How Can You Easily List Column Names in a Pandas DataFrame?
In the world of data analysis, the ability to manipulate and understand your dataset is crucial. Whether you’re a seasoned data scientist or just starting your journey with Python’s powerful Pandas library, knowing how to navigate your data effectively can make all the difference. One fundamental aspect of working with dataframes is understanding the structure of your dataset, particularly the column names. These names not only serve as identifiers for the data but also play a pivotal role in data manipulation and analysis.
Listing the column names in a Pandas dataframe is a straightforward yet essential task that can enhance your workflow significantly. By familiarizing yourself with the column names, you can quickly assess the variables at your disposal, identify any potential issues, and streamline your data processing tasks. This foundational skill sets the stage for more advanced operations, such as filtering, aggregating, and visualizing your data.
In this article, we will explore the various methods to list column names in Pandas, highlighting their importance and providing you with practical examples. Whether you are preparing for a data analysis project or simply looking to refine your skills, understanding how to access and manipulate column names will empower you to work more efficiently with your datasets. Join us as we delve into the nuances of this essential Pandas functionality!
Accessing Column Names in Pandas
To list the column names of a DataFrame in Pandas, you can utilize the `columns` attribute. This attribute returns an Index object containing all the column names. Here’s how you can do it:
“`python
import pandas as pd
Example DataFrame
data = {
‘Name’: [‘Alice’, ‘Bob’, ‘Charlie’],
‘Age’: [25, 30, 35],
‘City’: [‘New York’, ‘Los Angeles’, ‘Chicago’]
}
df = pd.DataFrame(data)
Listing column names
column_names = df.columns
print(column_names)
“`
The output will be:
“`
Index([‘Name’, ‘Age’, ‘City’], dtype=’object’)
“`
This method is straightforward and provides you with an easy way to access the column names.
Displaying Column Names as a List
If you prefer to have the column names in a list format, you can convert the Index object to a list using the `tolist()` method. This can be useful for further processing or simply for display purposes.
“`python
Convert column names to a list
column_names_list = df.columns.tolist()
print(column_names_list)
“`
The output will be:
“`
[‘Name’, ‘Age’, ‘City’]
“`
Accessing Column Names Using a Loop
Another approach is to iterate over the column names using a loop. This method allows you to perform operations on each column name as needed.
“`python
for col in df.columns:
print(col)
“`
This will print each column name on a new line:
“`
Name
Age
City
“`
Summary Table of Methods
The following table summarizes the different methods to list column names in a Pandas DataFrame:
Method | Code Example | Output |
---|---|---|
Using `columns` attribute | `df.columns` | `Index([‘Name’, ‘Age’, ‘City’], dtype=’object’)` |
Using `tolist()` method | `df.columns.tolist()` | `[‘Name’, ‘Age’, ‘City’]` |
Using a loop | `for col in df.columns: print(col)` | `Name\nAge\nCity` |
These methods provide flexibility depending on your needs, whether you require a simple listing, a more structured output, or an iterative approach for further manipulation.
Methods to List Column Names in Pandas
In Pandas, there are several effective methods to retrieve the names of columns in a DataFrame. Below are the most commonly used techniques.
Using the `columns` Attribute
The simplest way to obtain the column names is through the `columns` attribute of a DataFrame. This attribute returns an Index object containing the column labels.
“`python
import pandas as pd
Sample DataFrame
data = {‘A’: [1, 2], ‘B’: [3, 4]}
df = pd.DataFrame(data)
List column names
column_names = df.columns
print(column_names)
“`
- The output will be an Index object:
“`
Index([‘A’, ‘B’], dtype=’object’)
“`
Converting Column Names to a List
If you require the column names in a list format, you can convert the Index object to a list using the `tolist()` method.
“`python
Convert column names to a list
column_names_list = df.columns.tolist()
print(column_names_list)
“`
- The output will be:
“`
[‘A’, ‘B’]
“`
Accessing Column Names Directly with `list()`
Another approach to retrieve column names is to use the built-in `list()` function directly on the columns attribute.
“`python
List column names using list()
column_names_direct = list(df.columns)
print(column_names_direct)
“`
- The output will also be:
“`
[‘A’, ‘B’]
“`
Using the `keys()` Method
The `keys()` method of a DataFrame provides an alternative way to access the column names. This method behaves similarly to `columns`.
