How Can You Increment a Variable in Python Effectively?
In the world of programming, the ability to manipulate variables is fundamental to creating dynamic and responsive applications. Among the most common operations you’ll encounter is incrementing a variable—a simple yet powerful concept that forms the backbone of countless algorithms and processes. Whether you’re tallying scores in a game, counting iterations in a loop, or managing data in a database, knowing how to effectively increment a variable in Python can streamline your code and enhance its functionality.
At its core, incrementing a variable involves increasing its value by a specified amount, typically one. Python, with its clear syntax and user-friendly nature, offers several straightforward methods to achieve this. As you delve into the intricacies of variable manipulation, you’ll discover not only the basic techniques but also the nuances that can optimize your code for performance and readability.
Understanding how to increment a variable is not just about knowing the syntax; it’s about grasping the underlying principles of variable states and operations in Python. From simple loops to complex data structures, mastering this skill will empower you to write more efficient and effective code. So, let’s explore the various ways to increment a variable in Python and unlock the potential of this essential programming practice.
Incrementing a Variable
In Python, incrementing a variable is a straightforward operation that can be accomplished using the `+=` operator. This operator allows you to add a specified value to a variable and assign the result back to that variable, effectively updating its value.
For example, if you have a variable `x` initialized with a value of 5, you can increment it by 1 as follows:
“`python
x = 5
x += 1 Now x is 6
“`
This method is not only concise but also improves code readability. Below are some common practices for incrementing variables in Python:
- Using the `+=` operator: This is the most common and preferred way to increment variables.
- Using a simple addition assignment: While less common, you can also achieve the same by explicitly writing the addition:
“`python
x = x + 1 This also results in x being 6
“`
- Using a loop for repeated increments: When you need to increment a variable multiple times, a loop can be utilized:
“`python
x = 0
for _ in range(5): Increment x by 1, five times
x += 1
“`
Incrementing with Different Values
You can also increment a variable by values other than 1. This can be useful in various scenarios, such as when working with step counters or accumulators. Here’s how you can increment a variable by different values:
- To increment by 2:
“`python
x = 5
x += 2 Now x is 7
“`
- To increment by a variable:
“`python
increment_value = 3
x = 5
x += increment_value Now x is 8
“`
Incrementing in a Function
When working with functions, you might need to increment a variable passed as an argument. Since integers are immutable in Python, you cannot change the value of an integer directly within a function. However, you can return the incremented value:
“`python
def increment(num):
return num + 1
x = 5
x = increment(x) Now x is 6
“`
Alternatively, you can utilize mutable types, such as lists, to achieve similar functionality:
“`python
def increment(lst):
lst[0] += 1
x = [5] Using a list to hold the value
increment(x) Now x[0] is 6
“`
Common Mistakes
When incrementing variables, developers often make a few common mistakes. Here are some to watch out for:
Mistake | Description |
---|---|
Forgetting to reassign | Not assigning the incremented value back to the variable. |
Confusing `++` with `+=` | Python does not support the `++` operator as in some other languages. |
Overlooking variable scope | Attempting to increment a variable that is out of scope. |
By keeping these points in mind and using the correct syntax, you can effectively manage variable increments in your Python programs.
Incrementing a Variable Using the Addition Assignment Operator
In Python, one of the most common methods to increment a variable is by using the addition assignment operator (`+=`). This operator allows you to add a specific value to a variable and simultaneously update the variable with the new value.
For example:
“`python
count = 0
count += 1 count is now 1
count += 5 count is now 6
“`
This method is straightforward and efficient, particularly when incrementing by a constant value.
Incrementing a Variable Using the Increment Function
While Python does not have a built-in increment operator like some other programming languages (e.g., `++` in C++), you can define a custom function to increment values. This can enhance code readability and reusability.
“`python
def increment(value, amount=1):
return value + amount
counter = 0
counter = increment(counter) counter is now 1
counter = increment(counter, 3) counter is now 4
“`
This function can be customized to accept different increments, making it versatile.
