How Can You Import Pi in Python for Your Calculations?
In the world of programming, particularly in Python, the mathematical constant pi (π) holds a special place. Whether you’re delving into geometry, data analysis, or scientific computing, the ability to work with pi can elevate your projects to new heights. But how do you seamlessly incorporate this essential constant into your Python code? Understanding the various methods for importing pi can unlock a treasure trove of possibilities, allowing you to perform complex calculations with ease and precision. In this article, we’ll explore the different ways to bring pi into your Python environment, ensuring that your mathematical endeavors are as accurate as they are efficient.
When it comes to importing pi in Python, there are several straightforward approaches that cater to different needs and preferences. The most common method involves leveraging the built-in libraries that provide access to pi, ensuring that you can utilize this constant without any hassle. Additionally, you might find that certain libraries offer enhanced functionality, allowing for more advanced mathematical operations that go beyond simple calculations.
As we navigate through the intricacies of importing pi, we will also touch on best practices and tips that can help you optimize your code. Whether you’re a beginner eager to learn or an experienced programmer looking to refine your skills, understanding how to effectively incorporate pi into your Python projects is
Importing Pi from the Math Module
In Python, the mathematical constant π (pi) can be easily imported from the built-in `math` module. This module provides a wide range of mathematical functions and constants, including pi, which is essential for calculations involving circles and trigonometry.
To import pi, you can use the following code:
“`python
import math
“`
After importing the module, you can access pi using `math.pi`. Here’s an example of how to use it:
“`python
import math
radius = 5
circumference = 2 * math.pi * radius
print(“Circumference of the circle:”, circumference)
“`
This code calculates the circumference of a circle given its radius.
Alternative Import Method
If you prefer a more concise method, you can import pi directly from the `math` module like this:
“`python
from math import pi
“`
With this method, you can use `pi` directly without the `math.` prefix:
“`python
from math import pi
radius = 5
circumference = 2 * pi * radius
print(“Circumference of the circle:”, circumference)
“`
This approach can make your code cleaner, especially if you use pi frequently throughout your program.
Comparative Use of Pi in Different Libraries
In addition to the `math` module, other libraries such as NumPy also provide access to pi, which can be particularly useful in scientific computing. Below is a comparison of how pi is accessed in different libraries:
Library | Import Statement | Accessing Pi |
---|---|---|
math | import math | math.pi |
NumPy | import numpy as np | np.pi |
SymPy | from sympy import pi | pi |
Each library has its context of use, and choosing one depends on your specific needs. The `math` module is ideal for basic mathematical operations, while NumPy excels in handling arrays and numerical computations.
Using Pi in Calculations
When utilizing pi in calculations, it is important to remember that it is an irrational number, meaning it cannot be expressed as a simple fraction. This characteristic affects precision in floating-point arithmetic. Here are some common calculations involving pi:
- Area of a circle:
\( A = \pi r^2 \)
- Volume of a sphere:
\( V = \frac{4}{3} \pi r^3 \)
- Circumference of a circle:
\( C = 2\pi r \)
When performing these calculations, ensure that you maintain precision by using appropriate data types, such as `float` in Python.
In summary, importing pi in Python is straightforward, and understanding the context in which you need it will help you choose the right approach and library for your mathematical computations.
Importing Pi in Python
To utilize the mathematical constant pi in Python, the most common approach is to use the `math` module, which provides access to mathematical functions and constants, including pi.
Using the Math Module
To import pi from the `math` module, use the following syntax:
“`python
import math
pi_value = math.pi
print(pi_value)
“`
This code snippet demonstrates how to import the `math` module and access the constant `pi`. The variable `pi_value` will hold the value of pi, which is approximately 3.14159.
Alternative Method: Using the NumPy Library
If you are working with numerical data or require additional mathematical functions, consider using the NumPy library, which also includes pi. Here’s how to import and use it:
“`python
import numpy as np
pi_value = np.pi
print(pi_value)
“`
The NumPy library is particularly useful for array operations and more complex mathematical computations.
