How Can You Keep the Python Turtle Window Open for Longer?
If you’ve ever dabbled in Python programming, you might have encountered the delightful world of Turtle graphics. This unique library allows users to create stunning visualizations and animations with simple commands, making it a favorite among beginners and seasoned programmers alike. However, one common frustration that many face is how to keep the Python Turtle window open after executing their drawings. This seemingly simple task can often lead to confusion, especially for those new to the language. In this article, we will explore effective strategies to ensure that your Turtle graphics window remains open, allowing you to enjoy and showcase your creations without interruption.
When working with Turtle graphics, the default behavior of the window is to close immediately after the program finishes executing. This can be particularly disheartening after spending time crafting intricate designs or animations. Fortunately, there are several techniques and best practices that can help you maintain that window long enough to admire your handiwork. By understanding the underlying mechanics of the Turtle library and utilizing specific functions, you can easily control when your window closes.
In the following sections, we will delve into various methods to keep the Turtle window open, from using simple command prompts to implementing event listeners. Whether you’re creating a fun project for a class or just experimenting with code, these tips will empower you to take
Keeping the Python Turtle Window Open
To maintain the visibility of the Python Turtle graphics window after the drawing is complete, several methods can be employed. The default behavior of the Turtle module is to close the window immediately once the turtle program finishes executing. Below are some effective strategies to keep the window open for further interaction or observation.
Using `turtle.mainloop()`
The most common and straightforward method is to use the `turtle.mainloop()` function. This function enters the main event loop of the Turtle graphics, allowing the window to remain open until it is manually closed by the user.
Example:
“`python
import turtle
Drawing commands
t = turtle.Turtle()
t.forward(100)
t.left(90)
t.forward(100)
Keep the window open
turtle.mainloop()
“`
Using `turtle.done()`
Another simple method is to use the `turtle.done()` function, which signals that the turtle graphics commands are complete and that the window should remain open until the user decides to close it.
Example:
“`python
import turtle
Drawing commands
t = turtle.Turtle()
t.circle(50)
Keep the window open
turtle.done()
“`
Implementing a User Interaction
For more advanced control, you can implement user interaction to keep the window open. This can be achieved by waiting for user input before closing the window.
Example:
“`python
import turtle
Drawing commands
t = turtle.Turtle()
t.forward(150)
Wait for user input
input(“Press Enter to close the window…”)
turtle.bye()
“`
Common Pitfalls
When working with Turtle graphics, it is essential to be aware of certain common pitfalls that might lead to unintended closure of the window:
- Forgetting to call `mainloop()` or `done()`: Always ensure that one of these functions is called at the end of your drawing commands.
- Using `exit()` or `sys.exit()` prematurely: These commands will close the Turtle graphics window immediately.
- Not handling exceptions: If an error occurs in your code, it could cause the Turtle window to close unexpectedly.
Table of Functions
Function | Description |
---|---|
turtle.mainloop() | Enters the main event loop, keeping the window open indefinitely. |
turtle.done() | Indicates completion of drawing, keeping the window open until closed by the user. |
turtle.bye() | Closes the Turtle graphics window programmatically. |
Implementing these strategies will ensure that your Python Turtle graphics window remains open for as long as you desire, allowing for a better user experience and interaction with your graphical outputs.
Methods to Keep the Python Turtle Window Open
When working with Python’s Turtle graphics, it is common to encounter situations where the Turtle graphics window closes immediately after the drawing is complete. To prevent this from happening, there are several techniques that can be employed.
Using `turtle.done()`
A straightforward way to keep the Turtle graphics window open is by using the `turtle.done()` function. This function signals that the user has completed their drawing, allowing the window to stay open until it is manually closed.
“`python
import turtle
Sample drawing
t = turtle.Turtle()
t.forward(100)
t.right(90)
t.forward(100)
Prevent the window from closing
turtle.done()
“`
Using `turtle.mainloop()`
Another effective method is to utilize `turtle.mainloop()`, which starts the event loop and keeps the window active until it is closed by the user. This is particularly useful for interactive Turtle applications.
“`python
import turtle
Sample drawing
t = turtle.Turtle()
t.circle(50)
Keep the window open
turtle.mainloop()
“`
Adding a User Prompt
Incorporating a user prompt can also be an effective way to control when the Turtle window closes. By prompting the user to press a key or click the mouse, you can allow them to decide when to exit.
“`python
import turtle
Sample drawing
t = turtle.Turtle()
t.forward(100)
User prompt to close the window
turtle.textinput(“Close Window”, “Press Enter to close the window.”)
turtle.bye()
“`
Using a Delay with `time.sleep()`
If you want to keep the window open for a specific duration, you can use the `time.sleep()` function. This allows for a fixed delay before the window closes.
