How Can You Easily Install dotenv in Python?
In the world of software development, managing configuration settings can often feel like navigating a labyrinth. With the increasing complexity of applications, especially those that interact with various environments, the need for a streamlined approach to handle sensitive information such as API keys, database credentials, and other environment variables has never been more crucial. Enter `dotenv`, a powerful tool that simplifies this process for Python developers. By allowing you to store these configurations in a `.env` file, `dotenv` not only enhances security but also promotes cleaner code and easier deployment.
Installing `dotenv` in your Python environment is a straightforward process that opens the door to a more organized way of managing your application’s settings. This library leverages the simplicity of key-value pairs in a text file, making it accessible for developers of all skill levels. Whether you are working on a small personal project or a large-scale application, understanding how to implement `dotenv` can significantly improve your workflow and reduce the risk of exposing sensitive information.
As we delve deeper into the installation process and configuration of `dotenv`, you’ll discover the various benefits it offers, including improved collaboration among team members and seamless transitions between development, testing, and production environments. Get ready to transform the way you handle configurations in your Python projects, ensuring that your applications are not only functional
Installing Python Dotenv
To install the `dotenv` package in Python, you primarily use the package manager `pip`. This package allows you to manage environment variables conveniently by loading them from a `.env` file into your environment. Follow the steps below to install the `python-dotenv` package.
Steps to Install
- Open Your Terminal or Command Prompt: Depending on your operating system, access your terminal (Linux/Mac) or command prompt (Windows).
- Verify Python and pip Installation: Ensure that Python and pip are installed by running the following commands:
“`bash
python –version
pip –version
“`
- Install the dotenv Package: Execute the following command to install `python-dotenv`:
“`bash
pip install python-dotenv
“`
- Verify Installation: You can confirm that `python-dotenv` is installed successfully by checking the installed packages:
“`bash
pip list
“`
If you see `python-dotenv` in the list, the installation has been completed successfully.
Using Python Dotenv
Once installed, you can start using `python-dotenv` to load environment variables from a `.env` file. Here’s how to create a basic `.env` file and load it within your Python script.
- Create a .env File: In your project directory, create a file named `.env` and define your environment variables in the following format:
“`
DATABASE_URL=postgres://user:password@localhost:5432/dbname
SECRET_KEY=mysecretkey
DEBUG=True
“`
- Load the .env File in Your Python Script:
“`python
from dotenv import load_dotenv
import os
Load environment variables from .env file
load_dotenv()
Access the variables
database_url = os.getenv(‘DATABASE_URL’)
secret_key = os.getenv(‘SECRET_KEY’)
debug_mode = os.getenv(‘DEBUG’)
“`
Common Issues and Troubleshooting
When working with `python-dotenv`, you may encounter some common issues. Below is a table summarizing these issues along with their solutions.
Issue | Solution |
---|---|
Environment variables not loading | Ensure you have called load_dotenv() before accessing any variables. |
.env file not found | Make sure the file is named exactly .env and located in the same directory as your script. |
Incorrect variable names | Check for typos in your .env file and ensure you’re using the correct variable names in your code. |
By following these steps and addressing common issues, you will effectively integrate and utilize the `python-dotenv` package in your Python projects.
Installing dotenv in Python
To utilize the `dotenv` package in Python, follow these detailed steps to ensure a smooth installation and integration process.
Prerequisites
Before installing `dotenv`, ensure you have the following:
- Python installed (version 3.6 or higher is recommended)
- A package manager, such as pip, which is included with Python installations
Installation Steps
The installation of `dotenv` can be accomplished through pip. Execute the following command in your terminal or command prompt:
“`bash
pip install python-dotenv
“`
This command downloads and installs the `python-dotenv` package from the Python Package Index (PyPI).
Verifying the Installation
After installation, it is crucial to verify that the package has been successfully installed. You can do this by running the following command in a Python shell:
“`python
import dotenv
print(dotenv.__version__)
“`
If no errors are raised and you see the version number printed, the installation was successful.
Creating a .env File
The `.env` file is where you will store your environment variables. Here’s how to create one:
- Navigate to your project directory.
