How Can You Import an MPD File in Your Jest Tests?
In the world of JavaScript testing, Jest has emerged as a powerful tool that simplifies the process of writing and executing tests. However, as projects grow in complexity, developers often encounter the need to integrate various file formats into their testing workflows. One such format is the MPD (Media Presentation Description) file, commonly used in streaming applications. If you’ve ever wondered how to seamlessly import an MPD file into your Jest tests, you’re in the right place. This article will guide you through the essentials of handling MPD files within the Jest framework, enabling you to enhance your testing capabilities and ensure your applications perform as expected.
When working with Jest, the ability to import different file types can significantly enrich your testing environment. MPD files, which describe multimedia content for adaptive streaming, can be particularly useful for testing media-related applications. By incorporating these files into your Jest tests, you can simulate various scenarios and ensure that your code interacts correctly with media streams. This not only helps in validating functionality but also in identifying potential issues before they reach production.
Understanding how to import MPD files in Jest tests involves a few key steps, including configuring your Jest environment and utilizing appropriate libraries or plugins. Whether you’re testing a video player, a streaming service, or any application that relies on
Understanding MPD Files
MPD files, or Media Presentation Description files, are essential components used in MPEG-DASH (Dynamic Adaptive Streaming over HTTP) technology. They describe media content, including information about available bitrates, codecs, and segment URLs. When testing applications that involve media streaming, it may be necessary to import and manipulate MPD files programmatically.
Setting Up Jest for MPD File Testing
To effectively test functionalities involving MPD files using Jest, you must ensure that the testing environment is appropriately configured. This includes setting up necessary dependencies and ensuring that Jest can parse and handle MPD file formats.
- Install Jest if it is not already part of your project:
“`bash
npm install –save-dev jest
“`
- If your project requires handling XML (as MPD files are XML-based), consider installing an XML parser:
“`bash
npm install xml2js
“`
Importing MPD Files in Jest Tests
The process of importing MPD files in Jest tests involves reading the file and parsing its contents. Below is a step-by-step guide on how to achieve this.
- **Create an MPD file** for testing. Save it in a directory within your project, e.g., `__mocks__/sample.mpd`.
- **Write the Jest test case**. Use Node.js’s built-in `fs` module to read the MPD file and the `xml2js` library to parse it.
“`javascript
const fs = require(‘fs’);
const xml2js = require(‘xml2js’);
describe(‘MPD File Tests’, () => {
let mpdContent;
beforeAll((done) => {
fs.readFile(‘__mocks__/sample.mpd’, ‘utf8’, (err, data) => {
if (err) {
done(err);
} else {
xml2js.parseString(data, (parseErr, result) => {
if (parseErr) {
done(parseErr);
} else {
mpdContent = result;
done();
}
});
}
});
});
test(‘should contain baseURL’, () => {
expect(mpdContent.MPD.BaseURL).toBeDefined();
});
// Additional tests can be added here
});
“`
Example MPD File Structure
To understand what to expect when importing an MPD file, consider the following simplified structure of an MPD file:
Element | Description |
---|---|
MPD | The root element containing the entire MPD document. |
BaseURL | The base URL for the media segments. |
Period | Defines a period of content; can contain adaptation sets. |
AdaptationSet | Groups different representations of the same content. |
Representation | Describes a specific encoding of the media. |
This structure showcases the hierarchical organization of an MPD file, which is crucial for parsing and testing its content effectively. Understanding this structure can help in creating comprehensive tests that validate the attributes and values defined within the MPD file.
Importing MPD Files in Jest Tests
To effectively import MPD (Media Presentation Description) files in Jest tests, it is important to understand both the structure of MPD files and how Jest handles module imports. Below are detailed steps and considerations.
