How Can You Determine if a Checkbox is Checked in JavaScript?
When it comes to web development, interactivity is key to creating engaging user experiences. One of the simplest yet most effective ways to enhance user interaction is through the use of checkboxes. These small, clickable elements allow users to make selections, whether for forms, preferences, or surveys. However, to harness the full potential of checkboxes, developers must understand how to determine their state—specifically, how to know if a checkbox is checked using JavaScript. This knowledge not only empowers developers to create dynamic applications but also ensures that user inputs are accurately captured and processed.
In the world of JavaScript, checking the status of a checkbox is a fundamental skill that every developer should master. The process involves utilizing the Document Object Model (DOM) to access the checkbox element and examining its properties. By leveraging JavaScript’s built-in methods, developers can easily determine whether a checkbox is checked or unchecked, allowing for responsive designs and tailored user experiences. Understanding this concept opens the door to a myriad of possibilities, from simple form validation to complex interactive features.
As we delve deeper into the mechanics of checkboxes in JavaScript, we will explore various methods and best practices for effectively managing their states. Whether you’re building a straightforward form or a sophisticated web application, knowing how to handle checkbox interactions will
Checking Checkbox State with JavaScript
To determine if a checkbox is checked in JavaScript, you can access the checkbox element through the Document Object Model (DOM) and evaluate its `checked` property. The `checked` property returns a boolean value: `true` if the checkbox is checked and “ if it is not.
Here’s a simple method to check the state of a checkbox:
javascript
var checkbox = document.getElementById(“myCheckbox”);
if (checkbox.checked) {
console.log(“Checkbox is checked.”);
} else {
console.log(“Checkbox is not checked.”);
}
In this example, `myCheckbox` is the ID of the checkbox input element. This code retrieves the checkbox element and checks its state.
Using Event Listeners to Monitor Checkbox State
You can also use event listeners to monitor changes in the checkbox state. This approach is useful for dynamically responding to user interactions.
Here’s how you can implement it:
javascript
var checkbox = document.getElementById(“myCheckbox”);
checkbox.addEventListener(“change”, function() {
if (this.checked) {
console.log(“Checkbox is checked.”);
} else {
console.log(“Checkbox is not checked.”);
}
});
This code snippet adds an event listener that triggers whenever the checkbox’s state changes, providing immediate feedback.
Checkbox State in a Form
When dealing with forms, you may want to know the state of multiple checkboxes. You can gather the states of all checkboxes by selecting them using a common class name or attribute.
Consider the following HTML structure:
You can then use the following JavaScript to check which checkboxes are checked:
javascript
var checkboxes = document.querySelectorAll(‘.myCheckbox’);
checkboxes.forEach(function(checkbox) {
if (checkbox.checked) {
console.log(checkbox.value + ” is checked.”);
}
});
This code iterates through all checkboxes with the class `myCheckbox` and logs the value of each checked checkbox.
Table of Checkbox States
When presenting checkbox states, a table can be useful for clarity. Below is an example of how to display checkbox states in a simple HTML table format:
Checkbox | Checked State |
---|---|
Option 1 | |
Option 2 | |
Option 3 |
You can update the table with checkbox states dynamically using JavaScript:
javascript
checkboxes.forEach(function(checkbox, index) {
document.getElementById(‘state’ + (index + 1)).innerText = checkbox.checked ? “Checked” : “Not Checked”;
});
This approach ensures that the table reflects the current state of each checkbox, providing a clear and concise overview of the selections made by the user.
Checking the Status of a Checkbox in JavaScript
To determine if a checkbox is checked in JavaScript, you can utilize the `checked` property of the checkbox input element. This property returns a Boolean value indicating whether the checkbox is checked (`true`) or not (“).
Accessing the Checkbox Element
You can access the checkbox element using various methods, such as `getElementById`, `querySelector`, or `getElementsByName`. Below are examples of how to access a checkbox input using these methods:
javascript
// Using getElementById
const checkbox = document.getElementById(‘myCheckbox’);
// Using querySelector
const checkbox = document.querySelector(‘#myCheckbox’);
// Using getElementsByName
const checkboxes = document.getElementsByName(‘myCheckbox’);
const checkbox = checkboxes[0]; // Access the first checkbox
Checking If the Checkbox is Checked
Once you have a reference to the checkbox element, you can check if it is checked by accessing its `checked` property. Here’s how to do it:
javascript
if (checkbox.checked) {
console.log(‘Checkbox is checked.’);
} else {
console.log(‘Checkbox is not checked.’);
}
Handling Events with Checkboxes
To react to changes in the checkbox state, you can add an event listener for the `change` event. This allows you to perform actions whenever the checkbox is checked or unchecked.
