How Can You Effectively Iterate Through a List in Python?
Introduction
In the world of programming, lists are one of the most versatile and widely used data structures, especially in Python. Whether you’re managing a collection of user inputs, processing data from a file, or simply organizing your thoughts, knowing how to iterate through a list is an essential skill for any aspiring developer. As you embark on your Python journey, mastering list iteration will not only enhance your coding efficiency but also open doors to more complex data manipulations. In this article, we will explore the various methods to iterate through lists in Python, empowering you to handle data with confidence and creativity.
When it comes to iterating through a list in Python, there are several techniques at your disposal, each with its unique advantages. From the classic `for` loop to more advanced methods like list comprehensions and the `enumerate` function, Python offers a rich toolkit for traversing lists. Understanding these different approaches will enable you to choose the best one for your specific use case, whether you’re looking for simplicity, readability, or performance.
Moreover, list iteration is not just about accessing elements; it’s also about applying operations, filtering data, and transforming lists into new formats. As you delve deeper into the nuances of iteration, you’ll discover how to leverage Python’s powerful features to streamline
Using For Loops
One of the most common methods to iterate through a list in Python is by using a `for` loop. This method allows you to traverse each element in the list sequentially, performing operations on each item as needed. The syntax is straightforward:
python
for item in list_name:
# Perform operations with item
Here’s a simple example:
python
fruits = [‘apple’, ‘banana’, ‘cherry’]
for fruit in fruits:
print(fruit)
This will output each fruit in the list.
Using While Loops
Another way to iterate through a list is by using a `while` loop. This method gives you more control over the iteration process, especially when you need to manipulate the index of the list:
python
index = 0
while index < len(list_name):
# Perform operations with list_name[index]
index += 1
Example:
python
fruits = ['apple', 'banana', 'cherry']
index = 0
while index < len(fruits):
print(fruits[index])
index += 1
This will achieve the same output as the `for` loop example.
Using List Comprehensions
List comprehensions provide a concise way to create lists and can also be used for iteration. They are especially useful for applying an operation to each element in a list and generating a new list based on that operation.
The syntax is:
python
new_list = [expression for item in list_name]
Example:
python
fruits = [‘apple’, ‘banana’, ‘cherry’]
upper_fruits = [fruit.upper() for fruit in fruits]
print(upper_fruits)
This will output: `[‘APPLE’, ‘BANANA’, ‘CHERRY’]`.
Using the Enumerate Function
When you need both the index and the value of items in a list, the `enumerate()` function is particularly useful. It adds a counter to the iterable, returning it in the form of an enumerate object. This can be especially helpful in scenarios where the index is required for further processing.
Example:
python
fruits = [‘apple’, ‘banana’, ‘cherry’]
for index, fruit in enumerate(fruits):
print(f”{index}: {fruit}”)
This will output:
0: apple
1: banana
2: cherry
Iterating Through Nested Lists
For lists containing other lists (nested lists), you can use nested loops to access each element. This is common in multi-dimensional data structures.
Example of a nested list:
python
nested_list = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
for sublist in nested_list:
for item in sublist:
print(item)
This will output each number from the nested list.
Comparison of Iteration Methods
The following table summarizes the different methods of iterating through a list in Python, along with their use cases:
Method | Use Case | Syntax |
---|---|---|
For Loop | Simple iteration over items | for item in list_name: |
While Loop | When index manipulation is needed | while index < len(list_name): |
List Comprehension | Creating new lists from existing ones | new_list = [expression for item in list_name] |
Enumerate | Accessing both index and value | for index, item in enumerate(list_name): |
Nested Loops | Iterating through nested lists | for sublist in nested_list: |
These methods provide flexibility in how you can handle iteration in your Python programs, allowing you to choose the most suitable approach for your specific needs.
Iterating Through a List Using a For Loop
A common method for iterating through a list in Python is using a for loop. This approach allows you to access each element in the list sequentially. The basic syntax is as follows:
python
for element in list_name:
# process the element
This loop will execute the block of code inside it for each element in the list.
Example:
python
fruits = [‘apple’, ‘banana’, ‘cherry’]
for fruit in fruits:
print(fruit)
Output:
apple
banana
cherry
Using List Comprehensions
List comprehensions provide a concise way to create lists while iterating through another list. This approach can be more efficient and readable, especially for simple transformations.
Syntax:
python
new_list = [expression for item in iterable if condition]
Example:
python
squared_numbers = [x**2 for x in range(10)]
print(squared_numbers)
Output:
[0, 1, 4, 9, 16, 25, 36, 49, 64, 81]
Iterating with Enumerate
The `enumerate()` function adds a counter to an iterable. It is particularly useful when you need both the index and the value of each item in the list.
