an asterisk (*):
python
from module_name import *
While this method is convenient, it is generally discouraged as it can lead to code that is difficult to read and maintain due to potential name conflicts.
Organizing Your Python Files
When structuring your Python files, consider the following guidelines:
- File Naming: Use descriptive names for your Python files to reflect their purpose.
- Folder Structure: Organize your modules into directories to maintain clarity, especially in larger projects.
- __init__.py: Include an `__init__.py` file in your directories to treat them as packages.
Here’s an example of how you might organize your files:
Directory/File | Description |
---|---|
project/ | Main project directory |
project/utils.py | Module containing utility functions |
project/main.py | Main application file |
project/__init__.py | Package initializer |
Example of Importing Functions
Consider the following example where we have two files: `math_utils.py` and `main.py`.
math_utils.py:
python
def add(a, b):
return a + b
def subtract(a, b):
return a – b
main.py:
python
from math_utils import add
result = add(5, 3)
print(result) # Output: 8
This example illustrates how to import the `add` function from `math_utils.py` into `main.py` and use it directly. Following these practices will enhance your code organization, making it more modular and easier to maintain.
Importing Functions Using the `import` Statement
In Python, you can import functions from another file (module) using the `import` statement. This allows you to utilize code from different files in a structured manner.
To import functions, follow these basic steps:
- Ensure Both Files Are in the Same Directory:
- The file you want to import from (let’s call it `module.py`) and the file you’re working in (e.g., `main.py`) should be in the same directory for straightforward imports.
- Use the Import Statement:
- To import the entire module:
python
import module
- To import specific functions from the module:
python
from module import function_name
- Calling the Imported Functions:
- If you imported the entire module:
python
module.function_name()
- If you imported a specific function:
python
function_name()
Using Aliases for Imports
When importing modules or functions, you may encounter names that are lengthy or conflict with existing names. In such cases, you can use aliases.
- Importing with an Alias:
python
import module as mod
You can then call the function using:
python
mod.function_name()
- Importing Specific Functions with Aliases:
python
from module import function_name as fn
You can call the function like this:
python
fn()
Structuring Your Project
Proper structure of your Python project can simplify imports and improve readability. Here’s a recommended layout:
Directory/File Name | Purpose |
---|---|
`project/` | Root directory for your project |
`main.py` | The main script or entry point |
`module.py` | Contains reusable functions |
`utils/` | Folder for utility modules |
`utils/helper.py` | Additional helper functions |
To import from a subfolder, ensure you include an `__init__.py` file to treat it as a package.
Handling Import Errors
When importing functions, you may encounter errors. Common ones include:
- ModuleNotFoundError: Occurs when the module is not found.
- ImportError: Happens when the specified function does not exist in the module.
To troubleshoot:
- Check the file path and ensure both files are in the same directory or properly structured.
- Verify that the function name is correctly spelled and exists in the source module.
Best Practices for Imports
- Only Import What You Need: Importing specific functions instead of entire modules can improve performance.
- Organize Imports: Place all import statements at the beginning of your file for better readability.
- Use Absolute Imports: Prefer absolute imports over relative imports for clarity, especially in larger projects.
By following these guidelines, you can effectively manage and utilize functions across different Python files, enhancing code reusability and organization.
Expert Insights on Importing Functions in Python
Dr. Emily Carter (Senior Python Developer, Tech Innovations Inc.). “To import functions from another Python file, you should ensure that both files are in the same directory or that the directory of the file you want to import is in your Python path. Use the syntax ‘from filename import function_name’ to directly import specific functions, or ‘import filename’ to import the entire module.”
James Liu (Software Engineer, CodeCraft Solutions). “Utilizing relative imports can be beneficial when working within packages. By using a dot notation like ‘from .module import function’, you can maintain clean and organized code while avoiding namespace conflicts.”
Linda Martinez (Python Educator, LearnPythonNow). “It is crucial to understand the __init__.py file when dealing with packages in Python. This file allows Python to recognize directories as packages, enabling seamless function imports across multiple files within the same package.”
Frequently Asked Questions (FAQs)
How do I import a function from another Python file?
To import a function from another Python file, use the `import` statement followed by the filename (without the .py extension) and the function name. For example, if you have a file named `my_functions.py` with a function `my_function`, you can import it using `from my_functions import my_function`.
Can I import multiple functions from the same file?
Yes, you can import multiple functions from the same file by separating the function names with commas. For example, `from my_functions import function_one, function_two`.
What if the file I want to import from is in a different directory?
To import from a different directory, you can use the `sys.path.append()` method to add the directory to your Python path or use relative imports if the files are part of a package structure.
Is it possible to import all functions from a file?
Yes, you can import all functions from a file using the asterisk (*) wildcard. For example, `from my_functions import *` will import all functions defined in `my_functions.py`.
What are the best practices for importing functions in Python?
Best practices include importing only the necessary functions to avoid namespace pollution, using explicit imports rather than wildcard imports, and organizing your code into packages for better maintainability.
Can I rename a function when importing it from another file?
Yes, you can rename a function upon import using the `as` keyword. For example, `from my_functions import my_function as new_function_name` allows you to use `new_function_name` in your code.
Importing functions from another Python file is a fundamental practice that enhances code organization and reusability. By utilizing the import statement, developers can access functions, classes, and variables defined in different modules, which allows for cleaner and more manageable codebases. The process typically involves ensuring that the target file is in the same directory or in a directory that is part of the Python path, enabling seamless access to its contents.
There are several methods to import functions, including the use of the `import` statement, `from … import …`, and `import … as …`. Each method serves different purposes; for instance, using `from … import …` allows for importing specific functions directly, thereby avoiding the need to reference the module name each time. Understanding these import techniques is crucial for effective Python programming, especially in larger projects where modular design is essential.
Additionally, it is important to be mindful of naming conflicts and circular imports, which can complicate the importing process. Developers should adopt best practices such as using clear and descriptive module names and structuring their projects to minimize dependencies. By following these guidelines, programmers can create robust applications that are easier to maintain and extend.
Author Profile
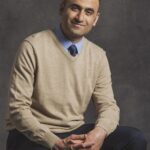
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?