How Do You Indent in Python: Mastering the Essential Formatting Technique?
### How to Indent in Python: Mastering the Art of Code Structure
In the world of programming, clarity and structure are paramount, and nowhere is this more evident than in Python. Unlike many other programming languages that rely on braces or keywords to define code blocks, Python uses indentation to convey the hierarchy and flow of the code. This unique approach not only enhances readability but also enforces a disciplined coding style that can significantly impact the development process. If you’ve ever found yourself puzzled by indentation errors or unsure about the best practices for structuring your Python code, you’re not alone. Understanding how to properly indent in Python is essential for both novice programmers and seasoned developers alike.
Indentation in Python serves as a visual cue that indicates which statements belong to which blocks of code. Whether you’re defining functions, creating loops, or implementing conditional statements, the way you indent your code can determine how it executes. This article will guide you through the fundamental principles of indentation, including the importance of consistency and the common pitfalls to avoid. By mastering these concepts, you’ll not only write cleaner code but also reduce the likelihood of errors that can arise from improper indentation.
As we delve deeper into this topic, we will explore various indentation styles, the significance of tabs versus spaces, and best practices that can help
Understanding Indentation in Python
Indentation is a crucial aspect of Python’s syntax. Unlike many programming languages that use braces or keywords to define blocks of code, Python uses indentation to determine the grouping of statements. This design decision makes Python code often more readable, but it requires a clear understanding of how to implement it correctly.
How Indentation Works
In Python, indentation is used to define the scope of loops, functions, and conditional statements. Each block of code must be indented consistently. The standard practice is to use four spaces per indentation level. Mixing spaces and tabs can lead to errors that are often difficult to trace, as Python will throw an `IndentationError` if the indentation is inconsistent.
- Consistent Indentation: Choose either spaces or tabs and stick with it throughout your code.
- Standard Practice: Use four spaces for each indentation level.
- Visual Clarity: Indentation helps to visually separate blocks of code, enhancing readability.
Common Indentation Patterns
When writing Python code, you will encounter several common patterns that require indentation. Below are some examples of these patterns:
- If Statements:
python
if condition:
# indented block of code
do_something()
- For Loops:
python
for i in range(5):
# indented block of code
print(i)
- Function Definitions:
python
def my_function():
# indented block of code
return True
Best Practices for Indentation
To ensure your code remains clean and error-free, follow these best practices regarding indentation:
- Use a Code Editor: Utilize an Integrated Development Environment (IDE) or a code editor that automatically handles indentation for you.
- Enable Whitespace Characters: Many editors allow you to visualize spaces and tabs. This feature can help you avoid mixing indentation types.
- Format Your Code: Use tools like `black` or `autopep8` to format your Python code automatically, ensuring consistent indentation.
Common Indentation Errors
Indentation errors in Python can lead to unexpected behavior or crashes. Below is a table highlighting common indentation issues and their solutions:
Error Type | Description | Solution |
---|---|---|
IndentationError | Occurs when there are inconsistencies in indentation. | Check for mixed spaces and tabs; use a consistent indentation style. |
TabError | Raised when the code contains inconsistent use of tabs and spaces. | Convert all tabs to spaces or vice versa. |
By adhering to these guidelines and understanding the importance of indentation, you will be able to write clean, effective Python code that adheres to the language’s syntax rules.
Understanding Indentation in Python
In Python, indentation is not merely a matter of style; it is a critical aspect of the syntax. Unlike many other programming languages that use braces or keywords to define blocks of code, Python relies on indentation levels to determine the grouping of statements.
How Indentation Works
- Indent Level: Each block of code must be consistently indented. Indentation can consist of spaces or tabs, but it’s essential to avoid mixing them within the same project.
- Code Blocks: Indentation is used to define:
- Function definitions
- Class definitions
- Control structures (e.g., if statements, loops)
Example:
python
def my_function():
print(“Hello, World!”) # This line is indented, part of the function block.
Best Practices for Indentation
To maintain clarity and avoid errors, adhere to the following best practices:
- Use Spaces Over Tabs: The Python community recommends using 4 spaces per indentation level.
- Consistent Indentation: Ensure that the same number of spaces is used throughout the project.
- Avoid Mixing Tabs and Spaces: This can lead to unexpected behavior and `IndentationError`.
