How Can You Insert a Rule to Make Code Stop in Python?
In the world of programming, control is key. As developers, we often find ourselves in situations where we need to manage the flow of our code, ensuring it behaves as expected under various conditions. One common requirement is the ability to stop or interrupt code execution based on specific rules or criteria. Whether you’re debugging a complex application or implementing a feature that requires conditional execution, knowing how to insert rules that halt your Python code can save you time and headaches. This article will guide you through the essential techniques for achieving this, empowering you to write more efficient and responsive code.
Understanding how to effectively control the execution of your Python programs is crucial for both novice and experienced developers. Python offers a variety of mechanisms to insert rules that can terminate code execution, ranging from simple conditional statements to more advanced exception handling techniques. By mastering these tools, you can create robust applications that respond dynamically to user input, data conditions, or even external events, ensuring that your code runs smoothly and predictably.
As we delve deeper into this topic, you’ll discover practical examples and best practices for implementing these rules in your Python projects. From using `if` statements to manage flow control to leveraging exceptions for unexpected situations, the insights shared here will enhance your programming toolkit. Prepare to elevate your coding skills and learn how to
Understanding Rule-Based Control in Python
In Python, implementing rule-based control to stop code execution can be achieved through various methods. These methods allow developers to enforce conditions under which the code should terminate or pause. Below are the key techniques for inserting rules into your Python code.
Using Conditional Statements
Conditional statements such as `if`, `elif`, and `else` can be used to evaluate specific criteria and determine whether the code should proceed or halt. This is a fundamental approach to implementing control flow in Python.
Example:
python
def process_data(data):
if data is None:
print(“No data provided, stopping execution.”)
return # Stops the function execution
# Continue processing data
Utilizing Exceptions
Python’s exception handling mechanism can also serve as a means to stop code execution based on certain rules. By raising exceptions, you can interrupt the normal flow of the program when a specific condition is met.
Example:
python
def divide_numbers(a, b):
if b == 0:
raise ValueError(“Denominator cannot be zero. Stopping execution.”)
return a / b
Implementing Break Statements
The `break` statement can be utilized within loops to stop execution when a certain rule is triggered. This is particularly useful in iterative processes where you want to exit based on a condition.
Example:
python
for number in range(10):
if number == 5:
print(“Reached the stopping condition.”)
break # Exits the loop
Using Flags for Control Flow
Flags can be employed to monitor conditions throughout your code. A flag is a variable that indicates whether a certain condition has been met, allowing for controlled stopping points.
Example:
python
stop_execution =
while not stop_execution:
user_input = input(“Enter a command (type ‘stop’ to end): “)
if user_input == ‘stop’:
stop_execution = True # Changes the flag to stop the loop
Defining Custom Functions for Stopping Execution
Creating custom functions to handle stopping conditions can enhance code readability and maintainability. This encapsulation allows for easy adjustments to stopping criteria.
Example:
python
def should_stop(condition):
if condition:
print(“Stopping execution due to condition.”)
return True
return
# Usage
if should_stop(data_is_invalid):
return # Stops further execution
Summary of Control Mechanisms
The following table summarizes the various methods to control and stop execution in Python:
Method | Description |
---|---|
Conditional Statements | Evaluate conditions to determine if execution should halt. |
Exceptions | Raise errors to interrupt the flow based on specific rules. |
Break Statements | Exit loops when a certain condition is met. |
Flags | Use boolean variables to monitor conditions throughout the code. |
Custom Functions | Encapsulate stopping logic for improved readability and maintainability. |
Inserting Breakpoints in Python Code
In Python, one effective way to control the flow of your code and stop execution is through the use of breakpoints. Breakpoints allow you to pause the execution of your program at a specific line, enabling you to inspect variables and the state of your application.
- Using the built-in `breakpoint()` function:
- Insert `breakpoint()` at the desired location in your code.
- This will invoke the Python debugger (pdb) when the code execution reaches that line.
Example:
python
def calculate_sum(a, b):
result = a + b
breakpoint() # Execution will pause here
return result
print(calculate_sum(5, 3))
- Using an Integrated Development Environment (IDE):
- Most IDEs, such as PyCharm or Visual Studio Code, provide graphical interfaces to set breakpoints.
- Simply click next to the line number in the editor to toggle a breakpoint.
Conditionally Stopping Execution
In some cases, you may want to stop execution based on specific conditions. This can be achieved using assertions or conditional statements.
- Using Assertions:
- Assertions are a way to test if a condition is true. If it is , the program will raise an AssertionError, effectively stopping execution.
Example:
python
def divide(a, b):
assert b != 0, “Denominator cannot be zero.”
return a / b
print(divide(10, 0)) # This will raise an AssertionError
- Using Conditional Statements:
- You can use `if` statements to check conditions and raise exceptions if necessary.
