How Can You Import MPD Files into Jest Test Files?
In the ever-evolving landscape of JavaScript testing, Jest has emerged as a powerful tool for developers seeking to ensure their code behaves as expected. As applications grow in complexity, the need for effective testing strategies becomes paramount. Among the various file formats that developers may encounter, MPD (Media Presentation Description) files can present unique challenges when it comes to testing. If you’re looking to streamline your testing process and integrate MPD files into your Jest test suite, you’re in the right place. This article will guide you through the essentials of importing MPD files into your Jest test files, enabling you to enhance your testing capabilities and maintain robust application performance.
Importing MPD files into Jest tests is not just about functionality; it’s about leveraging the full potential of your testing framework. Understanding how to effectively manage and utilize these files can significantly impact your testing workflow. Whether you’re working on a media streaming application or handling complex data structures, knowing how to integrate MPD files can help you simulate real-world scenarios, ensuring that your application performs optimally under various conditions.
In this article, we’ll explore the necessary steps and best practices for importing MPD files into your Jest test files. From setting up your project to troubleshooting common issues, we aim to equip you with the knowledge and tools
Understanding MPD Files
MPD files, or Media Presentation Description files, are typically used in adaptive streaming protocols like MPEG-DASH. They serve as a manifest file that describes how media content should be delivered and played back. When working with Jest, a popular JavaScript testing framework, you may need to import MPD files for testing purposes.
To effectively import MPD files into Jest test files, one must consider the file’s structure and how to handle it within the JavaScript environment. MPD files are XML-based, making them easy to parse and manipulate using JavaScript.
Setting Up Jest for MPD File Imports
Before importing MPD files in Jest, ensure that your testing environment is properly configured. Follow these steps:
- Install Necessary Packages: Ensure that you have Jest and any XML parser libraries installed. A commonly used library for XML parsing in JavaScript is `xml2js`.
“`bash
npm install jest xml2js
“`
- Configure Jest: Update your Jest configuration to handle file imports. You may need to add a custom transformer for MPD files.
Here’s an example of how to set up a transformer in your Jest configuration (usually in `jest.config.js`):
“`javascript
module.exports = {
moduleNameMapper: {
‘\\.(mpd)$’: ‘
},
};
“`
- **Create a Transformer**: Create a file named `mpdTransformer.js` that utilizes the `xml2js` library to convert MPD files into a JavaScript object.
“`javascript
const fs = require(‘fs’);
const xml2js = require(‘xml2js’);
module.exports = {
process(src, filename) {
const content = fs.readFileSync(filename, ‘utf-8’);
let result;
xml2js.parseString(content, (err, parsed) => {
if (err) throw err;
result = parsed;
});
return `module.exports = ${JSON.stringify(result)};`;
},
};
“`
Importing and Using MPD Files in Tests
Once the setup is complete, you can import MPD files directly in your test files. Use the following syntax:
“`javascript
import mpdData from ‘./path/to/your/file.mpd’;
describe(‘MPD File Tests’, () => {
test(‘should have a valid structure’, () => {
expect(mpdData).toHaveProperty(‘MPD’);
// Additional assertions can be added here.
});
});
“`
Best Practices for Testing with MPD Files
When working with MPD files in Jest, adhere to these best practices:
- Validation: Ensure that the MPD file conforms to the MPEG-DASH specifications.
- Mocking: If the MPD file is large or complex, consider mocking the import to streamline testing.
- Error Handling: Implement error handling in your transformer to manage potential parsing issues.
Best Practice | Description |
---|---|
Validation | Check MPD structure against MPEG-DASH standards. |
Mocking | Use mocks for large MPD files to improve test performance. |
Error Handling | Handle parsing errors gracefully in your transformer. |
Understanding MPD Files
MPD files, or Media Presentation Description files, are commonly used in streaming scenarios to describe media content. They contain information about the media segments, including their locations, durations, and encoding formats. To effectively utilize MPD files in Jest test files, it is essential to parse and manipulate these files correctly.
Setting Up Jest for MPD File Handling
Before importing MPD files into Jest test files, ensure that the Jest environment is appropriately set up to handle file imports. Follow these steps:
- Install necessary packages:
“`bash
npm install –save-dev jest
npm install –save-dev mpd-parser
“`
- Configure Jest to recognize the MPD file type in your `jest.config.js`:
“`javascript
module.exports = {
moduleNameMapper: {
‘\\.(mpd)$’: ‘
},
};
“`
Creating a Mock File for MPD
Since Jest does not natively understand MPD files, a mock file can be created. This allows you to simulate the import of MPD files without Jest encountering issues during testing.
