How Can You Easily List the Columns in a DataFrame Using Python?
In the world of data analysis, Python has emerged as a powerhouse, largely due to its versatile libraries like Pandas. As you dive into the realm of data manipulation, one of the foundational tasks you’ll encounter is working with DataFrames. These two-dimensional, size-mutable, and potentially heterogeneous tabular data structures are essential for storing and analyzing data. However, before you can perform any intricate operations, it’s crucial to understand the structure of your DataFrame, particularly the columns it contains.
Listing the columns in a DataFrame may seem like a simple task, but it serves as a gateway to more complex data exploration and manipulation. Whether you’re cleaning data, performing exploratory data analysis, or preparing for machine learning, knowing how to access and list the columns is an essential skill. This process not only helps you familiarize yourself with the dataset but also allows you to identify relevant features for your analysis.
In this article, we will explore various methods to list the columns in a DataFrame using Python. We’ll cover both straightforward approaches for beginners and more advanced techniques for seasoned data analysts. By the end, you will have a solid understanding of how to efficiently access and utilize the column information in your DataFrames, setting the stage for deeper data analysis and insights.
Accessing DataFrame Columns
To list the columns in a DataFrame using Python, particularly with the pandas library, you can utilize the `.columns` attribute. This attribute returns an index object containing the column labels of the DataFrame.
For example, if you have a DataFrame named `df`, you can list its columns as follows:
python
import pandas as pd
# Sample DataFrame
data = {
‘Name’: [‘Alice’, ‘Bob’, ‘Charlie’],
‘Age’: [25, 30, 35],
‘City’: [‘New York’, ‘Los Angeles’, ‘Chicago’]
}
df = pd.DataFrame(data)
# Listing columns
columns = df.columns
print(columns)
The output will be:
Index([‘Name’, ‘Age’, ‘City’], dtype=’object’)
Converting Column Names to List
If you prefer to have the column names in a list format, you can convert the Index object to a list using the `tolist()` method. This is particularly useful when you need to manipulate or iterate over the column names.
python
column_list = df.columns.tolist()
print(column_list)
The output will be:
[‘Name’, ‘Age’, ‘City’]
Using Other Methods to List Columns
In addition to the `.columns` attribute, there are other methods you can use to achieve similar results:
- Using `list()`: You can wrap the `.columns` attribute with the `list()` function.
python
column_list = list(df.columns)
print(column_list)
- Using `df.keys()`: This method also returns the column labels.
python
column_keys = df.keys()
print(column_keys.tolist())
- Using `df.info()`: This method provides a concise summary of the DataFrame, including the column names.
python
df.info()
This will output information about the DataFrame, including its columns and their types.
Method | Output Type | Example |
---|---|---|
df.columns | Index | df.columns |
df.columns.tolist() | List | df.columns.tolist() |
list(df.columns) | List | list(df.columns) |
df.keys() | Index | df.keys().tolist() |
By employing these methods, you can efficiently list and manipulate the column names in your pandas DataFrame, enhancing your data analysis capabilities.
Using Pandas to List DataFrame Columns
In Python, the Pandas library provides a straightforward way to handle and manipulate data in DataFrames. To list the columns in a DataFrame, several methods can be employed.
Methods to List Columns
- Using the .columns Attribute
The simplest way to retrieve the column names is by using the `.columns` attribute of the DataFrame. This will return an index object containing the column labels.
python
import pandas as pd
# Sample DataFrame
data = {‘A’: [1, 2], ‘B’: [3, 4]}
df = pd.DataFrame(data)
# List columns
columns = df.columns
print(columns)
- Using the list() Function
If a simple list format is preferred, you can convert the columns index to a list using the `list()` function.
python
columns_list = list(df.columns)
print(columns_list)
- Using the .keys() Method
The `.keys()` method is another way to access the column names, which is synonymous with the `.columns` attribute.
python
column_keys = df.keys()
print(column_keys)
- Using the .info() Method
For a more detailed summary of the DataFrame, including column names, use the `.info()` method. This method provides additional information about the DataFrame, such as data types and non-null counts.
python
df.info()
Example DataFrame for Demonstration
Column Name | Data Type | Sample Values |
---|---|---|
A | int64 | 1, 2 |
B | int64 | 3, 4 |
Using the methods outlined above on the example DataFrame will yield the following results:
- Using .columns: Index([‘A’, ‘B’], dtype=’object’)
- Using list(): [‘A’, ‘B’]
- Using .keys(): Index([‘A’, ‘B’], dtype=’object’)
- Using .info(): Will display additional information including column names.
These methods provide a comprehensive approach to listing and understanding the structure of columns in a Pandas DataFrame.
Expert Insights on Listing DataFrame Columns in Python
Dr. Emily Carter (Data Scientist, Tech Innovations Inc.). “To efficiently list the columns in a DataFrame using Python, the most straightforward method is to utilize the `columns` attribute of the DataFrame. This approach is not only easy to implement but also provides a clear view of the structure of your data.”
Michael Chen (Python Developer, Data Solutions LLC). “Using the Pandas library, one can access the columns of a DataFrame by calling `df.columns.tolist()`. This method converts the column index into a list, which is particularly useful for further data manipulation or analysis.”
Dr. Sarah Patel (Professor of Computer Science, University of Data Science). “For those looking to explore DataFrame attributes in a more interactive manner, employing the `info()` method can be beneficial. It not only lists the columns but also provides additional information such as data types and non-null counts.”
Frequently Asked Questions (FAQs)
How can I list the columns in a Pandas DataFrame?
You can list the columns of a Pandas DataFrame by accessing the `columns` attribute. For example, use `df.columns` where `df` is your DataFrame.
What method can I use to get a list of column names in a DataFrame?
You can convert the columns to a list by using `df.columns.tolist()`, which will return a standard Python list of column names.
Is there a way to display column names along with their data types?
Yes, you can use `df.dtypes` to display each column name along with its corresponding data type in the DataFrame.
Can I filter the columns based on specific criteria?
Yes, you can filter columns using conditions by applying boolean indexing or using methods like `filter()` to select columns that meet certain criteria.
How do I access a specific column in a DataFrame?
You can access a specific column by using the syntax `df[‘column_name’]` or `df.column_name` if the column name is a valid Python identifier.
What should I do if I want to rename the columns of a DataFrame?
To rename columns, use the `df.rename(columns={‘old_name’: ‘new_name’}, inplace=True)` method, which allows you to specify old and new column names.
In Python, particularly when using the Pandas library, listing the columns of a DataFrame is a straightforward task that can be accomplished using several methods. The most common approach is to access the `columns` attribute of the DataFrame, which returns an Index object containing the column labels. Alternatively, one can use the `keys()` method, which serves a similar purpose by providing the same column names. Both methods are efficient and widely used in data manipulation and analysis tasks.
Another useful method to list the columns is by converting the Index object to a list using the `tolist()` method. This can be particularly beneficial when the user requires the column names in a list format for further processing or iteration. Additionally, using the `info()` method provides a comprehensive overview of the DataFrame, including the column names, data types, and non-null counts, thus offering more context about the structure of the data.
In summary, there are multiple ways to list the columns in a DataFrame using Python’s Pandas library. Understanding these methods enhances data handling capabilities, allowing users to efficiently explore and manipulate their datasets. As data analysis often requires frequent inspection of DataFrame structures, familiarity with these techniques is essential for effective data management.
Author Profile
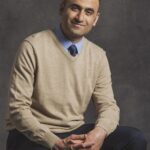
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?