How Can You Efficiently Load Data in Python?
In the ever-evolving landscape of data science and analytics, the ability to load data efficiently into Python is a foundational skill that empowers analysts, developers, and researchers alike. Whether you’re working with structured data from databases, unstructured data from text files, or streaming data from APIs, mastering the art of data loading can significantly streamline your workflow and enhance your productivity. As Python continues to dominate the programming world with its rich ecosystem of libraries and frameworks, understanding how to effectively load data is not just beneficial—it’s essential.
Loading data in Python can take many forms, depending on the source and format of the data you are working with. From CSV files and Excel spreadsheets to JSON documents and SQL databases, Python provides a variety of tools and libraries tailored for different data types. Popular libraries such as Pandas and NumPy offer powerful functionalities that simplify the process, allowing users to quickly import, manipulate, and analyze data. Additionally, Python’s versatility means that you can easily integrate data loading processes into larger applications, making it a go-to language for data-driven projects.
As you delve deeper into the intricacies of loading data in Python, you’ll discover best practices and techniques that can help you optimize performance and ensure data integrity. Whether you’re a beginner taking your first steps into data analysis or an experienced
Loading Data from CSV Files
One of the most common formats for data storage is the CSV (Comma-Separated Values) file. Python provides various libraries that simplify the process of loading CSV files, with the most popular being `pandas`.
To load data from a CSV file using pandas, follow these steps:
“`python
import pandas as pd
Load a CSV file
data = pd.read_csv(‘file_path.csv’)
“`
This command reads the CSV file and returns a DataFrame object, which is a powerful data structure for data manipulation. You can also specify parameters such as delimiter, header, and column types if your data requires it.
Key parameters for `pd.read_csv()` include:
- `sep`: The delimiter to use, default is `,`.
- `header`: Row number(s) to use as the column names.
- `dtype`: Data types for data or columns.
Loading Data from Excel Files
Excel files are another popular format for data storage. The `pandas` library also provides a straightforward method to read Excel files.
To load an Excel file, use the following command:
“`python
data = pd.read_excel(‘file_path.xlsx’, sheet_name=’Sheet1′)
“`
This reads the specified sheet from the Excel file into a DataFrame. You can adjust the `sheet_name` parameter to load different sheets or even a list of sheets.
Key parameters for `pd.read_excel()` include:
- `sheet_name`: Name or index of the sheet to read.
- `header`: Row number(s) to use as the column names.
- `usecols`: Specify which columns to read.
Loading Data from JSON Files
JSON (JavaScript Object Notation) is a lightweight data interchange format that’s easy for humans to read and write. To load JSON data in Python, you can use `pandas` as well:
“`python
data = pd.read_json(‘file_path.json’)
“`
This command will read the JSON file into a DataFrame. If your JSON file is nested, you may need to normalize it into a flat table using `json_normalize`.
Key parameters for `pd.read_json()` include:
- `orient`: The format of the JSON string (e.g., `split`, `records`).
- `typ`: Type of object to return; either `frame` or `series`.
Loading Data from Databases
Python can also connect to various databases using libraries such as `SQLAlchemy`. This allows you to load data directly from SQL databases.
To load data from a database, you can use:
“`python
from sqlalchemy import create_engine
Create a database connection
engine = create_engine(‘dialect+driver://username:password@host:port/database’)
Load data from a SQL query
data = pd.read_sql_query(‘SELECT * FROM table_name’, engine)
“`
This will execute the SQL query and return the results as a DataFrame.
Comparison of Loading Methods
Below is a summary table comparing different data loading methods in Python.
Method | Library | Common File Types |
---|---|---|
CSV | pandas | .csv |
Excel | pandas | .xlsx, .xls |
JSON | pandas | .json |
SQL Database | SQLAlchemy | SQL databases |
These methods provide a versatile set of tools to load data from various sources into Python for analysis and manipulation.
Loading Data from CSV Files
The most common format for data storage is CSV (Comma-Separated Values). Python provides several libraries to load data from CSV files efficiently.
- Using Pandas: The Pandas library simplifies the process of loading CSV files. You can install Pandas using pip if it is not already installed:
“`bash
pip install pandas
“`
To load a CSV file:
“`python
import pandas as pd
df = pd.read_csv(‘file_path.csv’)
“`
- Using the CSV Module: Python’s built-in CSV module is another option for loading CSV files.
“`python
import csv
with open(‘file_path.csv’, mode=’r’) as file:
reader = csv.reader(file)
for row in reader:
print(row)
“`
Loading Data from Excel Files
Excel files are prevalent in data analysis. The Pandas library can also read Excel files effectively.