“`python
Using keys() to get column names
column_names_keys = df.keys()
print(column_names_keys)
“`
- The output will be:
“`
Index([‘A’, ‘B’], dtype=’object’)
“`
Displaying Column Names with `DataFrame.info()`
The `info()` method provides a concise summary of the DataFrame, including column names, non-null counts, and data types.
“`python
Display DataFrame info
df.info()
“`
- The output will include the column names among other details:
“`
RangeIndex: 2 entries, 0 to 1
Data columns (total 2 columns):
Column Non-Null Count Dtype
— —— ————– —–
0 A 2 non-null int64
1 B 2 non-null int64
“`
Using `list()` on `DataFrame`
You can also use `list()` directly on the DataFrame to get the column names.
“`python
Get column names using list() on DataFrame
column_names_via_df = list(df)
print(column_names_via_df)
“`
- The output will be:
“`
[‘A’, ‘B’]
“`
These methods provide flexibility depending on whether you require the column names as an Index object, a list, or as part of a DataFrame summary.
Expert Insights on Listing Column Names in Pandas
Dr. Emily Chen (Data Scientist, Analytics Innovations). “To list the column names in a Pandas DataFrame, one can simply use the `.columns` attribute. This method provides a quick and efficient way to access the names of all columns, which is essential for data exploration and manipulation.”
Michael Thompson (Senior Python Developer, Tech Solutions Inc.). “Utilizing the `.columns.tolist()` method not only lists the column names but also converts them into a Python list. This is particularly useful when you need to perform further operations or checks on the column names.”
Sarah Patel (Machine Learning Engineer, Data Insights Group). “For users interested in a more comprehensive overview, combining the `.columns` attribute with the `print()` function can enhance readability, especially when working with large datasets. This approach allows for clear visibility of the DataFrame structure.”
Frequently Asked Questions (FAQs)
How do I list the column names in a pandas DataFrame?
You can list the column names in a pandas DataFrame by accessing the `.columns` attribute. For example, `df.columns` will return an Index object containing the column names.
Can I convert the column names to a list in pandas?
Yes, you can convert the column names to a list using the `.tolist()` method. For instance, `df.columns.tolist()` will provide a list of the column names.
Is there a way to get the column names along with their data types?
Yes, you can use the `.dtypes` attribute to obtain the data types of each column. Combining this with the `.items()` method allows you to iterate over column names and their corresponding data types.
What if I want to filter column names based on a specific condition?
You can filter column names using list comprehension. For example, `[col for col in df.columns if ‘keyword’ in col]` will return a list of column names that contain the specified keyword.
Can I rename the columns while listing them?
Renaming columns does not directly affect how you list them. However, you can rename columns using the `.rename()` method before listing them to see the updated names.
How do I display the column names in a specific order?
To display column names in a specific order, you can use indexing. For example, `df[[‘column1’, ‘column2’, ‘column3’]].columns` will show the column names in the specified order.
In the realm of data manipulation and analysis, the ability to list column names in a pandas DataFrame is a fundamental skill. This operation is crucial for understanding the structure of the dataset, facilitating further data exploration and processing. Pandas provides straightforward methods to achieve this, primarily through the use of the `.columns` attribute or the `list()` function. Both approaches yield a clear overview of the DataFrame’s columns, allowing users to efficiently navigate their data.
Utilizing the `.columns` attribute is the most direct method, as it returns an Index object containing the column names. Alternatively, converting this Index object to a list using the `list()` function can be beneficial for those who prefer working with lists in Python. These methods not only enhance the user’s ability to inspect the DataFrame but also serve as a stepping stone for more complex data operations, such as filtering or renaming columns.
In summary, mastering the technique of listing column names in pandas is essential for effective data analysis. It empowers users to quickly assess their datasets and lays the groundwork for more advanced data manipulation tasks. As one becomes more proficient in pandas, these foundational skills will significantly contribute to more complex data workflows and analyses.
Author Profile
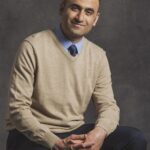
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?