Syntax:
python
for index, value in enumerate(list_name):
# process index and value
Example:
python
colors = [‘red’, ‘green’, ‘blue’]
for index, color in enumerate(colors):
print(f”Index {index}: {color}”)
Output:
Index 0: red
Index 1: green
Index 2: blue
Using While Loop
You can also iterate through a list using a while loop, though this is less common than for loops. Care must be taken to avoid infinite loops.
Example:
python
numbers = [1, 2, 3, 4]
i = 0
while i < len(numbers):
print(numbers[i])
i += 1
Output:
1
2
3
4
Iterating with List Slice
List slicing can also be utilized to iterate through portions of a list. It allows you to access a specific segment of the list easily.
Example:
python
letters = [‘a’, ‘b’, ‘c’, ‘d’, ‘e’]
for letter in letters[1:4]: # slicing from index 1 to 3
print(letter)
Output:
b
c
d
Using the Map Function
The `map()` function applies a given function to all items in an input list. This can be an efficient way to transform items in a list.
Syntax:
python
result = map(function, iterable)
Example:
python
def square(x):
return x * x
numbers = [1, 2, 3, 4]
squared = list(map(square, numbers))
print(squared)
Output:
[1, 4, 9, 16]
Iterating Through Nested Lists
When dealing with nested lists (lists within lists), you can use nested loops to access each element.
Example:
python
matrix = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
for row in matrix:
for item in row:
print(item)
Output:
1
2
3
4
5
6
7
8
9
Expert Insights on Iterating Through Lists in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “When iterating through a list in Python, it is essential to leverage the built-in `for` loop for its clarity and efficiency. This approach not only enhances code readability but also minimizes the risk of errors associated with index management.”
Michael Zhang (Data Scientist, Analytics Solutions Group). “Utilizing list comprehensions is a powerful technique for iterating through lists in Python. This method allows for concise and expressive code, especially when transforming or filtering elements within a list.”
Dr. Sarah Thompson (Python Educator, Code Academy). “For more complex iterations, such as when needing to access both the index and the value, the `enumerate()` function is invaluable. It provides a clean and Pythonic way to loop through lists while keeping track of the index.”
Frequently Asked Questions (FAQs)
How can I iterate through a list in Python?
You can iterate through a list in Python using a `for` loop. For example:
python
my_list = [1, 2, 3]
for item in my_list:
print(item)
What are the different ways to iterate through a list in Python?
You can iterate through a list using a `for` loop, a `while` loop, list comprehensions, or the `enumerate()` function for index-value pairs.
Can I modify a list while iterating through it in Python?
Modifying a list while iterating through it can lead to unexpected behavior. It is advisable to create a copy of the list or use list comprehensions for safe modifications.
What is the purpose of the `enumerate()` function in list iteration?
The `enumerate()` function allows you to iterate through a list while keeping track of the index of each item. This is useful when you need both the index and the value.
How do I iterate through a list of dictionaries in Python?
You can iterate through a list of dictionaries using a `for` loop. For example:
python
list_of_dicts = [{‘a’: 1}, {‘b’: 2}]
for d in list_of_dicts:
print(d)
Is there a way to iterate through a list in reverse order?
Yes, you can iterate through a list in reverse order using the `reversed()` function or by slicing the list with `my_list[::-1]`. For example:
python
for item in reversed(my_list):
print(item)
Iterating through a list in Python is a fundamental skill that enables developers to process and manipulate data effectively. Python provides several methods to achieve this, including the traditional for loop, list comprehensions, and the built-in functions such as map and filter. Each of these approaches offers unique advantages depending on the specific requirements of the task at hand.
The for loop is the most straightforward method, allowing for clear and readable code. It enables developers to access each element of the list in sequence, making it easy to perform operations such as printing, modifying, or aggregating values. List comprehensions, on the other hand, provide a concise way to create new lists by applying an expression to each item in the original list. This method is particularly useful for transforming data efficiently.
Moreover, using built-in functions like map and filter can enhance code readability and performance, especially when dealing with larger datasets. These functions allow for functional programming techniques, which can lead to more elegant solutions. Understanding when to use each method is crucial for writing efficient and maintainable code.
In summary, mastering the various techniques for iterating through lists in Python is essential for any programmer. Each method has its strengths and can be applied in different contexts to achieve
Author Profile
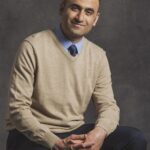
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?