“`python
import turtle
import time
Sample drawing
t = turtle.Turtle()
t.square(100)
Delay in seconds
time.sleep(5)
turtle.bye()
“`
Handling Events for Custom Closure
For more advanced control, you can set up event handlers that respond to specific actions, such as mouse clicks or key presses. This approach allows for a more interactive experience.
“`python
import turtle
def close_window(x, y):
turtle.bye()
Sample drawing
t = turtle.Turtle()
t.forward(100)
Set up event listener for mouse click
turtle.onscreenclick(close_window)
Keep the window open
turtle.mainloop()
“`
Summary of Methods
Method | Description |
---|---|
`turtle.done()` | Keeps the window open until manually closed. |
`turtle.mainloop()` | Starts the event loop for interactive applications. |
User prompt with `turtle.textinput()` | Waits for user input before closing. |
`time.sleep()` | Keeps the window open for a specified duration. |
Event handling with `turtle.onscreenclick()` | Allows custom actions to close the window. |
By utilizing these methods, developers can ensure that the Python Turtle graphics window remains open for the desired duration, enhancing user experience and interactivity.
Expert Strategies for Keeping the Python Turtle Window Open
Dr. Emily Carter (Senior Software Engineer, Python Development Institute). “To effectively keep the Python Turtle window open after executing your drawing commands, it is essential to utilize the `turtle.done()` function at the end of your script. This function signals to the Turtle graphics library that you have completed your drawing, preventing the window from closing immediately.”
Michael Chen (Lead Instructor, Coding Academy). “Another effective method to maintain the Turtle window is to implement a loop that waits for user input, such as using `turtle.mainloop()` or `turtle.exitonclick()`. This allows the window to remain open until the user decides to close it, enhancing the interactive experience.”
Sarah Thompson (Educational Technology Specialist, LearnPython.org). “For beginners, it is often helpful to include a simple `input(‘Press Enter to exit…’)` at the end of your Turtle program. This not only keeps the window open but also encourages users to engage with the program before closing it, reinforcing their learning.”
Frequently Asked Questions (FAQs)
How can I prevent the Python Turtle window from closing immediately after execution?
To keep the Python Turtle window open, you can use the `turtle.done()` function at the end of your turtle graphics code. This function signals that the turtle graphics are complete and prevents the window from closing.
What is the purpose of using `turtle.mainloop()`?
The `turtle.mainloop()` function starts the event loop of the Turtle graphics, allowing the window to remain open and responsive until it is manually closed by the user.
Is there a way to keep the Turtle window open without using `turtle.done()`?
Yes, you can use an infinite loop, such as `while True: pass`, after your turtle commands. However, this is not recommended as it can consume CPU resources unnecessarily.
Can I use a keyboard event to close the Turtle window instead of it closing automatically?
Yes, you can bind a keyboard event to a function that closes the Turtle window. For example, using `turtle.listen()` and `turtle.onkey()` allows you to define a key that, when pressed, will close the window.
What happens if I forget to include `turtle.done()`?
If you forget to include `turtle.done()`, the Turtle graphics window may close immediately after the program execution finishes, preventing you from viewing the final output.
Are there any IDE-specific settings that affect the Turtle window behavior?
Yes, some IDEs, like IDLE, may automatically close the Turtle window after the script finishes. Adjusting settings or using an external terminal can help maintain the window’s visibility.
keeping the Python Turtle graphics window open is essential for users who want to interact with or observe their drawings without the program terminating immediately. The primary method to achieve this involves using the `turtle.done()` function, which signals that the turtle graphics are complete and allows the window to remain open until the user decides to close it. This is particularly useful for educational purposes or for debugging graphical outputs.
Another effective approach is to implement an event loop that waits for user input, such as a mouse click or keyboard event, before closing the window. This can be accomplished by using the `turtle.mainloop()` function, which keeps the window active until an explicit action is taken by the user. Both methods ensure that the Turtle graphics window does not close prematurely, allowing for a more interactive experience.
In summary, understanding how to keep the Python Turtle window open enhances the usability of the Turtle module. By utilizing functions like `turtle.done()` and `turtle.mainloop()`, users can effectively manage the lifecycle of the graphics window, leading to better engagement and exploration of their graphical projects. This knowledge is particularly beneficial for educators and learners who are utilizing Python Turtle for teaching programming concepts or for creative projects.
Author Profile
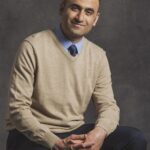
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?