How Do You Properly Initialize a Variable in Python?
In the world of programming, variables are the building blocks that allow us to store and manipulate data. Whether you’re a seasoned developer or just embarking on your coding journey, understanding how to initialize a variable in Python is a fundamental skill that paves the way for more complex programming concepts. Python, known for its simplicity and readability, offers a straightforward approach to variable initialization that can enhance your coding efficiency and effectiveness.
At its core, initializing a variable in Python involves assigning a value to a name, which serves as a reference to that value throughout your code. This process is not only essential for storing data but also for ensuring that your programs can perform calculations, manage user input, and handle various data types seamlessly. Python’s dynamic typing system allows you to create variables without explicitly declaring their type, making it an accessible language for beginners while still providing the flexibility that experienced developers appreciate.
As we delve deeper into this topic, we’ll explore the various methods and best practices for initializing variables in Python. From understanding the nuances of different data types to leveraging Python’s unique features, this guide will equip you with the knowledge needed to effectively manage your variables and enhance your programming prowess. Whether you’re looking to refine your skills or start from scratch, mastering variable initialization is a crucial step on your path to becoming
Variable Initialization in Python
In Python, initializing a variable involves assigning a value to it at the moment of creation. This can be done in several straightforward ways depending on the data type of the variable. Python is dynamically typed, which means you do not need to declare the variable type explicitly; Python infers it from the value assigned.
To initialize a variable, simply use the assignment operator `=`. Here are some examples:
“`python
x = 5 Integer
name = “Alice” String
pi = 3.14 Float
is_valid = True Boolean
“`
Variables can also be initialized with complex data types such as lists, dictionaries, or sets. For example:
“`python
fruits = [“apple”, “banana”, “cherry”] List
person = {“name”: “Alice”, “age”: 30} Dictionary
unique_numbers = {1, 2, 3, 4, 5} Set
“`
Multiple Variable Initialization
Python allows for the simultaneous initialization of multiple variables in a single line. This can enhance code readability and conciseness. Here are a few methods to achieve this:
- Comma-separated Assignment: You can assign values to multiple variables in one line.
“`python
a, b, c = 1, 2, 3
“`
- Single Value Assignment: Assign the same value to multiple variables.
“`python
x = y = z = 0
“`
Example Table of Variable Initialization
The following table illustrates various variable initializations with their respective data types:
Variable Name | Initialization Value | Data Type |
---|---|---|
x | 5 | Integer |
name | “Alice” | String |
pi | 3.14 | Float |
is_valid | True | Boolean |
fruits | [“apple”, “banana”] | List |
person | {“name”: “Alice”, “age”: 30} | Dictionary |
Dynamic Typing and Reassignment
One of the distinctive features of Python is its dynamic typing. This means that once a variable is initialized, you can change its value to any other data type without requiring a specific declaration. For instance:
“`python
x = 5 Initially an integer
x = “Hello” Now a string
x = [1, 2, 3] Now a list
“`
This flexibility allows developers to write code that is both efficient and easy to maintain. However, it can also lead to potential issues if the type of a variable changes unexpectedly during execution. Hence, it is crucial to manage variable types carefully to avoid runtime errors.
In summary, initializing variables in Python is a straightforward process that offers flexibility and ease of use, making it a powerful feature for developers across various applications.
Understanding Variable Initialization
In Python, initializing a variable is the process of assigning a value to a variable for the first time. This can be accomplished with a straightforward syntax, enabling efficient data manipulation throughout your code.
Basic Syntax for Variable Initialization
To initialize a variable in Python, you use the assignment operator (`=`). The syntax is as follows:
“`python
variable_name = value
“`
Here, `variable_name` is the identifier you choose, and `value` can be of any data type, including integers, floats, strings, lists, dictionaries, etc.
Examples of Variable Initialization
- Integer Initialization:
“`python
age = 30
“`
- Float Initialization:
“`python
price = 19.99
“`
- String Initialization:
“`python
name = “Alice”
“`
- List Initialization:
“`python
fruits = [“apple”, “banana”, “cherry”]
“`
- Dictionary Initialization:
“`python
student = {“name”: “John”, “age”: 22}
“`
Multiple Variable Initialization
Python allows the initialization of multiple variables in a single line. This can be done using commas to separate the variables and their corresponding values.
