How Can You Load a Dataset in Python Effortlessly?
Loading a dataset in Python is a fundamental skill that opens the door to a world of data analysis, machine learning, and scientific computing. Whether you’re a seasoned data scientist or a curious beginner, understanding how to efficiently load and manipulate datasets is crucial for extracting meaningful insights from your data. With a plethora of libraries and tools at your disposal, the process can be both straightforward and powerful, allowing you to dive deep into your data with ease.
In Python, the process of loading a dataset typically involves using libraries such as Pandas, NumPy, or even built-in functions for handling various file formats. These libraries not only simplify the task of reading data from CSV, Excel, JSON, or SQL databases but also provide a rich set of functionalities for data cleaning and preprocessing. Knowing how to load your data correctly sets the foundation for effective analysis, enabling you to focus on deriving insights rather than wrestling with data formats.
Moreover, the ability to load datasets efficiently can significantly enhance your workflow, especially when dealing with large volumes of data. As you explore the different methods available, you’ll discover best practices for optimizing performance and ensuring that your data is ready for analysis. In the following sections, we’ll delve deeper into the various techniques and tools that make loading datasets in Python a seamless experience,
Loading Datasets from CSV Files
One of the most common formats for datasets is CSV (Comma-Separated Values). Python’s `pandas` library offers a straightforward method to load CSV files using the `read_csv()` function. This function is versatile and allows for various parameters to customize how the data is read.
To load a CSV file, you can use the following syntax:
“`python
import pandas as pd
Load the dataset
data = pd.read_csv(‘path/to/your/dataset.csv’)
“`
Key parameters of `read_csv()` include:
- filepath_or_buffer: The path to the CSV file.
- sep: The delimiter that separates values; the default is a comma (`,`).
- header: Row number(s) to use as the column names; defaults to the first row.
- index_col: Column(s) to set as the index; this can be a single column or a list.
- dtype: Data type for data or columns; useful for optimizing memory usage.
Loading Datasets from Excel Files
Excel files are another common format for datasets, and `pandas` provides the `read_excel()` function to handle them. This function can read both `.xls` and `.xlsx` files.
Here’s an example of how to load an Excel file:
“`python
Load the dataset from an Excel file
data = pd.read_excel(‘path/to/your/dataset.xlsx’, sheet_name=’Sheet1′)
“`
Important parameters for `read_excel()` include:
- io: The file path or URL to the Excel file.
- sheet_name: Specify which sheet to read; can be a sheet name or an index.
- header: Row number(s) to use as the column names; defaults to the first row.
- usecols: Specify which columns to load; can be a list or a range.
- dtype: Data types for data or columns.
Loading Datasets from SQL Databases
To load data from SQL databases, `pandas` provides the `read_sql()` function. This function requires a connection to the database and a SQL query to select the data.
Here’s how you can do it:
“`python
import sqlite3
Create a database connection
conn = sqlite3.connect(‘path/to/your/database.db’)
Load the dataset using a SQL query
data = pd.read_sql(‘SELECT * FROM your_table_name’, conn)
Close the connection
conn.close()
“`
Parameters of `read_sql()` include:
- sql: The SQL query to execute.
- con: The database connection object.
- index_col: Column(s) to set as the index.
Loading Datasets from JSON Files
JSON (JavaScript Object Notation) is a lightweight data interchange format that is easy for humans to read and write. `pandas` can read JSON files using the `read_json()` function.
To load a JSON file, use the following code:
“`python
Load the dataset from a JSON file
data = pd.read_json(‘path/to/your/dataset.json’)
“`
Key parameters for `read_json()` include:
- path_or_buf: The file path or URL to the JSON file.
- orient: The expected format of the JSON string; options include `split`, `records`, `index`, etc.
File Format | Function | Common Parameters |
---|---|---|
CSV | pd.read_csv() | filepath_or_buffer, sep, header, index_col, dtype |
Excel | pd.read_excel() | io, sheet_name, header, usecols, dtype |
SQL | pd.read_sql() | sql, con, index_col |
JSON | pd.read_json() | path_or_buf, orient |
Loading Datasets Using Pandas
Pandas is one of the most popular libraries for data manipulation and analysis in Python. It provides a powerful DataFrame object for handling structured data and includes various functions to load datasets from different formats.
To load a dataset using Pandas, follow these steps:
- Install Pandas: Ensure you have Pandas installed in your Python environment. You can install it using pip:
“`bash
pip install pandas
“`
- Import Pandas: Import the library in your script:
“`python
import pandas as pd
“`
- Load from CSV: Use the `read_csv` function to load a CSV file:
“`python
df = pd.read_csv(‘path/to/your/file.csv’)
“`
- Load from Excel: For Excel files, utilize `read_excel`:
“`python
df = pd.read_excel(‘path/to/your/file.xlsx’, sheet_name=’Sheet1′)
“`
- Load from JSON: To load JSON data, use `read_json`:
“`python
df = pd.read_json(‘path/to/your/file.json’)
“`
- Load from SQL Database: If your data is stored in a SQL database, you can use `read_sql`:
“`python
import sqlite3
conn = sqlite3.connect(‘database.db’)
df = pd.read_sql(‘SELECT * FROM table_name’, conn)
“`
Loading Datasets with NumPy
NumPy is primarily used for numerical data and arrays. It can also load datasets from text files, binary files, and more.
