How Can You Install Third-Party Libraries in Python?
In the ever-evolving landscape of programming, Python stands out as a versatile and powerful language, beloved by developers for its simplicity and rich ecosystem. One of the key strengths of Python lies in its extensive collection of third-party libraries, which can significantly enhance functionality and streamline development processes. Whether you’re delving into data science, web development, or automation, knowing how to install and manage these libraries is essential for harnessing Python’s full potential. This article will guide you through the fundamental steps to effortlessly integrate third-party libraries into your Python projects.
Installing third-party libraries in Python is a straightforward process, primarily facilitated by the package manager known as pip. With just a few commands, you can access a vast array of libraries that cater to various needs, from data manipulation with Pandas to machine learning with TensorFlow. Understanding how to leverage pip not only empowers you to add new features to your applications but also ensures that your development environment remains organized and up to date.
Moreover, as you explore the world of Python libraries, you’ll discover the importance of virtual environments. These isolated spaces allow you to manage dependencies for different projects without conflict, making it easier to maintain a clean and efficient workflow. As we delve deeper into the specifics of installation methods, best practices, and troubleshooting tips, you’ll be
Understanding Python Package Management
Python utilizes a package management system to facilitate the installation and management of third-party libraries. The most widely used package manager is `pip`, which allows users to install libraries from the Python Package Index (PyPI) and other repositories. Understanding how to effectively use `pip` is essential for incorporating third-party libraries into your projects.
Installing Libraries with pip
To install a third-party library using `pip`, you can execute a command in the terminal or command prompt. The basic syntax is as follows:
bash
pip install library_name
Replace `library_name` with the actual name of the library you wish to install. For example, to install the popular library `requests`, you would enter:
bash
pip install requests
Managing Dependencies
When installing libraries, it is crucial to manage dependencies effectively. Libraries often rely on other libraries to function correctly. To avoid conflicts and ensure that you have the required versions of each library, consider using a virtual environment. This allows you to create isolated environments for different projects.
To create and activate a virtual environment, follow these steps:
- Install the `virtualenv` package if you haven’t already:
bash
pip install virtualenv
- Create a new virtual environment:
bash
virtualenv myenv
- Activate the virtual environment:
- On Windows:
bash
myenv\Scripts\activate
- On macOS/Linux:
bash
source myenv/bin/activate
Using Requirements Files
A requirements file is a convenient way to manage your project’s dependencies. It allows you to specify all required libraries and their versions in a single file, making it easier to replicate the environment.
To create a requirements file, you can use the following command:
bash
pip freeze > requirements.txt
This will generate a `requirements.txt` file with all installed libraries and their respective versions. To install all libraries listed in a requirements file, use:
bash
pip install -r requirements.txt
Common pip Commands
Here is a summary of some common `pip` commands:
Command | Description |
---|---|
pip install library_name |
Installs the specified library. |
pip uninstall library_name |
Uninstalls the specified library. |
pip list |
Lists all installed packages. |
pip show library_name |
Displays information about the specified package. |
pip search query |
Searches for packages by name or keyword. |
By mastering these commands and utilizing virtual environments, you can streamline your workflow and effectively manage third-party libraries in your Python projects.
Understanding Python Package Managers
Python utilizes several package managers to facilitate the installation of third-party libraries. The most common are `pip` and `conda`. Each has its own advantages and is suited to different use cases.
- Pip: The default package manager for Python, ideal for installing packages from the Python Package Index (PyPI).
- Conda: A package manager from the Anaconda distribution, which is particularly useful for data science and scientific computing, handling dependencies and environments efficiently.
Installing Libraries with Pip
To install a library using `pip`, follow these steps:
- Open your command line interface (CLI): This could be Command Prompt on Windows, Terminal on macOS, or a shell on Linux.
- Type the installation command: Use the following syntax:
pip install package_name
Replace `package_name` with the actual name of the library you wish to install.
- Example: To install the `requests` library, you would enter:
pip install requests
Installing Libraries with Conda
If you are using Anaconda, you can install libraries with `conda`:
- Open Anaconda Prompt or your CLI.
- Use the install command:
conda install package_name
Again, replace `package_name` with the name of the library.
- Example: To install `numpy`, you would type:
conda install numpy
Specifying Versions
Sometimes, you may need to install a specific version of a library. Both `pip` and `conda` allow you to specify versions.