javascript
checkbox.addEventListener(‘change’, function() {
if (this.checked) {
console.log(‘Checkbox is checked.’);
} else {
console.log(‘Checkbox is not checked.’);
}
});
Multiple Checkboxes
When working with multiple checkboxes, you can use the `querySelectorAll` method to retrieve all checkboxes with a specific class or name. Then, iterate through the NodeList to check their states.
javascript
const checkboxes = document.querySelectorAll(‘.myCheckbox’);
checkboxes.forEach((checkbox) => {
if (checkbox.checked) {
console.log(‘A checkbox is checked.’);
}
});
Summary of Key Points
- Access Checkbox: Use methods like `getElementById`, `querySelector`, or `getElementsByName`.
- Check Status: Use the `checked` property to determine if a checkbox is checked.
- Event Handling: Use the `change` event to respond to checkbox state changes.
- Multiple Checkboxes: Utilize `querySelectorAll` to manage multiple checkboxes and check their states collectively.
By following these practices, you can effectively manage checkbox states and enhance user interaction within your web applications.
Understanding Checkbox States in JavaScript: Expert Insights
Emily Carter (Senior Web Developer, CodeCraft Solutions). “To determine if a checkbox is checked in JavaScript, you can access the checkbox element using its ID or name and then check its ‘checked’ property. This is a straightforward method that ensures you get real-time feedback on user selections.”
Michael Tran (JavaScript Engineer, Tech Innovators). “Utilizing event listeners is crucial for dynamic applications. By adding a ‘change’ event listener to your checkbox, you can execute specific functions based on whether the checkbox is checked or not, providing a responsive user experience.”
Sarah Patel (Frontend Architect, Digital Solutions Inc.). “It is essential to ensure accessibility when working with checkboxes. Always ensure that your JavaScript code accounts for keyboard interactions and screen readers, as this will enhance usability and compliance with web standards.”
Frequently Asked Questions (FAQs)
How can I check if a checkbox is checked in JavaScript?
You can check if a checkbox is checked by accessing its `checked` property. For example, if your checkbox has an ID of “myCheckbox”, you can use:
javascript
var isChecked = document.getElementById(‘myCheckbox’).checked;
What is the difference between checked and value properties of a checkbox?
The `checked` property indicates whether the checkbox is selected (true) or not (). The `value` property represents the value assigned to the checkbox, which is submitted with the form if the checkbox is checked.
Can I use jQuery to check if a checkbox is checked?
Yes, you can use jQuery for this purpose. The syntax is:
javascript
var isChecked = $(‘#myCheckbox’).is(‘:checked’);
How do I handle checkbox change events in JavaScript?
You can use the `addEventListener` method to listen for changes. For example:
javascript
document.getElementById(‘myCheckbox’).addEventListener(‘change’, function() {
if (this.checked) {
// Checkbox is checked
} else {
// Checkbox is unchecked
}
});
What will happen if I try to access a checkbox that does not exist?
If you attempt to access a checkbox that does not exist, JavaScript will return `null` for `document.getElementById()`. Attempting to access the `checked` property on `null` will result in a TypeError.
Is it possible to check multiple checkboxes at once?
Yes, you can check multiple checkboxes by using a loop. For example:
javascript
var checkboxes = document.querySelectorAll(‘input[type=”checkbox”]’);
checkboxes.forEach(function(checkbox) {
if (checkbox.checked) {
// Do something with the checked checkbox
}
});
In JavaScript, determining whether a checkbox is checked involves accessing the checkbox element through the Document Object Model (DOM) and evaluating its `checked` property. This property returns a boolean value, which indicates whether the checkbox is currently selected (checked) or not selected (unchecked). The process typically involves using methods such as `getElementById`, `querySelector`, or `getElementsByName` to retrieve the checkbox element, followed by checking its `checked` property.
Additionally, event listeners can be employed to monitor changes to the checkbox state. By attaching an event listener for the `change` event, developers can execute specific functions whenever the checkbox is checked or unchecked. This approach enhances interactivity within web applications, allowing for dynamic responses based on user input.
Overall, understanding how to check the state of a checkbox in JavaScript is essential for creating responsive and user-friendly web applications. By leveraging the DOM and event handling, developers can effectively manage user interactions and ensure that their applications behave as expected based on user selections.
Author Profile
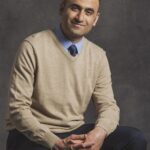
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?