Setting Up Your Jest Environment
Before importing MPD files, ensure your Jest environment is properly configured to handle the file types involved. Follow these steps:
- Install Necessary Dependencies:
- Ensure you have Jest installed in your project. You can install it via npm:
“`bash
npm install –save-dev jest
“`
- Depending on your needs, you might also require a library to parse MPD files, such as `xml2js`:
“`bash
npm install xml2js
“`
- Configure Jest for File Imports:
- Jest needs to know how to handle non-JavaScript files. You can create a mock for MPD files in your Jest configuration. Add the following to your Jest configuration (usually in `jest.config.js`):
“`javascript
module.exports = {
moduleNameMapper: {
‘\\.(mpd)$’: ‘
}
};
“`
- Create a Mock File:
- In the `__mocks__` directory, create a file named `mpdMock.js`:
“`javascript
module.exports = `
`;
“`
Importing and Using MPD Files in Tests
To import and utilize MPD files in your Jest tests, follow these guidelines:
- **Import the MPD File**:
- In your test file, you can import the MPD like any other module:
“`javascript
import mpdData from ‘./path/to/your/file.mpd’;
“`
- **Parsing the MPD Data**:
- If you need to manipulate or analyze the MPD content, parse it using a library like `xml2js`:
“`javascript
import { parseStringPromise } from ‘xml2js’;
test(‘MPD file structure’, async () => {
const result = await parseStringPromise(mpdData);
expect(result).toHaveProperty(‘MPD’);
});
“`
Writing Tests for MPD File Content
When writing tests, it is crucial to check specific properties and values within the MPD structure:
– **Check for Required Elements**:
“`javascript
test(‘MPD contains Period element’, async () => {
const result = await parseStringPromise(mpdData);
expect(result.MPD.Period).toBeDefined();
});
“`
– **Verify Attributes of Elements**:
“`javascript
test(‘Representation has correct attributes’, async () => {
const result = await parseStringPromise(mpdData);
const representation = result.MPD.Period[0].AdaptationSet[0].Representation[0];
expect(representation.$.id).toBe(‘1’);
expect(representation.$.mimeType).toBe(‘video/mp4’);
});
“`
By following these structured steps, you will be able to effectively import and test MPD files within your Jest testing framework, ensuring your media presentations are correctly structured and functioning as intended.
Expert Insights on Importing MPD Files in Jest Tests
Dr. Emily Carter (Software Testing Specialist, CodeQuality Labs). Importing MPD files in Jest tests requires a clear understanding of the file structure and the Jest configuration. I recommend using the `jest.mock` function to simulate the behavior of the MPD file, allowing you to isolate the tests effectively without relying on the actual file.
Michael Chen (Senior JavaScript Developer, Tech Innovations Inc.). To import an MPD file in Jest tests, you should first ensure that the file is accessible within your project’s directory. Utilizing `fs` to read the file and then parsing it appropriately will enable you to test its contents efficiently. Make sure to handle asynchronous operations properly to avoid test failures.
Sarah Thompson (Lead QA Engineer, Agile Solutions). When dealing with MPD files in Jest, it is crucial to create a mock implementation that mirrors the expected output of the MPD file. This approach not only enhances test reliability but also speeds up the testing process, as it eliminates the need for real file access during each test run.
Frequently Asked Questions (FAQs)
What is an MPD file?
An MPD file is a Media Presentation Description file used in MPEG-DASH (Dynamic Adaptive Streaming over HTTP) to describe the multimedia content and its segments for adaptive streaming.
How can I import an MPD file in a Jest test?
To import an MPD file in a Jest test, you can use a module like `jest.mock` to mock the file import or configure Jest to handle the file type through a custom transformer.
What libraries can assist with MPD file handling in Jest?
Libraries such as `dashjs` for DASH playback and `xml2js` for parsing XML can be useful for handling MPD files in Jest tests.
Can I test MPD file parsing in Jest?
Yes, you can test MPD file parsing in Jest by creating mock MPD files and using assertions to verify that the parsing logic correctly interprets the content.
Are there specific configurations needed in Jest for MPD files?
You may need to configure Jest to handle non-JavaScript file types by using a file transformer or by specifying the file extensions in the Jest configuration.
What is the best practice for testing MPD file imports in Jest?
The best practice is to create isolated tests that mock the MPD file’s content, ensuring that your tests are focused on the functionality being validated without relying on external files.
Importing an MPD (Media Presentation Description) file in Jest tests involves a few essential steps to ensure that the file is correctly recognized and utilized within the testing framework. Jest, being a JavaScript testing framework, typically requires that all imported files are in a format it can handle. Therefore, it is crucial to configure Jest to recognize MPD files, which may not be natively supported.
To successfully import an MPD file, developers often need to create a custom transformer or mock for the MPD file type. This can be achieved by using Jest’s moduleNameMapper configuration in the Jest configuration file. By mapping the MPD file extension to a specific mock or transformer, Jest can process these files during tests, allowing for accurate and efficient testing of components that rely on MPD files.
In summary, importing MPD files in Jest tests requires an understanding of Jest’s configuration options and the ability to set up custom mappings or transformers. By following these steps, developers can ensure that their tests run smoothly and that they can effectively validate the functionality of their code that interacts with MPD files.
Key takeaways include the importance of configuring Jest properly to handle non-standard file types and the value of creating mocks
Author Profile
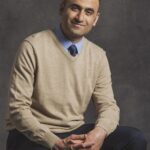
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?