Comparative Table: Math vs. NumPy
Feature | Math Module | NumPy Library |
---|---|---|
Import Syntax | `import math` | `import numpy as np` |
Constant Pi | `math.pi` | `np.pi` |
Additional Functions | Limited to math | Extensive, supports arrays and matrices |
Use Case | Basic math functions | Scientific computing, data analysis |
Accessing Pi with Other Libraries
In addition to the `math` and `numpy` libraries, you can also find pi in other libraries:
- SymPy: A library for symbolic mathematics, which can represent pi as a symbol.
“`python
from sympy import pi
pi_symbolic = pi
print(pi_symbolic)
“`
- SciPy: Often used for scientific and technical computing, it also inherits from NumPy and provides pi.
“`python
from scipy.constants import pi
pi_value = pi
print(pi_value)
“`
By selecting the appropriate library based on your project requirements, you can effectively incorporate pi into your Python applications.
Expert Insights on Importing Pi in Python
Dr. Emily Carter (Senior Data Scientist, Tech Innovations Inc.). “To import the mathematical constant pi in Python, one can utilize the math module, which provides a straightforward approach. By executing ‘import math’, users can access ‘math.pi’ to retrieve the value of pi with high precision.”
Michael Chen (Python Developer, Open Source Advocate). “For those working with scientific computations, using ‘from math import pi’ is an efficient method to directly access pi without the need to prefix it with ‘math.’. This can streamline code readability and efficiency in calculations.”
Sarah Thompson (Educational Technology Specialist, Python for Education). “In educational settings, it’s crucial to demonstrate how to import pi in Python effectively. I recommend showing students both ‘import math’ and ‘from math import pi’ to illustrate different import techniques and their applications in various scenarios.”
Frequently Asked Questions (FAQs)
How do I import the math module to access pi in Python?
To access the constant pi in Python, you need to import the math module using the statement `import math`. You can then use `math.pi` to get the value of pi.
Is there a way to import pi without using the math module?
Yes, you can define pi manually in your code. For example, you can set `pi = 3.14159` or use a more precise value like `pi = 3.141592653589793`.
Can I import pi from other libraries besides math?
Yes, other libraries such as NumPy also provide access to pi. You can import it using `import numpy as np` and then use `np.pi`.
What is the precision of pi in the math module?
The precision of pi in the math module is typically up to 15 decimal places, represented as `3.141592653589793`.
Are there any performance considerations when using pi from different libraries?
Generally, using pi from the math module is more efficient than from other libraries like NumPy, especially for simple calculations, due to its lower overhead.
Can I use pi in mathematical operations directly after importing it?
Yes, once you import pi from the math module or any other source, you can use it directly in mathematical operations, such as `area = math.pi * radius ** 2`.
Importing the mathematical constant pi in Python is a straightforward process that can be accomplished using the built-in math module. By utilizing the `import math` statement, users gain access to a variety of mathematical functions and constants, including pi, which can be referenced as `math.pi`. This approach ensures that calculations involving pi are both accurate and efficient, as the constant is defined to a high degree of precision within the math module.
Additionally, for those who prefer using libraries that offer more advanced mathematical capabilities, the NumPy library is another excellent option. By importing NumPy with `import numpy as np`, users can access pi through `np.pi`. This is particularly beneficial for scientific computing and data analysis, where the use of arrays and matrices is common, and pi is frequently required in various calculations.
In summary, whether one chooses to use the standard math module or the more specialized NumPy library, importing pi in Python is a simple yet essential task for anyone involved in mathematical programming. Understanding these methods not only enhances coding efficiency but also contributes to the overall accuracy of mathematical computations in Python applications.
Author Profile
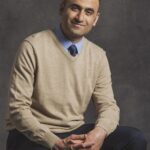
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?