How Do You Properly Indent Python Code for Optimal Readability?
How to Indent Python: Mastering the Art of Code Structure
In the world of programming, clarity and organization are paramount, and nowhere is this more evident than in Python. Unlike many other programming languages that rely on braces or keywords to define code blocks, Python uses indentation as a fundamental part of its syntax. This unique approach not only enhances the readability of the code but also enforces a disciplined structure that helps programmers understand the flow of their logic at a glance. Whether you are a seasoned developer or a novice just starting your coding journey, mastering the art of indentation in Python is essential for writing clean, efficient, and error-free code.
Indentation in Python serves as a visual cue that delineates different levels of code execution, such as loops, conditionals, and function definitions. By adhering to consistent indentation practices, developers can avoid common pitfalls, such as syntax errors and logical bugs, that may arise from misaligned code. Furthermore, Python’s strict requirement for indentation fosters a culture of best practices among programmers, encouraging them to write code that is not only functional but also aesthetically pleasing. As we delve deeper into the intricacies of Python indentation, we will explore the various conventions, tools, and techniques that can help you master this vital aspect of coding.
Understanding how
Understanding Indentation in Python
In Python, indentation is not merely a matter of style; it is a fundamental aspect of the syntax. Unlike many programming languages that use braces or keywords to delimit code blocks, Python uses indentation to define the scope of loops, functions, and conditional statements. This means that consistent indentation is crucial for both code readability and functionality.
When writing Python code, the standard practice is to use four spaces per indentation level. Although tabs can also be used, they should be avoided in favor of spaces to maintain consistency and prevent errors. Mixing tabs and spaces can lead to issues that are often difficult to diagnose.
Indentation Rules
Here are the key rules regarding indentation in Python:
- Consistent Indentation: All statements within the same block must be indented the same amount.
- Block Definition: An indented block begins after a colon (`:`) and is terminated when the indentation level decreases.
- Error Handling: An `IndentationError` occurs if the code is not properly indented, indicating that Python cannot determine the structure of the code.
For example, the following code illustrates correct indentation:
“`python
def example_function():
if True:
print(“This is indented correctly”)
else:
print(“This will not execute”)
“`
Best Practices for Indentation
To maintain high-quality code, adhere to these best practices when indenting Python code:
- Use Spaces, Not Tabs: Stick to spaces for indentation to avoid compatibility issues.
- Configure Your Editor: Most code editors allow you to configure the number of spaces per indentation level. Set it to four spaces, which is the Python community standard.
- Visual Alignment: Ensure that your indentation visually aligns to make the code easier to read.
Common Indentation Errors
Indentation errors can lead to unexpected behavior in your code. Here are some common mistakes:
Error Type | Description | Example |
---|---|---|
IndentationError | Occurs when the levels of indentation are inconsistent | “`if True: print(“Hello”)“` |
TabError | Happens when tabs and spaces are mixed | “`if True:\n\tprint(“Hello”)“` |
Unexpected Indent | When an unexpected indent occurs | “`if True:\n print(“Hello”)“` |
Tools for Managing Indentation
There are several tools and features available to help manage indentation in Python:
- Linters: Tools like `flake8` and `pylint` can analyze your code for indentation issues.
- Formatters: Code formatters such as `black` automatically correct indentation and other formatting issues.
- Integrated Development Environments (IDEs): Many IDEs provide visual cues for indentation levels and can automatically adjust indentation.
By following these guidelines and utilizing the appropriate tools, you can effectively manage indentation in your Python code, leading to improved readability and reduced errors.
Understanding Indentation in Python
In Python, indentation is not merely a matter of style; it is a fundamental aspect of the language’s syntax. Indentation is used to define the structure of code blocks such as loops, functions, and conditionals. Unlike many programming languages that use braces or keywords to denote blocks of code, Python relies solely on whitespace.
Rules of Indentation
To maintain the correct structure in Python, adhere to the following rules:
- Consistent Indentation: Use either spaces or tabs for indentation, but not both. Mixing them can lead to errors.
- Standard Practice: The Python community widely accepts four spaces as the standard for each indentation level. This convention enhances code readability.
- Code Blocks: Each new block of code must be indented consistently. For example, all lines within a function definition must have the same indentation level.
- Continuation Lines: When a statement spans multiple lines, the continuation lines should be indented to show they belong to the previous line.
