How Can You Loop Through Objects in Angular with TypeScript?
In the world of web development, Angular stands out as a powerful framework that enables developers to create dynamic and responsive applications with ease. One of the essential skills every Angular developer should master is the ability to loop through objects in TypeScript. This skill not only enhances data manipulation but also enriches the user experience by allowing for dynamic content rendering. Whether you’re displaying lists of items, iterating through configuration settings, or processing data from APIs, knowing how to effectively loop through objects is crucial for building robust applications.
When working with objects in TypeScript, developers often encounter scenarios where they need to iterate over properties or values. Unlike arrays, objects can present unique challenges due to their key-value structure. However, Angular provides several powerful tools and techniques to simplify this process. From using built-in methods to leveraging Angular’s structural directives, there are multiple ways to achieve efficient looping, each with its own advantages and use cases.
As we delve deeper into this topic, we will explore various methods for looping through objects in Angular, including practical examples and best practices. Whether you’re a beginner looking to grasp the basics or an experienced developer seeking to refine your skills, understanding how to loop through objects will empower you to create more dynamic and interactive applications. Get ready to unlock the full potential of
Looping Through Objects in Angular TypeScript
Looping through objects in Angular using TypeScript can be accomplished in various ways. Understanding these methods is essential for effectively handling data structures in your application. Below are some common techniques to iterate over objects.
Using `for…in` Loop
The `for…in` loop is a traditional method used to iterate through enumerable properties of an object. It is straightforward and works well for most scenarios.
“`typescript
const person = {
name: ‘John’,
age: 30,
occupation: ‘Developer’
};
for (const key in person) {
if (person.hasOwnProperty(key)) {
console.log(`${key}: ${person[key]}`);
}
}
“`
In this example, the loop iterates over the `person` object, logging each key-value pair to the console.
Using `Object.keys()` Method
Another approach is to use the `Object.keys()` method, which returns an array of the object’s own property names. This method is particularly useful when you want to manipulate the keys or when you need to work with array methods.
“`typescript
const person = {
name: ‘John’,
age: 30,
occupation: ‘Developer’
};
Object.keys(person).forEach((key) => {
console.log(`${key}: ${person[key]}`);
});
“`
This method is more concise and leverages array methods for iteration.
Using `Object.entries()` Method
`Object.entries()` returns an array of a given object’s own enumerable string-keyed property [key, value] pairs. This method allows for easy access to both keys and values in a single iteration.
“`typescript
const person = {
name: ‘John’,
age: 30,
occupation: ‘Developer’
};
Object.entries(person).forEach(([key, value]) => {
console.log(`${key}: ${value}`);
});
“`
This approach is particularly useful when you need to perform operations that involve both keys and values simultaneously.
Using `ngFor` in Angular Templates
In Angular templates, you can also loop through objects using the `*ngFor` directive. However, `*ngFor` is typically used with arrays. To use it with objects, you can convert the object into an array using `Object.entries()` or `Object.keys()`.
“`html
“`
In your component:
“`typescript
objectEntries = Object.entries(person);
“`
This renders each key-value pair in the view, making it user-friendly and easy to implement.
Performance Considerations
When looping through objects, consider the following performance aspects:
- Object Size: The larger the object, the more performance may be impacted.
- Method Choice: Using `Object.entries()` may be more efficient than `for…in` in certain cases, as it minimizes the checks for inherited properties.
- Change Detection: In Angular, frequent updates to objects can trigger change detection, which may affect performance.
Method | Description | Use Case |
---|---|---|
for…in | Iterates over properties of an object | Simple iteration without additional processing |
Object.keys() | Returns an array of object keys | When you need to manipulate keys |
Object.entries() | Returns an array of [key, value] pairs | When both keys and values are needed |
Looping Through Objects in Angular TypeScript
In Angular, TypeScript allows for effective manipulation of objects and arrays using various methods. Below are several approaches to loop through an object.
Using `for…in` Loop
The `for…in` loop is a straightforward way to iterate over the properties of an object. This method is efficient for enumerating keys.
“`typescript
const myObject = { name: ‘John’, age: 30, city: ‘New York’ };
for (const key in myObject) {
if (myObject.hasOwnProperty(key)) {
console.log(`${key}: ${myObject[key]}`);
}
}
“`
- hasOwnProperty: Ensures that only the object’s own properties are included, excluding inherited properties.
Using `Object.keys()` Method
The `Object.keys()` method retrieves an array of a given object’s own enumerable property names, which can then be looped through using `forEach`.
“`typescript
const myObject = { name: ‘Jane’, age: 25, city: ‘Los Angeles’ };
Object.keys(myObject).forEach((key) => {
console.log(`${key}: ${myObject[key]}`);
});
“`
- Advantages: This approach allows for cleaner syntax and is particularly useful when you need to perform operations on both keys and values.
Using `Object.entries()` Method
The `Object.entries()` method returns an array of a given object’s own enumerable string-keyed property `[key, value]` pairs. This method can simplify loops that require access to both keys and values.
