How Can You Easily Input a List in Python?
In the world of programming, Python stands out as a versatile and user-friendly language, making it a favorite among beginners and seasoned developers alike. One of the fundamental concepts that every Python programmer must grasp is the ability to work with lists. Lists are powerful data structures that allow you to store and manage collections of items efficiently. Whether you’re organizing data, processing user inputs, or manipulating information, knowing how to input a list in Python is crucial for effective coding.
Understanding how to input a list in Python opens up a myriad of possibilities for data handling and manipulation. From collecting user inputs to reading data from files, the methods for creating and populating lists can vary widely. Each approach offers unique advantages, whether you’re looking for simplicity, flexibility, or performance. As you delve deeper into the world of Python, mastering list input techniques will enhance your ability to write dynamic and responsive programs.
As we explore the various ways to input a list in Python, you’ll discover the different methods available, each suited for specific scenarios. From using built-in functions to leveraging libraries that streamline the process, this article will guide you through the essentials of list input. By the end, you’ll be equipped with the knowledge to confidently create and manipulate lists, paving the way for more complex programming challenges ahead.
Using Lists in Python
In Python, a list is a versatile data structure that can hold a collection of items. Lists can contain elements of different data types, including integers, strings, and even other lists. There are various methods to input a list depending on the context, such as predefined values, user input, or reading from a file.
Defining a List with Predefined Values
To create a list with predefined values, you can use square brackets. Here’s how you can initialize a list:
“`python
my_list = [1, 2, 3, 4, 5]
“`
You can also create a list with different data types:
“`python
mixed_list = [1, “two”, 3.0, True]
“`
Inputting a List from User Input
To input a list directly from the user, you can utilize the `input()` function. Since `input()` returns a string, you will often need to split the string into individual elements and convert them to the desired data type.
Here’s an example of how to input a list of integers:
“`python
user_input = input(“Enter numbers separated by spaces: “)
number_list = [int(num) for num in user_input.split()]
“`
This method effectively takes a space-separated string of numbers, splits it into individual components, and converts each component into an integer.
Reading a List from a File
Another common method to input a list is by reading from a file. This is particularly useful for larger datasets. You can read lines from a file and store them in a list. Here’s an example:
“`python
with open(‘data.txt’, ‘r’) as file:
file_list = [line.strip() for line in file]
“`
This code opens a file named `data.txt`, reads each line, removes any trailing whitespace, and stores the result in a list.
Summary of List Input Methods
Method | Description | Example Code |
---|---|---|
Predefined Values | Directly assign values to a list | `my_list = [1, 2, 3]` |
User Input | Collect input from the user and convert to a list | `number_list = [int(num) for num in input().split()]` |
File Input | Read data from a file and store it in a list | `file_list = [line.strip() for line in open(‘file.txt’)]` |
By utilizing these methods, you can effectively input lists in Python for various applications, enhancing your data handling capabilities.
Creating a List in Python
In Python, a list is a versatile data structure that can hold a collection of items. Lists are defined by enclosing elements in square brackets `[]` and separating them with commas.
“`python
my_list = [1, 2, 3, 4, 5]
“`
You can also create a list that contains different data types:
“`python
mixed_list = [1, “hello”, 3.14, True]
“`
Inputting a List from User Input
To input a list from user input, you can utilize the `input()` function. However, the input will initially be a string, and you may need to convert it into a list format. Here are two common methods to achieve this:
- Method 1: Using `split()` Method
This method allows users to enter elements separated by spaces or commas.
“`python
user_input = input(“Enter numbers separated by space: “)
number_list = user_input.split() Creates a list of strings
Convert to integers if necessary
number_list = [int(num) for num in number_list]
“`
- Method 2: Using `eval()` for Complex Inputs
This method can evaluate a string representation of a list directly, but it should be used cautiously due to security concerns.