Using Spaces vs. Tabs
While both spaces and tabs can be used for indentation, best practices recommend using spaces due to the following reasons:
Aspect | Spaces | Tabs |
---|---|---|
Compatibility | Universally supported | May render differently in various editors |
Readability | More consistent appearance | Can vary in width across editors |
PEP 8 Compliance | Recommended by PEP 8 style guide | Not recommended by PEP 8 |
Indentation Levels in Control Structures
When writing control structures, such as `if` statements or loops, proper indentation is essential. Here’s how to format them:
“`python
if condition:
Indented block for true condition
statement_1
statement_2
else:
Indented block for condition
statement_3
for i in range(5):
Indented block for loop
print(i)
“`
In the above examples, the lines following the `if` and `for` statements are indented to indicate they are part of the respective control structures.
Common Indentation Errors
When writing Python code, several common indentation errors can occur:
- Unindent does not match any outer indentation level: This error occurs when the indentation level is inconsistent. Ensure all blocks use the same number of spaces or tabs.
- IndentationError: expected an indented block: This arises when a block that requires indentation is not indented. For example, after defining a function, the body must be indented.
- TabError: inconsistent use of tabs and spaces: This error indicates that a mix of tabs and spaces has been used. Stick to one method throughout your code.
Tools and Editors for Managing Indentation
Many code editors provide features that help manage indentation effectively:
- Automatic Indentation: Most modern IDEs like PyCharm, VSCode, and Jupyter Notebook automatically manage indentation.
- Linting Tools: Tools like `flake8` and `pylint` can detect indentation issues and help enforce coding standards.
- Editor Settings: Configure your text editor to insert spaces when you press the Tab key, ensuring consistent indentation.
Proper indentation is crucial for writing clear and error-free Python code. Following these guidelines will enhance not only the functionality of your code but also its readability and maintainability.
Expert Insights on Indentation in Python Programming
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Indentation in Python is not just a matter of style; it is a fundamental part of the language’s syntax. Proper indentation ensures that the code is not only readable but also functional, as it defines the scope of loops, functions, and conditionals.”
Mark Thompson (Lead Python Developer, CodeCraft Solutions). “Many beginners overlook the importance of consistent indentation in Python. Utilizing spaces instead of tabs, or vice versa, can lead to syntax errors that are often hard to debug. I recommend sticking to four spaces per indentation level, as this is the widely accepted convention.”
Lisa Chen (Computer Science Educator, Future Coders Academy). “Teaching students how to properly indent Python code is crucial. I emphasize the use of IDEs that automatically handle indentation, which helps learners focus on logic and structure without getting bogged down by formatting issues.”
Frequently Asked Questions (FAQs)
How do I indent code in Python?
Indentation in Python is achieved by using spaces or tabs. The standard practice is to use four spaces per indentation level. You can set your text editor to insert spaces when you press the Tab key for consistency.
What happens if I don’t indent my Python code properly?
Improper indentation will lead to IndentationError or unexpected behavior in your code. Python uses indentation to define the structure and flow of the program, such as loops and function definitions.
Can I mix tabs and spaces for indentation in Python?
Mixing tabs and spaces is not recommended as it can lead to errors and inconsistencies. Python 3 disallows mixing the two, and it is best to stick to one method throughout your code.
How can I configure my text editor for Python indentation?
Most text editors, including Visual Studio Code, PyCharm, and Sublime Text, allow you to configure indentation settings. Look for options to set the tab key to insert spaces and define the number of spaces per indentation level.
Is there a way to automatically fix indentation in Python code?
Yes, tools like `autopep8` and `black` can automatically format your Python code, including fixing indentation issues. These tools help ensure your code adheres to PEP 8 style guidelines.
What is the impact of indentation on Python functions and loops?
Indentation defines the scope of functions and loops in Python. Proper indentation ensures that the code within these blocks executes as intended, while incorrect indentation can lead to logic errors or unintended behavior.
Indentation in Python is a fundamental aspect of the language’s syntax that dictates the structure and flow of code. Unlike many other programming languages that use braces or keywords to define blocks of code, Python relies on whitespace indentation. This means that proper indentation is not only a matter of style but is essential for the correct execution of the program. Each block of code, such as those following control statements like loops and conditionals, must be indented consistently to avoid syntax errors.
To achieve proper indentation in Python, developers typically use four spaces per indentation level, as per the widely accepted PEP 8 style guide. It is crucial to avoid mixing tabs and spaces, as this can lead to confusion and errors. Most modern code editors and IDEs provide features to automatically handle indentation, making it easier for programmers to maintain consistency throughout their code.
In summary, understanding and applying correct indentation in Python is vital for writing effective and error-free code. By adhering to established conventions and utilizing the tools available in development environments, programmers can enhance the readability and maintainability of their code. This attention to detail not only improves individual projects but also fosters better collaboration within teams, as consistent indentation practices lead to clearer communication among developers.
Author Profile
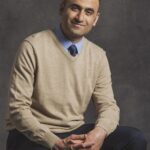
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?