How Can You Easily Load Data into Python?
Loading data into Python is a fundamental skill that opens the door to a world of possibilities in data analysis, machine learning, and scientific computing. Whether you’re a seasoned programmer or a newcomer to the realm of coding, understanding how to effectively import and manipulate data is crucial for transforming raw information into actionable insights. In today’s data-driven landscape, the ability to seamlessly integrate various data sources into your Python environment can set you apart and empower you to tackle complex problems with confidence.
In this article, we will explore the myriad ways to load data into Python, ranging from simple text files to more complex databases and web APIs. Python’s versatility is one of its greatest strengths, and with a plethora of libraries at your disposal, you’ll discover that importing data can be both straightforward and efficient. We will touch upon popular libraries such as Pandas, NumPy, and others that facilitate data loading, providing you with the tools needed to handle diverse data formats with ease.
As we delve deeper, you’ll learn about the various methods and best practices for loading data, ensuring that you can choose the right approach for your specific needs. From understanding file types to navigating data structures, this guide will equip you with the knowledge to confidently load and manipulate data in Python, paving the way for more advanced data analysis and visualization techniques
Loading Data from CSV Files
One of the most common methods for loading data into Python is through CSV (Comma-Separated Values) files. The `pandas` library simplifies this process with its `read_csv` function. This function allows you to read a CSV file and convert it into a DataFrame, which is a powerful data structure for data analysis.
To load a CSV file, you can use the following syntax:
“`python
import pandas as pd
Load data from a CSV file
df = pd.read_csv(‘file_path.csv’)
“`
Key parameters of `read_csv` include:
- `sep`: Specifies the delimiter to use; default is a comma.
- `header`: Indicates which row to use as the column names.
- `index_col`: Sets a specific column as the index of the DataFrame.
- `usecols`: Allows you to select specific columns to load.
Loading Data from Excel Files
Excel files are another prevalent data format, and `pandas` provides the `read_excel` function for this purpose. This function can read both .xls and .xlsx files.
Example code to load an Excel file:
“`python
df = pd.read_excel(‘file_path.xlsx’, sheet_name=’Sheet1′)
“`
Important parameters for `read_excel` include:
- `sheet_name`: Name or index of the sheet to read.
- `header`: Row(s) to use as the column labels.
- `usecols`: Specify which columns to load.
Loading Data from SQL Databases
If your data resides in a SQL database, you can easily load it into Python using `pandas` in combination with SQLAlchemy. First, ensure you have the necessary database driver installed.
Example code to load data from a SQL database:
“`python
from sqlalchemy import create_engine
Create a connection to the database
engine = create_engine(‘database_connection_string’)
Load data into a DataFrame
df = pd.read_sql(‘SELECT * FROM table_name’, con=engine)
“`
The `create_engine` function creates a connection to your database, allowing you to execute SQL queries directly.
Loading Data from JSON Files
JSON (JavaScript Object Notation) is a flexible data format that can also be easily loaded into Python using `pandas`. The `read_json` function is utilized for this purpose.
Here is an example of how to load a JSON file:
“`python
df = pd.read_json(‘file_path.json’)
“`
The `read_json` function can handle various formats, including:
- JSON objects
- JSON arrays
Loading Data from APIs
Loading data from APIs involves sending requests to web services and receiving data, typically in JSON format. Python’s `requests` library is often used in conjunction with `pandas` to achieve this.
Example code to load data from an API:
“`python
import requests
Send a GET request to the API
response = requests.get(‘https://api.example.com/data’)
Load the JSON data into a DataFrame
df = pd.json_normalize(response.json())
“`
This approach allows for dynamic data retrieval from online sources.
Summary of Loading Methods
The following table summarizes the methods for loading data into Python:
Data Source | Function | Library |
---|---|---|
CSV Files | pd.read_csv() | pandas |
Excel Files | pd.read_excel() | pandas |
SQL Databases | pd.read_sql() | pandas + SQLAlchemy |
JSON Files | pd.read_json() | pandas |
APIs | requests + pd.json_normalize() | requests + pandas |
Loading Data from CSV Files
CSV (Comma-Separated Values) files are one of the most common formats for data storage. Python’s `pandas` library provides a straightforward method to load data from CSV files.
To load a CSV file, use the following syntax:
“`python
import pandas as pd
data = pd.read_csv(‘file_path.csv’)
“`
Key options for `pd.read_csv()` include:
- `delimiter`: Specify a different delimiter if necessary (e.g., `delimiter=’;’`).
- `header`: Indicate the row to use as column names (default is the first row).
- `dtype`: Define data types for columns (e.g., `dtype={‘column_name’: str}`).
Loading Data from Excel Files
Excel files can also be loaded using `pandas`. The `read_excel` function allows you to read both `.xls` and `.xlsx` formats.
Example usage:
“`python
data = pd.read_excel(‘file_path.xlsx’, sheet_name=’Sheet1′)
“`
Consider the following parameters:
- `sheet_name`: Specify the sheet to load (default is the first sheet).
- `usecols`: Load specific columns (e.g., `usecols=’A:C’`).
- `skiprows`: Skip a specified number of rows before reading data.