Example:
python
def process_data(data):
if not data:
raise ValueError(“Data cannot be empty.”)
# Continue processing
return data
process_data([]) # This will raise a ValueError
Using Exception Handling to Stop Code
Exception handling allows you to manage errors and can be used to gracefully stop code execution when encountering an unexpected situation.
- Using Try/Except Blocks:
- By wrapping code in a try block, you can catch exceptions and stop the execution as desired.
Example:
python
try:
result = 10 / 0
except ZeroDivisionError:
print(“Cannot divide by zero, stopping execution.”)
exit() # Exit the program
Utilizing the `sys.exit()` Method
For situations where you need to halt execution immediately, the `sys.exit()` method can be used.
- Importing the sys module:
- To use this method, import the `sys` module and call `sys.exit()` wherever you need to terminate the program.
Example:
python
import sys
def main():
print(“Processing…”)
if some_condition_is_met:
print(“Stopping execution.”)
sys.exit()
main()
Using the `os` Module to Terminate Processes
In scenarios where you need to stop a running process or script from the operating system level, the `os` module can be utilized.
- Using `os.kill()`:
- This method allows you to send a termination signal to a specific process.
Example:
python
import os
import sys
pid = os.getpid() # Get the current process ID
os.kill(pid, 9) # Send a SIGKILL signal to terminate the process
By employing these techniques, you can effectively manage how and when your Python code execution stops, catering to various debugging and operational requirements.
Strategies for Implementing Control Flow in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “To effectively insert a rule that makes your code stop in Python, consider using the built-in `break` statement within loops or the `raise` statement to trigger exceptions. This allows for controlled exits based on specific conditions, ensuring your program behaves predictably.”
Michael Chen (Python Developer and Author, CodeMaster Publications). “Implementing a stopping rule can be achieved through conditional statements. By utilizing `if` statements to check for certain conditions, you can use `sys.exit()` to terminate the program when those conditions are met, providing a clear and effective way to manage code execution.”
Sarah Patel (Lead Data Scientist, AI Solutions Group). “Inserting a rule to stop execution in Python can also be accomplished through the use of custom exceptions. By defining your own exception class and raising it at the appropriate point in your code, you can halt execution gracefully while providing informative error messages to the user.”
Frequently Asked Questions (FAQs)
How can I insert a rule to stop a loop in Python?
You can use the `break` statement to exit a loop when a specific condition is met. For example, within a `while` or `for` loop, you can check for a condition and call `break` to terminate the loop.
What is the purpose of the `return` statement in Python?
The `return` statement is used to exit a function and optionally pass an expression back to the caller. It effectively stops the execution of the function and can be used to provide output based on certain conditions.
How do I implement a timeout to stop a process in Python?
You can use the `signal` module to set an alarm signal that interrupts the execution of a process after a specified time. Alternatively, you can use threading or multiprocessing to manage timeouts more effectively.
Can I use exceptions to stop code execution in Python?
Yes, you can raise an exception using the `raise` statement to stop execution. When an exception is raised, it can be caught by a `try-except` block, allowing you to handle the error gracefully or terminate the program.
What is the role of the `exit()` function in Python?
The `exit()` function from the `sys` module is used to terminate a Python program. It can be called with an optional exit status code, where `0` indicates a successful termination and any non-zero value indicates an error.
How do I stop a script based on user input in Python?
You can use the `input()` function to prompt the user for input. If the input matches a specific condition (e.g., “exit”), you can call the `break` statement or `exit()` to stop the script.
Inserting rules to control the execution of code in Python can be accomplished through various methods, each serving specific purposes. Developers often utilize conditional statements, exception handling, and control flow mechanisms to dictate when and how code should stop executing. By implementing these strategies, programmers can create more robust and error-resistant applications that respond appropriately to different scenarios.
One effective approach is to use conditional statements such as `if`, `elif`, and `else` to evaluate specific conditions. These statements allow the code to make decisions based on the input or the state of the program, thereby controlling the flow of execution. Additionally, the `break` statement can be employed within loops to terminate the loop when a certain condition is met, effectively stopping the code from continuing its execution in that context.
Another critical aspect is exception handling, which can be achieved using `try`, `except`, and `finally` blocks. This mechanism enables developers to gracefully handle errors and unexpected situations, allowing the code to stop executing when an exception occurs. By anticipating potential issues and providing fallback solutions, programmers can enhance the reliability of their applications.
In summary, effectively managing code execution in Python involves a combination of conditional logic, loop control, and exception handling. By
Author Profile
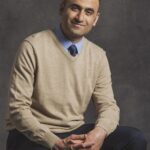
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementHow Can You View ConfigMaps in Kubernetes?
- April 13, 2025Kubernetes ManagementWhen Should You Consider Using Kubernetes for Your Applications?
- April 13, 2025Kubernetes ManagementWhat Is CNI in Kubernetes and Why Is It Important?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?