- Using Pip:
pip install package_name==version_number
Example:
pip install requests==2.25.1
- Using Conda:
conda install package_name=version_number
Example:
conda install numpy=1.19.2
Managing Dependencies
When installing libraries, managing dependencies is crucial to avoid conflicts. Both `pip` and `conda` handle this, but in different ways:
- Pip:
- Automatically resolves and installs dependencies.
- Use a `requirements.txt` file to specify multiple libraries and their versions:
pip install -r requirements.txt
- Conda:
- Offers a more robust environment management feature.
- Create an environment with:
conda create –name env_name package_name
- Activate the environment using:
conda activate env_name
Checking Installed Packages
To verify which packages are currently installed in your environment, you can use:
- For Pip:
pip list
- For Conda:
conda list
This will display all installed libraries along with their versions, allowing you to manage your environment effectively.
Uninstalling Libraries
If you need to remove a library, both package managers provide a straightforward method:
- Using Pip:
pip uninstall package_name
- Using Conda:
conda remove package_name
These commands will prompt for confirmation before proceeding with the uninstallation process.
Expert Insights on Installing Third-Party Libraries in Python
Dr. Emily Carter (Senior Software Engineer, Python Software Foundation). “To install third-party libraries in Python, utilizing the package manager pip is essential. It simplifies the process significantly, allowing developers to install libraries directly from the Python Package Index (PyPI) with a simple command: `pip install library_name`. This method ensures that you have the latest version and all dependencies are managed efficiently.”
Mark Thompson (Lead Developer, Open Source Initiative). “When installing third-party libraries, it is crucial to consider using a virtual environment. This practice isolates project dependencies and prevents version conflicts. Tools like `venv` or `conda` can help create these environments, ensuring that your main Python installation remains clean and unaffected by library changes.”
Lisa Chen (Data Scientist, Tech Innovations Inc.). “Documentation is key when integrating third-party libraries into your Python projects. Always refer to the library’s official documentation for installation instructions, as some libraries may require additional steps or dependencies. Additionally, keeping track of your installed libraries using a requirements.txt file can streamline future installations and deployments.”
Frequently Asked Questions (FAQs)
How can I install third-party libraries in Python?
You can install third-party libraries in Python using the package manager `pip`. Open your command line or terminal and run the command `pip install library_name`, replacing `library_name` with the name of the library you wish to install.
What is the difference between `pip` and `pip3`?
`pip` is typically used for Python 2.x installations, while `pip3` is specifically for Python 3.x. It is advisable to use `pip3` if you are working with Python 3 to ensure compatibility.
How do I install a specific version of a library?
To install a specific version of a library, use the command `pip install library_name==version_number`, replacing `library_name` with the library’s name and `version_number` with the desired version.
Can I install libraries from a requirements file?
Yes, you can install libraries listed in a requirements file by using the command `pip install -r requirements.txt`, where `requirements.txt` is the name of your file containing the list of libraries and their versions.
What should I do if I encounter permission errors during installation?
If you encounter permission errors, try running the installation command with elevated privileges by adding `sudo` before the command on Unix-based systems, or consider using a virtual environment to avoid permission issues.
How can I verify if a library has been installed successfully?
You can verify the installation of a library by running `pip show library_name`, which will display information about the library if it is installed. Alternatively, you can try importing the library in a Python shell to check for any import errors.
Installing third-party libraries in Python is a fundamental skill for developers looking to enhance their projects with additional functionality. The most common method for installation is through the Python Package Index (PyPI) using the package manager pip. This tool allows users to easily download and install libraries directly from the command line, streamlining the process significantly. Users can execute commands such as `pip install library_name` to retrieve the desired packages, ensuring that they have access to a vast array of resources to support their coding needs.
Additionally, it is essential to manage dependencies effectively, particularly when working on larger projects. Utilizing virtual environments, created with tools like `venv` or `conda`, can help isolate project-specific dependencies and prevent conflicts between libraries. This practice not only enhances project organization but also simplifies the management of different versions of libraries across various projects. Furthermore, understanding how to create and manage requirements files can facilitate the sharing and reproduction of environments, making collaboration with other developers more efficient.
mastering the installation of third-party libraries in Python is crucial for any developer aiming to leverage the extensive ecosystem of available tools. By utilizing pip, managing dependencies through virtual environments, and effectively using requirements files, developers can ensure that their projects are robust,
Author Profile
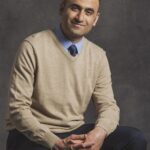
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?