How Can You Effectively Limit Decimal Places in Python?
When working with numerical data in Python, precision is often of utmost importance. Whether you’re developing a financial application, performing scientific calculations, or simply displaying results to users, managing decimal places can significantly impact the clarity and usability of your output. In a world where data visualization and presentation matter more than ever, knowing how to limit decimal places in Python is a valuable skill that every programmer should master.
In Python, there are several methods to control the number of decimal places in your output, each suited for different scenarios. From formatting strings to using built-in functions, the language offers a range of tools that can help you present your data cleanly and effectively. Understanding the nuances of these methods will not only enhance your coding skills but also improve the overall quality of your applications.
As you dive deeper into the topic, you’ll discover practical techniques that can be applied in various contexts, whether you’re rounding numbers for display or ensuring consistent precision in calculations. With the right approach, you can make your numerical data more readable and professional, paving the way for clearer communication and better decision-making. Get ready to explore the world of decimal management in Python and unlock the potential of your numerical outputs!
Using the round() Function
The simplest way to limit decimal places in Python is by utilizing the built-in `round()` function. This function rounds a floating-point number to a specified number of decimal places.
To use the `round()` function, you can follow this syntax:
python
round(number, ndigits)
Where `number` is the floating-point number you wish to round, and `ndigits` is the number of decimal places to which you want to round.
Example:
python
value = 12.34567
rounded_value = round(value, 2) # Output: 12.35
Formatting Strings
Another effective method for limiting decimal places is by formatting strings. Python provides several ways to format strings, including f-strings (formatted string literals) and the `format()` method.
- Using f-strings (Python 3.6 and above):
python
value = 12.34567
formatted_value = f”{value:.2f}” # Output: ‘12.35’
- Using the format() method:
python
value = 12.34567
formatted_value = “{:.2f}”.format(value) # Output: ‘12.35’
Decimal Module for High Precision
For applications requiring high precision, Python’s `decimal` module is advisable. This module provides support for fast correctly-rounded decimal floating point arithmetic. You can specify the precision and limit the number of decimal places effectively.
Here’s how to use the `decimal` module:
python
from decimal import Decimal, ROUND_DOWN
value = Decimal(‘12.34567’)
limited_value = value.quantize(Decimal(‘0.01’), rounding=ROUND_DOWN) # Output: Decimal(‘12.34’)
Table of Rounding Methods
The following table summarizes various methods available for limiting decimal places in Python:
Method | Description | Example |
---|---|---|
round() | Rounds a number to a specified number of decimal places. | round(12.34567, 2) → 12.35 |
f-strings | Formats floating-point numbers with specified precision. | f”{12.34567:.2f}” → ‘12.35’ |
format() | Formats numbers using the format() method. | “{:.2f}”.format(12.34567) → ‘12.35’ |
decimal module | Provides high precision rounding with customizable options. | Decimal(‘12.34567’).quantize(Decimal(‘0.01’)) → Decimal(‘12.35’) |
By utilizing these methods, you can effectively control the number of decimal places in your Python applications, ensuring precision and clarity in your numerical outputs.
Using the round() Function
The simplest method to limit decimal places in Python is by using the built-in `round()` function. This function takes two arguments: the number you want to round and the number of decimal places.
python
rounded_value = round(3.14159, 2) # Returns 3.14
- The first argument is the float you want to round.
- The second argument specifies the number of decimal places.
String Formatting
String formatting can also be employed to limit decimal places when displaying numbers. There are different ways to format strings in Python:
- f-Strings (Python 3.6+):
python
value = 3.14159
formatted_value = f”{value:.2f}” # Returns ‘3.14’
- format() Method:
python
formatted_value = “{:.2f}”.format(value) # Returns ‘3.14’
- Percentage Formatting:
python
formatted_value = “%.2f” % value # Returns ‘3.14’
Each method allows for concise control over the number of decimal places displayed.
Decimal Module
For more precise control over decimal places, especially in financial applications, the `decimal` module is recommended. This module provides a `Decimal` data type for decimal floating point arithmetic.
python
from decimal import Decimal, ROUND_DOWN
value = Decimal(‘3.14159’)
limited_value = value.quantize(Decimal(‘0.00’), rounding=ROUND_DOWN) # Returns Decimal(‘3.14’)
- The `quantize()` method adjusts the decimal to the specified format.