“`python
x, y, z = 1, 2, 3
“`
Variable Initialization with Data Types
Python is a dynamically typed language, meaning that you do not need to explicitly declare the data type of a variable during initialization. The interpreter infers the type based on the assigned value.
Variable Name | Value | Data Type |
---|---|---|
age | 25 | int |
height | 5.8 | float |
name | “Bob” | str |
is_student | True | bool |
Reassigning Values
Variables in Python can be reassigned at any point, allowing for flexibility. For example:
“`python
count = 10 Initial assignment
count = 20 Reassignment
“`
Common Practices for Variable Naming
When initializing variables, follow these best practices for naming:
- Use descriptive names (e.g., `total_price` instead of `tp`).
- Use lowercase letters with underscores for multi-word variables (e.g., `user_age`).
- Avoid starting variable names with numbers.
- Do not use special characters or spaces.
Variable initialization is a foundational aspect of programming in Python, allowing for effective data handling and manipulation. Understanding the syntax and best practices is essential for writing clean, efficient code.
Expert Insights on Initializing Variables in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “In Python, initializing a variable is straightforward. You simply assign a value to a variable name using the equals sign. For example, ‘x = 10’ initializes the variable x with the integer value 10. This flexibility allows developers to easily manage data types without explicit declarations.”
Michael Chen (Lead Python Developer, CodeMasters). “When initializing variables in Python, it is crucial to choose meaningful variable names that reflect their purpose. For instance, instead of using ‘a’ or ‘b’, using ‘user_age’ or ‘total_price’ enhances code readability and maintainability, especially in larger projects.”
Jessica Patel (Data Scientist, Analytics Hub). “Understanding variable scope is essential when initializing variables in Python. Variables initialized within a function are local to that function, while those initialized outside are global. This distinction is vital for managing data flow and avoiding potential conflicts in larger codebases.”
Frequently Asked Questions (FAQs)
How do I initialize a variable in Python?
To initialize a variable in Python, simply assign a value to it using the equals sign (`=`). For example, `x = 5` initializes the variable `x` with the integer value 5.
Can I initialize multiple variables in one line?
Yes, you can initialize multiple variables in one line by separating them with commas. For example, `a, b, c = 1, 2, 3` initializes `a` with 1, `b` with 2, and `c` with 3.
What types of values can I assign to a variable?
You can assign various types of values to a variable, including integers, floats, strings, lists, tuples, dictionaries, and more. For example, `name = “Alice”` assigns a string to the variable `name`.
Is it necessary to declare a variable’s type in Python?
No, Python is dynamically typed, which means you do not need to declare a variable’s type explicitly. The type is inferred from the value assigned to the variable.
What happens if I try to use a variable before initializing it?
If you attempt to use a variable before initializing it, Python will raise a `NameError`, indicating that the variable is not defined.
Can I change the value of a variable after initialization?
Yes, you can change the value of a variable at any time by reassigning it. For example, `x = 5` can later be changed to `x = 10`.
Initializing a variable in Python is a fundamental concept that is essential for effective programming. In Python, variables are created when a value is assigned to them, using the assignment operator `=`. This straightforward approach allows for the easy storage and manipulation of data, as Python is dynamically typed, meaning that the type of a variable is determined at runtime rather than in advance.
It is important to note that variable names in Python must adhere to specific naming conventions. They should begin with a letter or an underscore, followed by letters, numbers, or underscores. Additionally, Python is case-sensitive, which means that `Variable` and `variable` would be considered two distinct identifiers. Following these conventions ensures code readability and reduces the likelihood of errors.
Moreover, Python supports multiple variable assignments in a single line, which can enhance code efficiency. For example, one can initialize multiple variables simultaneously using a single statement, such as `a, b, c = 1, 2, 3`. This feature is particularly useful for simplifying code and improving clarity, especially when dealing with related data.
understanding how to initialize variables in Python is crucial for anyone looking to develop their programming skills. By adhering to naming conventions and leveraging
Author Profile
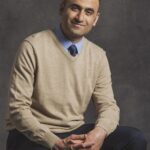
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?