- Using Pandas:
“`python
df = pd.read_excel(‘file_path.xlsx’, sheet_name=’Sheet1′)
“`
- Dependencies: Ensure you have the `openpyxl` or `xlrd` library installed, as Pandas relies on these for reading Excel files. Install them using:
“`bash
pip install openpyxl
“`
Loading Data from JSON Files
JSON (JavaScript Object Notation) is a widely-used format for data exchange. Loading JSON data in Python can be accomplished as follows:
- Using Pandas:
“`python
df = pd.read_json(‘file_path.json’)
“`
- Using the JSON Module: Python’s built-in JSON module is useful for more complex manipulations.
“`python
import json
with open(‘file_path.json’, ‘r’) as file:
data = json.load(file)
“`
Loading Data from SQL Databases
Python can interface with various SQL databases, allowing for efficient data retrieval.
- Using SQLAlchemy: This library provides a powerful way to connect to databases.
“`python
from sqlalchemy import create_engine
import pandas as pd
engine = create_engine(‘sqlite:///database.db’) Example for SQLite
df = pd.read_sql(‘SELECT * FROM table_name’, engine)
“`
- SQLite Example: For SQLite, the connection string differs from other databases like PostgreSQL or MySQL.
Database Type | Connection String Example |
---|---|
SQLite | `sqlite:///path_to_db.db` |
PostgreSQL | `postgresql://username:password@host/db_name` |
MySQL | `mysql+pymysql://username:password@host/db_name` |
Loading Data from APIs
Data can also be loaded from web APIs using the `requests` library.
- Using Requests:
“`python
import requests
response = requests.get(‘https://api.example.com/data’)
data = response.json() Assuming the API returns JSON
“`
This method allows for dynamic data retrieval from remote sources, making it a versatile option for data loading in Python.
Expert Insights on Loading Data in Python
Dr. Emily Chen (Data Scientist, Tech Innovations Inc.). “Loading data in Python can be efficiently accomplished using libraries such as Pandas and NumPy. These libraries provide robust methods for reading various file formats, including CSV, Excel, and JSON, allowing for seamless data manipulation and analysis.”
Michael Thompson (Senior Software Engineer, Data Solutions Group). “For optimal performance when loading large datasets, consider using the Dask library, which allows for parallel computing and can handle data that exceeds memory capacity. This approach is particularly useful in big data scenarios.”
Sarah Patel (Machine Learning Engineer, AI Research Lab). “When loading data, it is crucial to preprocess it correctly. Utilizing the ‘read_csv’ function in Pandas with appropriate parameters can help manage missing values and data types, ensuring a clean dataset for your analysis or model training.”
Frequently Asked Questions (FAQs)
How can I load CSV files in Python?
You can load CSV files in Python using the `pandas` library with the `read_csv()` function. For example: `import pandas as pd; data = pd.read_csv(‘filename.csv’)`.
What libraries are commonly used to load data in Python?
Common libraries for loading data in Python include `pandas`, `numpy`, `csv`, and `json` for structured data, as well as `sqlite3` for database access.
How do I load Excel files in Python?
To load Excel files, you can use the `pandas` library with the `read_excel()` function. Ensure you have the `openpyxl` or `xlrd` library installed for compatibility.
Can I load JSON data in Python?
Yes, you can load JSON data using the built-in `json` module. Use `import json; data = json.load(open(‘filename.json’))` to read the data into a Python object.
What is the method to load data from a SQL database in Python?
You can load data from a SQL database using the `pandas` library with the `read_sql()` function. This requires a connection object from libraries like `sqlite3` or `SQLAlchemy`.
How do I handle large datasets when loading data in Python?
For large datasets, consider using the `chunksize` parameter in `pandas` functions like `read_csv()` to load data in smaller, manageable chunks, or utilize the `dask` library for out-of-core computation.
Loading data in Python is a fundamental skill that enables data analysis, machine learning, and various data-driven applications. Python offers a variety of libraries and methods for loading data from different sources, including local files, databases, and web APIs. Common libraries used for this purpose include Pandas for structured data, NumPy for numerical data, and built-in functions for simpler file formats like CSV and JSON. Understanding the appropriate library and method for your specific data type is crucial for efficient data handling.
One of the key takeaways is the versatility of Python in handling diverse data formats. For instance, Pandas provides robust functions such as `read_csv()` and `read_excel()` for loading data from CSV and Excel files, respectively. Similarly, it supports loading data from SQL databases using `read_sql()`, which is particularly useful for integrating with relational databases. This flexibility allows users to seamlessly transition between different data sources and formats, enhancing productivity and workflow efficiency.
Moreover, it is essential to consider data preprocessing after loading. Data often requires cleaning and transformation before analysis, which can be efficiently handled using Pandas and other libraries. Techniques such as handling missing values, data type conversions, and filtering are integral to preparing data for further analysis. By mastering
Author Profile
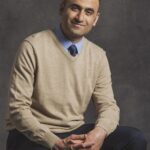
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?