“`typescript
const myObject = { name: ‘Emily’, age: 22, city: ‘Chicago’ };
Object.entries(myObject).forEach(([key, value]) => {
console.log(`${key}: ${value}`);
});
“`
- Use Case: Ideal when the relationship between keys and values is needed in a concise manner.
Using `Object.values()` Method
If you only need to loop through the values of an object, `Object.values()` can be utilized. This method returns an array of the object’s own enumerable property values.
“`typescript
const myObject = { name: ‘Michael’, age: 28, city: ‘Houston’ };
Object.values(myObject).forEach((value) => {
console.log(value);
});
“`
- Application: Best suited for scenarios where only the values are of interest.
Using `forEach` with `Map` Objects
If you are working with `Map` objects, the `forEach` method provides a way to loop through key-value pairs easily.
“`typescript
const myMap = new Map([
[‘name’, ‘Sarah’],
[‘age’, 32],
[‘city’, ‘San Francisco’]
]);
myMap.forEach((value, key) => {
console.log(`${key}: ${value}`);
});
“`
- Features: Maps maintain the insertion order of the keys and allow for any type of key.
Summary Table of Looping Methods
Method | Description | Use Case |
---|---|---|
`for…in` | Loops over keys in an object | Simple property iteration |
`Object.keys()` | Returns array of keys, can use `forEach` | When keys are required |
`Object.entries()` | Returns key-value pairs for iteration | When both keys and values needed |
`Object.values()` | Returns values only for iteration | When only values are needed |
`Map.forEach()` | Iterates over `Map` key-value pairs | When using `Map` data structure |
These methods provide robust options for iterating over objects in Angular applications, enhancing code readability and maintainability.
Expert Insights on Looping Objects in Angular TypeScript
Dr. Emily Carter (Senior Software Engineer, Angular Development Group). “When looping through objects in Angular with TypeScript, it is essential to utilize the `Object.keys()` method to retrieve the keys of the object. This approach allows for clean iteration and ensures type safety, which is a cornerstone of TypeScript.”
Michael Thompson (Lead Frontend Developer, Tech Innovations Inc.). “Using the `ngFor` directive in Angular is a powerful way to loop through an array of objects. However, if you need to loop through an object itself, converting it into an array using `Object.entries()` can simplify the process and enhance readability in your templates.”
Sarah Johnson (Angular Consultant, CodeCraft Solutions). “In TypeScript, leveraging the `for…in` loop can be effective for iterating over object properties. However, it is crucial to implement type guards to ensure that the properties being accessed are indeed part of the object, thereby avoiding potential runtime errors.”
Frequently Asked Questions (FAQs)
How do I loop through an object in Angular TypeScript?
You can loop through an object in Angular TypeScript using the `Object.keys()`, `Object.values()`, or `Object.entries()` methods. For example, you can use `forEach` to iterate over the keys or values of the object.
What is the syntax for using Object.keys() in Angular TypeScript?
The syntax for using `Object.keys()` is `Object.keys(yourObject).forEach(key => { /* logic here */ });`. This retrieves an array of the object’s keys, allowing you to loop through them.
Can I use the *ngFor directive to loop through an object in Angular?
No, the `*ngFor` directive is specifically designed for iterating over arrays. To loop through an object, you must first convert it into an array format using methods like `Object.keys()`, `Object.values()`, or `Object.entries()`.
What is the difference between Object.entries() and Object.values()?
`Object.entries()` returns an array of a given object’s own enumerable string-keyed property [key, value] pairs, while `Object.values()` returns an array of the object’s own enumerable property values. Use `Object.entries()` when you need both keys and values.
How can I loop through an object and display its properties in a template?
You can create a method in your component that returns an array of the object’s entries using `Object.entries()`, then use `*ngFor` in your template to display each property. For example:
“`typescript
getEntries() {
return Object.entries(yourObject);
}
“`
In the template:
“`html
“`
Is it possible to loop through nested objects in Angular TypeScript?
Yes, you can loop through nested objects by using recursive functions or by chaining `Object.keys()` or `Object.entries()` calls. Ensure to handle each level of nesting appropriately to access the desired properties.
In Angular TypeScript, looping through objects can be accomplished using various methods, each suited for different scenarios. The most common approaches include using the `for…in` loop, the `Object.keys()`, `Object.values()`, or `Object.entries()` methods. These techniques allow developers to iterate over the properties of an object efficiently, enabling the extraction of keys, values, or both, depending on the requirements of the application.
When utilizing the `for…in` loop, it is essential to ensure that the properties being accessed are part of the object itself and not inherited from its prototype. This can be managed by using the `hasOwnProperty()` method. Alternatively, `Object.keys()` provides a straightforward way to obtain an array of an object’s own enumerable property names, which can then be iterated over using standard array methods such as `forEach()`.
Key takeaways from this discussion include the importance of understanding the structure of objects in TypeScript and the nuances of different looping methods. By selecting the appropriate method for looping through objects, developers can write cleaner, more efficient code that enhances maintainability and performance within Angular applications. As TypeScript continues to evolve, leveraging its features effectively will contribute to building robust and scalable applications
Author Profile
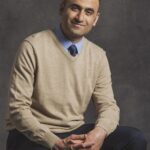
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?