- Install NumPy: First, ensure NumPy is installed:
“`bash
pip install numpy
“`
- Import NumPy: Import the library in your script:
“`python
import numpy as np
“`
- Load from Text Files: Use `loadtxt` or `genfromtxt` for loading text files:
“`python
data = np.loadtxt(‘path/to/your/file.txt’, delimiter=’,’)
“`
- Load from Binary Files: For binary files, use `load`:
“`python
data = np.load(‘path/to/your/file.npy’)
“`
Loading Datasets from Web URLs
In many cases, datasets are available online. Python can fetch these datasets directly from URLs.
- Using Pandas: You can load CSV files directly from a URL:
“`python
df = pd.read_csv(‘https://example.com/dataset.csv’)
“`
- Using Requests: For more complex data fetching, the `requests` library can be useful:
“`python
import requests
url = ‘https://example.com/dataset.json’
response = requests.get(url)
data = response.json()
“`
Common Parameters for Loading Functions
When loading datasets, several parameters can enhance the process. Below is a table of common parameters used in Pandas’ `read_csv` function:
Parameter | Description |
---|---|
`sep` | Specify a custom delimiter (default is ‘,’). |
`header` | Row to use as the column names; default is the first row. |
`index_col` | Column(s) to set as index; can use column names or indices. |
`usecols` | Specify which columns to read, can be a list of names or indices. |
`dtype` | Specify the data type for data or columns. |
`na_values` | Additional strings to recognize as NA/NaN. |
Utilizing these methods and parameters will facilitate efficient loading and handling of datasets in your Python projects.
Expert Insights on Loading Datasets in Python
Dr. Emily Carter (Data Scientist, Tech Innovations Inc.). “Loading a dataset in Python can be efficiently accomplished using libraries such as Pandas. It is crucial to understand the format of your data, whether it is CSV, Excel, or JSON, as each requires specific functions like `pd.read_csv()` or `pd.read_json()` for optimal performance.”
Michael Chen (Senior Software Engineer, Data Solutions Corp.). “When loading large datasets, I recommend utilizing chunking methods provided by Pandas, such as `pd.read_csv(chunksize=…)`. This approach prevents memory overload and allows for more manageable data processing.”
Sarah Patel (Machine Learning Engineer, AI Research Lab). “For users dealing with diverse data sources, employing the Dask library can be advantageous. It allows for parallel computing and can handle larger-than-memory datasets, making it an excellent choice for scalable data loading in Python.”
Frequently Asked Questions (FAQs)
How can I load a CSV file in Python?
You can load a CSV file in Python using the `pandas` library with the `read_csv()` function. For example: `import pandas as pd; df = pd.read_csv(‘file.csv’)`.
What libraries are commonly used to load datasets in Python?
Common libraries include `pandas` for structured data, `numpy` for numerical data, and `openpyxl` or `xlrd` for Excel files. Each library offers specific functions for loading data.
Can I load datasets from a URL in Python?
Yes, you can load datasets from a URL using `pandas` with `read_csv()`, like this: `df = pd.read_csv(‘http://example.com/file.csv’)`. Ensure the URL points directly to the dataset.
How do I load JSON data in Python?
You can load JSON data using the `pandas` library with the `read_json()` function. For example: `df = pd.read_json(‘file.json’)` will convert the JSON data into a DataFrame.
Is it possible to load datasets from databases in Python?
Yes, you can load datasets from databases using libraries like `SQLAlchemy` or `sqlite3`. For example, you can use `pandas.read_sql()` to execute SQL queries and load the results into a DataFrame.
What should I do if my dataset has missing values?
You can handle missing values using `pandas` functions such as `dropna()` to remove them or `fillna()` to replace them with a specified value or method, depending on your analysis requirements.
Loading a dataset in Python is a fundamental skill for data analysis and machine learning. Various libraries facilitate this process, with Pandas being one of the most widely used due to its powerful data manipulation capabilities. Other libraries such as NumPy, Dask, and built-in Python functions also offer methods for loading datasets, depending on the file format and size. Understanding the specific requirements of your dataset, such as its format (CSV, Excel, JSON, etc.), is essential for selecting the appropriate loading method.
Key methods for loading datasets include using `pandas.read_csv()` for CSV files, `pandas.read_excel()` for Excel files, and `pandas.read_json()` for JSON files. Each of these functions provides various parameters to customize the loading process, such as handling missing values, specifying column types, and managing delimiters. Additionally, for larger datasets that do not fit into memory, libraries like Dask can be employed to load data in a more efficient manner.
mastering the techniques for loading datasets in Python is crucial for anyone working in data science or analytics. By leveraging the appropriate libraries and understanding the nuances of different file formats, users can efficiently prepare their data for analysis. This foundational skill not only streamlines
Author Profile
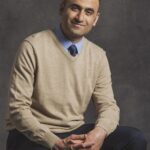
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?