How Can You Import a Text File in Python? A Step-by-Step Guide
In the world of programming, data is king, and the ability to manipulate and analyze that data is essential for any aspiring developer. Python, known for its simplicity and versatility, provides a myriad of ways to work with data, including the straightforward task of importing text files. Whether you’re a seasoned programmer or just starting your coding journey, understanding how to efficiently import and handle text files in Python can unlock new possibilities for your projects.
Importing text files is a fundamental skill that lays the groundwork for more complex data processing tasks. With Python’s robust libraries and built-in functions, you can easily read, write, and manipulate text data, making it an invaluable tool for data analysis, automation, and application development. This process not only enhances your coding abilities but also equips you with the knowledge to handle real-world data challenges effectively.
As we delve deeper into the nuances of importing text files in Python, you’ll discover various methods and best practices that can streamline your workflow. From understanding file formats to leveraging Python’s powerful libraries, this guide will empower you to confidently manage text data and elevate your programming skills to new heights. So, let’s embark on this journey to master the art of importing text files in Python!
Reading a Text File
To read a text file in Python, the built-in `open()` function is utilized. This function allows you to specify the file path and the mode in which you want to open the file. Common modes include:
- `’r’`: Read (default mode)
- `’w’`: Write (overwrites the file if it exists)
- `’a’`: Append (adds to the end of the file)
- `’b’`: Binary mode (for non-text files)
Here is a simple example of how to read a text file:
“`python
with open(‘example.txt’, ‘r’) as file:
content = file.read()
print(content)
“`
Using the `with` statement ensures that the file is properly closed after its suite finishes, even if an error occurs.
Reading Line by Line
If you wish to read a file line by line, you can use a loop. This method is particularly useful for large files, as it reduces memory consumption. Here’s how to do it:
“`python
with open(‘example.txt’, ‘r’) as file:
for line in file:
print(line.strip()) strip() removes any leading/trailing whitespace
“`
This approach allows you to process each line individually, making it easy to implement logic based on the content of each line.
Reading All Lines into a List
If you prefer to read all lines at once and store them in a list, you can use the `readlines()` method. This method returns a list where each element is a line from the file.
“`python
with open(‘example.txt’, ‘r’) as file:
lines = file.readlines()
print(lines)
“`
This can be useful for applications where you need to perform operations on all lines simultaneously.
Writing to a Text File
To write to a text file, use the `open()` function with the `’w’` or `’a’` mode. The following example demonstrates how to create a new file and write to it:
“`python
with open(‘output.txt’, ‘w’) as file:
file.write(“Hello, World!\n”)
file.write(“This is a new line.”)
“`
If you want to append to an existing file instead of overwriting it, use the `’a’` mode:
“`python
with open(‘output.txt’, ‘a’) as file:
file.write(“\nAppending a new line.”)
“`
Handling Exceptions
When working with file operations, it’s crucial to handle exceptions to avoid crashes due to issues like missing files or permission errors. Use a try-except block as shown below:
“`python
try:
with open(‘example.txt’, ‘r’) as file:
content = file.read()
except FileNotFoundError:
print(“The file does not exist.”)
except IOError:
print(“Error occurred while reading the file.”)
“`
This practice enhances the robustness of your code by gracefully managing errors.
File Handling Summary
The following table summarizes the key methods and their use cases:
Method | Description |
---|---|
open(file, mode) | Opens a file and returns a file object. |
read() | Reads the entire content of the file. |
readline() | Reads the next line from the file. |
readlines() | Reads all lines and returns them as a list. |
write(string) | Writes the given string to the file. |
close() | Closes the file, freeing up system resources. |
Incorporating these techniques will facilitate effective file handling in Python applications.
Reading a Text File
To read a text file in Python, the built-in `open()` function is commonly used. This function requires the file name and mode as parameters. The most common modes are:
- `’r’`: Read (default mode).
- `’w’`: Write (overwrites the file).
- `’a’`: Append (adds to the file).
- `’b’`: Binary mode.
Example of Reading a File
“`python
with open(‘example.txt’, ‘r’) as file:
content = file.read()
print(content)
“`
In this example, the `with` statement ensures proper acquisition and release of resources, automatically closing the file after its suite finishes.
Reading Line by Line
For large files, reading them line by line is more efficient. This can be achieved using a loop:
Example of Line-by-Line Reading
“`python
with open(‘example.txt’, ‘r’) as file:
for line in file:
print(line.strip())
“`
The `strip()` method removes any leading and trailing whitespace, including newlines.
Reading File into a List
To read all lines of a file into a list, you can use the `readlines()` method.
Example of Using readlines()
“`python
with open(‘example.txt’, ‘r’) as file:
lines = file.readlines()
print(lines)
“`
This returns a list where each element corresponds to a line in the file.