Common Indentation Errors
Indentation errors can arise from various mistakes:
Error Type | Description | Example Code |
---|---|---|
IndentationError | Occurs when blocks are not properly indented. | `if condition:` `print(“Error”)` (missing indentation) |
TabError | Occurs when mixing tabs and spaces. | `if condition:` `\tprint(“Error”)` (mixed usage) |
Tools for Managing Indentation
Several tools can help maintain proper indentation:
- Code Editors: Use IDEs (Integrated Development Environments) like PyCharm, VSCode, or Atom, which offer automatic indentation.
- Linters: Tools like `flake8` or `pylint` can help identify indentation issues in your code.
- Formatter Tools: Code formatters like `black` or `autopep8` can automatically adjust indentation to meet PEP 8 standards.
While this section does not provide a formal conclusion, understanding how to properly indent code in Python is vital for writing functional and readable programs. Indentation defines code structure and readability, making it an essential skill for developers. By adhering to established practices and utilizing the right tools, you can avoid common pitfalls associated with indentation in Python.
Understanding Indentation in Python: Perspectives from Experts
Dr. Emily Carter (Senior Software Engineer, Python Development Group). “Indentation in Python is not merely a matter of style; it is a fundamental aspect of the language’s syntax. Proper indentation is crucial for defining code blocks, which directly impacts the flow and execution of the program. Misalignment can lead to syntax errors or unintended behavior.”
James Liu (Lead Python Instructor, Code Academy). “For beginners, understanding how to indent in Python can be challenging. I always emphasize the importance of using consistent spaces or tabs throughout the code. Adopting a standard practice, such as using four spaces for each indentation level, can help avoid common pitfalls and improve code readability.”
Sarah Thompson (Technical Writer, Python Programming Journal). “In Python, indentation serves as a visual cue for the structure of the code. It is essential for defining loops, conditionals, and function definitions. Developers should familiarize themselves with the PEP 8 guidelines, which recommend using spaces over tabs to maintain consistency across different editors and platforms.”
Frequently Asked Questions (FAQs)
How do I indent code blocks in Python?
Indentation in Python is achieved by using spaces or tabs. Consistently use either spaces (typically four spaces per indentation level) or tabs throughout your code to define code blocks.
What happens if I mix tabs and spaces for indentation?
Mixing tabs and spaces can lead to `IndentationError` or unexpected behavior in your code. It is best practice to stick to one method of indentation throughout your script.
Can I use the `textwrap` module for indentation in Python?
Yes, the `textwrap` module can be used to format text and add indentation. It provides functions like `indent` to add specified whitespace to each line of a given text.
Is there a way to automatically convert tabs to spaces in my code editor?
Most modern code editors and IDEs have settings to convert tabs to spaces automatically. Check the preferences or settings menu to enable this feature.
What is the standard indentation size recommended in Python?
The standard indentation size recommended by PEP 8, the Python style guide, is four spaces per indentation level. This improves code readability and maintains consistency.
How can I check for inconsistent indentation in my Python code?
You can use linters like `flake8` or `pylint` to check for inconsistent indentation in your Python code. These tools analyze your code and highlight any indentation issues.
Indentation in Python is a fundamental aspect of the language’s syntax, serving as a means to define the structure and flow of the code. Unlike many other programming languages that utilize braces or keywords to delineate blocks of code, Python relies solely on whitespace. This unique feature requires programmers to be meticulous in their indentation practices, as improper indentation can lead to syntax errors or unexpected behavior in the code.
In Python, the standard practice is to use four spaces for each level of indentation. This convention not only enhances code readability but also ensures consistency across different codebases. While tabs can be used, mixing tabs and spaces is discouraged, as it can lead to confusion and errors. Developers are encouraged to configure their text editors or IDEs to automatically insert spaces when the tab key is pressed, thereby minimizing the risk of indentation-related issues.
Moreover, understanding the significance of indentation is crucial for control structures such as loops, conditionals, and function definitions. Each of these constructs relies on indentation to determine the scope of the code that belongs to them. Consequently, mastering indentation in Python is essential for writing clean, functional, and maintainable code. By adhering to best practices in indentation, developers can improve the overall quality of their programming and facilitate collaboration
Author Profile
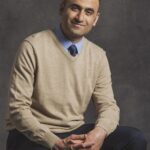
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?