- Create a directory named `__mocks__` and add a file named `fileMock.js`:
“`javascript
module.exports = ‘test-mpd-file.mpd’; // or any default string
“`
Importing MPD Files in Your Test Files
Once Jest is configured, you can import MPD files directly into your test files. Here is how you can do it:
- Import the MPD file in your test:
“`javascript
import mpdFile from ‘./path/to/your/file.mpd’;
“`
- Use the MPD file in your tests:
“`javascript
test(‘should parse MPD file correctly’, () => {
const parsedData = parseMpd(mpdFile);
expect(parsedData).toHaveProperty(‘mediaSegments’);
expect(parsedData.mediaSegments.length).toBeGreaterThan(0);
});
“`
Parsing MPD Files
Parsing MPD files requires a suitable parser. The `mpd-parser` package can efficiently handle this task. Here is a basic example of how to parse an MPD file:
- Import the parser:
“`javascript
const { parseMpd } = require(‘mpd-parser’);
“`
- Implement the parsing function:
“`javascript
const parseMpd = (mpdContent) => {
// Logic to parse MPD content
return parsedMpdData; // Return parsed data structure
};
“`
Example Test Implementation
Here is an example of a complete test file that demonstrates the importing and parsing of an MPD file:
“`javascript
import mpdFile from ‘./path/to/your/file.mpd’;
const { parseMpd } = require(‘mpd-parser’);
describe(‘MPD File Tests’, () => {
test(‘should correctly parse MPD file’, () => {
const parsedData = parseMpd(mpdFile);
expect(parsedData).toHaveProperty(‘mediaSegments’);
expect(parsedData.mediaSegments).toBeInstanceOf(Array);
});
});
“`
This structure allows you to efficiently test the functionality related to MPD files in your application while leveraging Jest’s powerful testing framework.
Expert Insights on Importing MPD Files into Jest Test Files
Dr. Emily Carter (Software Testing Specialist, Code Quality Institute). “Importing MPD files into Jest test files requires a clear understanding of the module system used by Jest. Utilizing the correct import syntax and ensuring that the MPD files are properly structured will facilitate seamless integration into your test suite.”
Michael Thompson (Senior JavaScript Developer, Tech Innovations Corp). “When working with MPD files in Jest, it is crucial to configure your Jest environment correctly. This includes setting up the appropriate file transformers and ensuring that your test files can resolve the MPD paths accurately, which can significantly enhance your testing efficiency.”
Sarah Kim (Lead QA Engineer, Agile Solutions Group). “To effectively import MPD files into Jest, one should consider using mocks for any dependencies within the MPD files. This approach not only streamlines the testing process but also allows for more controlled and predictable test outcomes.”
Frequently Asked Questions (FAQs)
What are MPD files and why would I need them in Jest tests?
MPD files, or Media Presentation Description files, are used in multimedia streaming to describe the media content and its properties. In Jest tests, you may need to import MPD files to validate the playback or processing of media streams in your application.
How can I import MPD files into Jest test files?
You can import MPD files into Jest test files by using the `import` statement. Ensure that your Jest configuration allows for importing non-JavaScript files by using a suitable transformer, such as `jest-file-loader` or configuring a custom module mapper.
What Jest configuration is required to handle MPD file imports?
You need to configure Jest to recognize MPD files by adding a module mapper in your Jest configuration. For example, you can use the following configuration in your `jest.config.js` file:
“`javascript
module.exports = {
moduleNameMapper: {
‘\\.(mpd)$’: ‘
},
};
“`
Can I use MPD files directly in my test cases?
Yes, once you have configured Jest to handle MPD files, you can use them directly in your test cases. You can import the MPD file and utilize it in your tests as needed, ensuring that your assertions validate the expected behavior.
Are there any specific libraries that can help with MPD file handling in Jest?
Yes, libraries such as `dash.js` or `mpd-parser` can assist in handling MPD files. These libraries can parse MPD files and provide functionalities to test media playback scenarios effectively within your Jest tests.
What should I do if I encounter errors while importing MPD files in Jest?
If you encounter errors, first check your Jest configuration for proper module mapping. Ensure that the file paths are correct and that the necessary transformers are installed. Additionally, review the console output for specific error messages that can guide you in troubleshooting the issue.
Importing MPD files into Jest test files involves understanding both the structure of MPD files and the Jest testing framework. MPD, or Media Presentation Description, is commonly used in streaming media applications, and integrating it into Jest tests can be crucial for testing media-related functionalities. To achieve this, developers typically utilize appropriate libraries or custom loaders that can parse MPD files and make them accessible during the test execution phase.
One effective approach is to use a mock or a stub that simulates the behavior of the MPD file within the Jest testing environment. This allows for testing of how the application interacts with the media presentation without needing to rely on actual MPD files. Additionally, it is essential to ensure that the test environment is configured correctly to handle any dependencies or modules required for processing MPD files.
integrating MPD files into Jest tests requires a strategic approach that balances the need for accurate media representation with the practicalities of testing. By leveraging mocking techniques and ensuring proper configuration, developers can effectively test their applications’ media functionalities. This not only enhances the robustness of the tests but also contributes to better overall application quality.
Author Profile
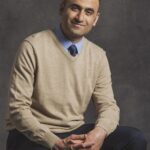
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?