How Can You Import a Python File Effectively?
In the world of Python programming, efficiency and organization are key to writing clean, maintainable code. One of the fundamental skills every Python developer must master is the ability to import files. Whether you’re working on a small script or a large-scale application, knowing how to import Python files effectively can streamline your workflow, enhance code reusability, and foster collaboration. This article will guide you through the various methods of importing Python files, empowering you to harness the full potential of modular programming.
When you import a Python file, you essentially bring in functions, classes, and variables defined in that file into your current script. This not only saves time but also helps in structuring your code logically. Python provides several ways to import files, allowing developers to choose the method that best suits their project’s needs. From simple imports to more advanced techniques involving packages and modules, understanding these options is crucial for any aspiring programmer.
Moreover, the process of importing isn’t just about pulling in code; it’s also about managing namespaces and avoiding conflicts. As you delve deeper into the intricacies of importing Python files, you’ll discover best practices that can prevent common pitfalls and enhance the overall quality of your code. So, whether you’re a beginner looking to grasp the basics or an experienced developer seeking to refine
Importing a Python File
To import a Python file, you can use the `import` statement, which allows you to access functions, classes, and variables defined in that file. The basic syntax for importing a module is straightforward:
“`python
import module_name
“`
This imports the entire module, and you can then access its contents using the dot notation:
“`python
module_name.function_name()
“`
If you only need specific parts of a module, you can use the following syntax:
“`python
from module_name import specific_function
“`
This allows you to call `specific_function()` directly without needing to prefix it with the module name.
Different Import Methods
There are several ways to import Python files, each serving different purposes. Below are the most common methods:
- Importing the entire module:
This gives you access to all the functions and classes within the module.
“`python
import math
result = math.sqrt(16)
“`
- Importing specific functions or classes:
This reduces memory usage and can make the code cleaner.
“`python
from math import sqrt
result = sqrt(16)
“`
- Importing with an alias:
You can create an alias for a module, which can be useful for shortening long module names.
“`python
import pandas as pd
df = pd.DataFrame()
“`
Example of Module Structure
Assuming you have the following structure:
“`
project/
│
├── main.py
└── my_module.py
“`
In `my_module.py`, you may define a function:
“`python
my_module.py
def greet(name):
return f”Hello, {name}!”
“`
In `main.py`, you can import and use this function:
“`python
main.py
from my_module import greet
print(greet(“Alice”)) Output: Hello, Alice!
“`
Using the `__init__.py` File
To treat a directory as a package, it should contain an `__init__.py` file. This file can be empty or can contain initialization code for the package. When importing from a package, you would do it as follows:
“`python
from package_name import module_name
“`
Handling Import Errors
When importing modules, you may encounter errors. Here are some common ones and their resolutions:
Error Type | Description | Resolution |
---|---|---|
ModuleNotFoundError | The specified module could not be found. | Check the module name and path. |
ImportError | The module does not have the specified function. | Ensure the function is defined in the module. |
Circular Import | Two modules import each other. | Refactor the code to avoid circular dependencies. |
Best Practices for Imports
- Always import modules at the top of your file.
- Use absolute imports over relative imports for better readability.
- Avoid wildcard imports (using `from module_name import *`) as they can lead to confusion about which names are present in the namespace.
- Group your imports into standard library imports, third-party imports, and local application/library imports, separated by blank lines for clarity.
By following these guidelines, you can effectively manage module imports in Python, leading to cleaner and more maintainable code.
Importing Python Files
To import a Python file, you can utilize several methods depending on your needs and the structure of your project. The most common way is by using the `import` statement, which allows you to access functions, classes, and variables defined in another Python file.
Basic Import Syntax
The simplest form of importing a Python file is using the `import` keyword followed by the name of the file (without the `.py` extension).
Example:
“`python
import my_module
“`
This command imports `my_module.py`, allowing you to access its contents using the module name as a prefix.
Using from…import
If you want to import specific functions or classes from a module, you can use the `from…import` syntax. This method can help you avoid cluttering your namespace.
Example:
“`python
from my_module import my_function
“`
After this import, you can directly call `my_function()` without needing to prefix it with the module name.
Importing All Contents
To import all functions and classes from a module, you can use the asterisk (`*`) wildcard. However, this practice is generally discouraged due to the potential for name clashes.
Example:
“`python
from my_module import *
“`
This will import everything defined in `my_module.py`.
Relative Imports
In larger projects where modules are organized into packages, you can use relative imports. This method allows you to import a module relative to the location of the current module.
Example:
“`python
from . import my_module Current package
from .. import another_module Parent package
“`
Relative imports can enhance code readability and maintainability in complex applications.