- Create a new file named `.env`.
- Open the `.env` file and add your variables in the following format:
“`
VARIABLE_NAME=value
ANOTHER_VARIABLE=another_value
“`
Loading Environment Variables
To load the variables from the `.env` file into your Python application, you need to use the `load_dotenv` function. Below is a simple example:
“`python
from dotenv import load_dotenv
import os
Load the environment variables from .env file
load_dotenv()
Access the variables
variable_value = os.getenv(‘VARIABLE_NAME’)
print(variable_value)
“`
This code snippet imports the `load_dotenv` function, loads the variables, and retrieves the value of a specific variable.
Using Environment Variables in Your Application
You can utilize the loaded environment variables throughout your application as follows:
- Secure API keys
- Database connection strings
- Configuration settings
Consider the following example:
“`python
API_KEY = os.getenv(‘API_KEY’)
DATABASE_URL = os.getenv(‘DATABASE_URL’)
“`
This ensures that sensitive information is not hard-coded within your source files, enhancing security and flexibility.
Common Issues and Troubleshooting
If you encounter issues, consider the following:
Issue | Solution |
---|---|
ModuleNotFoundError | Ensure `python-dotenv` is installed correctly. |
Variables not loading | Check the file path and ensure the `.env` file exists. |
Incorrect variable access | Verify the variable names in the `.env` file match those in the code. |
By following these steps and recommendations, you can successfully install and utilize the `dotenv` package in your Python projects.
Expert Insights on Installing Dotenv in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Installing dotenv in Python is a straightforward process that significantly enhances your application’s configuration management. I recommend using pip, as it simplifies the installation and ensures you have the latest version.”
Michael Chen (Python Developer Advocate, Open Source Community). “To install dotenv, you should first ensure that your virtual environment is activated. This practice not only keeps your dependencies organized but also prevents conflicts between projects.”
Sarah Patel (Lead Backend Developer, CodeCraft Solutions). “After installation, remember to create a .env file in your project directory. This file is where you will securely store your environment variables, making your application more secure and easier to manage.”
Frequently Asked Questions (FAQs)
How do I install the dotenv package in Python?
To install the dotenv package, use the pip package manager. Run the command `pip install python-dotenv` in your terminal or command prompt.
What is the purpose of the dotenv package in Python?
The dotenv package is used to read key-value pairs from a `.env` file and set them as environment variables, which helps manage configuration settings in a secure and organized manner.
Can I use dotenv with any Python project?
Yes, dotenv can be used with any Python project that requires environment variable management, making it suitable for web applications, scripts, and more.
How do I create a .env file for my Python project?
To create a .env file, simply create a new text file named `.env` in your project directory. Add your environment variables in the format `KEY=VALUE`, one per line.
How do I load environment variables from the .env file in my Python code?
To load environment variables, import the `load_dotenv` function from the `dotenv` module and call it at the beginning of your script. Use `os.getenv(‘KEY’)` to access the variables.
Is it safe to store sensitive information in a .env file?
Yes, it is generally safe to store sensitive information in a .env file, provided that the file is not included in version control (e.g., by adding it to `.gitignore`) and is kept secure.
installing the `dotenv` package in Python is a straightforward process that enhances the management of environment variables within applications. This package allows developers to store sensitive information such as API keys and database credentials in a `.env` file, which can then be easily loaded into the application environment. The installation can typically be accomplished using package managers like `pip`, with the command `pip install python-dotenv` being the most common method.
Furthermore, once the package is installed, utilizing it within a Python script is equally simple. By importing the `load_dotenv` function from the `dotenv` module, developers can load the environment variables defined in the `.env` file at the start of their application. This practice not only promotes better security by keeping sensitive data out of the source code but also enhances code portability and maintainability.
Overall, the use of `dotenv` is a best practice in Python development, particularly for projects that require configuration management. By following the installation and usage guidelines, developers can ensure their applications are both secure and efficient, ultimately leading to a more robust software development process.
Author Profile
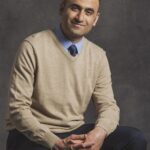
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?