Using a Loop to Increment a Variable
When you need to increment a variable multiple times, using a loop can be beneficial. The following example demonstrates how to increment a variable within a `for` loop:
“`python
total = 0
for i in range(5): This will loop 5 times
total += 1 Increment total by 1 each iteration
“`
After the loop, `total` will equal 5, showcasing how loops can be used for repeated increments.
Incrementing Variables in Data Structures
In situations where you are working with data structures, such as lists or dictionaries, you can increment values contained within them. Here’s how to do this for both types:
For Lists:
“`python
numbers = [1, 2, 3]
for i in range(len(numbers)):
numbers[i] += 1 Each element is incremented by 1
“`
For Dictionaries:
“`python
scores = {‘Alice’: 5, ‘Bob’: 3}
scores[‘Alice’] += 1 Increment Alice’s score by 1
“`
This method allows for selective incrementing based on keys or indices.
Table of Increment Methods
Method | Description | Example |
---|---|---|
Addition Assignment Operator (`+=`) | Directly increments the variable by a specified amount. | `count += 1` |
Custom Increment Function | A reusable function to increment variables. | `counter = increment(counter)` |
Loop | Repeatedly increments a variable over iterations. | `for i in range(5): total += 1` |
List Increment | Increment elements within a list. | `numbers[i] += 1` |
Dictionary Increment | Increment values associated with keys in a dictionary. | `scores[‘Alice’] += 1` |
Each of these methods offers flexibility depending on the context and requirements of your code.
Expert Insights on Incrementing Variables in Python
Dr. Emily Carter (Senior Python Developer, Tech Innovations Inc.). “Incrementing a variable in Python is straightforward and can be achieved using the ‘+=’ operator. This operator simplifies the process, allowing developers to write cleaner and more efficient code.”
Michael Thompson (Lead Software Engineer, CodeCraft Solutions). “In Python, incrementing a variable is not only about adding one but understanding the context of the variable’s use. Using the correct data type and method is crucial for maintaining code integrity.”
Lisa Nguyen (Python Educator, LearnPythonToday). “For beginners, mastering the increment operation is essential. It lays the foundation for more complex programming concepts, and using the ‘+= 1’ syntax is a great way to start practicing variable manipulation.”
Frequently Asked Questions (FAQs)
How do I increment a variable in Python?
You can increment a variable in Python using the `+=` operator. For example, `x += 1` increases the value of `x` by 1.
Can I increment a variable by a value other than 1?
Yes, you can increment a variable by any numeric value. For instance, `x += 5` increases the value of `x` by 5.
Is there a difference between `x += 1` and `x = x + 1`?
Both expressions achieve the same result, but `x += 1` is more concise and can be more efficient in certain contexts, especially with mutable types.
What happens if I increment a variable that hasn’t been initialized?
If you attempt to increment an uninitialized variable, Python will raise a `NameError`, indicating that the variable is not defined.
Can I use the increment operator in a loop?
Yes, you can use the increment operator within loops to update a variable, such as in a `for` or `while` loop, to control iterations or accumulate values.
Are there any best practices for incrementing variables in Python?
It is advisable to use clear and descriptive variable names to enhance code readability. Additionally, avoid unnecessary increments that can lead to confusion or errors in logic.
In Python, incrementing a variable is a straightforward process that can be accomplished using the addition assignment operator (`+=`). This operator allows you to add a specified value to an existing variable efficiently. For example, if you have a variable `x` and you want to increment it by 1, you can simply write `x += 1`. This method not only enhances code readability but also promotes a more concise coding style.
Another common method to increment a variable is by using the standard addition operation. For instance, you can achieve the same result with `x = x + 1`. While this approach is equally valid, it is generally less preferred in Python due to its verbosity compared to the addition assignment operator. Both methods yield the same outcome, but the choice between them often depends on personal or team coding style preferences.
Additionally, it is important to consider the context in which you are incrementing a variable. In loops, such as `for` or `while`, incrementing is often used to control the number of iterations. Understanding how to effectively manage variable increments can significantly impact the performance and clarity of your code. Overall, mastering variable incrementation is a fundamental skill that enhances programming proficiency in Python.
Author Profile
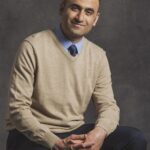
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?