Importing from Subdirectories
If your Python file is located in a subdirectory (package), you’ll need to ensure that the directory contains an `__init__.py` file to be recognized as a package.
Example structure:
“`
my_project/
│
├── main.py
└── my_package/
├── __init__.py
└── my_module.py
“`
You can import `my_module` in `main.py` like this:
“`python
from my_package import my_module
“`
Using the sys.path for Custom Imports
If your module is located outside the standard directories, you can add its path to `sys.path`.
Example:
“`python
import sys
sys.path.append(‘/path/to/your/module’)
import my_module
“`
This method allows Python to recognize and import files from custom locations.
Importing JSON or CSV Files
For non-Python files like JSON or CSV, you can use built-in libraries such as `json` or `csv`.
Example for JSON:
“`python
import json
with open(‘data.json’) as f:
data = json.load(f)
“`
Example for CSV:
“`python
import csv
with open(‘data.csv’, newline=”) as f:
reader = csv.reader(f)
for row in reader:
print(row)
“`
Best Practices for Module Imports
- Limit Imports: Only import what you need to keep the namespace clean.
- Order of Imports: Follow a logical order—standard libraries first, third-party libraries next, and local application imports last.
- Use Aliases: For long module names, use aliases to simplify access.
Example:
“`python
import numpy as np
“`
- Avoid Circular Imports: Structure your code to prevent circular dependencies, which can lead to import errors.
Using these methods and practices, you can effectively manage imports in your Python projects, enhancing modularity and maintainability.
Expert Insights on Importing Python Files
Dr. Emily Chen (Senior Software Engineer, Tech Innovations Inc.). “When importing a Python file, it is crucial to ensure that the file is in the same directory as your script or that the directory is included in your Python path. This allows Python to locate the module you wish to import seamlessly.”
James Patel (Python Developer, CodeCraft Solutions). “Utilizing the ‘import’ statement effectively can enhance code organization and reusability. For larger projects, consider structuring your code into packages, which can be imported using the ‘from … import …’ syntax for better clarity.”
Lisa Nguyen (Data Scientist, Analytics Hub). “Always remember to handle potential import errors gracefully. Using try-except blocks when importing modules can prevent your program from crashing due to missing files or incorrect paths, ensuring a smoother user experience.”
Frequently Asked Questions (FAQs)
How do I import a Python file located in the same directory?
To import a Python file located in the same directory, use the `import` statement followed by the filename without the `.py` extension. For example, if the file is named `my_module.py`, use `import my_module`.
Can I import a Python file from a different directory?
Yes, you can import a Python file from a different directory by modifying the `sys.path` list to include the directory path. For example:
“`python
import sys
sys.path.append(‘/path/to/directory’)
import my_module
“`
What is the difference between `import` and `from … import`?
The `import` statement imports the entire module, requiring the module name as a prefix to access its functions or classes. The `from … import` statement allows you to import specific functions or classes directly, enabling you to use them without the module prefix.
How can I import a Python file with a different name?
You can import a Python file with a different name using the `import` statement followed by the `as` keyword to create an alias. For example:
“`python
import my_module as mm
“`
This allows you to refer to `my_module` as `mm`.
What should I do if I encounter an ImportError?
If you encounter an ImportError, verify that the file exists in the specified directory, check for typos in the filename, and ensure that the directory is included in the Python path. Additionally, confirm that there are no circular imports.
Can I import a Python file that is not in the Python path?
Yes, you can import a Python file that is not in the Python path by appending its directory to `sys.path` or using relative imports if the file is part of a package. Ensure that the directory containing the file is accessible during runtime.
In summary, importing a Python file is a fundamental skill that every Python programmer should master. The process typically involves using the `import` statement, which allows you to access functions, classes, and variables defined in another Python file. This capability not only promotes code reusability but also enhances the organization of code by enabling modular programming. Understanding the different ways to import files, such as using `import`, `from … import`, and `import as`, is essential for effective coding practices.
Moreover, it is important to recognize the significance of the Python module search path, which determines where Python looks for the modules to import. By default, Python searches in the current directory and the directories listed in the `sys.path` variable. If a module is not found, it will raise an `ImportError`, highlighting the need for proper file management and organization within your project structure.
Key takeaways include the importance of maintaining a clear directory structure for your Python files to facilitate easy imports. Additionally, leveraging the `__init__.py` file can help in treating directories as packages, allowing for more complex project structures. By adhering to these practices, developers can create more maintainable and scalable Python applications, ultimately leading to improved productivity and collaboration
Author Profile
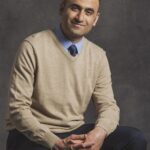
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?