“`python
user_input = input(“Enter a list (e.g., [1, 2, 3]): “)
number_list = eval(user_input) Directly evaluates the input string
“`
Accepting Comma-Separated Values
For a more structured approach, especially when dealing with comma-separated values, you can modify the input method accordingly:
“`python
user_input = input(“Enter numbers separated by commas: “)
number_list = user_input.split(“,”) Splits the input string by commas
number_list = [int(num.strip()) for num in number_list] Converts to integers after stripping whitespace
“`
Using List Comprehension for Input Transformation
List comprehension provides a concise way to create lists based on existing lists. When inputting values, you can combine the `input()` and transformation in a single line:
“`python
number_list = [int(num) for num in input(“Enter numbers separated by space: “).split()]
“`
Handling Exceptions During Input
It is essential to handle potential exceptions when converting user input to a list. Using a try-except block can help manage errors gracefully:
“`python
try:
user_input = input(“Enter numbers separated by space: “)
number_list = [int(num) for num in user_input.split()]
except ValueError:
print(“Please enter valid integers.”)
“`
Displaying the List
To visualize the list after input, simply use the `print()` function:
“`python
print(“The list you entered is:”, number_list)
“`
This approach ensures that users can create and manipulate lists dynamically, providing a robust way to collect and process data in Python.
Expert Insights on Inputting Lists in Python
Dr. Emily Chen (Senior Software Engineer, Tech Innovations Inc.). “When inputting a list in Python, it is essential to understand the various methods available, such as using the `input()` function combined with `split()` to gather user input efficiently. This approach allows for dynamic list creation based on user-defined values.”
Mark Thompson (Python Developer Advocate, CodeCraft). “Utilizing list comprehensions can significantly enhance the way lists are created from user input. This method not only makes the code more readable but also optimizes performance by reducing the number of lines needed to achieve the same result.”
Sarah Patel (Data Scientist, Analytics Hub). “For data-driven applications, it is crucial to validate and sanitize user input before converting it into a list. Implementing error handling and type checks ensures that the input data is clean and usable, which is vital for maintaining data integrity in your Python applications.”
Frequently Asked Questions (FAQs)
How do I create a list in Python?
To create a list in Python, use square brackets `[]` and separate the elements with commas. For example, `my_list = [1, 2, 3, ‘four’]` defines a list containing integers and a string.
What are the different ways to input a list from the user in Python?
You can input a list from the user using the `input()` function, often combined with `split()` to separate values. For example, `user_input = input(“Enter numbers separated by spaces: “)` followed by `my_list = user_input.split()` creates a list of strings.
How can I convert a list of strings to a list of integers in Python?
To convert a list of strings to integers, use a list comprehension. For example, `my_list = [int(x) for x in string_list]` will convert each string in `string_list` to an integer.
Can I input a list of different data types in Python?
Yes, Python lists can contain elements of different data types. You can mix integers, floats, strings, and even other lists within a single list, such as `mixed_list = [1, 2.5, ‘hello’, [3, 4]]`.
How do I handle empty inputs when creating a list in Python?
You can check for empty inputs by using an `if` statement after receiving input. For example, if `user_input = input(“Enter values: “)`, you can check `if user_input: my_list = user_input.split()` to ensure the list is only created if the input is not empty.
What is the best practice for input validation when creating a list in Python?
Best practices for input validation include using try-except blocks to handle exceptions, checking data types, and ensuring that the input meets expected criteria. For example, you can validate that all elements can be converted to integers before adding them to the list.
In Python, inputting a list can be accomplished through various methods, depending on the context and requirements of the task. One common approach is to use the built-in `input()` function, which allows users to enter a string that can then be processed into a list. For example, by prompting the user to input values separated by commas, one can split the string and convert it into a list using the `split()` method. This method is straightforward and effective for obtaining user input in a controlled format.
Another method for inputting a list is by utilizing list comprehensions or loops to gather data programmatically. This approach is particularly useful when the data is being generated or retrieved from a source such as a file, database, or an API. By iterating over a range or a collection, you can append items to a list dynamically, allowing for greater flexibility in how the list is constructed.
Additionally, when working with predefined data, you can directly assign values to a list using square brackets. This method is efficient for initializing lists with known elements without requiring user interaction. Understanding these various techniques for inputting lists in Python is essential for effective programming and data manipulation.
In summary, Python offers multiple ways to input
Author Profile
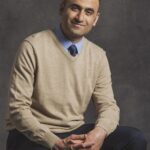
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?