Writing to a Text File
To write to a text file, use the `open()` function with the `’w’` or `’a’` mode.
Example of Writing to a File
“`python
with open(‘example.txt’, ‘w’) as file:
file.write(“Hello, World!\n”)
“`
This code overwrites the content of `example.txt` with “Hello, World!”.
Appending to a Text File
To add content to the end of an existing file, use the `’a’` mode.
Example of Appending to a File
“`python
with open(‘example.txt’, ‘a’) as file:
file.write(“Appending this line.\n”)
“`
This appends “Appending this line.” to the end of `example.txt`.
Using Context Managers
Using the `with` statement is a best practice for file operations because it ensures that the file is properly closed after its suite finishes, even if an error occurs.
Advantages of Using Context Managers
- Automatically handles file closure.
- Reduces the risk of memory leaks.
- Simplifies code structure.
Handling File Errors
When dealing with file operations, it’s essential to handle potential errors using `try` and `except`.
Example of Error Handling
“`python
try:
with open(‘non_existent_file.txt’, ‘r’) as file:
content = file.read()
except FileNotFoundError:
print(“The file does not exist.”)
“`
This code snippet will catch a `FileNotFoundError` and print a user-friendly message.
Conclusion on File Operations
Understanding how to import and manipulate text files in Python is crucial for data handling and processing. By leveraging the various methods available, you can efficiently read from and write to files, while ensuring robust error handling and resource management.
Expert Insights on Importing Text Files in Python
Dr. Emily Chen (Data Scientist, Tech Innovations Inc.). “When importing text files in Python, utilizing the built-in `open()` function is essential. It allows for efficient reading and writing operations, especially when handling large datasets. I recommend always using context managers to ensure files are properly closed after their use.”
Michael Thompson (Software Engineer, CodeCraft Solutions). “For beginners, the `pandas` library provides an intuitive way to import text files, especially CSVs. Using `pandas.read_csv()` not only simplifies the process but also offers powerful data manipulation capabilities right after importing.”
Sarah Patel (Python Developer, Data Insights Group). “Understanding the encoding of your text files is crucial when importing them in Python. Using the `encoding` parameter with `open()` or `pandas` functions can prevent common pitfalls related to character encoding issues, ensuring data integrity.”
Frequently Asked Questions (FAQs)
How do I read a text file in Python?
You can read a text file in Python using the built-in `open()` function along with the `read()`, `readline()`, or `readlines()` methods. For example, `with open(‘file.txt’, ‘r’) as file: content = file.read()` reads the entire file content.
What is the difference between ‘r’, ‘w’, and ‘a’ modes in file handling?
The ‘r’ mode opens a file for reading, ‘w’ mode opens a file for writing (and truncates the file if it exists), while ‘a’ mode opens a file for appending data at the end of the file without truncating it.
How can I import a text file into a list in Python?
You can import a text file into a list by using the `readlines()` method. For example, `with open(‘file.txt’, ‘r’) as file: lines = file.readlines()` will create a list where each element is a line from the file.
Is it necessary to close a file after opening it in Python?
Yes, it is essential to close a file after opening it to free up system resources. Using the `with` statement automatically handles closing the file for you, ensuring proper resource management.
Can I read a text file line by line in Python?
Yes, you can read a text file line by line using a for loop. For example, `with open(‘file.txt’, ‘r’) as file: for line in file: print(line)` processes each line individually.
What should I do if the text file does not exist while trying to import it?
If the text file does not exist, Python will raise a `FileNotFoundError`. You can handle this by using a try-except block to catch the exception and take appropriate action, such as notifying the user or creating the file.
Importing a text file in Python is a straightforward process that can be accomplished using various built-in functions and libraries. The most common method involves using the `open()` function, which allows you to specify the file’s path and the mode in which to open it (e.g., read, write, or append). Once the file is opened, you can read its contents using methods such as `read()`, `readline()`, or `readlines()`, depending on your specific needs for data handling.
Additionally, Python provides context managers, which are particularly useful for managing file operations. By using the `with` statement, you can ensure that files are properly closed after their contents have been processed, thus preventing potential memory leaks or file corruption. This approach enhances code readability and reliability, making it a best practice for file handling in Python.
Moreover, for more complex data manipulation, libraries such as `pandas` can be utilized to import text files into data frames, allowing for more advanced data analysis and processing. This is especially beneficial when dealing with structured data formats like CSV or TSV, where the ability to manipulate data efficiently is crucial.
importing text files in Python is an essential skill that
Author Profile
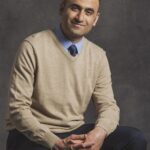
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?