- You can also specify different rounding modes, such as `ROUND_UP`, `ROUND_HALF_UP`, etc.
NumPy Library
If you are working with arrays or matrices, the NumPy library provides functionalities to limit decimal places efficiently.
python
import numpy as np
array = np.array([3.14159, 2.71828])
rounded_array = np.round(array, 2) # Returns array([3.14, 2.72])
This method is particularly useful for vectorized operations, improving performance with large datasets.
Pandas Library
For data manipulation with tabular data, the Pandas library can be utilized to limit decimal places in DataFrames.
python
import pandas as pd
df = pd.DataFrame({‘Values’: [3.14159, 2.71828]})
df[‘Rounded_Values’] = df[‘Values’].round(2) # Creates a new column with rounded values
This approach allows you to maintain the original data while also presenting rounded values for analysis or reporting.
Custom Function
Creating a custom function can encapsulate the rounding logic for reuse throughout your code.
python
def limit_decimal_places(value, decimal_places):
return round(value, decimal_places)
result = limit_decimal_places(3.14159, 2) # Returns 3.14
This method enhances code readability and maintainability by centralizing rounding logic.
Expert Insights on Limiting Decimal Places in Python
Dr. Emily Chen (Senior Data Scientist, Tech Innovations Inc.). “When working with floating-point numbers in Python, it is essential to understand the various methods for limiting decimal places. The built-in `round()` function is a straightforward approach, but for more control over formatting, utilizing the `format()` function or f-strings can yield cleaner outputs.”
Michael Torres (Software Engineer, Python Programming Hub). “In Python, limiting decimal places can be accomplished effectively using the `decimal` module, which provides precise control over decimal arithmetic. This is particularly useful in financial applications where accuracy is paramount.”
Sarah Patel (Lead Python Developer, CodeCraft Solutions). “For developers looking to present numerical data with limited decimal places, leveraging string formatting techniques such as `”{:.2f}”.format(value)` or f-strings is often the most user-friendly method. This ensures that numbers are displayed consistently without altering their underlying precision.”
Frequently Asked Questions (FAQs)
How can I limit decimal places when printing a float in Python?
You can use formatted string literals (f-strings) or the `format()` method. For example, `print(f”{value:.2f}”)` limits the float to two decimal places.
What function can I use to round a number to a specific number of decimal places?
The built-in `round()` function can be used, e.g., `round(value, 2)` rounds the number to two decimal places.
Is there a way to limit decimal places in a Pandas DataFrame?
Yes, you can use the `round()` method on a DataFrame, such as `df.round(2)`, which limits all numeric columns to two decimal places.
How do I format a float to a specific number of decimal places without rounding?
You can use the `Decimal` class from the `decimal` module, which allows for precise control over decimal representation without rounding.
Can I limit decimal places in a NumPy array?
Yes, you can use `numpy.around(array, decimals=2)` to limit the decimal places of all elements in a NumPy array to two.
What is the difference between formatting and rounding in Python?
Formatting displays a number with a specified number of decimal places without altering the original value, while rounding changes the value to fit the specified precision.
In Python, there are several methods to limit the number of decimal places in numerical outputs. The most common approaches include using the built-in `round()` function, string formatting techniques, and the `Decimal` module from the `decimal` library. Each method has its own advantages and is suited for different scenarios depending on the level of precision required and the context in which the numbers are being used.
The `round()` function is straightforward and allows for quick rounding of floating-point numbers to a specified number of decimal places. However, it is important to note that `round()` can sometimes lead to unexpected results due to the way floating-point arithmetic works in Python. For more control over the formatting of numbers, string formatting methods such as f-strings or the `format()` function provide a robust solution, allowing for precise control over how numbers are displayed.
For applications that require high precision and accurate decimal representation, the `Decimal` module is recommended. This module avoids the pitfalls of floating-point arithmetic by allowing users to define the precision explicitly, making it ideal for financial calculations or other scenarios where accuracy is paramount. By choosing the appropriate method based on the specific requirements of a task, Python developers can effectively manage decimal places in their applications.
Author Profile
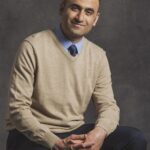
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?