Loading Data from JSON Files
JSON (JavaScript Object Notation) is frequently used for data interchange. The `pandas` library provides the `read_json` function for loading JSON files.
Example:
“`python
data = pd.read_json(‘file_path.json’)
“`
Important options include:
- `orient`: Define the expected format of the JSON data (e.g., `orient=’records’`).
- `lines`: Specify if the JSON file contains multiple lines (default is “).
Loading Data from SQL Databases
Python can also interface with SQL databases using the `pandas` library in conjunction with a database connector such as `sqlite3`, `SQLAlchemy`, or `MySQLdb`.
Example using SQLite:
“`python
import sqlite3
import pandas as pd
connection = sqlite3.connect(‘database.db’)
data = pd.read_sql_query(‘SELECT * FROM table_name’, connection)
connection.close()
“`
Noteworthy parameters:
- `sql`: The SQL query to execute.
- `con`: The connection object to the database.
Loading Data from APIs
Many applications provide APIs to access their data. Python can retrieve data from APIs using the `requests` library, followed by parsing the data with `pandas`.
Example:
“`python
import requests
import pandas as pd
response = requests.get(‘https://api.example.com/data’)
data = pd.json_normalize(response.json())
“`
Key considerations:
- Ensure to handle authentication if required by the API.
- Check the API documentation for rate limits and data formats.
Loading Data from Text Files
Text files, including tab-delimited files, can be loaded into Python using `pandas`.
Example for a tab-delimited file:
“`python
data = pd.read_csv(‘file_path.txt’, delimiter=’\t’)
“`
Common parameters:
- `encoding`: Define the text encoding (e.g., `encoding=’utf-8’`).
- `na_values`: Specify additional strings to recognize as NA/NaN.
Loading Data from Pickle Files
For serialized data, Python’s `pickle` module can be used. This method is efficient for storing and loading complex data structures.
Example:
“`python
import pandas as pd
data = pd.read_pickle(‘file_path.pkl’)
“`
Considerations include:
- Ensure that the data being unpickled is trusted to avoid security risks.
- Pickle files are not human-readable, unlike other formats.
Expert Insights on Loading Data into Python
Dr. Emily Chen (Data Scientist, Tech Innovations Inc.). “Loading data into Python can be seamlessly achieved using libraries such as Pandas and NumPy. These libraries provide powerful functions to read various file formats, including CSV, Excel, and JSON, allowing for efficient data manipulation and analysis.”
Michael Thompson (Software Engineer, Data Solutions Group). “For optimal performance when loading large datasets into Python, consider using the Dask library. It allows for parallel computing and can handle data that exceeds memory capacity, making it an excellent choice for big data applications.”
Sarah Patel (Machine Learning Engineer, AI Research Labs). “When loading data into Python, it’s crucial to preprocess the data appropriately. Libraries like scikit-learn offer utilities to handle missing values and normalize data, ensuring that the dataset is ready for analysis or model training.”
Frequently Asked Questions (FAQs)
How can I load a CSV file into Python?
You can load a CSV file into Python using the `pandas` library with the `read_csv()` function. For example: `import pandas as pd; data = pd.read_csv(‘file.csv’)`.
What libraries are commonly used to load data in Python?
Common libraries for loading data in Python include `pandas` for structured data, `numpy` for numerical data, and `json` for JSON files. Each library provides specific functions to facilitate data loading.
Can I load Excel files into Python?
Yes, you can load Excel files using the `pandas` library with the `read_excel()` function. Ensure you have the `openpyxl` or `xlrd` library installed for compatibility.
How do I load data from a SQL database into Python?
You can load data from a SQL database using the `SQLAlchemy` library along with `pandas`. Use the `read_sql()` function to execute SQL queries and return the results as a DataFrame.
Is it possible to load data from a web API into Python?
Yes, you can load data from a web API using the `requests` library to make HTTP requests and retrieve data. After fetching the data, use `json.loads()` to convert it into a usable format.
What is the best way to load large datasets into Python?
For large datasets, consider using `dask` or `pandas` with the `chunksize` parameter in `read_csv()` to load data in smaller, manageable chunks, which helps to optimize memory usage.
Loading data into Python is a fundamental skill for data analysis, machine learning, and various other applications. There are several methods available, each suited to different data formats and sources. Common libraries such as Pandas, NumPy, and built-in Python functions provide robust tools to facilitate the import of data from CSV files, Excel spreadsheets, databases, and even web APIs. Understanding the appropriate method for your specific data type is crucial for efficient data manipulation and analysis.
One of the most widely used libraries for data loading is Pandas, which offers functions like `read_csv()`, `read_excel()`, and `read_sql()`. These functions allow users to easily load structured data into DataFrames, which are versatile data structures that enable powerful data manipulation and analysis capabilities. Additionally, NumPy provides functions for loading numerical data, while Python’s built-in capabilities can handle simpler data formats such as text files.
Moreover, it is essential to consider data preprocessing after loading, as raw data often requires cleaning and transformation before it can be effectively analyzed. Techniques such as handling missing values, data type conversions, and normalization are critical steps that follow the initial loading process. By mastering these techniques, users can ensure that their data is in the best possible shape
Author Profile
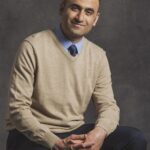
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?